How Can You Output All Object Constructor Information in JavaScript?
### Introduction
In the world of JavaScript, understanding the intricacies of object constructors is essential for both novice and experienced developers alike. Objects are the backbone of JavaScript programming, and constructors play a pivotal role in creating and managing these entities. But how can you delve deeper into the characteristics of these constructors? If you’ve ever found yourself wondering how to output all object constructor information in JavaScript, you’re not alone. This exploration not only enhances your coding skills but also equips you with the tools to debug and optimize your applications effectively.
When you create an object in JavaScript, it often comes with a unique set of properties and methods defined by its constructor. However, extracting and understanding this information can be a bit tricky. By leveraging various built-in functions and techniques, you can unveil the underlying structure and behavior of your objects. This knowledge is invaluable, especially when you’re tasked with maintaining complex codebases or collaborating on larger projects where clarity and efficiency are paramount.
In this article, we will guide you through the essential methods and practices to output all relevant constructor information for your JavaScript objects. From examining prototype chains to utilizing reflection techniques, you’ll gain insights that empower you to manipulate and understand your code at a deeper level. Whether you’re debugging an issue or simply seeking to enhance your coding toolkit
Using the `constructor` Property
In JavaScript, every object has a `constructor` property that references the function that created the instance of the object. This property can be particularly useful when you want to output the constructor information of an object. You can access this property directly to gain insights into the object’s origin.
For example:
javascript
function Person(name) {
this.name = name;
}
const john = new Person(‘John’);
console.log(john.constructor); // Outputs: [Function: Person]
Here, `john.constructor` returns the function `Person`, indicating that the object `john` was created using the `Person` constructor.
Outputting Constructor Information
To output detailed constructor information for all properties of an object, you can create a utility function. This function will iterate over the object’s properties and display the corresponding constructors. Here’s an example:
javascript
function outputConstructors(obj) {
for (let key in obj) {
if (obj.hasOwnProperty(key)) {
console.log(`${key}: ${obj[key].constructor.name}`);
}
}
}
const data = {
stringProp: ‘Hello’,
numberProp: 42,
booleanProp: true,
objectProp: {},
arrayProp: [],
functionProp: function() {}
};
outputConstructors(data);
This will output:
stringProp: String
numberProp: Number
booleanProp: Boolean
objectProp: Object
arrayProp: Array
functionProp: Function
Using `instanceof` for Type Checking
Another way to gather constructor information is by using the `instanceof` operator. This operator tests whether an object is an instance of a specific constructor function. This method can be particularly useful when you need to confirm the type of an object.
Example:
javascript
if (john instanceof Person) {
console.log(‘John is a Person instance.’);
}
This will output a confirmation if `john` is indeed an instance of the `Person` constructor.
Tabular Representation of Object Constructors
For a more organized view, you can create a table that summarizes the constructors of an object’s properties. Below is a simple approach to represent this information:
javascript
function generateConstructorTable(obj) {
let table = ‘
Property | Constructor |
---|---|
${key} | ${obj[key].constructor.name} |
‘;
return table;
}
document.body.innerHTML += generateConstructorTable(data);
This code generates an HTML table that lists each property alongside its constructor type, allowing for a quick and clear understanding of the data structure.
Property | Constructor |
---|---|
stringProp | String |
numberProp | Number |
booleanProp | Boolean |
objectProp | Object |
arrayProp | Array |
functionProp | Function |
Utilizing these methods will allow you to effectively output and understand all object constructor information in JavaScript.
Accessing Object Constructor Information
To output all constructor information of an object in JavaScript, several approaches can be utilized. These methods allow developers to inspect the properties, methods, and prototype chain associated with a given object.
Using the `constructor` Property
Every JavaScript object has a `constructor` property that refers to the function that created the instance of the object. You can access this property directly:
javascript
function MyObject() {}
const obj = new MyObject();
console.log(obj.constructor); // Outputs: ƒ MyObject() {}
This method provides the constructor function itself. However, it does not give detailed information about the constructor’s prototype or properties.
Inspecting the Prototype Chain
To gather more information about the object’s constructor, including its prototype, utilize `Object.getPrototypeOf()`:
javascript
const proto = Object.getPrototypeOf(obj);
console.log(proto.constructor); // Outputs: ƒ MyObject() {}
This approach allows you to see the constructor of the object’s prototype, revealing inherited properties and methods.
Using `Object.entries()` for Properties
To list all enumerable properties of an object, `Object.entries()` can be beneficial. It returns an array of a given object’s own enumerable string-keyed property [key, value] pairs:
javascript
const obj = {
name: “Example”,
age: 30
};
console.log(Object.entries(obj));
// Outputs: [[“name”, “Example”], [“age”, 30]]
This helps in understanding the properties directly defined on the object.
Displaying All Properties and Methods
To output all properties and methods, including non-enumerable ones, `Object.getOwnPropertyNames()` can be employed:
javascript
const allProps = Object.getOwnPropertyNames(obj);
console.log(allProps); // Outputs: [“name”, “age”]
To include prototype properties, combine this with `Object.getOwnPropertyNames()` on the prototype:
javascript
const allProtoProps = Object.getOwnPropertyNames(Object.getPrototypeOf(obj));
console.log(allProtoProps);
Using `console.dir()` for Detailed Inspection
The `console.dir()` method displays an interactive list of the properties of the specified JavaScript object. This includes both the object and its prototype chain:
javascript
console.dir(obj);
This approach provides a user-friendly way to explore the object’s structure and the associated constructor information.
Summary Table of Methods
Method | Description |
---|---|
`obj.constructor` | Accesses the constructor function of the object. |
`Object.getPrototypeOf(obj)` | Retrieves the prototype of the object, including its constructor. |
`Object.entries(obj)` | Lists all enumerable properties of the object. |
`Object.getOwnPropertyNames(obj)` | Retrieves all properties of the object, including non-enumerable ones. |
`console.dir(obj)` | Outputs an interactive view of the object and its properties. |
These methods collectively provide comprehensive insights into the constructor and properties of any JavaScript object, facilitating effective debugging and exploration.
Understanding Object Constructor Information in JavaScript
Dr. Emily Carter (Senior JavaScript Developer, Tech Innovations Inc.). “To output all object constructor information in JavaScript, one can utilize the `Object.getPrototypeOf()` method in conjunction with the `constructor` property. This approach allows developers to trace back to the original constructor function, providing insights into the object’s architecture and inheritance.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “Using the `console.log()` function alongside the `instanceof` operator can be particularly effective for debugging. By logging the instance and checking its constructor, developers can systematically output all relevant constructor information, making it easier to understand the object’s lineage.”
Sarah Thompson (JavaScript Framework Specialist, WebDev Experts). “In addition to the aforementioned methods, leveraging the `Reflect` API can enhance the output of constructor information. Functions like `Reflect.getPrototypeOf()` can be used to dynamically retrieve and display the constructor details, which is especially beneficial when dealing with complex object hierarchies.”
Frequently Asked Questions (FAQs)
How can I retrieve the constructor information of an object in JavaScript?
You can access the constructor of an object using the `constructor` property. For example, `object.constructor` will return the function that created the instance’s prototype.
What method can I use to list all properties and methods of an object in JavaScript?
You can use `Object.getOwnPropertyNames(object)` to retrieve an array of all properties (including non-enumerable ones) and `Object.keys(object)` for only enumerable properties.
Is there a way to see the prototype chain of an object in JavaScript?
Yes, you can use `Object.getPrototypeOf(object)` to access the prototype of an object, and you can traverse the prototype chain using this method recursively.
Can I output the constructor information of all objects within an array?
Yes, you can iterate through the array using `forEach()` and log the constructor of each object with `console.log(object.constructor.name)`.
How do I check if an object is an instance of a specific constructor function?
You can use the `instanceof` operator. For example, `object instanceof ConstructorFunction` will return `true` if the object is an instance of the specified constructor.
What tools can help visualize object constructor information in JavaScript?
You can use browser developer tools, such as the console in Chrome or Firefox, which allows you to inspect objects and view their constructors and prototypes in a structured format.
In JavaScript, understanding and outputting object constructor information is essential for debugging and enhancing code clarity. Constructors are functions that create and initialize objects, and recognizing their properties and methods can significantly improve the development process. By utilizing tools such as the `constructor` property, `Object.getPrototypeOf()`, and the `instanceof` operator, developers can efficiently retrieve and display the constructor details of any object.
Moreover, the `console.log()` function can be employed to output comprehensive information about an object’s constructor, including its prototype and inherited properties. This practice not only aids in identifying the structure and behavior of objects but also assists in ensuring that the correct constructors are being used throughout the codebase. Utilizing these methods fosters a deeper understanding of JavaScript’s prototypal inheritance model.
In summary, effectively outputting object constructor information in JavaScript requires a combination of built-in properties and methods. By mastering these techniques, developers can enhance their coding practices, leading to more robust and maintainable applications. Emphasizing the importance of constructor knowledge will ultimately contribute to a more efficient development workflow.
Author Profile
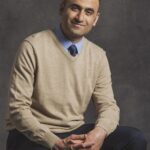
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?