How Can I Remove Active Class from Each Slide in a JS Slider?
In the world of web design, sliders have become a staple for showcasing images, products, or content in an engaging and interactive way. They not only enhance the visual appeal of a website but also improve user experience by allowing visitors to browse through multiple pieces of information seamlessly. However, as simple as they may seem, creating a functional and visually appealing slider requires a solid understanding of JavaScript and CSS. One common challenge developers face is managing the active state of each slide, especially when transitioning between them. In this article, we’ll delve into the intricacies of JavaScript sliders, focusing on how to effectively remove the active class from each slide to ensure a smooth and polished user experience.
When building a slider, the active class plays a crucial role in determining which slide is currently visible to the user. This class not only influences the visual presentation but also impacts the functionality of navigation controls. As users interact with the slider—clicking through slides or using keyboard shortcuts—it’s essential to manage the active state properly. Failing to do so can lead to confusing experiences, where multiple slides appear active or none at all, detracting from the overall design and usability of the site.
In this exploration, we will cover the fundamental techniques for implementing a JavaScript slider that dynamically updates
Implementing a JavaScript Slider with Active Slide Management
In a JavaScript slider, managing the active state of each slide is crucial for creating a seamless user experience. When a user navigates through slides, the active slide should be visually distinct. This can be achieved by removing the active class from the previous slide before adding it to the current slide.
To implement this functionality, consider the following approach:
- HTML Structure: Create a basic structure for the slider, including navigation buttons.
“`html
“`
- **CSS Styles**: Define styles for the active slide to differentiate it from inactive ones.
“`css
.slide {
display: none;
}
.slide.active {
display: block;
}
“`
- **JavaScript Logic**: Use JavaScript to manage the active class. Below is a sample implementation using vanilla JavaScript.
“`javascript
const slides = document.querySelectorAll(‘.slide’);
let currentSlide = 0;
function showSlide(index) {
slides.forEach((slide, i) => {
slide.classList.remove(‘active’); // Remove active class from all slides
if (i === index) {
slide.classList.add(‘active’); // Add active class to the current slide
}
});
}
document.querySelector(‘.next’).addEventListener(‘click’, () => {
currentSlide = (currentSlide + 1) % slides.length; // Move to the next slide
showSlide(currentSlide);
});
document.querySelector(‘.prev’).addEventListener(‘click’, () => {
currentSlide = (currentSlide – 1 + slides.length) % slides.length; // Move to the previous slide
showSlide(currentSlide);
});
// Initial display
showSlide(currentSlide);
“`
This implementation ensures that only one slide is visible at any time by removing the active class from all slides and applying it only to the current slide.
Enhancements for User Experience
To further enhance the slider, consider the following features:
- Automatic Slide Transition: Implement a timer that automatically transitions slides after a set interval.
- Dot Navigation: Add dots below the slides to indicate the current slide and allow users to navigate directly to any slide.
- Swipe Functionality: Integrate touch events for mobile users to swipe left or right to change slides.
Performance Considerations
When developing a slider, it’s essential to keep performance in mind. Here are some tips:
- Minimize Reflows: Batch DOM updates to reduce reflows and repaints.
- Lazy Loading: Load slides only when they are about to be displayed to save memory and improve initial load time.
- Debouncing Events: Implement debouncing on resize or scroll events to prevent performance degradation.
Feature | Description | Benefit |
---|---|---|
Automatic Transition | Slides change automatically after a specified time. | Improves user engagement by reducing the need for manual navigation. |
Dot Navigation | Visual indicators for each slide that allow direct access. | Enhances usability and visibility of the current slide. |
Swipe Support | Allows users to swipe left or right on touch devices. | Increases accessibility and improves the mobile user experience. |
By following these guidelines, you can create an effective and user-friendly JavaScript slider that manages active slides efficiently.
Implementing a JS Slider with Active Class Management
To effectively manage the active state of slides in a JavaScript slider, you can implement a straightforward function that removes the active class from all slides before adding it to the current slide. This ensures that only one slide is marked as active at any given time.
Basic Structure of the Slider
Consider the following HTML structure for the slider:
“`html
“`
In this example, each slide has a class of `slide`, and the currently visible slide has an additional class of `active`.
JavaScript Functionality for Managing Active Slides
To switch between slides and manage the active class, use the following JavaScript code:
“`javascript
const slides = document.querySelectorAll(‘.slide’);
let currentSlide = 0;
function showSlide(index) {
slides.forEach((slide, i) => {
slide.classList.remove(‘active’);
if (i === index) {
slide.classList.add(‘active’);
}
});
}
document.querySelector(‘.next’).addEventListener(‘click’, () => {
currentSlide = (currentSlide + 1) % slides.length;
showSlide(currentSlide);
});
document.querySelector(‘.prev’).addEventListener(‘click’, () => {
currentSlide = (currentSlide – 1 + slides.length) % slides.length;
showSlide(currentSlide);
});
// Initialize the slider
showSlide(currentSlide);
“`
Explanation of the Code
- Selecting Elements: The `slides` variable holds all slide elements, while `currentSlide` keeps track of the currently displayed slide index.
- showSlide Function: This function:
- Iterates through each slide.
- Removes the `active` class from all slides.
- Adds the `active` class to the slide corresponding to the passed index.
- Event Listeners: The buttons for previous and next slides adjust the `currentSlide` index and call `showSlide` to update the display.
Considerations for Enhancements
To improve user experience and functionality, consider the following enhancements:
- Auto-slide Feature: Implement an automatic transition after a specific interval.
- Indicators: Add visual indicators to show the current slide position.
- Touch Support: Enable swipe gestures for mobile devices.
- Accessibility Features: Ensure keyboard navigation and ARIA roles for better accessibility.
Feature | Description |
---|---|
Auto-slide | Automatically move to the next slide after a set time. |
Indicators | Display dots or thumbnails for each slide. |
Touch Support | Allow swiping on touch devices. |
Accessibility | Implement keyboard navigation and screen reader support. |
This approach ensures a robust and user-friendly slider that effectively manages the active state of slides, providing a seamless experience for users.
Expert Insights on Managing Active States in JavaScript Sliders
Emily Carter (Front-End Developer, CodeCraft Solutions). “To effectively manage active states in a JavaScript slider, it is crucial to implement a function that dynamically removes the ‘active’ class from the previously active slide. This ensures that only the current slide is highlighted, enhancing user experience and visual clarity.”
Michael Thompson (UI/UX Designer, Creative Interfaces). “Incorporating a method to remove the active class from each slide not only streamlines the navigation process but also prevents potential confusion among users. A well-structured event listener can facilitate this process, ensuring that the transitions between slides are seamless and intuitive.”
Sarah Lee (JavaScript Framework Specialist, DevSphere). “When developing a slider, it is essential to consider the implications of removing the active class from each slide. This practice not only optimizes performance but also contributes to a more maintainable codebase. Implementing a clear logic for managing these classes can significantly enhance the functionality of the slider component.”
Frequently Asked Questions (FAQs)
How can I remove the active class from each slide in a JS slider?
To remove the active class from each slide, you can loop through all slides and use the `classList.remove()` method to eliminate the active class before adding it to the current slide.
What JavaScript method is commonly used to select slides in a slider?
The `document.querySelectorAll()` method is frequently used to select all slide elements in a slider. This allows you to manipulate each slide individually.
Is it necessary to use event listeners for slide transitions?
Yes, using event listeners is essential for handling slide transitions effectively. They allow you to detect when a slide changes and perform necessary actions, such as updating the active class.
Can I achieve slide transitions without a library?
Yes, you can create slide transitions using pure JavaScript and CSS. However, libraries like jQuery or frameworks like React can simplify the process and provide additional functionality.
What is the best way to ensure only one slide has the active class at a time?
The best approach is to remove the active class from all slides before adding it to the currently active slide. This ensures that only one slide is highlighted as active.
How do I handle dynamic slides in a JavaScript slider?
For dynamic slides, you should update the slide list and reapply the active class logic whenever slides are added or removed. This can be done by re-running the loop that manages the active class.
In the context of JavaScript sliders, the functionality of removing the active class from each slide is crucial for ensuring a seamless user experience. This process typically involves identifying the currently active slide and then modifying its class to reflect its inactive state before applying the active class to the new slide. This mechanism not only enhances visual feedback for users but also maintains the integrity of the slider’s functionality.
Implementing a JavaScript slider that effectively manages the active state of slides requires a clear understanding of DOM manipulation and event handling. By utilizing methods such as `classList.remove()` and `classList.add()`, developers can efficiently toggle the active class between slides. This approach allows for smooth transitions and ensures that only one slide is highlighted at any given time, thereby preventing confusion and enhancing usability.
Moreover, it is essential to consider performance optimization when developing sliders. Minimizing the number of DOM manipulations and leveraging CSS transitions can significantly improve the responsiveness of the slider. Additionally, ensuring that the slider is accessible and functions well across various devices and screen sizes is paramount in today’s web development landscape.
In summary, effectively managing the active state of slides in a JavaScript slider is vital for creating an intuitive and engaging user interface. By focusing on
Author Profile
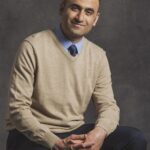
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?