How Can You Resolve PHP 8 and Old Laravel Dependency Errors with Composer?
In the fast-evolving world of web development, staying current with the latest technologies is essential for building robust applications. However, upgrading to newer versions of PHP, like PHP 8, can sometimes lead to unexpected challenges, especially when dealing with legacy frameworks such as older versions of Laravel. Developers often find themselves facing dependency conflicts that can halt progress and create frustration. In this article, we’ll explore the intricacies of resolving these issues, ensuring that your transition to PHP 8 is as smooth as possible.
As you embark on the journey to upgrade your Laravel application, understanding the nuances of Composer and its role in managing dependencies becomes crucial. Composer, the dependency management tool for PHP, is designed to streamline the process of integrating various libraries and frameworks. However, when old Laravel dependencies are involved, conflicts may arise due to incompatibilities with PHP 8 features. This can lead to a tangled web of errors that can be daunting to untangle.
Navigating these dependency errors requires a strategic approach. Developers must assess their current package versions, identify which dependencies are incompatible, and explore potential solutions that allow for a seamless upgrade. By leveraging Composer’s powerful capabilities and understanding the underlying issues, you can effectively resolve these conflicts and ensure that your application remains up-to-date and functional
Understanding Dependency Conflicts
When using Composer to manage dependencies in a Laravel project, conflicts may arise, especially when integrating PHP 8 with older packages. These conflicts often stem from version constraints specified in the `composer.json` file. It’s essential to understand how these constraints work to effectively troubleshoot and resolve issues.
Dependencies can have strict version requirements, which may not align with the newer PHP version. Here are some common reasons for conflicts:
- Incompatible Package Versions: Some Laravel packages may not yet support PHP 8, leading to conflicts.
- Transitive Dependencies: A package may depend on another package that has its own version constraints.
- Deprecated Features: Older packages might use features that have been deprecated or removed in PHP 8.
Resolving Dependency Issues
To resolve dependency issues, you can take several approaches. Here are recommended steps to guide you through the process:
- Check Current Dependencies: Use the command `composer show` to list all installed packages and their versions.
- Update Composer: Run `composer update` to see if newer versions of packages are available that support PHP 8.
- Modify Version Constraints: Edit the `composer.json` file to adjust the version constraints for the problematic packages.
For example, if a package requires an older PHP version, you can specify the desired version range:
“`json
“require”: {
“package/name”: “^1.0 || ^2.0”
}
“`
- Use Composer’s Conflict Resolution: You can explicitly state conflicts in your `composer.json` to avoid installing incompatible packages:
“`json
“conflict”: {
“package/old-package”: “*”
}
“`
- Utilize the `composer why` Command: This command helps you understand why a specific package is installed and which package depends on it, guiding your decisions on resolution.
Example of Resolving a Conflict
Consider a scenario where you receive an error related to a package that is incompatible with PHP 8. Here’s a structured approach:
Step | Command/Action | Result |
---|---|---|
Check Installed | `composer show` | Lists all packages and versions |
Update Packages | `composer update` | Updates all packages to latest versions |
Modify Constraints | Edit `composer.json` | Adjust version constraints |
Check Conflicts | `composer why package/old-package` | Identifies dependencies causing issues |
By following these steps, you can systematically address and resolve conflicts that arise when working with PHP 8 and older Laravel dependencies.
Understanding Dependency Resolution Issues in Laravel with PHP 8
When working with Laravel applications, especially older versions, upgrading to PHP 8 can introduce several dependency resolution issues due to incompatibilities. Laravel’s packages often rely on specific PHP versions or other packages that may not yet support PHP 8. Here are some common causes and solutions.
Common Causes of Dependency Errors
- Outdated Packages: Many Laravel packages may not be updated to support PHP 8, leading to conflicts.
- Incompatible PHP Features: PHP 8 introduces features such as union types and named arguments, which may conflict with older package implementations.
- Version Constraints: Composer may enforce strict version constraints that limit the installation of compatible packages.
Steps to Resolve Dependency Errors
- Update Composer: Ensure you are using the latest version of Composer. Run the following command:
“`bash
composer self-update
“`
- Check Laravel and Package Versions: Verify that your Laravel version and its dependencies are compatible with PHP 8. You can find this information in the `composer.json` file and the package’s documentation.
- Use the `composer why-not` Command: This command helps identify which package is preventing an upgrade. For example:
“`bash
composer why-not vendor/package-name
“`
- Update or Replace Packages: If certain packages are outdated:
- Run:
“`bash
composer outdated
“`
- Update packages using:
“`bash
composer update vendor/package-name
“`
- If a package is not maintained, consider finding an alternative that supports PHP 8.
- Modify Version Constraints: Sometimes, adjusting the version constraints in your `composer.json` can help:
“`json
“require”: {
“vendor/package”: “^1.0 || ^2.0”
}
“`
Example of Composer.json Adjustments
Package | Required Version | Notes |
---|---|---|
laravel/framework | ^8.0 | Ensure you are on at least Laravel 8 for PHP 8 support. |
phpunit/phpunit | ^9.0 | PHPUnit 9+ is compatible with PHP 8. |
guzzlehttp/guzzle | ^7.0 | Guzzle 7+ supports PHP 8. |
Using Composer Flags for Compatibility
When updating packages, you can use various Composer flags to manage compatibility:
- `–ignore-platform-reqs`: Temporarily ignores PHP version requirements.
“`bash
composer update –ignore-platform-reqs
“`
- `–with-all-dependencies`: Updates packages and their dependencies.
“`bash
composer update –with-all-dependencies
“`
- `–prefer-lowest`: Useful for testing compatibility with the lowest versions of dependencies.
“`bash
composer update –prefer-lowest
“`
Testing and Validation
After making changes, ensure to:
- Run Tests: Execute your test suite to confirm that the application behaves as expected.
- Check for Deprecated Features: Review the codebase for any deprecated features that may be removed in PHP 8.
By following these steps and considerations, you can effectively manage and resolve dependency issues when upgrading to PHP 8 in an older Laravel environment.
Addressing Composer Resolve Issues with PHP8 and Legacy Laravel Dependencies
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When dealing with Composer resolve issues in PHP8, especially with older Laravel dependencies, it’s crucial to first ensure that all your packages are compatible with PHP8. Running `composer why-not` can provide insights into conflicting packages and help you identify which dependencies need updating or replacing.”
Mark Thompson (Lead PHP Developer, CodeCraft Solutions). “One effective approach to resolving dependency errors is to incrementally update your Laravel packages. Start with the core framework and gradually move to other dependencies. This method allows you to isolate issues and ensures that you maintain compatibility with PHP8 throughout the process.”
Lisa Nguyen (Open Source Contributor and Laravel Specialist). “It’s important to leverage the community resources available for Laravel. Many developers have faced similar issues with PHP8 and older versions of Laravel. Checking forums, GitHub issues, and community discussions can often reveal workarounds or patches that can help you resolve dependency conflicts effectively.”
Frequently Asked Questions (FAQs)
What causes the PHP 8 and old Laravel dependency error?
The error typically arises due to compatibility issues between PHP 8 and older versions of Laravel or its packages, which may not support the new features or deprecations introduced in PHP 8.
How can I check which Laravel version is compatible with PHP 8?
You can consult the official Laravel documentation or the release notes for each version. Laravel 8 and later versions are designed to be compatible with PHP 8.
What steps should I take to resolve the dependency error in Composer?
You should first update your Laravel version to a compatible one. If that’s not feasible, consider downgrading PHP to a version supported by your current Laravel setup. Additionally, check for updated packages that may resolve the conflicts.
Can I use Composer to automatically resolve these dependency issues?
Composer can attempt to resolve dependencies automatically using the `composer update` command. However, it may not always succeed if there are significant incompatibilities between your PHP version and the Laravel dependencies.
Are there any specific commands to diagnose dependency issues in Composer?
Yes, you can use `composer diagnose` to identify potential issues in your setup. Additionally, `composer why-not
What should I do if I cannot resolve the dependency error?
If the error persists, consider seeking help from the Laravel community forums or Stack Overflow. Providing detailed error messages and your current setup can facilitate better assistance.
In the context of resolving dependency issues with Composer when upgrading to PHP 8 and dealing with older Laravel dependencies, it is crucial to understand the compatibility between the PHP version and the Laravel packages in use. Many older Laravel packages may not be compatible with PHP 8, leading to conflicts that can prevent successful installation or updates. It is essential to review the package documentation and the Laravel version requirements to identify any potential incompatibilities.
One effective strategy is to check for updated versions of the Laravel packages that support PHP 8. Often, package maintainers will release updates to ensure compatibility with newer PHP versions. If an update is not available, consider looking for alternative packages that offer similar functionality but are compatible with PHP 8. Additionally, using Composer’s `–ignore-platform-reqs` option can temporarily bypass version checks, but this should be approached with caution as it may lead to runtime issues.
Another important aspect is to ensure that your project’s dependencies are properly managed. Utilizing tools like Composer’s `composer update` and `composer require` commands can help in resolving and installing the correct versions of dependencies. It is also advisable to maintain a backup of your project before making significant changes to dependencies, as this allows for a rollback in case of
Author Profile
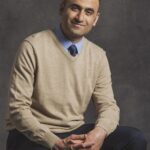
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?