How Can You Effectively Find a Missing File Dependency?
In the fast-paced world of software development, encountering missing file dependencies can feel like stumbling into a labyrinth without a map. Whether you’re a seasoned programmer or a novice coder, the frustration of a project failing to compile or run due to a missing file can be daunting. These elusive dependencies can halt progress, lead to wasted hours of troubleshooting, and even derail project timelines. However, understanding how to effectively track down these missing links can empower you to navigate through the complexities of your codebase with confidence and efficiency.
Finding a missing file dependency involves a blend of detective work and technical know-how. It often starts with identifying the symptoms of the issue, such as error messages or unexpected behaviors in your application. From there, you can employ various strategies, including checking configuration files, utilizing dependency management tools, and leveraging version control systems to trace the origins of the missing file. Each approach can provide valuable insights into the structure and requirements of your project, guiding you closer to a solution.
As you delve deeper into the process of locating missing file dependencies, you’ll discover a range of techniques and best practices that can streamline your workflow. By honing these skills, you not only resolve current issues but also equip yourself with the knowledge to prevent similar challenges in the future. So, let’s embark on this journey to
Using Dependency Management Tools
Dependency management tools play a crucial role in identifying missing file dependencies within software projects. These tools automate the tracking of file dependencies, ensuring that all required files are properly linked. Common tools include:
- Maven: Primarily used for Java projects, Maven can automatically resolve and download dependencies from a central repository.
- npm: This tool is essential for JavaScript projects, helping to manage and install packages, while also detecting missing dependencies.
- Composer: A dependency manager for PHP, Composer allows developers to declare the libraries their project depends on, and it handles the installation and updates.
Using these tools typically involves running specific commands that will scan your project for missing dependencies and automatically download them if they are available in the configured repositories.
Manual Inspection Techniques
In cases where automated tools are not available or effective, manual inspection can help identify missing file dependencies. This process may involve:
- Reviewing Project Configuration Files: Check files such as `package.json` for JavaScript projects, `pom.xml` for Maven, or `composer.json` for PHP. These files list dependencies and may indicate which files are missing.
- Examining Build Scripts: Look into build scripts (like `Makefile`, `build.gradle`, or others) to determine if any dependencies are referenced but not included.
- Cross-Referencing Documentation: Consult project documentation or README files to identify required dependencies that might not be explicitly listed in configuration files.
Utilizing Static Code Analysis
Static code analysis tools can help in detecting missing dependencies by analyzing the codebase without executing it. These tools can highlight unused imports, missing files, or incorrect references. Some popular static analysis tools include:
- SonarQube: Offers comprehensive analysis for various languages, helping to identify code quality issues and missing dependencies.
- ESLint: For JavaScript projects, ESLint can detect problems in the code, including missing imports.
- Pylint: In Python projects, Pylint can help identify missing dependencies and import errors.
Common Signs of Missing Dependencies
Identifying missing dependencies can often be inferred from specific symptoms in your code or during execution. Some common signs include:
- Compilation Errors: Errors that indicate a file cannot be found.
- Runtime Exceptions: Exceptions that arise when the application attempts to access a missing file or library.
- Unexpected Behavior: Features that do not work as expected may indicate a dependency issue.
Sign | Possible Cause |
---|---|
Compilation Error | Missing import or library |
Runtime Exception | File not found during execution |
Unexpected Behavior | Dependency not loaded or initialized |
By understanding these signs and using appropriate tools, developers can efficiently locate and resolve missing file dependencies, ensuring smoother project workflows and reducing the likelihood of critical failures.
Identifying Missing File Dependencies
Determining missing file dependencies involves a systematic approach to identify and rectify issues that may hinder application performance or functionality. Here are some strategies to effectively locate these dependencies:
- Check Error Logs: Most programming environments and platforms generate error logs that can provide insights into missing files. Review these logs for specific error messages related to file loading or dependencies.
- Use Dependency Management Tools: Tools like Maven for Java, npm for JavaScript, or Composer for PHP automatically manage file dependencies. Run commands to check for missing files:
- For npm: `npm install`
- For Maven: `mvn dependency:tree`
- For Composer: `composer install`
- Inspect Project Configuration Files: Configuration files often list dependencies explicitly. Common files include:
- `package.json` for JavaScript
- `pom.xml` for Java
- `composer.json` for PHP
Review these files to ensure all dependencies are declared.
- Static Code Analysis: Utilize static analysis tools to scan your codebase. These tools can identify references to files that do not exist. Popular tools include:
- ESLint for JavaScript
SonarQube for multiple languages
FindBugs for Java
Utilizing Development Environment Features
Development environments often include features that facilitate the identification of missing dependencies:
- IDE Error Highlighting: Integrated Development Environments (IDEs) like Visual Studio Code or IntelliJ IDEA highlight unresolved references. Hovering over highlighted code can provide additional context and potential fixes.
- Code Navigation: Use features like ‘Go to Definition’ or ‘Find Usages’ to navigate through your code. If a dependency is missing, these features may fail to locate the expected files.
- Build and Compilation Outputs: When building or compiling your project, observe the output. Missing dependencies often result in compile-time errors, which can guide you to the specific files that are unaccounted for.
Manual Dependency Tracking Techniques
In cases where automated tools do not suffice, manual tracking can be employed:
- File Search: Conduct a comprehensive search of your project directory for expected file names or extensions. Use command-line tools like `grep` or `find` in UNIX-based systems, or use the search functionality in your IDE.
- Version Control History: Examine the version control history (e.g., Git logs) for changes to dependencies. This can reveal when a file was added, modified, or removed.
- Documentation Review: Often, projects have documentation that outlines dependencies. Review README files or project wikis to verify required files.
Using Package Managers and System Tools
Package managers and system tools can assist in managing dependencies effectively:
Tool | Description |
---|---|
npm | Manages JavaScript dependencies and can install missing files. |
pip | Python’s package installer that can resolve missing packages. |
Bundler | Ruby dependency management tool for tracking gem dependencies. |
apt/yum | Linux package managers that can manage system-level dependencies. |
Utilizing these tools can streamline the process of identifying and installing missing dependencies efficiently.
Best Practices for Dependency Management
To prevent missing file dependencies in future projects, consider adopting best practices:
- Regularly Update Dependencies: Keep libraries and frameworks up to date to minimize compatibility issues.
- Use Lock Files: Employ lock files (e.g., `package-lock.json`, `Gemfile.lock`) that capture the exact versions of dependencies used.
- Establish Clear Documentation: Maintain updated documentation that includes all dependencies and their versions to facilitate onboarding and troubleshooting.
By implementing these strategies and practices, locating and managing missing file dependencies can become a more straightforward and efficient process.
Strategies for Identifying Missing File Dependencies
Dr. Emily Carter (Software Development Consultant, CodeWise Solutions). “To effectively find a missing file dependency, one should utilize dependency management tools that can analyze the project structure and highlight any unresolved references. Tools like Maven or npm can automatically identify and suggest missing dependencies, streamlining the troubleshooting process.”
Mark Thompson (Senior DevOps Engineer, TechSphere Innovations). “Implementing a robust logging system during the build process can significantly aid in identifying missing file dependencies. By reviewing the logs, developers can pinpoint the exact files that failed to load, allowing for quicker resolution of the issue.”
Linda Garcia (IT Project Manager, AgileTech Group). “Conducting a thorough code review can uncover missing file dependencies that automated tools might overlook. Engaging team members in collaborative reviews not only enhances code quality but also fosters a shared understanding of the project’s dependencies.”
Frequently Asked Questions (FAQs)
How can I identify missing file dependencies in my project?
To identify missing file dependencies, utilize tools such as dependency analyzers or package managers that can scan your project and list all required files. Review error logs or console outputs for indications of missing files during compilation or runtime.
What tools are available for tracking file dependencies?
Common tools for tracking file dependencies include npm for JavaScript projects, pip for Python, and Maven for Java. Additionally, IDEs like Visual Studio and IntelliJ IDEA offer built-in dependency management features.
How do I resolve missing dependencies once identified?
To resolve missing dependencies, locate the required files or libraries and ensure they are correctly installed in your project environment. You may need to update your configuration files or package manifests to include these dependencies.
Can version mismatches cause missing file dependencies?
Yes, version mismatches can lead to missing file dependencies. Ensure that all libraries and packages are compatible with each other and that the correct versions are specified in your project configuration.
What steps should I take if a dependency is not available in the repository?
If a dependency is not available in the repository, consider checking alternative repositories, downloading the file directly from the source, or using a different package that offers similar functionality.
How can I automate the detection of missing dependencies?
You can automate the detection of missing dependencies by integrating continuous integration (CI) tools that run dependency checks as part of the build process. Tools like Jenkins, Travis CI, or GitHub Actions can be configured to alert you of any missing files.
Finding a missing file dependency can be a challenging task, particularly in complex software projects where multiple files and libraries interact with one another. The process typically involves a systematic approach that includes identifying the specific dependency that is missing, understanding the context in which it is used, and utilizing various tools and techniques to trace and resolve the issue. This may involve checking configuration files, reviewing documentation, and leveraging debugging tools to pinpoint the root cause of the missing dependency.
One effective strategy is to utilize dependency management tools that can automatically detect and resolve missing dependencies. These tools often provide detailed logs and reports that can help developers understand which files are required and how they relate to one another. Additionally, version control systems can be beneficial in tracking changes and identifying when a dependency may have been inadvertently removed or altered.
Furthermore, collaboration and communication within development teams play a crucial role in addressing missing file dependencies. Engaging with team members can provide insights that may not be immediately apparent, and sharing knowledge about the project’s architecture can facilitate quicker resolutions. Ultimately, a proactive approach that combines the use of technology with effective teamwork will yield the best results in managing and resolving missing file dependencies.
Author Profile
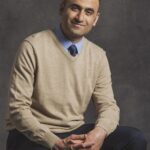
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?