How to Resolve the ‘0: Error: SOAP Struct Not Initialized’ Issue?
In the world of software development, encountering errors is an inevitable part of the journey. One particularly perplexing error that developers may face is the infamous `0: error: soap struct not initialized?`. This cryptic message can pop up unexpectedly, often leaving programmers scratching their heads and scrambling for solutions. Understanding this error is crucial for anyone working with SOAP (Simple Object Access Protocol) web services, as it can hinder the functionality of applications and lead to frustrating debugging sessions. In this article, we will delve into the intricacies of this error, exploring its causes, implications, and effective strategies for resolution.
The `soap struct not initialized` error typically arises in environments where SOAP is used to facilitate communication between web services. At its core, this error indicates that a necessary data structure, which is essential for the SOAP operations to function correctly, has not been properly initialized before being used. This can occur due to various reasons, such as misconfigured service settings, improper handling of data structures, or even issues stemming from the underlying libraries used for SOAP communication. Understanding the context in which this error occurs is vital for developers aiming to create robust and reliable applications.
As we navigate through the details of this error, we will uncover the common pitfalls that lead to its emergence and
Understanding the Error
The error message `0: error: soap struct not initialized?` typically occurs in environments utilizing SOAP (Simple Object Access Protocol) for web services. This error indicates that a data structure within the SOAP library has not been properly initialized before it is used. Understanding the context and implications of this error is crucial for developers working with SOAP-based services.
When a SOAP struct is not initialized, it may lead to several issues:
- Runtime Exceptions: Attempting to access uninitialized fields can lead to unpredictable behavior or crashes.
- Data Integrity Issues: If the struct is meant to hold important information, its uninitialized state may result in the loss of critical data.
- Debugging Complexity: Identifying the root cause may require extensive tracing of the code, adding to development time.
Common Causes
Several factors can lead to this error, including:
- Incomplete Initialization: The struct may not have been assigned a proper value or reference before use.
- Memory Management Issues: In languages where manual memory management is prevalent, improper allocation can result in uninitialized structs.
- Code Path Logic: If certain code paths skip the initialization due to conditional statements, the struct may remain uninitialized.
Resolution Strategies
To address the `soap struct not initialized?` error, developers can employ various strategies:
- Ensure Proper Initialization: Always initialize SOAP structs before use, either by using constructors or specific initialization functions.
- Implement Error Checking: Introduce validation checks to confirm that structs are initialized before any operations are performed on them.
- Review Code Paths: Thoroughly examine the logic flow in the application to ensure that all possible paths initialize the struct correctly.
Example of Struct Initialization
Here is a simplified example of how to correctly initialize a SOAP struct in a typical programming scenario:
“`c
struct soap_struct {
char *data;
int value;
};
// Initialization function
void initialize_soap_struct(struct soap_struct *s) {
s->data = malloc(256 * sizeof(char)); // Allocate memory for data
s->value = 0; // Initialize value
}
// Usage
struct soap_struct my_struct;
initialize_soap_struct(&my_struct);
“`
This code illustrates the importance of memory allocation and initialization for preventing the error.
Best Practices
To prevent encountering the `soap struct not initialized?` error in future projects, consider the following best practices:
- Always Initialize: Make it a habit to initialize all structs and variables at the point of declaration.
- Use Smart Pointers: In languages that support them, utilize smart pointers to manage memory automatically and reduce the risk of uninitialized data.
- Code Reviews: Regularly conduct code reviews focusing on initialization patterns and memory management practices.
Cause | Resolution |
---|---|
Incomplete Initialization | Ensure structs are initialized before use |
Memory Management Issues | Use dynamic memory allocation correctly |
Code Path Logic | Review and refactor code paths |
By adhering to these guidelines, developers can minimize the risk of encountering this error and enhance the robustness of their SOAP-based applications.
Understanding the Error
The error message `0: error: soap struct not initialized?` typically indicates that a SOAP (Simple Object Access Protocol) structure has not been properly initialized before it is used in a web service interaction. This can lead to various issues when attempting to send or receive messages through SOAP.
Key aspects to consider include:
- SOAP Struct Initialization: SOAP structures must be initialized to ensure they contain all necessary fields before being processed. This usually involves creating an instance of the structure and populating it with relevant data.
- Common Causes: The error can occur due to:
- Missing initialization code.
- Incorrect handling of memory or data types.
- Use of uninitialized pointers or references.
Steps to Resolve the Error
To address the `soap struct not initialized?` error, follow these steps:
- Check Initialization Code: Ensure that the SOAP struct is properly initialized before use. For example, if using C or C++, confirm that you have allocated the struct memory correctly.
Example in C:
“`c
struct soap mySoap;
soap_init(&mySoap);
“`
- Review SOAP Method Calls: Verify that all SOAP method calls are using initialized structs. If you are passing structs to functions, ensure those structs are not NULL.
- Debugging: Implement logging or debugging tools to trace the flow of data and identify where the initialization may be failing.
- Validate Input Data: Check that all required input data for the SOAP requests is present and correctly formatted.
- Consult Documentation: Reference the specific library or framework documentation to understand the expected initialization patterns for your SOAP implementation.
Best Practices for SOAP Struct Initialization
To prevent this error from occurring in the future, adopt the following best practices:
- Always Initialize Structures: Ensure that all SOAP structs are initialized before any operations.
- Use Helper Functions: Create utility functions that encapsulate struct initialization to minimize redundancy and reduce the risk of uninitialized usage.
- Error Handling: Implement robust error handling mechanisms that check for NULL or uninitialized structs before making SOAP calls.
- Unit Testing: Write unit tests that cover edge cases, ensuring that your SOAP requests handle both initialized and uninitialized states gracefully.
Example of Proper Initialization
Here’s a practical example of initializing a SOAP struct correctly:
“`c
include
include “soapH.h”
int main() {
struct soap soap_instance;
// Initialize the soap structure
soap_init(&soap_instance);
// Prepare SOAP request
struct ns__MyRequest request;
request.param1 = “value1”; // Example of setting a parameter
// Perform SOAP call
if (soap_call_ns__MyService(&soap_instance, “http://example.com/service”, “”, &request, NULL) == SOAP_OK) {
// Handle successful response
} else {
// Handle error
soap_print_fault(&soap_instance, stderr);
}
// Clean up
soap_end(&soap_instance);
soap_free(&soap_instance);
return 0;
}
“`
This code demonstrates the proper initialization of a SOAP struct and includes error handling to ensure robust application behavior.
Understanding SOAP Struct Initialization Errors
Dr. Emily Carter (Senior Software Architect, Tech Innovations Inc.). “The error ‘0: error: soap struct not initialized?’ typically arises when a SOAP struct is referenced before it has been properly instantiated. It is crucial for developers to ensure that all necessary initializations are completed before invoking any methods that rely on these structures.”
Michael Chen (Lead Developer, Cloud Solutions Group). “In my experience, this error often indicates a missing or incorrect initialization routine in the SOAP client code. Developers should review their initialization logic and ensure that all required fields in the SOAP struct are set up correctly to avoid runtime exceptions.”
Lisa Patel (Technical Consultant, Enterprise Software Solutions). “To resolve the ‘soap struct not initialized’ error, I recommend implementing thorough error handling and logging mechanisms. This approach not only helps in identifying the exact point of failure but also aids in ensuring that the struct is initialized before any operations are performed.”
Frequently Asked Questions (FAQs)
What does the error “0: error: soap struct not initialized” indicate?
This error signifies that a SOAP struct has not been properly initialized before it is used in a SOAP request or response, leading to potential null reference issues.
How can I resolve the “soap struct not initialized” error?
To resolve this error, ensure that all SOAP structs are initialized correctly before use. This typically involves calling the appropriate initialization functions or constructors for the structs in your code.
What are common causes of uninitialized SOAP structs?
Common causes include forgetting to initialize the struct, improper handling of memory allocation, or errors in the code logic that prevent initialization from occurring.
Can this error occur in both client and server SOAP implementations?
Yes, this error can occur in both client and server implementations of SOAP if the respective structs are not initialized before they are accessed or manipulated.
Is there a way to debug the “soap struct not initialized” error?
Debugging can involve checking the initialization code, using logging to track when and where structs are initialized, and employing debugging tools to step through the code execution.
Are there any best practices to prevent this error?
Best practices include always initializing structs upon declaration, implementing comprehensive error handling, and using automated testing to catch uninitialized variables early in the development process.
The error message “0: error: soap struct not initialized” typically indicates that a SOAP (Simple Object Access Protocol) structure has not been properly initialized before it is used in a web service call. This can occur in various programming environments that utilize SOAP for communication between client and server. The error suggests that the necessary memory allocation or configuration for the SOAP structure has not been performed, leading to potential failures in executing web service requests.
To resolve this issue, developers should ensure that all SOAP-related structures are initialized correctly before invoking any functions or methods that depend on them. This may involve checking the documentation for the specific SOAP library being used and following best practices for initialization. Additionally, debugging techniques such as logging and step-by-step execution can help identify where the initialization process may be failing.
In summary, the “soap struct not initialized” error serves as a reminder of the importance of proper initialization in programming. By adhering to initialization protocols and thoroughly testing code, developers can prevent such errors from occurring, thereby enhancing the reliability and robustness of their web service interactions. Understanding and addressing this error is crucial for maintaining effective communication in distributed systems that rely on SOAP.
Author Profile
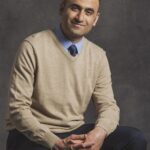
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?