How Can You Calculate the Average in Python? A Step-by-Step Guide
Calculating averages is a fundamental skill in programming, particularly in data analysis, statistics, and scientific computing. In Python, a versatile and widely-used programming language, finding the average of a set of numbers is not only straightforward but also an essential task that can unlock insights from data. Whether you’re a beginner looking to enhance your coding skills or an experienced programmer seeking to refine your techniques, understanding how to calculate averages in Python will empower you to handle numerical data with confidence and ease.
At its core, the average is a measure of central tendency that provides a summary of a dataset. In Python, there are various methods to compute the average, ranging from simple arithmetic operations to utilizing built-in libraries that streamline the process. This article will explore different approaches, catering to various levels of expertise, and highlight the advantages of each method. By grasping these techniques, you’ll be well-equipped to perform calculations that can inform decisions, drive analyses, and enhance your programming projects.
As we delve deeper into the topic, we will cover not just the mechanics of calculating averages, but also the importance of understanding data types, handling exceptions, and optimizing performance. Whether you’re working with small lists or large datasets, the principles you learn here will serve as a solid foundation for further exploration in the world
Calculating Average Using Built-in Functions
In Python, calculating the average of a list of numbers can be efficiently accomplished using built-in functions. The most common approach is to use the `sum()` function to get the total sum of the elements and the `len()` function to determine the number of elements in the list.
To compute the average, you can use the following formula:
\[ \text{Average} = \frac{\text{Sum of elements}}{\text{Number of elements}} \]
Here is a simple example:
“`python
numbers = [10, 20, 30, 40, 50]
average = sum(numbers) / len(numbers)
print(“The average is:”, average)
“`
This code snippet will output:
“`
The average is: 30.0
“`
Using NumPy for Average Calculation
For more advanced computations, especially with large datasets, the NumPy library provides a highly optimized way to calculate averages. The `numpy.mean()` function can be utilized to achieve this efficiently.
To use NumPy, you first need to install it if you haven’t done so already:
“`bash
pip install numpy
“`
Then, you can calculate the average as follows:
“`python
import numpy as np
numbers = [10, 20, 30, 40, 50]
average = np.mean(numbers)
print(“The average is:”, average)
“`
This will also yield the output:
“`
The average is: 30.0
“`
Handling Different Data Types
When calculating averages, it is essential to ensure that the data types of the elements in the list are compatible with arithmetic operations. Python handles integers and floats seamlessly, but if the list contains mixed data types, it may lead to errors or unexpected results.
Consider the following scenarios:
- All integers or floats: The calculation will proceed without issues.
- Mixed data types: You may encounter a `TypeError`.
To filter out non-numeric types, you can use a list comprehension:
“`python
numbers = [10, ’20’, 30, None, 40, 50.5]
numeric_numbers = [num for num in numbers if isinstance(num, (int, float))]
average = sum(numeric_numbers) / len(numeric_numbers) if numeric_numbers else 0
print(“The average is:”, average)
“`
Average Calculation for Large Datasets
When dealing with large datasets, performance becomes crucial. Using libraries like NumPy or pandas can significantly enhance performance due to their optimized algorithms. Below is a comparison table of methods for calculating averages:
Method | Performance | Ease of Use |
---|---|---|
Built-in Functions | Good for small datasets | Simple and straightforward |
NumPy | Excellent for large datasets | Requires additional library |
pandas | Optimal for DataFrame operations | Intuitive for data analysis |
In summary, Python provides several efficient methods to calculate averages, ranging from simple built-in functions to powerful libraries like NumPy and pandas, which are suitable for more complex data manipulation tasks.
Methods to Calculate Average in Python
Calculating the average in Python can be accomplished through various methods, depending on the data structure used and the specific requirements of the calculation. Here are some common approaches:
Using Built-in Functions
Python provides built-in functions that simplify the average calculation process. The most straightforward method is to use the `sum()` function combined with `len()`.
“`python
numbers = [10, 20, 30, 40, 50]
average = sum(numbers) / len(numbers)
print(“Average:”, average)
“`
Explanation:
- `sum(numbers)`: Computes the total sum of the elements in the list.
- `len(numbers)`: Returns the count of items in the list, which serves as the denominator.
Using the Statistics Module
For enhanced functionality and readability, Python’s `statistics` module can be utilized to calculate the average.
“`python
import statistics
numbers = [10, 20, 30, 40, 50]
average = statistics.mean(numbers)
print(“Average:”, average)
“`
Advantages:
- Readability: The code clearly expresses intent by using `mean()`.
- Additional Functions: The module includes other statistical functions like median and mode.
Using NumPy for Large Datasets
When working with large datasets, leveraging the NumPy library is beneficial due to its efficiency.
“`python
import numpy as np
numbers = np.array([10, 20, 30, 40, 50])
average = np.mean(numbers)
print(“Average:”, average)
“`
Benefits:
- Performance: NumPy is optimized for numerical operations, making it faster for large arrays.
- Versatility: Supports multi-dimensional data and provides various mathematical functions.
Handling Edge Cases
While calculating the average, certain edge cases should be considered:
- Empty List: Ensure the list is not empty to avoid division by zero.
“`python
numbers = []
if numbers:
average = sum(numbers) / len(numbers)
else:
average = 0
“`
- Non-Numeric Values: Filter out non-numeric entries if the list may contain them.
“`python
numbers = [10, ‘a’, 30, None, 50]
numeric_values = [num for num in numbers if isinstance(num, (int, float))]
average = sum(numeric_values) / len(numeric_values) if numeric_values else 0
“`
Summary of Considerations:
Edge Case | Handling Method |
---|---|
Empty List | Check if the list is populated before division |
Non-Numeric Values | Filter the list to retain only numeric entries |
Utilizing these methods allows for efficient and effective average calculations in Python, catering to various data types and structures. Proper handling of edge cases ensures robustness in your calculations.
Expert Insights on Calculating Averages in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Calculating the average in Python is straightforward, utilizing built-in functions like sum() and len(). However, for large datasets, employing libraries such as NumPy can optimize performance and efficiency.”
Michael Chen (Software Engineer, CodeCraft Solutions). “While the basic method of averaging numbers in Python is effective for small lists, leveraging the statistics module can provide additional functionalities, such as handling edge cases and improving code readability.”
Sarah Thompson (Python Instructor, LearnPython Academy). “For beginners, I recommend starting with simple lists and gradually moving to NumPy arrays. Understanding how to calculate averages in different contexts will enhance your programming skills significantly.”
Frequently Asked Questions (FAQs)
How do I calculate the average of a list of numbers in Python?
To calculate the average of a list of numbers in Python, you can use the `sum()` function to get the total and divide it by the length of the list using `len()`. For example:
“`python
numbers = [1, 2, 3, 4, 5]
average = sum(numbers) / len(numbers)
“`
Can I calculate the average of a list with non-numeric values in Python?
No, you cannot directly calculate the average of a list containing non-numeric values. You must filter out non-numeric entries or handle exceptions before performing the average calculation.
What is the difference between mean, median, and mode in Python?
The mean is the average of all numbers, the median is the middle value when numbers are sorted, and the mode is the most frequently occurring number in the dataset. You can use libraries like `statistics` to calculate these values easily.
Is there a built-in function in Python to calculate the average?
Python does not have a built-in function specifically for calculating the average. However, you can use the `mean()` function from the `statistics` module for this purpose. Example:
“`python
from statistics import mean
average = mean(numbers)
“`
How can I calculate a weighted average in Python?
To calculate a weighted average, multiply each value by its corresponding weight, sum these products, and then divide by the total of the weights. Example:
“`python
values = [10, 20, 30]
weights = [1, 2, 3]
weighted_average = sum(v * w for v, w in zip(values, weights)) / sum(weights)
“`
Can I calculate the average of a NumPy array in Python?
Yes, you can calculate the average of a NumPy array using the `mean()` method provided by the NumPy library. Example:
“`python
import numpy as np
array = np.array([1, 2, 3, 4, 5])
average = np.mean(array)
“`
Calculating the average in Python can be accomplished through various methods, depending on the context and data structure being used. The most straightforward approach involves utilizing the built-in functions such as `sum()` and `len()`, which can be applied to lists or other iterable objects. By summing the elements of a collection and dividing by the number of elements, one can easily derive the average value.
In addition to the basic method, Python also offers libraries such as NumPy, which provide more advanced capabilities for handling numerical data. NumPy’s `mean()` function is particularly useful for calculating the average of large datasets efficiently. This is especially beneficial in data analysis and scientific computing, where performance and accuracy are paramount.
Moreover, when calculating averages, it is essential to consider the type of average that is most appropriate for the dataset in question. While the arithmetic mean is the most common, other forms such as the median and mode may be more suitable in certain scenarios, particularly when dealing with skewed distributions or outliers. Understanding these distinctions can lead to more informed data analysis and interpretation.
Author Profile
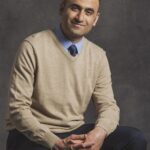
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?