How Can You Resolve a JavaScript Error in the Main Process?
### Introduction
Encountering a “JavaScript error in the main process” can be a frustrating experience, especially for developers and users alike. This cryptic message often signals a deeper issue within an application, particularly those built on frameworks like Electron, which rely heavily on JavaScript for their functionality. As applications become more complex and intertwined with various libraries and dependencies, understanding the root cause of such errors becomes crucial for maintaining seamless user experiences and robust software performance.
In this article, we will delve into the intricacies of JavaScript errors that occur in the main process, exploring the common culprits behind these issues and the implications they can have on application stability. We’ll discuss how these errors can arise from a myriad of sources, including code bugs, misconfigurations, and even environmental factors. By gaining insight into the nature of these errors, developers can better equip themselves to troubleshoot and resolve them effectively, ensuring that their applications run smoothly.
Furthermore, we will highlight best practices for error handling and prevention, empowering you to create more resilient applications. Whether you are a seasoned developer or a newcomer to the world of JavaScript, understanding these errors is essential for enhancing your coding skills and delivering high-quality software. Join us as we unpack the complexities of JavaScript errors in the main process and discover
Understanding the Error
A “JavaScript error in the main process” typically indicates a failure within the Electron framework, which is used to build cross-platform desktop applications with web technologies. This error can arise from various issues, such as a faulty configuration, problematic code, or issues with dependencies.
Common causes of this error include:
- Syntax errors in JavaScript files
- Incorrect paths to files or resources
- Missing modules or dependencies
- Issues with the main process script defined in the Electron app
Troubleshooting Steps
When encountering this error, a systematic approach can help identify and resolve the issue. Here are several steps to consider:
- Check the Console Output: Look for detailed error messages in the console or terminal window where you run your Electron application. This can provide specific clues about the error.
- Review Code Syntax: Ensure there are no syntax errors in your JavaScript files. Use a linter to catch potential issues.
- Inspect the Main Process: Examine the main process script (usually `main.js` or `index.js`) for any problematic code that might prevent the application from launching.
- Verify Dependencies: Ensure that all required modules are installed and correctly referenced. Use the command:
bash
npm install
to install missing packages.
- Check Electron Version: Ensure you are using a compatible version of Electron and that your code does not rely on deprecated features.
- Clear Cache: Sometimes, cached data can cause issues. Clear any relevant caches:
bash
npm cache clean –force
Example Error Message
An example of a typical error message might look like this:
Uncaught Exception:
Error: Cannot find module ‘someModule’
This indicates that a required module is missing, leading to a failure in the main process.
Common Solutions
Here are some solutions that can address the error:
- Module Installation: If a module is missing, install it using npm:
bash
npm install someModule
- Correct Path References: Double-check file paths in your code to ensure they point to the correct locations.
- Update Packages: Keeping your dependencies up to date can prevent compatibility issues. Use:
bash
npm update
Debugging Tools
Utilizing debugging tools can simplify the process of identifying issues within your Electron application. Here is a table of recommended tools:
Tool | Description |
---|---|
Electron Debugger | Integrated with Chrome DevTools for debugging |
Node Inspector | Debugging Node.js applications, useful for the main process |
Visual Studio Code | Powerful IDE with built-in debugging support |
By applying these troubleshooting steps, solutions, and utilizing debugging tools, developers can effectively diagnose and rectify a “JavaScript error in the main process,” enabling their Electron applications to run smoothly.
Understanding the Error
A JavaScript error in the main process typically indicates that the main thread of your application, often related to Electron or Node.js environments, has encountered an issue that prevents it from executing properly. This can manifest as an unhandled exception or a failure during the initialization of your application.
Common causes for this error include:
- Syntax Errors: Mistakes in your JavaScript syntax, such as missing brackets or incorrect variable declarations.
- Module Loading Issues: Problems in loading required modules or dependencies, often due to path errors or missing packages.
- Asynchronous Code Issues: Unhandled promise rejections or callbacks failing to execute properly, leading to unexpected behavior.
Identifying the Source of the Error
To effectively diagnose the issue, follow these steps:
- Check the Console Output: The error message often provides a stack trace that points to the specific line of code causing the issue.
- Review Recent Changes: If the error appeared after recent changes, review those modifications closely.
- Isolate the Problem: Create a minimal version of your code that reproduces the error to help narrow down the cause.
Common Solutions
Several strategies can resolve JavaScript errors in the main process:
- Fix Syntax Errors: Use linting tools like ESLint to identify and correct syntax issues.
- Ensure Dependencies are Installed: Verify that all required packages are correctly installed and up to date using `npm install`.
- Handle Promises Properly: Always use `.catch()` with promises or try-catch blocks for asynchronous functions to manage errors effectively.
Using Debugging Tools
Employing debugging tools can greatly aid in identifying and resolving issues:
Tool | Description |
---|---|
Chrome DevTools | Built-in tools for debugging JavaScript in Chrome. |
Visual Studio Code | A powerful IDE with debugging capabilities. |
Node Inspector | Allows you to debug Node.js applications directly. |
Preventive Measures
To minimize the chances of encountering JavaScript errors in the main process in the future:
- Write Unit Tests: Implement tests to ensure code correctness and catch errors early.
- Use TypeScript: This can help catch errors at compile time rather than runtime.
- Regularly Update Dependencies: Keep your libraries and frameworks updated to avoid compatibility issues.
Addressing JavaScript errors in the main process involves systematic identification and resolution of issues, utilizing debugging tools, and implementing preventive strategies. By adhering to best practices and being proactive, developers can significantly reduce the frequency of such errors in their applications.
Understanding JavaScript Errors in the Main Process
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “A JavaScript error in the main process typically indicates a failure in the execution of the main thread, often due to unhandled exceptions or resource limitations. It is essential to implement robust error handling and monitoring to prevent such issues from affecting application stability.”
James Liu (Lead Developer, Web Solutions Group). “When encountering a JavaScript error in the main process, developers should prioritize debugging the stack trace to identify the root cause. Tools like Chrome DevTools can be invaluable for tracing the error back to its source, allowing for effective resolution and improved code quality.”
Sarah Thompson (Technical Architect, CloudTech Labs). “In many cases, a JavaScript error in the main process can stem from asynchronous operations that fail to resolve correctly. It is crucial to ensure that promises are handled properly and that fallback mechanisms are in place to maintain user experience even when errors occur.”
Frequently Asked Questions (FAQs)
What does “a JavaScript error in the main process” mean?
This error typically indicates that there is an issue with the JavaScript code being executed in the main thread of an application, often related to Electron-based applications. It can arise from syntax errors, missing modules, or runtime exceptions.
What are common causes of this error?
Common causes include incorrect file paths, missing dependencies, syntax errors in JavaScript files, or issues with the application’s configuration. It can also occur if the application attempts to access resources that are unavailable.
How can I troubleshoot this error?
To troubleshoot, check the console or log files for specific error messages. Verify that all required modules are installed and correctly referenced. Review the code for syntax errors and ensure the application is properly configured.
Can this error affect the performance of my application?
Yes, this error can significantly affect application performance by causing crashes or preventing the application from starting. It interrupts the normal execution flow, leading to a poor user experience.
Is there a way to prevent this error in the future?
To prevent this error, implement thorough error handling in your code, use linters to catch syntax errors, and regularly update dependencies. Additionally, conduct comprehensive testing before deploying your application.
Where can I find more information about fixing this error?
More information can be found in the documentation of the framework you are using, such as Electron’s official documentation. Online forums, developer communities, and Stack Overflow can also provide valuable insights and solutions.
In summary, encountering a “JavaScript error in the main process” typically indicates a failure in the execution of a JavaScript code that is critical to the operation of an application, often related to Electron-based applications. This error can arise from various issues, including syntax errors, missing modules, or problems with the application’s configuration. Identifying the root cause requires a thorough examination of the error logs and the codebase to pinpoint the source of the problem.
Key takeaways from the discussion include the importance of proper error handling and debugging practices. Developers should utilize tools such as console logging and debugging utilities to trace the error’s origin. Additionally, ensuring that all dependencies are correctly installed and up-to-date can help mitigate the risk of encountering such errors. Regular testing and code reviews can also significantly reduce the likelihood of errors in the main process.
Moreover, it is crucial for developers to understand the context in which the error occurs. This understanding can aid in distinguishing between issues that are inherent to the code versus those that may stem from the environment in which the application runs. By adopting a systematic approach to diagnosing and resolving these errors, developers can enhance the stability and reliability of their applications.
Author Profile
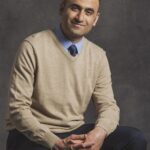
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?