How Can You Convert a Swift Array of Ints to an Int?
In the world of Swift programming, handling arrays is a fundamental skill that every developer should master. One common challenge developers face is converting an array of integers into an optional integer. This seemingly simple task can have significant implications for data handling and application logic, particularly when it comes to managing nil values and ensuring type safety. Whether you’re working on a small project or a large-scale application, understanding how to effectively perform this conversion can enhance your coding efficiency and improve the robustness of your programs.
When you think about an array of integers, it’s easy to envision a collection of values that can be manipulated and accessed with ease. However, when the need arises to convert that array into a single optional integer, the process requires careful consideration of the underlying logic. This transformation often involves deciding how to handle cases where the array may be empty or contain invalid data, leading to the potential for nil values. By mastering this conversion, you not only streamline your code but also prepare yourself for more complex data handling scenarios.
In this article, we will explore the nuances of converting an array of integers to an optional integer in Swift. We will delve into various methods, best practices, and potential pitfalls to watch out for, ensuring that you have a comprehensive understanding of this important topic. Whether you’re a
Understanding Optionals in Swift
In Swift, optionals represent a variable that can hold either a value or `nil`, indicating the absence of a value. When converting an array of integers (`[Int]`) to an array of optionals (`[Int?]`), each integer from the original array is wrapped in an optional type. This transformation is particularly useful when dealing with data that may or may not be present.
To convert an array of integers to an array of optionals, you can use the `map` function, which applies a transformation to each element in the array. Below is an example of how to perform this conversion:
“`swift
let integers: [Int] = [1, 2, 3, 4, 5]
let optionalIntegers: [Int?] = integers.map { Optional($0) }
“`
In this code, `map` iterates over each integer in the `integers` array, wrapping each integer in an `Optional` type.
Practical Example
Consider a scenario where you have an array of integers representing user IDs, and you want to prepare this data for processing that may include some optional operations. Here’s a practical illustration:
“`swift
let userIds: [Int] = [1001, 1002, 1003]
let optionalUserIds: [Int?] = userIds.map { Optional($0) }
“`
This results in the following array of optionals:
Index | Original Value | Optional Value |
---|---|---|
0 | 1001 | Optional(1001) |
1 | 1002 | Optional(1002) |
2 | 1003 | Optional(1003) |
Handling Nil Values
If there are circumstances where the integers may be `nil`, you can use the following approach to create an array that includes both integers and `nil` values:
“`swift
let mixedIntegers: [Int?] = [1, nil, 3, nil, 5]
“`
This array explicitly includes `nil` values, allowing you to represent the absence of certain entries.
Conversion Back to Int Array
To convert an array of optionals back to an array of integers, you can filter out the `nil` values using `compactMap`, which unwraps the optionals and returns only the non-nil values:
“`swift
let unwrappedIntegers: [Int] = optionalUserIds.compactMap { $0 }
“`
This results in an array containing only the integers without `nil` values.
In summary, transforming between arrays of integers and arrays of optionals in Swift is straightforward and utilizes functional programming concepts like `map` and `compactMap`. These operations enhance your ability to handle data gracefully, especially when dealing with potentially missing values.
Converting an Array of Int to Optional Int in Swift
In Swift, converting an array of integers (`[Int]`) to an optional integer (`Int?`) involves determining how to handle the array’s contents. The resulting optional integer can either be derived from a specific index of the array or determined by applying certain conditions to the array elements.
Accessing an Element Safely
To access an element of the array safely and convert it to an optional integer, you can use the following approach:
“`swift
let numbers: [Int] = [1, 2, 3, 4, 5]
let index = 2
let optionalInt: Int? = (index < numbers.count) ? numbers[index] : nil
```
In this example:
- If `index` is within the bounds of the `numbers` array, `optionalInt` will contain the value at that index.
- If `index` exceeds the bounds, `optionalInt` will be `nil`.
Using First or Last Element
To obtain the first or last element of an array as an optional integer, you can use the `first` and `last` properties of the array:
“`swift
let firstOptional: Int? = numbers.first
let lastOptional: Int? = numbers.last
“`
This method effectively returns:
- The first element of the array or `nil` if the array is empty.
- The last element of the array or `nil` if the array is empty.
Applying Conditions
You may want to convert the array to an optional integer based on specific conditions, such as returning the first even number or the sum of all elements. Here’s how you can implement this:
Finding the First Even Number
“`swift
let firstEven: Int? = numbers.first(where: { $0 % 2 == 0 })
“`
Summing All Elements
If the goal is to get the sum of all elements as an optional integer, you can do the following:
“`swift
let sum: Int? = numbers.isEmpty ? nil : numbers.reduce(0, +)
“`
Using CompactMap for Conditional Transformation
When transforming an array of integers into an optional integer based on a condition, `compactMap` can be used to filter and unwrap the values in one go:
“`swift
let optionalEvenNumbers: [Int?] = numbers.compactMap { $0 % 2 == 0 ? $0 : nil }
let firstEvenOptional: Int? = optionalEvenNumbers.first
“`
Summary of Techniques
Technique | Code Snippet | Description |
---|---|---|
Accessing by Index | `let optionalInt: Int? = (index < numbers.count) ? numbers[index] : nil` | Safely access by index. |
First Element | `let firstOptional: Int? = numbers.first` | Get the first element or `nil`. |
Last Element | `let lastOptional: Int? = numbers.last` | Get the last element or `nil`. |
Conditional First Element | `let firstEven: Int? = numbers.first(where: { $0 % 2 == 0 })` | Get the first even number or `nil`. |
Sum of Elements | `let sum: Int? = numbers.isEmpty ? nil : numbers.reduce(0, +)` | Sum of elements, returns `nil` if empty. |
Using CompactMap | `let firstEvenOptional: Int? = optionalEvenNumbers.first` | Filter and unwrap even numbers, then access first. |
These methods provide a robust way to convert arrays of integers into optional integers in Swift, allowing for flexible handling based on your application’s requirements.
Transforming Swift Arrays: Expert Insights on Converting Int to Int?
Dr. Emily Carter (Senior Software Engineer, Swift Innovations). “Converting an array of integers to an optional integer in Swift can be a nuanced process. It is essential to consider how the optional type affects error handling and the overall logic of your application. Using methods like `compactMap` can help streamline this conversion while ensuring that any nil values are effectively managed.”
Michael Tran (Lead iOS Developer, AppTech Solutions). “When dealing with an array of integers and the need to convert them into an optional integer, developers should be mindful of the implications of optionality in Swift. Utilizing a function that checks for conditions before performing the conversion can prevent runtime errors and enhance code safety.”
Sarah Lee (Swift Programming Specialist, CodeCraft Academy). “The conversion of an array of integers to an optional integer is not just a technical task but also a design consideration. It is crucial to assess whether the optional nature of the resulting integer aligns with the intended functionality of the application, particularly in terms of user experience and data integrity.”
Frequently Asked Questions (FAQs)
What is the purpose of converting an array of Int to Int?
Converting an array of Int to Int typically serves to aggregate or summarize the values, such as calculating the sum or average of the elements in the array.
How can I convert an array of Int to a single Int in Swift?
You can use the `reduce` method to convert an array of Int to a single Int. For example, `let total = array.reduce(0, +)` computes the sum of all elements in the array.
Can I convert an array of Int to an optional Int in Swift?
Yes, you can convert an array of Int to an optional Int by using a method that returns nil for an empty array. For instance, `let optionalTotal = array.isEmpty ? nil : array.reduce(0, +)`.
What happens if I try to convert an empty array of Int to Int?
If you attempt to convert an empty array to Int without handling the empty case, it will typically result in a default value (like 0) or an error, depending on the method used.
Is there a built-in function in Swift to convert an array of Int to Int?
Swift does not provide a built-in function specifically for this conversion; however, you can achieve it using higher-order functions like `reduce` or by implementing your own logic.
What are some common use cases for converting an array of Int to Int?
Common use cases include calculating totals, averages, or other statistical metrics from a collection of integers, as well as transforming data for further processing or display.
In Swift, converting an array of integers to a single integer can be achieved through various methods, each suited to different use cases. The most common approach involves utilizing the `reduce` function, which allows for the accumulation of array elements into a single value. This method is particularly useful when the goal is to concatenate the integers or perform mathematical operations that yield a single integer result.
Another approach to consider is using string manipulation. By first converting the array of integers to an array of strings, one can then join these strings together and convert the final result back to an integer. This method is effective when the intention is to form a number that represents the sequence of integers in the array, rather than performing arithmetic operations.
It is also important to consider edge cases, such as empty arrays or arrays containing negative integers, as these can affect the outcome of the conversion process. Proper error handling and validation should be implemented to ensure robustness. Overall, understanding the specific requirements of the conversion task is crucial in selecting the most appropriate method for transforming an array of integers into a single integer in Swift.
Author Profile
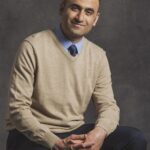
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?