How Can You Call a Function Within Another Function in Python?
In the world of Python programming, functions are the building blocks that allow developers to write clean, efficient, and reusable code. But what happens when you want to take your coding skills to the next level? Enter the concept of calling a function within another function. This powerful technique not only enhances the modularity of your code but also opens up a realm of possibilities for organizing complex tasks into manageable pieces. Whether you’re building a simple script or a sophisticated application, understanding how to effectively nest functions can significantly streamline your workflow and improve the readability of your code.
At its core, calling a function within another function allows you to leverage the capabilities of one function while executing another. This can be particularly useful in scenarios where a specific task needs to be performed multiple times or when you want to break down a larger problem into smaller, more manageable functions. By nesting functions, you can create a hierarchy of operations, making your code not only more organized but also easier to debug and maintain.
As you delve deeper into this topic, you’ll discover various strategies and best practices for implementing nested function calls in Python. From understanding the scope of variables to managing return values, mastering this technique will empower you to write more dynamic and efficient code. So, whether you’re a beginner eager to learn or an experienced developer looking
Defining Functions in Python
To call a function within another function in Python, it is essential first to define the functions correctly. A function is defined using the `def` keyword followed by the function name and parentheses. Parameters can be included within the parentheses if needed. Here’s an example of defining two functions:
“`python
def outer_function():
print(“This is the outer function.”)
def inner_function():
print(“This is the inner function.”)
“`
In this example, `outer_function` and `inner_function` are standalone functions that can be called independently.
Calling a Function Within Another Function
To call one function within another, simply use the function name followed by parentheses inside the body of the first function. For example, if you want to call `inner_function` within `outer_function`, you would do it like this:
“`python
def outer_function():
print(“This is the outer function.”)
inner_function() Calling the inner function
def inner_function():
print(“This is the inner function.”)
“`
When `outer_function` is executed, it will print its message and then call `inner_function`, displaying its message as well.
Example of Nested Function Calls
Here is a more comprehensive example that illustrates how function calls can be nested:
“`python
def greet(name):
print(f”Hello, {name}!”)
def welcome(name):
print(“Welcome to the program.”)
greet(name) Calling the greet function within welcome
Calling the welcome function
welcome(“Alice”)
“`
In this case, calling `welcome(“Alice”)` will first print “Welcome to the program.” and then invoke `greet(“Alice”)`, resulting in “Hello, Alice!” being printed as well.
Passing Arguments Between Functions
When calling a function within another, you can also pass arguments. This allows for more dynamic interactions between functions. Consider the following:
“`python
def multiply(a, b):
return a * b
def calculate_and_print(a, b):
result = multiply(a, b) Calling multiply and storing the result
print(f”The product of {a} and {b} is {result}.”)
Example usage
calculate_and_print(3, 4)
“`
Here, `calculate_and_print` calls `multiply`, passing `a` and `b` as arguments. The result is then printed.
Table of Function Calling Scenarios
The following table summarizes different scenarios for calling functions within functions:
Scenario | Description | Code Example |
---|---|---|
Basic Call | Calling a function without parameters | outer_function() |
Call with Parameters | Passing arguments to an inner function | greet("Alice") |
Return Value Usage | Using a return value from one function in another | result = multiply(a, b) |
These examples and scenarios illustrate how to effectively call functions within other functions in Python, enhancing code organization and functionality.
Defining Functions in Python
In Python, a function is defined using the `def` keyword followed by the function name and parentheses. Here’s a simple example:
“`python
def greet():
print(“Hello, World!”)
“`
This function, `greet`, when called, will output “Hello, World!” to the console.
Calling Functions Within Functions
To call a function within another function, simply invoke the inner function by its name within the body of the outer function. This technique allows for modular code and promotes reusability.
Example of Function Calling
Here is an illustration of how to call a function within another function:
“`python
def greet():
print(“Hello, World!”)
def call_greet():
greet() Calling the greet function
call_greet()
“`
When `call_greet` is invoked, it in turn calls the `greet` function, resulting in the output “Hello, World!”.
Passing Arguments Between Functions
Functions can also take parameters, allowing for more dynamic behavior. Here’s how to pass arguments:
“`python
def greet(name):
print(f”Hello, {name}!”)
def call_greet(name):
greet(name) Passing the argument to greet
call_greet(“Alice”)
“`
In this example, the `greet` function takes a parameter `name`, and `call_greet` passes the string “Alice” to it, resulting in “Hello, Alice!” being printed.
Returning Values from Functions
Functions can return values that can be used by other functions. The return value can be stored in a variable or directly used in expressions. Here’s how:
“`python
def add(a, b):
return a + b
def display_sum(x, y):
result = add(x, y) Calling add and storing the return value
print(f”The sum is: {result}”)
display_sum(5, 7)
“`
In this case, the `add` function returns the sum of `a` and `b`, which is then printed by `display_sum`.
Nesting Functions
Functions can also be defined within other functions, creating a nested structure. The inner function can only be called within the scope of the outer function:
“`python
def outer_function():
def inner_function():
print(“This is the inner function.”)
inner_function() Calling the inner function
outer_function()
“`
The `inner_function` is not accessible outside of `outer_function`, demonstrating encapsulation.
Using Lambda Functions
Python also supports lambda functions, which are small anonymous functions defined with the `lambda` keyword. They can be useful for short, throwaway functions:
“`python
def outer_function():
inner_function = lambda x: x * 2 Lambda function
print(inner_function(5)) Calling the lambda function
outer_function()
“`
In this example, `inner_function` doubles the input value when called.
Best Practices
When calling functions within functions, consider the following best practices:
- Clarity: Ensure that function names are descriptive to clarify their purpose.
- Modularity: Keep functions focused on a single task to enhance readability and maintainability.
- Avoid Side Effects: Minimize changes to global state within functions to prevent unintended consequences.
By adhering to these principles, the code will be more understandable and easier to maintain.
Expert Insights on Calling Functions Within Functions in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, calling a function within another function is straightforward and enhances code modularity. It allows for better organization of code and promotes reusability, which is essential in large-scale applications.”
Michael Chen (Python Developer, CodeCraft Solutions). “When nesting function calls in Python, it’s crucial to ensure that the inner function is defined before it is called. This practice prevents runtime errors and maintains the logical flow of the program.”
Sarah Patel (Lead Instructor, Python Programming Academy). “Utilizing functions within functions can lead to cleaner and more efficient code. However, developers should be cautious of scope issues, as variables defined in the inner function are not accessible outside of it, which can lead to confusion if not managed properly.”
Frequently Asked Questions (FAQs)
How do I define a function within another function in Python?
To define a function within another function in Python, simply declare the inner function using the `def` keyword inside the outer function. This allows the inner function to be scoped to the outer function.
Can I call the inner function from outside the outer function?
No, the inner function cannot be called from outside the outer function. It is only accessible within the scope of the outer function where it is defined.
What is the purpose of calling a function within a function?
Calling a function within a function helps to encapsulate functionality, promotes code reuse, and organizes code logically. It allows for better management of complex operations.
Can I pass arguments to the inner function?
Yes, you can pass arguments to the inner function just like any other function. The inner function can accept parameters defined in its own signature.
What happens to the inner function after the outer function executes?
Once the outer function completes execution, the inner function is no longer accessible. It is discarded as it is defined within the scope of the outer function.
Can I return a value from the inner function to the outer function?
Yes, you can return a value from the inner function. To do so, you can call the inner function and return its result from the outer function, allowing the outer function to use that value.
In Python, calling a function within another function is a straightforward process that enhances the modularity and readability of code. This practice allows developers to break down complex tasks into smaller, manageable components. By defining a function and then invoking it inside another function, one can effectively organize code, reduce redundancy, and improve maintainability. This technique is particularly useful in scenarios where a specific task needs to be performed multiple times or when a function serves as a helper for another function.
When calling a function within another function, it is essential to ensure that the inner function is defined before it is called. This can be achieved by placing the inner function definition above the outer function or by defining both functions in the same scope. Additionally, parameters can be passed to the inner function, allowing for greater flexibility and functionality. This capability enables developers to create dynamic and responsive applications that can handle a variety of input scenarios.
calling functions within functions in Python is a fundamental programming technique that promotes code organization and efficiency. By leveraging this approach, developers can create cleaner, more structured code that is easier to debug and extend. Understanding how to implement this concept effectively is crucial for anyone looking to enhance their Python programming skills and develop robust applications.
Author Profile
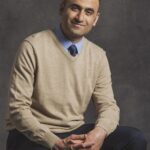
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?