How Can You Create a Script in Python? A Step-by-Step Guide
In the ever-evolving landscape of technology, Python has emerged as a powerhouse programming language, beloved by beginners and seasoned developers alike. Its simplicity and versatility make it an ideal choice for a wide array of applications, from web development to data analysis. But how does one harness the full potential of Python? The answer lies in mastering the art of scripting. Whether you’re looking to automate mundane tasks, create dynamic web applications, or delve into data science, understanding how to make a script in Python is an essential skill that can open up a world of possibilities.
Creating a script in Python is not just about writing lines of code; it’s about crafting a solution to a problem. At its core, a Python script is a collection of commands that the interpreter executes sequentially, allowing you to automate processes, manipulate data, or even build interactive applications. The beauty of Python lies in its readability, which encourages clean and efficient coding practices. As you embark on this journey, you’ll discover the fundamental concepts that underpin scripting, including variables, control structures, and functions, all of which will empower you to bring your ideas to life.
Moreover, the Python community is rich with resources and libraries that can enhance your scripting capabilities. With a plethora of modules available, you can extend the functionality
Setting Up Your Python Environment
To create a script in Python, the first step is to set up your development environment. This involves installing Python and selecting an appropriate code editor or integrated development environment (IDE). Follow these steps:
- Install Python: Download the latest version of Python from the official website (python.org). During installation, ensure you check the option to add Python to your system PATH.
- Choose an IDE or Text Editor: Popular choices include:
- PyCharm: A feature-rich IDE specifically for Python.
- Visual Studio Code: A lightweight but powerful code editor with Python extensions.
- Jupyter Notebook: Ideal for data science and interactive coding.
- Set Up Virtual Environments: It’s advisable to use virtual environments to manage dependencies for different projects. This can be achieved using `venv` or `virtualenv`.
Writing Your First Python Script
Creating a Python script is straightforward. Open your chosen IDE or text editor and follow these steps:
- Create a New File: Save the file with a `.py` extension, for example, `my_script.py`.
- Write Your Code: Start with a simple print statement to test your setup.
“`python
print(“Hello, World!”)
“`
- Save Your File: Ensure you save your changes before running the script.
Running Your Python Script
You can execute your Python script in several ways:
- Using Command Line:
- Open your terminal or command prompt.
- Navigate to the directory where your script is saved.
- Run the script using the command:
“`bash
python my_script.py
“`
- From an IDE: Most IDEs have a run button or option in the menu to execute the script directly.
Basic Structure of a Python Script
Understanding the basic structure of a Python script is essential for writing effective code. Here’s a simple breakdown:
- Import Statements: Used to include libraries or modules.
- Function Definitions: Define reusable blocks of code.
- Main Program Logic: The core logic of the script.
- Conditional Statements: Control the flow of the script based on conditions.
“`python
import math
def calculate_area(radius):
return math.pi * (radius ** 2)
if __name__ == “__main__”:
radius = 5
area = calculate_area(radius)
print(f”The area of the circle with radius {radius} is: {area}”)
“`
Common Python Script Functions
Here is a table illustrating some commonly used built-in functions in Python scripts:
Function | Description |
---|---|
print() | Outputs data to the console. |
input() | Accepts user input from the console. |
len() | Returns the length of an object (e.g., string, list). |
range() | Generates a sequence of numbers. |
type() | Returns the type of an object. |
This basic understanding will equip you with the foundational skills needed to create more complex scripts and applications in Python.
Understanding the Basics of Python Scripts
A Python script is simply a file containing Python code, which is executed when the script is run. The file typically has a `.py` extension. To create an effective Python script, familiarize yourself with the basic structure and functionality of Python programming.
Key Components of a Python Script:
- Variables: Store data values.
- Data Types: Common types include integers, floats, strings, and lists.
- Control Structures: Use `if`, `for`, and `while` statements to control the flow of execution.
- Functions: Define reusable pieces of code using the `def` keyword.
- Modules: Import libraries to extend functionality.
Setting Up Your Environment
Before writing a script, set up your Python development environment. This includes installing Python and choosing a code editor.
Installation Steps:
- Download the latest version of Python from the [official website](https://www.python.org/downloads/).
- Follow the installation instructions for your operating system.
- Verify the installation by running `python –version` in the terminal or command prompt.
Recommended Code Editors:
- Visual Studio Code: Highly customizable with extensions.
- PyCharm: A powerful IDE specifically for Python.
- Sublime Text: Lightweight and fast with a simple interface.
Writing Your First Script
Creating a Python script can be done using any text editor. Follow these steps to write your first simple script.
- Open your code editor.
- Create a new file and save it as `hello.py`.
- Write the following code:
“`python
print(“Hello, World!”)
“`
- Save the file.
Running the Script
To execute your Python script, follow the steps below:
- Using Command Line/Terminal:
- Navigate to the directory where your script is saved using `cd
`. - Run the script with the command: `python hello.py`.
- Using an IDE: Most IDEs have a built-in run feature. Simply click the run button to execute the script.
Enhancing Your Script
Once you have a basic script running, you can enhance it by adding functionality. Consider using variables, user input, and control structures.
**Example: User Input Script**
“`python
name = input(“Enter your name: “)
print(f”Hello, {name}!”)
“`
**Control Structure Example:**
“`python
age = int(input(“Enter your age: “))
if age >= 18:
print(“You are an adult.”)
else:
print(“You are a minor.”)
“`
Debugging Your Script
Debugging is crucial for identifying and fixing errors. Use the following techniques:
- Print Statements: Insert print statements to check variable values.
- Error Messages: Read error messages carefully; they often indicate where the issue is.
- Use a Debugger: Many IDEs provide debugging tools to step through your code.
Common Python Errors:
Error Type | Description |
---|---|
SyntaxError | Mistakes in the code structure |
NameError | Using a variable that has not been defined |
TypeError | Operations on incompatible data types |
Best Practices for Writing Python Scripts
To ensure your scripts are maintainable and efficient, adhere to these best practices:
- Use Meaningful Variable Names: Choose clear, descriptive names.
- Comment Your Code: Explain complex sections for future reference.
- Follow PEP 8 Guidelines: Adhere to the Python style guide for readability.
- Test Your Code: Regularly test scripts to catch issues early.
By understanding these fundamentals and practices, you can create effective and efficient Python scripts tailored to your needs.
Expert Insights on Creating Python Scripts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively create a script in Python, one must first understand the fundamental syntax and structure of the language. Starting with simple tasks allows for gradual learning, which is crucial for mastering more complex functionalities.”
James Lin (Lead Python Developer, CodeCraft Solutions). “When making a script in Python, it is essential to plan your code structure beforehand. Utilizing functions and modules not only enhances readability but also promotes code reusability, which is a best practice in software development.”
Dr. Sarah Patel (Professor of Computer Science, University of Technology). “Testing and debugging are critical components of script development in Python. Implementing unit tests early in the development process can significantly reduce errors and improve the overall quality of the code.”
Frequently Asked Questions (FAQs)
What is a Python script?
A Python script is a file containing Python code that can be executed to perform a specific task or set of tasks. It typically has a `.py` file extension.
How do I create a Python script?
To create a Python script, open a text editor or an Integrated Development Environment (IDE), write your Python code, and save the file with a `.py` extension.
What tools do I need to run a Python script?
You need to have Python installed on your computer. You can run scripts using the command line, terminal, or an IDE like PyCharm or Visual Studio Code.
How can I execute a Python script from the command line?
To execute a Python script from the command line, navigate to the directory containing the script and type `python script_name.py`, replacing `script_name.py` with the name of your script.
Can I run a Python script on any operating system?
Yes, Python scripts can run on any operating system that has Python installed, including Windows, macOS, and Linux.
What are some common errors when running Python scripts?
Common errors include syntax errors, indentation errors, and runtime errors. These can often be resolved by carefully reviewing the code for mistakes and ensuring proper syntax.
Creating a script in Python involves several fundamental steps that are essential for both beginners and seasoned programmers. Firstly, one must ensure that Python is installed on their system, which can be done by downloading it from the official Python website. Once installed, selecting a suitable code editor or Integrated Development Environment (IDE) is crucial for writing and managing the script effectively. Popular choices include PyCharm, Visual Studio Code, and Jupyter Notebook, each offering unique features that enhance the coding experience.
After setting up the environment, the next step is to write the script. This involves using Python’s syntax to define functions, control structures, and data types, which together form the backbone of the script. It is important to structure the code logically, using comments for clarity and maintaining readability. Testing the script through debugging is also vital to ensure that it performs as intended. This iterative process helps identify and fix errors, ultimately leading to a more robust final product.
Finally, once the script is complete and thoroughly tested, it can be executed in the terminal or command prompt. Additionally, scripts can be saved with a .py extension, allowing for easy sharing and reuse. Understanding how to make a script in Python not only empowers individuals to automate tasks and solve problems
Author Profile
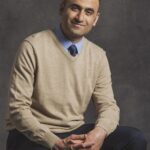
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?