How Can You Effectively Use RenderHook RTL in Your Projects?
In the ever-evolving landscape of web development, the demand for seamless user experiences has never been greater. As developers strive to create intuitive interfaces, tools that enhance efficiency and streamline workflows are invaluable. One such tool is RenderHook, a powerful feature within the React Testing Library that allows developers to manage and test component rendering with ease. For those working with Right-to-Left (RTL) languages or layouts, mastering how to use RenderHook can significantly improve the quality and accessibility of your applications.
Understanding how to utilize RenderHook in RTL contexts opens up a world of possibilities for developers. It allows for the creation of robust test cases that ensure components behave as expected, regardless of text direction. By leveraging RenderHook, developers can simulate user interactions and validate component rendering, making it easier to identify and resolve issues that may arise in RTL environments. This not only enhances the functionality of your application but also ensures that it is user-friendly for diverse audiences.
As we delve deeper into the specifics of using RenderHook with RTL, you’ll discover practical tips and techniques that can elevate your testing strategy. Whether you are a seasoned developer or just starting out, this guide will equip you with the knowledge needed to effectively implement RenderHook, ensuring your applications are both functional and accessible to all users. Prepare to
Understanding RenderHook in RTL
RenderHook is a powerful feature in React Testing Library (RTL) that allows developers to test components by rendering them in isolation. This is particularly useful for testing custom hooks or components that rely on a specific context or props. By using RenderHook, you can ensure that your components behave as expected under various conditions.
To utilize RenderHook, you must first import the necessary utilities from `@testing-library/react-hooks`. This package provides a straightforward API to render hooks and manage their lifecycle.
Basic Usage of RenderHook
The basic syntax for RenderHook involves calling the `renderHook` function, which takes a function that returns the hook you want to test. The function can also accept an options object to specify additional configurations, such as initial props or context.
Here is an example of how to use RenderHook:
“`javascript
import { renderHook } from ‘@testing-library/react-hooks’;
const { result } = renderHook(() => useCustomHook());
“`
In this example, `useCustomHook` is the custom hook being tested. The `result` object contains the current value returned by the hook, allowing you to assert its output.
Testing Hook Behavior
When testing hooks, it is crucial to verify that they return the expected values and handle state changes appropriately. You can use the `result` object to access the hook’s return value and trigger any updates as needed.
Consider the following steps when testing a hook:
– **Assert Initial Value**: Verify the initial state or return value of the hook.
– **Update State**: Simulate actions that would change the state.
– **Assert Final Value**: Check if the hook returns the expected value after state changes.
Example testing snippet:
“`javascript
const { result, rerender } = renderHook(() => useCounter());
expect(result.current.count).toBe(0); // Initial value assertion
act(() => {
result.current.increment(); // Simulate increment action
});
expect(result.current.count).toBe(1); // Final value assertion
“`
Using RenderHook with Context Providers
When your hook relies on context, you need to wrap it in the appropriate provider during testing. RenderHook allows you to pass a wrapper function to the `renderHook` options, ensuring that the hook can access the necessary context.
Example of using a context provider:
“`javascript
const wrapper = ({ children }) => (
);
const { result } = renderHook(() => useContextHook(), { wrapper });
“`
This setup ensures that `useContextHook` has access to `MyContext`, allowing for thorough testing of context-dependent logic.
Best Practices for RenderHook
- Keep Tests Isolated: Each test should focus on a single aspect of the hook’s functionality.
- Utilize `act`: Always wrap state updates in `act()` to ensure proper state management and avoid warnings.
- Cleanup: Ensure that you are cleaning up after tests to prevent memory leaks or unintended side effects.
Best Practice | Description |
---|---|
Isolation | Test one functionality at a time for clarity. |
Use `act` | Wrap updates in `act()` to manage state correctly. |
Cleanup | Ensure proper cleanup to avoid side effects. |
By following these guidelines, you can effectively use RenderHook in RTL to test your hooks, ensuring they perform as intended under various scenarios.
Understanding RenderHook in RTL
RenderHook is a powerful utility in RTL (React Testing Library) that allows developers to create custom hooks for testing React components. By leveraging RenderHook, you can encapsulate the logic of your hooks and test their behavior independently from the components that use them. This approach facilitates thorough testing and ensures that your hooks behave as expected in different scenarios.
Setting Up RenderHook
To use RenderHook, you need to install React Testing Library and its dependencies. Ensure you have the following in your project:
“`bash
npm install @testing-library/react @testing-library/react-hooks
“`
Once installed, you can import the necessary functions from the library in your test files:
“`javascript
import { renderHook } from ‘@testing-library/react-hooks’;
“`
Basic Usage
You can use RenderHook to test a custom hook by passing it as an argument to the `renderHook` function. Here’s a basic example demonstrating how to test a simple counter hook:
“`javascript
import { useCounter } from ‘./useCounter’; // Assuming you have a custom hook
test(‘should increment counter’, () => {
const { result } = renderHook(() => useCounter());
expect(result.current.count).toBe(0);
act(() => {
result.current.increment();
});
expect(result.current.count).toBe(1);
});
“`
In this example, `useCounter` is a custom hook that manages a counter state.
Advanced Features
RenderHook offers several advanced features to enhance your testing capabilities:
– **Initial Props**: You can pass initial props to your hook.
“`javascript
const { result } = renderHook(({ initialCount }) => useCounter(initialCount), {
initialProps: { initialCount: 10 }
});
“`
– **Re-rendering**: You can trigger re-renders by updating the props.
“`javascript
act(() => {
result.current.setCount(5);
});
expect(result.current.count).toBe(5);
“`
- Cleaning Up: RenderHook automatically cleans up after each test, ensuring no side effects persist.
Testing Side Effects
When your hook interacts with external resources or uses side effects, you can test them using `act`. For example, if your hook fetches data, you can mock the fetch call:
“`javascript
jest.mock(‘axios’);
test(‘should fetch data’, async () => {
axios.get.mockResolvedValue({ data: { items: [] } });
const { result, waitForNextUpdate } = renderHook(() => useFetchData());
await waitForNextUpdate();
expect(result.current.data).toEqual({ items: [] });
});
“`
This demonstrates how to handle asynchronous operations within your hooks while testing their behavior effectively.
Best Practices
To ensure efficient and effective testing of hooks with RenderHook, consider the following best practices:
- Keep Tests Isolated: Each test should focus on a single aspect of the hook’s functionality.
- Use Mocks for Dependencies: Mock external dependencies to control your hook’s environment during tests.
- Test Edge Cases: Include tests for various input scenarios to validate the hook’s robustness.
- Utilize Cleanup: Rely on RenderHook’s built-in cleanup to avoid memory leaks and unexpected behavior in your tests.
By following these guidelines, you can leverage RenderHook to create comprehensive tests for your custom hooks, enhancing your React application’s reliability and maintainability.
Expert Insights on Using RenderHook in RTL Applications
Dr. Emily Chen (Senior Software Engineer, Frontend Innovations). “When utilizing RenderHook in RTL (Right-to-Left) applications, it is crucial to ensure that your components are designed to handle text direction dynamically. This means incorporating CSS properties that support RTL layouts and testing your components thoroughly in both LTR and RTL contexts to avoid layout issues.”
Mohammed Al-Farsi (UI/UX Designer, Global Design Agency). “To effectively use RenderHook for RTL interfaces, developers should prioritize accessibility and user experience. Implementing localization strategies that account for cultural nuances in RTL languages will enhance usability. Additionally, leveraging libraries that support RTL out of the box can save time and reduce complexity.”
Lisa Patel (Technical Lead, Web Development Solutions). “Integrating RenderHook into your RTL workflow requires a solid understanding of both React hooks and the intricacies of RTL rendering. I recommend creating a dedicated context for managing direction state, which can simplify the management of component behavior and styling across different text directions.”
Frequently Asked Questions (FAQs)
What is RenderHook RTL?
RenderHook RTL is a React library that facilitates the rendering of components in a right-to-left layout, primarily used for supporting languages such as Arabic and Hebrew.
How do I install RenderHook RTL?
You can install RenderHook RTL using npm or yarn by running the command `npm install renderhook-rtl` or `yarn add renderhook-rtl` in your project directory.
How do I implement RenderHook RTL in my React application?
To implement RenderHook RTL, import the library in your component file and wrap your components with the `RenderHook` component, specifying the `direction` prop as “rtl”.
Can I customize styles when using RenderHook RTL?
Yes, you can customize styles by applying CSS classes or inline styles to the components wrapped within the `RenderHook` component, ensuring they adhere to the right-to-left layout.
Does RenderHook RTL support accessibility features?
Yes, RenderHook RTL is designed with accessibility in mind, allowing you to implement ARIA roles and properties to enhance the usability of your right-to-left layouts.
Is RenderHook RTL compatible with other React libraries?
RenderHook RTL is compatible with most React libraries, but it is advisable to test integration with specific libraries to ensure that right-to-left rendering works as expected.
In summary, utilizing RenderHook RTL (React Testing Library) effectively requires a solid understanding of both the RenderHook function and the principles of testing in a React environment. RenderHook allows developers to test custom hooks in isolation, providing a means to validate their behavior without needing to render an entire component. This approach enhances the testing process by focusing on the logic encapsulated within the hooks, ensuring that they function correctly under various scenarios.
Key takeaways from the discussion include the importance of setting up the testing environment properly, including necessary providers and context that the hook may depend on. Additionally, it is crucial to write comprehensive test cases that cover different states and side effects of the hooks to ensure robust testing. By leveraging the capabilities of RenderHook RTL, developers can streamline their testing processes, leading to more reliable and maintainable code.
Furthermore, adopting best practices such as using descriptive naming conventions for test cases and organizing tests logically can significantly improve the readability and maintainability of the test suite. Ultimately, RenderHook RTL serves as a powerful tool in the React testing arsenal, enabling developers to create high-quality applications with confidence in their custom hooks’ functionality.
Author Profile
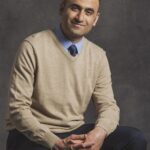
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?