How Can You Retrieve All Products from the Cart in Magento 2?
In the dynamic world of e-commerce, Magento 2 stands out as a powerful platform that enables businesses to create robust online stores. One of the essential functionalities that every online retailer must master is managing the shopping cart. Understanding how to retrieve all products from a customer’s cart not only enhances user experience but also empowers merchants to make informed decisions about inventory, promotions, and customer engagement. If you’re looking to streamline your Magento 2 operations and improve your customers’ shopping journey, knowing how to effectively access cart data is crucial.
When a customer adds items to their cart, they are expressing intent to purchase, and capturing that data is vital for any e-commerce strategy. Magento 2 provides a flexible framework that allows developers to interact with the shopping cart programmatically. By leveraging the platform’s built-in methods, you can easily retrieve all products currently in the cart, along with their details, such as quantities and prices. This capability not only aids in providing a seamless checkout experience but also helps in analyzing customer behavior and preferences.
Moreover, accessing cart information opens up a wealth of opportunities for personalized marketing and targeted promotions. By understanding what products customers are interested in, businesses can tailor their communications and offers to meet specific needs, ultimately driving conversions and enhancing customer loyalty. As we delve deeper into the methods
Accessing the Cart Object
To retrieve all products from the cart in Magento 2, the first step is to access the cart object. This can be accomplished through the `\Magento\Checkout\Model\Cart` class, which provides methods to interact with the shopping cart.
“`php
$cart = $this->cart; // Assuming $this->cart is an instance of \Magento\Checkout\Model\Cart
“`
Make sure to inject the cart model into your class constructor if you are working within a custom module or block.
Retrieving Cart Items
Once you have access to the cart object, you can retrieve the cart items using the `getQuote()->getAllItems()` method. This returns a collection of all items currently in the cart.
“`php
$items = $cart->getQuote()->getAllItems();
“`
Each item in this collection represents a product that has been added to the cart. The following attributes are typically available for each item:
- Product ID: The unique identifier for the product.
- Name: The name of the product.
- Quantity: The number of units added to the cart.
- Price: The price of the product.
Displaying Cart Item Details
You can loop through the retrieved items and extract relevant details as follows:
“`php
foreach ($items as $item) {
echo ‘Product ID: ‘ . $item->getProductId();
echo ‘Name: ‘ . $item->getName();
echo ‘Quantity: ‘ . $item->getQty();
echo ‘Price: ‘ . $item->getPrice();
}
“`
For better presentation, you may want to store this information in a structured array or directly display it in a table format.
Example Table of Cart Items
You can create a table to visually represent the items in the cart:
Product ID | Name | Quantity | Price |
---|---|---|---|
getProductId(); ?> | getName(); ?> | getQty(); ?> | getPrice(); ?> |
This table format offers a clear overview of all products in the cart, enhancing user experience and making it easier to manage cart contents.
Handling Empty Cart Situations
It is also essential to handle scenarios where the cart may be empty. Before processing the items, check if the cart has any products:
“`php
if (empty($items)) {
echo ‘Your cart is currently empty.’;
} else {
// Display items as shown above
}
“`
This check ensures that users receive appropriate feedback when no items are present in the cart, thereby improving the overall shopping experience.
Accessing the Cart Model in Magento 2
To retrieve all products from the cart in Magento 2, you first need to access the cart model. The cart model allows you to interact with the shopping cart and retrieve various details, including the items added to it.
To access the cart model, you can use dependency injection to get an instance of the `\Magento\Checkout\Model\Cart` class. Here is an example of how to do this in a custom module or controller:
“`php
use Magento\Checkout\Model\Cart as CustomerCart;
public function __construct(
CustomerCart $cart
) {
$this->cart = $cart;
}
“`
Retrieving Products from the Cart
Once you have access to the cart model, you can retrieve all items in the cart. The `getQuote()` method of the cart model provides access to the quote, which contains all the items added by the customer.
Here’s how to retrieve all products from the cart:
“`php
$quote = $this->cart->getQuote();
$items = $quote->getAllItems();
“`
This code snippet retrieves all items in the customer’s cart and stores them in the `$items` variable.
Extracting Product Information
After retrieving the cart items, you can loop through the items to extract relevant product information, such as SKU, name, price, and quantity. This can be done as follows:
“`php
foreach ($items as $item) {
$product = $item->getProduct();
echo ‘SKU: ‘ . $product->getSku() . ‘
‘;
echo ‘Name: ‘ . $product->getName() . ‘
‘;
echo ‘Price: ‘ . $item->getPrice() . ‘
‘;
echo ‘Quantity: ‘ . $item->getQty() . ‘
‘;
}
“`
This will output the SKU, name, price, and quantity of each product in the cart.
Formatting the Product Data for Display
To present the product data in a more structured format, you can create an associative array or a table. Here’s an example of organizing the product information into an array:
“`php
$productData = [];
foreach ($items as $item) {
$productData[] = [
‘SKU’ => $item->getProduct()->getSku(),
‘Name’ => $item->getProduct()->getName(),
‘Price’ => $item->getPrice(),
‘Quantity’ => $item->getQty()
];
}
“`
You can then convert this array to JSON for use in JavaScript or render it in a table format in your view:
SKU | Name | Price | Quantity |
---|---|---|---|
This structured display enhances the readability of the cart contents for both administrators and customers.
Expert Insights on Retrieving All Products from a Magento 2 Cart
Jessica Lin (E-commerce Solutions Architect, Digital Commerce Insights). “To effectively retrieve all products from a cart in Magento 2, developers should utilize the `\Magento\Checkout\Model\Session` class, which provides access to the current quote. By calling the `getQuote()` method, you can access all items in the cart, ensuring a seamless integration with your e-commerce functionalities.”
Michael Chen (Magento Certified Developer, Tech Innovations). “It is essential to understand the structure of the quote object in Magento 2. The `getAllItems()` method on the quote object returns an array of all products currently in the cart. This allows for further manipulation or display of product details as needed in your application.”
Laura Thompson (Senior Magento Consultant, E-commerce Strategies). “When working with Magento 2, leveraging the dependency injection pattern is crucial for retrieving cart products. By injecting the `\Magento\Checkout\Model\Session` into your custom module or block, you can easily access the cart items and enhance the user experience with dynamic updates to the cart contents.”
Frequently Asked Questions (FAQs)
How can I get all products from the cart in Magento 2?
To retrieve all products from the cart in Magento 2, you can use the `\Magento\Checkout\Model\Cart` class. By injecting this class into your custom module or block, you can call the `getQuote()->getAllItems()` method to obtain all items in the cart.
What is the difference between getQuote() and getItems() in Magento 2?
`getQuote()` returns the entire quote object, which includes all details about the cart, while `getItems()` directly retrieves the items in the cart. To access detailed information about the products, you should use `getQuote()->getAllItems()`.
Can I access the cart items from a custom controller in Magento 2?
Yes, you can access cart items from a custom controller by using dependency injection to include the `\Magento\Checkout\Model\Cart` class. Then, you can call the methods to get the quote and its items.
Is it possible to get cart items for a specific customer in Magento 2?
Yes, it is possible to retrieve cart items for a specific customer by loading their quote using the customer ID. Use the `\Magento\Quote\Model\QuoteFactory` to load the quote associated with the customer.
How do I format the output of cart items in Magento 2?
You can format the output of cart items by iterating through the array returned by `getAllItems()`. For each item, you can access properties like `getProductId()`, `getName()`, and `getPrice()` to display the desired information.
What should I do if I encounter issues retrieving cart items in Magento 2?
If you encounter issues, check for any custom modules that might be interfering with the cart functionality. Additionally, ensure that the Magento cache is cleared and that you are using the correct dependencies in your code.
In Magento 2, retrieving all products from the cart is a fundamental task that developers often need to perform. The process involves utilizing Magento’s built-in classes and methods to access the cart’s contents efficiently. The primary class used for this purpose is the `Quote` class, which represents the shopping cart for a customer. By leveraging the `getAllItems()` method of the `Quote` object, developers can obtain a complete list of products currently in the cart.
To implement this functionality, it is essential to first load the customer’s cart using the `QuoteFactory` or `Cart` classes. Once the cart is loaded, calling the `getAllItems()` method will return an array of `QuoteItem` objects. Each item contains detailed information about the product, such as the product ID, quantity, and price. This information can then be used for various purposes, including displaying cart contents, calculating totals, or processing checkout.
Additionally, understanding the structure of the cart data is crucial for effective manipulation and presentation. Developers should be aware of how to handle different product types, including configurable and bundled products, as these may require additional logic to retrieve associated options and selections. Overall, mastering the retrieval of cart products in Magento 2
Author Profile
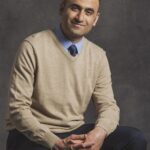
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?