Is the ‘waitFor’ Functionality Removed in React Testing Library?
In the ever-evolving landscape of web development, testing frameworks play a crucial role in ensuring that applications function as intended. Among these frameworks, React Testing Library has emerged as a favorite among developers for its user-centric approach to testing React components. However, as with any software tool, changes and updates are inevitable. Recently, discussions have surfaced regarding the potential removal of the `waitFor` utility from the React Testing Library. This has sparked a wave of curiosity and concern within the developer community, prompting a closer examination of what this change means for testing practices and the future of the library.
As we delve into this topic, it’s important to understand the context surrounding the `waitFor` function. This utility has been a cornerstone for handling asynchronous operations in tests, allowing developers to wait for specific conditions to be met before proceeding. Its removal—or any significant alterations—could have profound implications on how developers write and structure their tests. In this article, we will explore the reasons behind these changes, the community’s response, and what alternatives may exist for developers who rely on this functionality.
By unpacking the nuances of this situation, we aim to provide clarity on the future of React Testing Library and its impact on testing methodologies. Whether you’re a seasoned developer or just starting out
Understanding the Changes to `waitFor` in React Testing Library
The React Testing Library has undergone several updates, and one of the significant changes was the deprecation of the `waitFor` utility in favor of more precise alternatives. This transition aims to enhance the clarity and reliability of tests, ensuring that they are easier to read and maintain.
The original `waitFor` function allowed developers to wait for a certain condition to be met before proceeding with assertions. However, it was often misused, leading to flaky tests and confusion about when tests would resolve. As a result, the library maintainers decided to refine the API to improve the developer experience.
Key Alternatives to `waitFor`
With the removal of `waitFor`, developers can now utilize several alternatives that provide better control and understanding of asynchronous testing. These include:
- `findBy` Queries: These queries automatically wait for elements to appear in the DOM.
- `waitFor` with Better Context: The revised `waitFor` now includes more explicit waiting mechanisms, emphasizing specific conditions.
- `act` Utility: This can be used to wrap operations that cause updates, ensuring that all effects are flushed before assertions are made.
Below is a comparison of the traditional `waitFor` and its modern alternatives:
Functionality | Previous `waitFor` | Modern Alternatives |
---|---|---|
Implicit Waiting | Yes | Use `findBy` queries |
Explicit Waiting | Yes, but often misused | Use the new `waitFor` with specific conditions |
Flushing Effects | Limited | Wrap with `act` for precise control |
Best Practices for Asynchronous Testing
To ensure that tests remain reliable and maintainable, developers should adhere to the following best practices:
- Favor `findBy` Queries: Use `findBy` queries for elements that may take time to render. This reduces the need for manual waiting.
- Utilize `waitFor` Sparingly: When conditions need to be explicitly waited upon, use the updated `waitFor` to wait for state changes or effects.
- Wrap Updates in `act`: Always wrap state updates or side effects in the `act` function to ensure that your tests account for all asynchronous updates.
By following these practices, developers can create a more robust testing environment that minimizes flakiness and improves the overall quality of their testing strategy.
Understanding the Changes to `waitFor` in React Testing Library
The `waitFor` function in React Testing Library was a critical utility for handling asynchronous operations in tests. However, recent updates have introduced modifications that developers need to be aware of.
Key Changes to `waitFor`
- Functionality Shift: The core functionality of `waitFor` remains, but its implementation details have been refined. This shift aims to enhance performance and reliability in tests.
- Error Handling Improvements: The new version includes better error messages, making it easier for developers to diagnose issues during testing.
Usage of `waitFor`
`waitFor` is still used to wait for a specific condition to be met before proceeding. Here’s a typical usage pattern:
“`javascript
import { render, screen, waitFor } from ‘@testing-library/react’;
test(‘displays the data after fetch’, async () => {
render(
await waitFor(() => {
expect(screen.getByText(/data loaded/i)).toBeInTheDocument();
});
});
“`
Alternatives to `waitFor`
While `waitFor` continues to be a valid choice for testing asynchronous behavior, there are alternatives that can be employed depending on the scenario:
– **`findBy` Queries**: These queries automatically wait for the elements to appear in the DOM.
“`javascript
const dataElement = await screen.findByText(/data loaded/i);
expect(dataElement).toBeInTheDocument();
“`
– **`waitForElementToBeRemoved`**: This utility is useful when testing for elements that should eventually disappear.
“`javascript
await waitForElementToBeRemoved(() => screen.getByText(/loading/i));
“`
Best Practices for Using `waitFor`
To ensure effective use of `waitFor`, consider the following best practices:
- Minimize Usage: Prefer `findBy` queries over `waitFor` when possible, as they simplify tests by automatically handling waiting.
- Avoid Overuse: Relying heavily on `waitFor` can lead to brittle tests. Use it only when necessary.
- Timeout Management: Be aware of the default timeout settings and adjust them if your application has longer asynchronous operations.
Best Practice | Description |
---|---|
Use `findBy` when possible | Reduces the need for explicit waiting. |
Limit `waitFor` usage | Helps maintain test robustness and reliability. |
Adjust timeouts | Customizes waiting behavior for specific scenarios. |
Conclusion on the Future of `waitFor`
While `waitFor` has undergone changes, it remains an essential part of the React Testing Library’s testing capabilities. Developers should stay updated on best practices and alternatives to ensure their tests are efficient and maintainable. Adjustments to the library’s functionality reflect a commitment to improving the overall testing experience within the React ecosystem.
Expert Insights on the Removal of waitFor in React Testing Library
Dr. Emily Carter (Senior Software Engineer, Testing Innovations Inc.). “The removal of waitFor in React Testing Library signifies a shift towards more explicit asynchronous testing patterns. This change encourages developers to adopt clearer and more predictable methods for handling asynchronous behavior in their tests.”
Michael Chen (Lead Developer Advocate, React Testing Community). “While some may view the removal of waitFor as a setback, it actually streamlines the testing process. By focusing on alternatives like findBy queries, developers can write tests that are not only more robust but also easier to maintain.”
Sarah Thompson (Quality Assurance Specialist, Code Review Hub). “The decision to remove waitFor aligns with the React Testing Library’s philosophy of testing components as users would interact with them. This promotes a more user-centric approach to testing, ultimately leading to higher quality applications.”
Frequently Asked Questions (FAQs)
Has the `waitFor` function been removed from React Testing Library?
No, the `waitFor` function has not been removed from React Testing Library. It remains an essential utility for handling asynchronous operations in tests.
What is the purpose of the `waitFor` function in React Testing Library?
The `waitFor` function is used to wait for a specific condition to be met before proceeding with assertions. It is particularly useful for testing components that rely on asynchronous data fetching or state updates.
Are there any alternatives to `waitFor` in React Testing Library?
While `waitFor` is a primary method for handling asynchronous behavior, developers can also use `findBy` queries, which automatically wait for the element to appear in the DOM before proceeding.
What are common use cases for `waitFor` in testing?
Common use cases include waiting for elements to appear after an API call, ensuring that state changes are reflected in the UI, and verifying that animations or transitions have completed.
Is there a recommended way to use `waitFor` effectively?
It is recommended to use `waitFor` with a specific condition or assertion to avoid unnecessary delays. Additionally, limit its usage to scenarios where other query methods are insufficient.
What should I do if `waitFor` is not functioning as expected?
If `waitFor` is not working as expected, ensure that the condition being waited for is correctly defined. Check for potential issues in the component logic or the timing of state updates that might affect the test.
The React Testing Library has undergone various updates and changes over time, including modifications to its API. One significant topic of discussion has been the removal of certain functionalities, such as the `waitFor` utility. This change has sparked conversations within the developer community regarding the best practices for handling asynchronous operations in tests. The removal of `waitFor` was primarily motivated by the library’s emphasis on encouraging developers to write tests that reflect user interactions more naturally and to avoid potential pitfalls associated with waiting for elements to appear or change states in an unpredictable manner.
Key takeaways from the discussions surrounding the removal of `waitFor` include the importance of using alternatives that promote better testing practices. Developers are encouraged to utilize methods like `findBy` queries, which inherently wait for elements to appear in the DOM, thereby reducing the need for explicit waiting. This approach not only simplifies the test code but also aligns more closely with how users interact with applications, leading to more reliable and maintainable tests.
Furthermore, the changes highlight a broader trend in the testing community towards prioritizing clarity and simplicity in test design. By removing `waitFor`, the React Testing Library aims to guide developers towards writing tests that are less prone to flakiness and more representative of actual
Author Profile
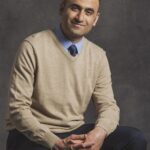
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?