Is Your Code Throwing Errors? Understanding ‘The String is Missing the Terminator’
In the world of programming, where precision and attention to detail are paramount, encountering errors can be both frustrating and enlightening. One common issue that developers face is the dreaded message: “the string is missing the terminator.” This seemingly innocuous phrase can halt progress and send even seasoned programmers into a tailspin, prompting them to sift through lines of code in search of the elusive culprit. Understanding this error is not just about fixing it; it’s about grasping the fundamental principles of how strings operate within various programming languages. In this article, we will delve into the intricacies of string terminators, explore the common pitfalls that lead to this error, and arm you with the knowledge to prevent it from derailing your coding endeavors.
When we talk about strings in programming, we are referring to sequences of characters that are used to represent text. Each programming language has its own conventions for how strings are defined and terminated, often using specific characters like quotation marks or apostrophes. A string terminator signals the end of the string, ensuring that the compiler or interpreter knows where the data stops. When this terminator is missing, it can lead to a cascade of errors that not only disrupt the flow of code but can also create vulnerabilities in software applications.
The causes of
Common Causes of Missing Terminators
One of the primary reasons for encountering the error message “the string is missing the terminator” is the improper handling of string literals in code. This issue can arise due to several factors, including:
- Unmatched Quotes: When opening and closing quotes do not match, the compiler cannot determine where the string begins and ends.
- Escaped Characters: If special characters (like quotes) are included in the string without proper escaping, it can lead to confusion in string termination.
- Multi-line Strings: In languages that do not support multi-line strings, attempting to break a string across multiple lines without concatenation may trigger this error.
- Commenting Out Code: If code is commented out incorrectly, it may unintentionally disrupt the string literal.
How to Identify Missing Terminators
To effectively identify missing terminators in your code, consider the following strategies:
- Code Review: Regularly reviewing your code can help catch errors before they become problematic.
- Syntax Highlighting: Use an Integrated Development Environment (IDE) with syntax highlighting, which can visually indicate mismatched quotes.
- Linting Tools: Incorporate linting tools that analyze your code for potential errors, including missing string terminators.
Error Type | Description | Solution |
---|---|---|
Unmatched Quotes | Opening and closing quotes do not match. | Ensure all quotes are properly matched. |
Unescaped Characters | Special characters included without escaping. | Use backslashes to escape special characters. |
Multi-line Strings | Strings broken across multiple lines improperly. | Concatenate strings or use appropriate syntax. |
Improper Comments | Comments disrupt string syntax. | Check commenting syntax. |
Best Practices for String Management
To avoid the issue of missing string terminators, adhere to these best practices:
- Consistent Quoting: Choose either single or double quotes and stick with it throughout your code.
- Use of Raw Strings: In languages that support them, raw strings can prevent the need for escaping characters.
- String Interpolation: Utilize string interpolation features where available, as they often handle terminators more gracefully.
- Testing: Regularly run tests to catch errors early in the development process.
By implementing these practices, developers can minimize the risk of encountering the “the string is missing the terminator” error and enhance the overall quality of their code.
Common Causes of Missing Terminators
The error message “the string is missing the terminator” often arises from various coding mistakes. Understanding these causes can help in effectively troubleshooting and resolving the issue.
- Unmatched Quotes: A frequent cause is the use of mismatched quotation marks. For instance:
- Using a single quote to start a string and a double quote to end it.
- Escaping Characters: Forgetting to escape special characters can lead to premature termination of strings. For example:
- In languages like JavaScript, using a backslash (`\`) before quotes within the string is crucial.
- Multi-line Strings: Improperly formatted multi-line strings can lead to this error. Ensure that:
- The syntax allows for multi-line strings, such as using backticks in JavaScript.
- Commented Code: Sometimes, a closing comment tag can accidentally terminate a string. Check for:
- Unintended comments placed within string literals.
How to Diagnose the Issue
Diagnosing the “missing terminator” error involves methodical checking of your code. Here are some steps to follow:
- Review Your Code: Carefully read through the lines of code where the error occurs.
- Check Syntax: Use a syntax checker or linter to highlight errors in string declarations.
- Test in Isolation: Isolate the problematic string by commenting out other code sections.
- Use Debugging Tools: Employ debugging tools available in your development environment to step through the code execution.
- Consult Documentation: Refer to the specific language documentation for string handling best practices.
Preventive Measures
To avoid encountering the “missing terminator” error in the future, consider implementing the following best practices:
- Consistent Quoting: Always use the same type of quotes for strings.
- Escape Characters: Familiarize yourself with the proper escaping techniques for your programming language.
- Linting Tools: Utilize linting tools to catch syntax errors before running your code.
- Code Reviews: Engage in peer reviews to get fresh eyes on your code, which can help identify overlooked issues.
- Write Unit Tests: Implement unit tests to cover string handling functions, ensuring they behave as expected.
Examples of Fixing Common Issues
Here are examples demonstrating how to resolve typical causes of the “missing terminator” error:
Issue | Code Example | Fixed Code Example |
---|---|---|
Unmatched Quotes | `let str = “Hello World’` | `let str = “Hello World”` |
Missing Escape Character | `let str = “He said, “Hello!”` | `let str = “He said, \”Hello!\””` |
Incorrect Multi-line | `let str = “This is a \n multi-line string` | `let str = `This is a \n multi-line string“ |
Comment Misplacement | `let str = “Hello” // a comment` | `let str = “Hello” /* a comment */` |
By understanding the common causes, diagnosing effectively, and employing preventive measures, developers can significantly reduce the occurrence of the “the string is missing the terminator” error in their code.
Understanding the Implications of Missing String Terminators
Dr. Emily Carter (Software Development Specialist, Tech Innovations Inc.). “The absence of a string terminator in programming languages, particularly in C and C++, can lead to behavior, memory corruption, and security vulnerabilities. It is crucial for developers to ensure proper string termination to maintain code integrity and prevent runtime errors.”
Michael Chen (Cybersecurity Analyst, SecureTech Solutions). “When strings are not properly terminated, attackers can exploit these vulnerabilities to perform buffer overflow attacks, potentially gaining unauthorized access to sensitive data. String handling must be a priority in secure coding practices to mitigate these risks.”
Laura Thompson (Lead Software Engineer, CodeSafe Technologies). “In languages that require explicit string termination, such as C, failing to include a terminator can lead to unpredictable program behavior. It is essential for developers to adopt best practices in string management to avoid these common pitfalls and ensure robust application performance.”
Frequently Asked Questions (FAQs)
What does it mean when the string is missing the terminator?
When a string is missing the terminator, it indicates that the end of the string is not properly defined, which can lead to errors in processing or interpreting the data.
What are common causes of a missing string terminator?
Common causes include syntax errors in code, such as forgetting to include a closing quotation mark, or issues with data input where the expected format is not adhered to.
How can I fix a missing string terminator error in my code?
To fix this error, review the code for any unclosed string literals and ensure that all strings are properly enclosed with matching quotation marks.
What programming languages are most affected by missing string terminator issues?
Languages such as C, C++, and Java are particularly sensitive to missing string terminators, as they rely on explicit termination to define string boundaries.
Can a missing string terminator affect program performance?
Yes, a missing string terminator can lead to behavior, crashes, or security vulnerabilities, ultimately impacting the overall performance and reliability of the program.
Are there tools available to detect missing string terminators in code?
Yes, many integrated development environments (IDEs) and static analysis tools can automatically detect syntax errors, including missing string terminators, providing developers with immediate feedback.
The phrase “the string is missing the terminator” often refers to a common programming error encountered in various coding languages. This error typically arises when a string literal is not properly closed with the appropriate delimiter, such as a quotation mark. Such oversights can lead to syntax errors, preventing the code from compiling or executing correctly. Understanding the significance of string terminators is crucial for developers to ensure their code functions as intended and to avoid unnecessary debugging time.
One of the key takeaways from this discussion is the importance of attention to detail in programming. Developers must be vigilant about syntax rules, as even minor mistakes can lead to significant issues in code execution. Additionally, utilizing integrated development environments (IDEs) that provide syntax highlighting and error detection can help mitigate these errors by alerting programmers to missing terminators and other common mistakes.
Moreover, fostering good coding practices, such as consistent formatting and thorough testing, can further reduce the likelihood of encountering such errors. By adopting a disciplined approach to coding and leveraging available tools, programmers can enhance their productivity and reduce the frustration associated with debugging string-related issues. Ultimately, understanding and addressing the implications of missing string terminators is essential for writing robust and reliable code.
Author Profile
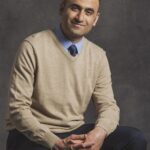
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?