How Can You Convert Stored Data into HTML Using JavaScript?
### Introduction
In the ever-evolving landscape of web development, the ability to dynamically display stored data using HTML and JavaScript is a fundamental skill that can elevate your projects from static pages to interactive experiences. Whether you’re building a simple personal website or a complex web application, knowing how to retrieve and present data effectively is crucial. This article will guide you through the essential techniques and best practices for transforming your stored data into visually appealing HTML elements using JavaScript.
To begin with, understanding how to access and manipulate data is key. Data can be stored in various formats, such as JSON, XML, or even databases, and JavaScript provides powerful tools to interact with these formats. By leveraging JavaScript’s capabilities, you can fetch data from local storage, APIs, or databases and seamlessly integrate it into your HTML structure. This process not only enhances user engagement but also allows for real-time updates, making your web applications more dynamic and responsive.
As we delve deeper into the topic, we will explore various methods and techniques for displaying stored data, including the use of DOM manipulation, template literals, and modern frameworks. Each approach offers unique advantages and can be tailored to fit different project requirements. By the end of this article, you’ll have a solid understanding of how to effectively convert your stored
Storing Data in JavaScript
To effectively utilize data in your HTML using JavaScript, it’s important to understand how to store and retrieve this data efficiently. JavaScript provides several methods for storing data, including variables, arrays, and objects, which can be used in conjunction with the Document Object Model (DOM) to dynamically update HTML content.
Using Local Storage
Local Storage is a web storage feature that allows you to store key-value pairs in a web browser. This data persists even when the browser is closed and reopened. Here’s how you can use Local Storage to store and retrieve data:
- Storing data: Use the `setItem` method to store data.
- Retrieving data: Use the `getItem` method to retrieve stored data.
- Removing data: Use the `removeItem` method to delete specific data.
Example code for storing and retrieving data:
javascript
// Storing data
localStorage.setItem(‘username’, ‘JohnDoe’);
// Retrieving data
const username = localStorage.getItem(‘username’);
console.log(username); // Outputs: JohnDoe
Displaying Stored Data in HTML
To display data stored in Local Storage on your HTML page, you can manipulate the DOM using JavaScript. Below is an example of how to retrieve data from Local Storage and display it in an HTML element.
Welcome,
Using JSON for Complex Data
For more complex data structures, such as arrays or objects, you can use JSON (JavaScript Object Notation) to serialize and deserialize data. This is particularly useful when you want to store multiple values in Local Storage.
- Stringifying data: Use `JSON.stringify()` to convert an object or array into a JSON string before storing it.
- Parsing data: Use `JSON.parse()` to convert the JSON string back into an object or array when retrieving it.
Example code:
javascript
// Storing an array
const users = [‘Alice’, ‘Bob’, ‘Charlie’];
localStorage.setItem(‘users’, JSON.stringify(users));
// Retrieving the array
const storedUsers = JSON.parse(localStorage.getItem(‘users’));
console.log(storedUsers); // Outputs: [‘Alice’, ‘Bob’, ‘Charlie’]
Example of Displaying Multiple Stored Items
To display an array of stored items in HTML, you can loop through the array and create HTML elements dynamically.
User List
Summary Table of Storage Methods
Method | Description | Persistence |
---|---|---|
Local Storage | Stores data as key-value pairs | Persistent across sessions |
Session Storage | Stores data for one session | Cleared when the page session ends |
Cookies | Stores data as key-value pairs, can have expiration | Depends on expiration set |
Accessing Stored Data
To utilize stored data in an HTML document using JavaScript, you first need to determine where the data is stored. Common storage methods include:
- Local Storage: Data is stored in a key-value format within the user’s browser.
- Session Storage: Similar to local storage but data is cleared when the session ends.
- Cookies: Small pieces of data stored in the browser, useful for session management.
- Database: More complex data can be stored in a backend database, accessed via APIs.
Retrieving Data from Local Storage
When using local storage, JavaScript provides an easy way to retrieve and manipulate data. Here’s how you can achieve this:
javascript
// Store data in local storage
localStorage.setItem(‘exampleData’, JSON.stringify({ name: ‘John’, age: 30 }));
// Retrieve data from local storage
const storedData = JSON.parse(localStorage.getItem(‘exampleData’));
console.log(storedData); // Output: { name: ‘John’, age: 30 }
Displaying Data in HTML
Once the data is retrieved, you can dynamically insert it into your HTML document. Use the Document Object Model (DOM) to accomplish this.
javascript
// Assuming you have a div with id ‘data-display’
const displayDiv = document.getElementById(‘data-display’);
displayDiv.innerHTML = `Name: ${storedData.name}
Age: ${storedData.age}
`;
Using Session Storage
Session storage works similarly to local storage but is limited to the duration of the page session. Here’s an example:
javascript
// Store data in session storage
sessionStorage.setItem(‘sessionData’, JSON.stringify({ city: ‘New York’, country: ‘USA’ }));
// Retrieve data from session storage
const sessionData = JSON.parse(sessionStorage.getItem(‘sessionData’));
document.getElementById(‘session-display’).innerHTML = `City: ${sessionData.city}
Country: ${sessionData.country}
`;
Working with Cookies
Cookies can be accessed using JavaScript but require more handling due to their format. Here’s an example of how to create, read, and display cookie data:
javascript
// Function to set a cookie
function setCookie(name, value, days) {
const expires = new Date(Date.now() + days * 864e5).toUTCString();
document.cookie = name + ‘=’ + encodeURIComponent(value) + ‘; expires=’ + expires;
}
// Function to get a cookie
function getCookie(name) {
return document.cookie.split(‘; ‘).reduce((r, v) => {
const parts = v.split(‘=’);
return parts[0] === name ? decodeURIComponent(parts[1]) : r;
}, ”);
}
// Set a cookie
setCookie(‘username’, ‘JaneDoe’, 7);
// Retrieve and display the cookie
const cookieData = getCookie(‘username’);
document.getElementById(‘cookie-display’).innerHTML = `Username: ${cookieData}
`;
Fetching Data from a Backend Database
For more complex scenarios, such as fetching data from a server-side database, you will typically use AJAX or the Fetch API. Here’s an example using the Fetch API:
javascript
fetch(‘https://api.example.com/data’)
.then(response => response.json())
.then(data => {
const dataDisplay = document.getElementById(‘api-display’);
dataDisplay.innerHTML = `Name: ${data.name}
Age: ${data.age}
`;
})
.catch(error => console.error(‘Error fetching data:’, error));
Implementing these methods will allow you to effectively retrieve and display stored data in your HTML documents using JavaScript. Each method has its suitable use cases depending on the nature and lifespan of the data you are handling.
Transforming Stored Data into HTML with JavaScript: Expert Insights
Dr. Emily Carter (Web Development Specialist, Tech Innovations Group). “To effectively convert stored data into HTML using JavaScript, one should leverage the power of the Document Object Model (DOM). By dynamically creating HTML elements and appending them to the DOM, developers can present data in a structured and visually appealing manner.”
Mark Thompson (Senior Software Engineer, Data Solutions Inc.). “Utilizing JSON as a data format is crucial when working with JavaScript. By fetching data from a server and parsing it into JSON, developers can easily iterate through the data and generate corresponding HTML elements, ensuring a seamless integration of data and presentation.”
Linda Garcia (Front-End Developer, Creative Web Agency). “Incorporating template literals in JavaScript can significantly enhance the process of rendering stored data into HTML. This approach allows for cleaner and more readable code, making it easier to manage dynamic content and maintain the overall structure of the web application.”
Frequently Asked Questions (FAQs)
How can I retrieve stored data in JavaScript?
You can retrieve stored data in JavaScript using the `localStorage` or `sessionStorage` APIs. Use `localStorage.getItem(‘key’)` to fetch data stored under a specific key.
What format should the stored data be in for HTML display?
Stored data can be in various formats, such as strings, JSON objects, or arrays. For HTML display, JSON is commonly used, as it can be easily converted to a JavaScript object.
How do I convert JSON data to HTML elements?
You can convert JSON data to HTML elements by parsing the JSON string into an object using `JSON.parse()`, then iterating through the object to create HTML elements dynamically using methods like `document.createElement()`.
Can I use templates to render stored data in HTML?
Yes, using template literals in JavaScript allows you to create HTML strings that can be easily injected into the DOM. This approach enhances readability and maintainability of your code.
What libraries can help with rendering data to HTML?
Libraries such as React, Vue.js, and Handlebars.js can facilitate rendering stored data to HTML. These libraries provide efficient ways to manage and display dynamic content.
How do I handle errors when retrieving stored data?
To handle errors when retrieving stored data, use try-catch blocks around your retrieval code. This approach allows you to manage exceptions gracefully and provide fallback mechanisms if data is unavailable.
In summary, converting stored data into HTML using JavaScript involves a systematic approach that leverages the capabilities of the Document Object Model (DOM). By utilizing JavaScript, developers can dynamically create HTML elements and populate them with data fetched from various sources, such as databases or APIs. This process enhances the interactivity and responsiveness of web applications, allowing for real-time updates and user engagement.
Key techniques include using methods like `document.createElement()` to create new HTML elements and `appendChild()` to insert them into the DOM. Additionally, developers can utilize templating libraries or frameworks to streamline the process of rendering complex data structures into user-friendly HTML formats. Understanding how to manipulate the DOM effectively is crucial for creating seamless user experiences on the web.
Moreover, it is essential to consider best practices for data handling, such as ensuring data is properly sanitized to prevent security vulnerabilities like XSS (Cross-Site Scripting). By maintaining a focus on performance and security, developers can ensure that their applications not only function well but also safeguard user data. Overall, mastering the conversion of stored data to HTML with JavaScript is a fundamental skill for modern web development.
Author Profile
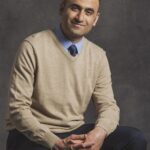
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?