How Can I Auto Fill Forms Using JavaScript?
In the fast-paced digital age, efficiency is key, especially when it comes to filling out online forms. Whether you’re a developer looking to streamline user experience or a user tired of repetitive data entry, mastering the art of auto-filling forms with JavaScript can save you time and frustration. This powerful scripting language offers a variety of methods to automate the completion of forms, enhancing both functionality and user satisfaction. In this article, we will explore the techniques and best practices for implementing auto-fill features in your web applications.
Auto-filling forms with JavaScript not only simplifies the user experience but also reduces the chance of errors that can occur during manual entry. By leveraging the capabilities of JavaScript, developers can create dynamic and responsive forms that automatically populate fields based on user input or predefined data. This not only speeds up the process but also encourages users to complete forms more frequently, ultimately leading to higher conversion rates for businesses.
As we delve deeper into the topic, we will examine various methods for auto-filling forms, including the use of event listeners, local storage, and third-party libraries. Each approach offers unique advantages, allowing developers to choose the best fit for their specific needs. Whether you’re looking to enhance a simple contact form or create a complex multi-step application, understanding how to effectively implement
Understanding Form Elements
To effectively auto-fill forms using JavaScript, it is crucial to understand the various types of form elements, their properties, and how they can be accessed through the DOM (Document Object Model). Common form elements include:
- Input: Text fields, password fields, checkboxes, and radio buttons.
- Select: Dropdown lists.
- Textarea: Multi-line text fields.
- Button: Submit and reset buttons.
Each element can be targeted using its unique ID, class, or name attributes, allowing for precise manipulation.
Using JavaScript to Auto-Fill Forms
JavaScript enables developers to programmatically set the value of form elements. The following example demonstrates how to auto-fill a form using JavaScript:
In this example, the `value` property of each input field is set to the desired data.
Event-Driven Auto-Filling
For a more dynamic approach, auto-filling can be triggered by specific events, such as clicking a button or selecting a value from another field. This enhances user experience by automatically populating fields based on previous inputs.
Form Auto-Filling with Data from External Sources
In some cases, you may want to populate a form with data retrieved from external sources, such as APIs. This can be accomplished using the Fetch API or XMLHttpRequest to obtain data asynchronously.
javascript
fetch(‘https://api.example.com/user/1’)
.then(response => response.json())
.then(data => {
document.getElementById(‘name’).value = data.name;
document.getElementById(’email’).value = data.email;
document.getElementById(‘age’).value = data.age;
});
This code snippet fetches user data from an API and fills the form fields accordingly.
Best Practices for Auto-Filling Forms
When implementing auto-fill functionality, consider the following best practices:
- Ensure that auto-filled data is relevant and accurate.
- Provide users with the option to override auto-filled values.
- Test across multiple browsers to ensure compatibility.
- Use secure methods to handle sensitive data.
Example of a Complete Auto-Fill Implementation
Below is a complete example that combines all concepts discussed, showcasing a functional form with auto-fill capabilities:
This example provides a user-friendly interface for auto-filling a form with a button click, enhancing usability and efficiency.
Understanding the Basics of Auto Filling Forms
Auto-filling forms using JavaScript involves manipulating the Document Object Model (DOM) to automatically populate input fields with data. This can enhance user experience by reducing repetitive input tasks.
To auto-fill forms, you typically use the following methods:
- Direct DOM Manipulation: Accessing input elements and setting their values.
- Event Listeners: Triggering auto-fill actions based on user interactions.
- JavaScript Libraries: Utilizing libraries that streamline the process.
Direct DOM Manipulation
The most straightforward way to auto-fill a form is by directly modifying the input elements. Here’s a basic example of how to do this:
javascript
document.getElementById(“username”).value = “JohnDoe”;
document.getElementById(“email”).value = “[email protected]”;
document.getElementById(“password”).value = “securePassword123”;
In this snippet, the values for the username, email, and password fields are set programmatically. This method is efficient for simple forms.
Using Event Listeners for Auto-Filling
You can enhance user experience by triggering the auto-fill process based on specific events, such as clicking a button or selecting an option. Below is an example that auto-fills a form when a button is clicked:
Here, an event listener is used to fill the form when the button is clicked, providing a more interactive experience.
Using JavaScript Libraries
JavaScript libraries can simplify the auto-fill process. Libraries like jQuery or frameworks such as React provide methods to manipulate the DOM more effectively. Here’s an example using jQuery:
javascript
$(“#fillForm”).click(function() {
$(“#username”).val(“JohnDoe”);
$(“#email”).val(“[email protected]”);
$(“#password”).val(“securePassword123”);
});
This approach reduces the amount of code required and can enhance readability.
Best Practices for Auto-Filling Forms
When implementing auto-fill functionality, consider the following best practices:
- User Consent: Always ensure the user is aware of auto-filling actions.
- Validation: Validate data before auto-filling to prevent errors.
- Security: Be cautious with sensitive data and avoid hardcoding passwords.
- Accessibility: Ensure forms are accessible, using appropriate labels for input fields.
Best Practice | Description |
---|---|
User Consent | Notify users when auto-filling occurs. |
Validation | Check input data before auto-filling. |
Security | Avoid exposing sensitive data in scripts. |
Accessibility | Use labels and ARIA attributes for clarity. |
Implementing auto-fill functionality in forms using JavaScript can significantly enhance user experience. By understanding the basics, utilizing event listeners, and leveraging libraries, developers can create more efficient and user-friendly web applications.
Expert Insights on Auto Filling Forms with JavaScript
Dr. Emily Carter (Senior Web Developer, Tech Innovations Inc.). “Auto-filling forms using JavaScript can significantly enhance user experience. By leveraging the browser’s local storage or session storage, developers can store user inputs and retrieve them when needed, streamlining the process.”
Michael Chen (Lead Front-End Engineer, Digital Solutions Group). “Implementing auto-fill functionality requires careful consideration of security. It is essential to validate and sanitize user inputs to prevent vulnerabilities such as XSS attacks, ensuring that the auto-fill feature does not compromise the application’s integrity.”
Sarah Patel (UX/UI Designer, User-Centric Designs). “From a user experience perspective, auto-filling forms can reduce friction in the submission process. However, it is crucial to provide users with clear options to edit or clear the auto-filled data, as personalization can vary significantly between users.”
Frequently Asked Questions (FAQs)
What is auto-filling a form in JavaScript?
Auto-filling a form in JavaScript refers to the process of programmatically populating input fields within a web form using JavaScript code. This can enhance user experience by reducing manual data entry.
How can I auto-fill a form using JavaScript?
To auto-fill a form, you can select the input elements using `document.getElementById()` or `document.querySelector()`, and then set their `value` properties to the desired data. For example:
javascript
document.getElementById(‘name’).value = ‘John Doe’;
Can I auto-fill forms based on user actions?
Yes, you can trigger auto-filling based on user actions such as button clicks or input changes. You can attach event listeners to elements and execute the auto-fill logic when the event occurs.
Is it possible to auto-fill forms with data from an API?
Absolutely. You can fetch data from an API using `fetch()` or `XMLHttpRequest`, and then use the retrieved data to populate the form fields dynamically.
Are there any security concerns with auto-filling forms?
Yes, auto-filling forms can pose security risks, especially if sensitive information is involved. Always ensure that data is sanitized and that you are not exposing sensitive information through client-side scripts.
What browsers support auto-filling forms with JavaScript?
Most modern browsers, including Chrome, Firefox, Safari, and Edge, support JavaScript-based form manipulation. However, specific features may vary, so testing across different browsers is advisable.
auto-filling forms using JavaScript is a powerful technique that enhances user experience by streamlining data entry processes. By leveraging JavaScript’s capabilities, developers can create scripts that automatically populate form fields based on predefined criteria, user input, or data retrieved from external sources. This functionality not only saves time for users but also minimizes the chances of errors that can occur during manual entry.
Key takeaways from the discussion include the importance of understanding the Document Object Model (DOM) and how to manipulate it effectively to access and modify form elements. Utilizing event listeners to trigger auto-fill actions based on user interactions, such as focusing on a field or submitting a form, is crucial for creating a responsive and intuitive interface. Additionally, incorporating validation checks ensures that the auto-filled data meets the required format and standards.
Moreover, developers should consider user privacy and data security when implementing auto-fill features. It is essential to handle sensitive information responsibly and comply with relevant regulations. By following best practices and maintaining a user-centric approach, developers can create efficient and secure auto-fill functionalities that significantly enhance the overall usability of web forms.
Author Profile
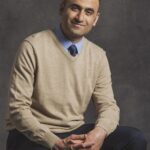
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?