What Does the Number 1 Mean in Python: Understanding Its Significance and Usage?
When diving into the world of Python programming, one of the first concepts you’ll encounter is the significance of numbers, particularly the integer `1`. While it may seem simple at first glance, the number `1` serves as a fundamental building block in Python, embodying a wealth of meaning and functionality that extends far beyond its numerical value. Understanding what `1` represents in Python not only enhances your grasp of the language but also opens the door to more complex programming concepts.
In Python, the integer `1` is more than just a digit; it plays a crucial role in various operations and data structures. As a common representation of truthiness, `1` is often associated with the boolean value `True`, influencing control flow and logical operations. Additionally, it serves as a key player in mathematical computations, enabling developers to perform arithmetic operations, iterate through loops, and manipulate data effectively. The versatility of `1` exemplifies how even the simplest elements in Python can have profound implications for coding practices.
Moreover, the significance of `1` extends into the realm of data structures, where it can denote the first index in lists or arrays, making it essential for accessing and managing data. As you progress in your Python journey, recognizing the multifaceted nature of `1` will empower
Understanding the Integer 1 in Python
In Python, the integer `1` is a fundamental data type representing a whole number. It plays various roles in programming and can be utilized in numerous contexts. Here are some important aspects of how `1` is interpreted and used in Python.
Boolean Context
In Python, the integer `1` is often associated with the boolean value `True`. This means that when `1` is used in a condition, it is evaluated as `True`, while `0` is evaluated as “. This relationship allows for intuitive boolean operations and comparisons.
- Examples:
- `if 1:` evaluates to `True`.
- `if 0:` evaluates to “.
Arithmetic Operations
The integer `1` is frequently used in arithmetic operations. It serves as a multiplicative identity, meaning that any number multiplied by `1` remains unchanged. This property is crucial in various mathematical calculations.
- Basic operations involving `1`:
- Addition: `x + 1` increments `x` by `1`.
- Multiplication: `x * 1` yields `x`.
- Exponentiation: `x ** 1` also results in `x`.
Data Structures and Iteration
In data structures, `1` can be utilized as an index or a key. It is particularly useful in lists and dictionaries. When indexing lists, Python uses zero-based indexing, so `1` refers to the second element of the list.
- Example of list indexing:
“`python
my_list = [10, 20, 30]
second_element = my_list[1] This will be 20
“`
Table of Common Uses of 1
Context | Description |
---|---|
Boolean | Represents True in conditional statements. |
Arithmetic | Identity element in addition and multiplication. |
Indexing | Refers to the second element in zero-based indexing. |
Looping | Often used to control the number of iterations. |
Loops and Control Structures
The integer `1` is also commonly used in control structures such as loops. For instance, in `for` loops, it can define the start or increment within a range.
- Example:
“`python
for i in range(1, 5): Iterates from 1 to 4
print(i)
“`
In this loop, `1` serves as the starting point, demonstrating its role in dictating the flow of execution.
Conclusion on the Role of 1
The integer `1` in Python is a versatile and essential element that serves multiple purposes across various programming scenarios. Understanding its role in boolean contexts, arithmetic operations, data structures, and control flows is crucial for effective Python programming.
Understanding the Value of 1 in Python
In Python, the integer `1` is a fundamental data type that represents a numeric value. It is often utilized in various programming contexts, including mathematical operations, logical comparisons, and control structures. Here are some critical aspects of its usage:
Numeric Data Type
- The integer `1` is classified as an integer (`int`) in Python.
- It can be used in arithmetic operations:
- Addition: `1 + 1` results in `2`
- Subtraction: `1 – 1` results in `0`
- Multiplication: `1 * 5` results in `5`
- Division: `1 / 1` results in `1.0`
Boolean Context
In Python, the integer `1` is also significant in boolean contexts. Specifically:
- `1` is treated as `True`
- `0` is treated as “
This behavior is particularly relevant in conditional statements and loops:
“`python
if 1:
print(“This will print because 1 is True.”)
“`
Data Structures
The integer `1` can be used in various data structures, such as lists and dictionaries. Examples include:
- Lists:
“`python
numbers = [1, 2, 3]
“`
- Dictionaries:
“`python
counts = {‘one’: 1, ‘two’: 2}
“`
Control Structures
In control structures, `1` can play a crucial role:
- For loop iterations:
“`python
for i in range(1, 5): Iterates from 1 to 4
print(i)
“`
- Conditional evaluations:
“`python
if x == 1:
print(“x is equal to 1”)
“`
Mathematical Functions
The integer `1` serves as a base case in several mathematical functions and algorithms:
- Factorials: The factorial of `1` is `1`.
- Powers: Any number raised to the power of `1` remains unchanged.
- Identity element in multiplication: Multiplying any number by `1` yields the original number.
Common Use Cases
The integer `1` appears frequently in programming scenarios:
- Loop counters
- Boolean flags
- Initialization of variables
Use Case | Example |
---|---|
Loop Counter | `for i in range(1, n):` |
Boolean Flag | `is_active = 1` |
Initialization | `count = 1` |
Conclusion on Integer Representation
The integer `1` is not just a numeric value but a versatile component in Python programming. Its implications span various applications, from mathematical calculations to control flow and data handling, making it an essential element for developers.
Understanding the Significance of ‘1’ in Python Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, the integer ‘1’ is not just a number; it represents a fundamental building block for data manipulation and control flow. It is often used in loops and conditionals, serving as a boolean equivalent of ‘True’ in many contexts.”
Michael Chen (Lead Python Developer, CodeMasters). “The value ‘1’ in Python is crucial for indexing. Python uses zero-based indexing, but ‘1’ is commonly used to reference the second element in a list or array, highlighting its importance in data structures.”
Sarah Thompson (Data Scientist, Analytics Hub). “When working with numerical data in Python, ‘1’ can signify a binary state or a single instance in datasets. Its utility extends to mathematical operations, where it often serves as a multiplicative identity.”
Frequently Asked Questions (FAQs)
What does the number 1 represent in Python?
The number 1 in Python represents an integer data type, which is a whole number without a decimal point. It can be used in mathematical operations and comparisons.
How can I use the number 1 in Python programming?
The number 1 can be utilized in various ways, such as performing arithmetic operations, indexing lists, or controlling loops and conditions within your code.
Is the number 1 considered a truthy value in Python?
Yes, in Python, the number 1 is considered a truthy value. This means it evaluates to True in a boolean context, while 0 evaluates to .
Can I assign the number 1 to a variable in Python?
Yes, you can assign the number 1 to a variable in Python using the assignment operator. For example, `my_variable = 1` assigns the integer 1 to the variable `my_variable`.
What is the significance of the number 1 in Python lists?
In Python lists, the number 1 can be used as an index to access the second element of the list, as indexing starts from 0. For example, `my_list[1]` retrieves the second item in `my_list`.
Are there any special functions associated with the number 1 in Python?
While there are no specific functions solely associated with the number 1, it is commonly used in functions involving mathematical calculations, such as `abs(1)` to get the absolute value or `pow(1, exponent)` to raise 1 to any power.
In Python, the number 1 is an integer that represents a fundamental numeric value. It is often used in various programming contexts, such as arithmetic operations, indexing, and control flow. As a basic data type, integers in Python can be manipulated using standard mathematical operators, making 1 a versatile component in coding practices. Its significance extends beyond mere numerical value, as it is frequently employed in loops, conditional statements, and as a placeholder in algorithms.
Moreover, the number 1 serves as a critical building block in Python’s object-oriented programming paradigm. It can be utilized in creating instances of classes, where it may represent a count or a state. Additionally, in data structures such as lists and arrays, 1 can denote the first element, given that Python uses zero-based indexing. This foundational role underscores the importance of understanding how basic numeric types function within the language.
Overall, the number 1 in Python is not just a simple integer; it embodies a range of functionalities that are essential for effective programming. Its applications span across various domains, from mathematical computations to data manipulation and algorithm development. Recognizing the versatility of the integer 1 can significantly enhance a programmer’s ability to write efficient and effective Python code.
Author Profile
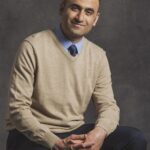
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?