Why Does My Single Row Subquery Return More Than One Row?
In the world of database management and SQL queries, the ability to retrieve and manipulate data efficiently is paramount. However, one common pitfall that developers and analysts often encounter is the notorious “single row subquery returns more than one row” error. This seemingly innocuous message can become a significant roadblock, leading to confusion and frustration. Understanding the nuances of subqueries and the implications of their results is essential for anyone looking to master SQL and optimize their data operations.
At its core, a single row subquery is designed to return only one record, typically used in scenarios where a specific value is required for comparison or filtering. When this subquery unexpectedly returns multiple rows, it triggers an error that can halt the execution of your SQL statement. This situation often arises from misunderstandings about the data structure or the logic of the query itself. As a result, developers must delve into the intricacies of their queries to identify the source of the issue and implement the necessary corrections.
Navigating the complexities of SQL requires a solid grasp of how subqueries function and the contexts in which they operate. By exploring the reasons behind this error and the strategies to resolve it, database practitioners can enhance their SQL skills and improve their overall data handling capabilities. In the following sections, we will dissect the causes
Understanding Single Row Subqueries
A single row subquery is designed to return a single value or a single row of data from a database. However, there are instances where this type of query can inadvertently return more than one row. This typically occurs due to the conditions specified in the subquery, which may not be restrictive enough to limit the results.
When a single row subquery returns multiple rows, it leads to an error in SQL execution because the query syntax expects a single result. Understanding the reasons behind this behavior can help in troubleshooting and refining your SQL queries.
Common Causes of Multiple Row Returns
Several factors can contribute to a single row subquery returning more than one row:
- Non-unique Conditions: If the subquery is based on a column that does not have unique values, it may return multiple rows. For instance, querying a table where multiple entries share the same value in the filtering column.
- Improper Use of Operators: Utilizing comparison operators such as `IN`, `ANY`, or `ALL` can also lead to situations where multiple values are returned. These operators are designed to check against a set of values, which may yield more than one result.
- Lack of Aggregation: When the subquery involves aggregate functions but does not apply a `GROUP BY` clause, it may return multiple rows if the conditions encompass more than one group.
Example Scenario
Consider the following SQL query example where the intention is to retrieve employee details based on their department’s ID:
“`sql
SELECT employee_name
FROM employees
WHERE department_id = (SELECT department_id FROM departments WHERE location_id = 1000);
“`
If the `departments` table has multiple entries for `location_id = 1000`, this will cause the subquery to return more than one `department_id`, leading to an execution error.
Best Practices to Avoid Errors
To prevent the issue of a single row subquery returning multiple rows, consider the following practices:
- Use DISTINCT: Incorporate the `DISTINCT` keyword in your subquery to ensure unique results.
- Limit Conditions: Ensure that the conditions in your subquery are specific enough to return only one row. For example, you might want to include additional filters.
- Use Aggregate Functions: If applicable, utilize aggregate functions like `MAX()`, `MIN()`, or `COUNT()` to condense results to a single value.
- Review Your Schema: Verify the uniqueness of the columns involved in the subquery to ascertain that they are designed to return a single result.
Subquery Type | Returns | Example |
---|---|---|
Single Row | 1 Row | (SELECT MAX(salary) FROM employees) |
Multiple Row | More than 1 Row | (SELECT department_id FROM departments WHERE location_id = 1000) |
By adhering to these best practices, you can mitigate the risk of encountering issues when executing single row subqueries.
Understanding Single Row Subqueries
A single row subquery is designed to return exactly one row. However, certain conditions can lead to a scenario where a subquery unexpectedly returns multiple rows. This can result in runtime errors, especially in SQL statements that expect a single value.
Common Causes of Multiple Row Returns
- Improper Filtering: The WHERE clause might not be restrictive enough, allowing more rows to be included in the result set.
- Join Conditions: If a subquery is joined with another table incorrectly, it may produce multiple rows.
- Aggregation Issues: Failing to use aggregate functions correctly can lead to multiple values being returned when only one is expected.
Examples of Problematic Queries
Consider the following SQL query:
“`sql
SELECT employee_name
FROM employees
WHERE department_id = (SELECT department_id FROM departments WHERE location = ‘New York’);
“`
If the subquery returns multiple department IDs for ‘New York’, the outer query will fail with an error.
Strategies for Resolving the Issue
To avoid errors with single row subqueries, consider the following strategies:
- Use Aggregate Functions: If appropriate, use functions like MAX(), MIN(), or COUNT() to ensure a single value is returned.
“`sql
SELECT employee_name
FROM employees
WHERE department_id = (SELECT MAX(department_id) FROM departments WHERE location = ‘New York’);
“`
- Add Additional Filters: Ensure the WHERE clause in the subquery is specific enough to return only one row.
“`sql
SELECT employee_name
FROM employees
WHERE department_id = (SELECT department_id FROM departments WHERE location = ‘New York’ AND department_name = ‘Sales’);
“`
- Use LIMIT Clause: If you need just one result, consider using a LIMIT clause in the subquery.
“`sql
SELECT employee_name
FROM employees
WHERE department_id = (SELECT department_id FROM departments WHERE location = ‘New York’ LIMIT 1);
“`
Best Practices for Subqueries
To mitigate issues with subqueries, adhere to the following best practices:
Best Practice | Description |
---|---|
Validate Subquery Output | Always check that the subquery returns a single row before using it in the main query. |
Test with Sample Data | Run queries with known data sets to ensure expected behavior. |
Utilize EXISTS Instead | When applicable, consider using EXISTS for boolean checks instead of a subquery that returns rows. |
Employing these strategies can help maintain database integrity and prevent runtime errors associated with subqueries returning multiple rows.
Understanding the Implications of Single Row Subqueries
Dr. Emily Chen (Database Architect, Tech Innovations Inc.). “When a single row subquery returns more than one row, it indicates a fundamental design flaw in the query. Such scenarios can lead to unexpected results and performance issues, as the main query cannot handle multiple values where a single value is expected.”
Mark Thompson (Senior SQL Developer, Data Solutions Group). “The occurrence of a single row subquery returning multiple rows often results from improper filtering in the WHERE clause. It is crucial to ensure that the subquery is designed to return a unique result, typically by using aggregation functions or additional conditions.”
Lisa Patel (Data Analyst, Insight Analytics). “In practice, encountering a single row subquery that returns multiple rows can lead to runtime errors. Developers should employ techniques such as EXISTS or IN clauses to handle such cases gracefully, ensuring that the main query can still execute without failure.”
Frequently Asked Questions (FAQs)
What is a single row subquery?
A single row subquery is a type of subquery that is designed to return only one row of results. It is typically used in a situation where a single value is required for comparison in the main query.
What happens if a single row subquery returns more than one row?
If a single row subquery returns more than one row, it will result in an error. Most database systems, such as SQL Server and Oracle, will throw an exception indicating that the subquery returned multiple rows when only one was expected.
How can I prevent a single row subquery from returning multiple rows?
To prevent this, you can use aggregate functions like `MAX()`, `MIN()`, or `COUNT()`, or you can add conditions to the `WHERE` clause to ensure that the subquery filters down to a single result.
What are some alternatives to single row subqueries?
Alternatives include using `JOIN` operations to combine tables or using `WITH` clauses (Common Table Expressions) to simplify complex queries. Both methods can often achieve the same results without the limitations of single row subqueries.
How can I troubleshoot a single row subquery that unexpectedly returns multiple rows?
To troubleshoot, examine the subquery’s `SELECT` statement to identify the criteria used. Ensure that the filtering conditions are sufficient to limit the results to one row. You can also run the subquery independently to see the actual results.
What error message indicates a single row subquery issue?
The error message typically states that the subquery returned more than one row. For example, in SQL Server, the message might read: “Subquery returned more than 1 value. This is not permitted when the subquery follows =, !=, <, <= , >, >= or when the subquery is used as an expression.”
The issue of a single row subquery returning more than one row is a common challenge encountered in SQL queries. A single row subquery is designed to return exactly one value, which is often used in the WHERE clause of a SQL statement to filter results based on a specific condition. When such a subquery returns multiple rows, it leads to an error, as the SQL engine cannot process more than one value in that context. Understanding the structure and constraints of subqueries is essential for effective database querying.
To address this issue, it is crucial to ensure that the subquery is constructed to return a single value. This can be achieved by using aggregate functions like COUNT, MAX, or MIN, or by applying additional filters to limit the result set. Alternatively, using the IN or EXISTS clauses can allow for multiple values to be processed without causing errors. Properly utilizing these techniques can enhance query performance and accuracy, ensuring that the intended results are achieved without runtime errors.
recognizing the limitations of single row subqueries and employing best practices in SQL query design are vital for database management. By adhering to these principles, developers can avoid common pitfalls and create more robust and efficient SQL statements. Ultimately, a thorough understanding of subquery behavior will
Author Profile
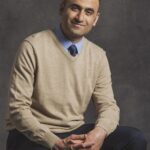
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?