How Can You Use sapply in R to Replace Else If Statements?
In the world of R programming, data manipulation and analysis often require efficient and elegant solutions to common problems. One such challenge is the need to apply conditional logic across vectors or data frames. While traditional control structures like `if-else` statements are familiar to many, they can become cumbersome when dealing with larger datasets or when needing to apply the same logic repeatedly. Enter `sapply`, a powerful function that not only simplifies the process but also enhances readability and performance. In this article, we will explore how `sapply` can effectively replace the need for complex `else if` statements, streamlining your code and making your data analysis tasks more intuitive.
At its core, `sapply` is designed to apply a function to each element of a list or vector, returning a simplified output. This functionality allows for the implementation of conditional logic in a more compact form, reducing the clutter that often accompanies nested `if-else` structures. By leveraging `sapply`, you can efficiently categorize or transform data based on specific conditions without sacrificing clarity. This approach not only saves time but also minimizes the potential for errors that can arise from more intricate coding patterns.
As we delve deeper into the nuances of `sapply`, we will uncover practical examples and scenarios where this function shines. From
sapply in R: A Powerful Tool for Vectorized Operations
The `sapply` function in R is a versatile tool that allows users to apply a function to each element of a list or vector, simplifying the output into a vector or matrix. This function is particularly useful when you want to perform operations that would otherwise require explicit loops, making your code cleaner and more efficient.
One common scenario where `sapply` shines is when you want to perform conditional replacements similar to using `else if` statements. Instead of writing lengthy conditional structures, you can leverage `sapply` to make your code more concise.
For example, consider a situation where you want to replace values in a numeric vector based on specific conditions. You can create a custom function that defines these conditions and use `sapply` to apply it across your data.
Here’s an illustration of how this can be achieved:
“`R
Sample numeric vector
numbers <- c(1, 2, 3, 4, 5, 6)
Define a custom function for replacements
replace_values <- function(x) {
if (x < 3) {
return("Low")
} else if (x <= 5) {
return("Medium")
} else {
return("High")
}
}
Apply the function using sapply
result <- sapply(numbers, replace_values)
print(result)
```
This code snippet demonstrates how the `replace_values` function categorizes each number based on the specified conditions. The output will be a character vector indicating the category of each number.
Advantages of Using sapply Over else if
Using `sapply` instead of traditional `if-else` statements can provide several advantages:
- Conciseness: Reduces the amount of code needed for element-wise operations.
- Readability: The intent of the code is clearer, as the function’s purpose is encapsulated.
- Performance: Vectorized operations generally execute faster than iterative loops.
Feature | sapply | if-else |
---|---|---|
Code Length | Shorter | Longer |
Ease of Understanding | High | Moderate |
Performance | Better | Variable |
In summary, `sapply` serves as an efficient alternative to `else if` statements for vectorized operations, allowing for clearer and more maintainable code. By defining a function that encapsulates your conditional logic, you can apply it seamlessly across your data structures, enhancing both performance and readability.
Using `sapply` to Replace `else if` in R
The `sapply` function in R is a powerful tool for applying a function over a list or vector and simplifying the output. It can often replace multiple `if-else` statements, making the code more concise and readable. Below are examples and explanations of how to effectively use `sapply` in this context.
Basic Syntax of `sapply`
The general syntax of `sapply` is as follows:
“`R
sapply(X, FUN, …, simplify = TRUE, USE.NAMES = TRUE)
“`
- X: A list or vector that you want to apply a function to.
- FUN: The function to apply.
- …: Additional arguments to pass to the function.
- simplify: If TRUE, attempts to simplify the result to a vector or matrix.
- USE.NAMES: If TRUE, the result will be named if X is a named list.
Example of Replacing `else if` with `sapply`
Consider a scenario where you want to categorize numerical scores into letter grades. Traditionally, this would involve multiple `if-else` statements:
“`R
get_grade <- function(score) {
if (score >= 90) {
return(“A”)
} else if (score >= 80) {
return(“B”)
} else if (score >= 70) {
return(“C”)
} else if (score >= 60) {
return(“D”)
} else {
return(“F”)
}
}
scores <- c(95, 85, 75, 65, 55) grades <- sapply(scores, get_grade) ```
Using a Vectorized Approach
Instead of defining a function with `if-else`, you can use a vectorized approach with `sapply`. This method utilizes conditions directly within `sapply`:
“`R
scores <- c(95, 85, 75, 65, 55)
grades <- sapply(scores, function(score) {
if (score >= 90) {
“A”
} else if (score >= 80) {
“B”
} else if (score >= 70) {
“C”
} else if (score >= 60) {
“D”
} else {
“F”
}
})
“`
While the above still uses `if-else`, we can enhance this further using a single vector of conditions:
“`R
grades <- sapply(scores, function(score) {
cut(score, breaks = c(-Inf, 59, 69, 79, 89, 100),
labels = c("F", "D", "C", "B", "A"))
})
```
Benefits of Using `sapply`
- Conciseness: Reduces the number of lines of code compared to traditional `if-else` statements.
- Readability: Makes the intent of the code clearer, especially for categorical mappings.
- Performance: Can be faster for large vectors due to vectorization.
Limitations and Considerations
- Complex Logic: For highly complex decision trees, `sapply` may not be the best choice as it could lead to less readable code.
- Debugging: If issues arise, debugging vectorized functions may be more challenging than traditional conditional statements.
Utilizing `sapply` in R allows for efficient replacements of `if-else` constructs, especially in scenarios requiring mapping or categorization. By leveraging vectorized operations, you can enhance both the performance and clarity of your R code.
Expert Insights on Using `sapply` in R for Conditional Replacement
Dr. Emily Chen (Data Scientist, Analytics Innovations Inc.). “In R, the `sapply` function is incredibly versatile for applying functions over lists or vectors. When considering the need for conditional replacements akin to ‘else if’ statements, leveraging `sapply` with a custom function can streamline your code. This approach not only enhances readability but also maintains efficiency in data manipulation.”
Michael Thompson (Senior Statistician, StatTech Solutions). “While `sapply` is a powerful tool for vectorized operations, it is essential to recognize its limitations in complex conditional logic. Instead of using multiple `ifelse` statements, which can become cumbersome, I recommend defining a separate function that incorporates your conditional logic. This method can be seamlessly integrated with `sapply`, improving both clarity and performance.”
Dr. Sarah Patel (Professor of Statistics, University of Data Science). “The use of `sapply` for replacing values based on conditions can be a game-changer in data analysis workflows. However, when faced with intricate conditions, I advise considering the `dplyr` package for more readable syntax. Functions like `mutate` combined with `case_when` can serve as an elegant alternative to `sapply`, particularly for those who prefer a tidyverse approach.”
Frequently Asked Questions (FAQs)
What is the purpose of the `sapply` function in R?
The `sapply` function in R is used to apply a function over a list or vector and simplify the result into a vector or matrix. It is particularly useful for performing operations on each element of a list or vector efficiently.
How can I use `sapply` to replace values conditionally in R?
You can use `sapply` in conjunction with a custom function that includes conditional statements to replace values. For example, you can define a function that checks each element and returns a new value based on specific conditions.
Can I use `sapply` instead of `ifelse` for conditional replacements?
Yes, `sapply` can be used for conditional replacements, but it is generally more verbose than `ifelse`. While `ifelse` is vectorized and more concise for simple conditions, `sapply` allows for more complex logic within a custom function.
What is the difference between `sapply` and `lapply` in R?
The primary difference is that `sapply` simplifies the output to a vector or matrix when possible, while `lapply` always returns a list. Use `sapply` when you expect a simplified output and `lapply` when you want to maintain the list structure.
How can I handle multiple conditions using `sapply`?
You can handle multiple conditions by incorporating `if` and `else if` statements within the function passed to `sapply`. This allows you to define different outputs based on various conditions for each element of the input.
Is it possible to use `sapply` for data frame columns?
Yes, `sapply` can be applied to data frame columns by specifying the column as a vector. It is commonly used to apply functions across columns to perform operations like data transformation or conditional replacements.
The `sapply` function in R is a powerful tool for applying a function to each element of a list or vector, simplifying the output into a vector or matrix. It is particularly useful for operations that would otherwise require the use of loops, allowing for more concise and readable code. While `sapply` is effective for many tasks, it does not inherently support complex conditional logic like `if…else if…else` statements. Instead, users often need to combine `sapply` with other functions or utilize alternative approaches to achieve similar functionality.
One common method to replace `else if` constructs when using `sapply` is to define a custom function that encapsulates the conditional logic. This custom function can then be passed to `sapply`, allowing for the evaluation of conditions for each element in the input. By structuring the function to handle multiple conditions, users can effectively replicate the behavior of `else if` statements within the `sapply` framework.
In summary, while `sapply` is an efficient alternative to loops in R, it requires careful consideration when dealing with multiple conditions. By leveraging custom functions, users can maintain the clarity and efficiency of their code while still implementing complex decision-making processes. This approach not
Author Profile
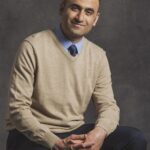
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?