How Can You Remove New Lines from a String in Python?
In the world of programming, handling strings efficiently is a fundamental skill that every developer should master. One common challenge that often arises is the presence of unwanted new lines within strings. Whether you’re processing user input, reading data from files, or scraping content from the web, these extraneous line breaks can disrupt your workflow and lead to unexpected results. In Python, a versatile and powerful language, there are several techniques to effectively remove new lines from strings, ensuring your data remains clean and manageable.
Understanding how to manipulate strings in Python not only enhances your coding proficiency but also empowers you to tackle a variety of data processing tasks with ease. New lines can manifest in various forms, such as `\n` or `\r\n`, and knowing how to identify and eliminate them is crucial for maintaining the integrity of your data. By employing built-in string methods and regular expressions, you can streamline your code and enhance its readability, making it easier to maintain and debug.
As we delve into the methods for removing new lines from strings, you’ll discover practical solutions that cater to different scenarios. From simple replacements to more complex regex patterns, the following sections will equip you with the knowledge and tools needed to keep your strings tidy and your applications running smoothly. Get ready to transform your string manipulation skills and elevate
Using the `replace()` Method
The `replace()` method is a straightforward way to remove new lines from a string in Python. It allows you to specify the substring you want to replace and what you want to replace it with. In the case of new lines, you can replace them with an empty string.
“`python
text_with_new_lines = “Hello,\nWorld!\nWelcome to Python.”
cleaned_text = text_with_new_lines.replace(“\n”, “”)
print(cleaned_text) Output: Hello,World!Welcome to Python.
“`
This method is efficient and works well for simple string manipulations. It can be used for any character or substring, not just new lines.
Using the `join()` and `split()` Methods
Another effective approach to remove new lines is by using the `split()` method in combination with `join()`. The `split()` method divides the string into a list of substrings based on the specified delimiter, while `join()` concatenates them back into a single string.
“`python
text_with_new_lines = “Hello,\nWorld!\nWelcome to Python.”
cleaned_text = “”.join(text_with_new_lines.split(“\n”))
print(cleaned_text) Output: Hello,World!Welcome to Python.
“`
This method allows for more complex manipulations, such as adding spaces or other characters between the concatenated elements.
Using Regular Expressions
For more advanced scenarios, particularly when dealing with multiple whitespace characters or various types of new lines, Python’s `re` module offers a powerful solution. The `re.sub()` function can be employed to substitute new lines with a specified string or remove them entirely.
“`python
import re
text_with_new_lines = “Hello,\nWorld!\nWelcome to Python.”
cleaned_text = re.sub(r’\n+’, ”, text_with_new_lines)
print(cleaned_text) Output: Hello,World!Welcome to Python.
“`
This method is particularly useful when you need to handle different newline formats (like `\r\n` or `\r` in addition to `\n`).
Comparison of Methods
The choice of method depends on the specific requirements of your application. Here’s a comparison of the discussed methods:
Method | Complexity | Use Case |
---|---|---|
replace() | Simple | Basic newline removal |
split() and join() | Moderate | Custom concatenation |
Regular Expressions | Advanced | Multiple newline formats or patterns |
Each method has its advantages, and selecting the right one can enhance the efficiency and readability of your code.
Methods to Remove New Lines from a String in Python
In Python, there are several effective methods to remove new line characters (`\n`) from strings. These methods cater to different use cases, depending on whether you want to remove all new lines, just leading or trailing new lines, or replace them with spaces. Below are the most common approaches.
Using the `str.replace()` Method
The `str.replace()` method is a straightforward way to replace occurrences of a substring in a string with another substring. To remove new lines, you can replace them with an empty string.
“`python
text = “Hello\nWorld\nThis is a test.”
cleaned_text = text.replace(‘\n’, ”)
print(cleaned_text) Output: HelloWorldThis is a test.
“`
Using the `str.split()` and `str.join()` Methods
This method involves splitting the string into a list of substrings using the new line as a delimiter and then joining them back together without new lines.
“`python
text = “Hello\nWorld\nThis is a test.”
cleaned_text = ”.join(text.split(‘\n’))
print(cleaned_text) Output: HelloWorldThis is a test.
“`
Using the `str.strip()` Method
To remove leading and trailing new line characters, the `str.strip()` method is effective. It can also be used with `lstrip()` and `rstrip()` to target specific sides.
“`python
text = “\nHello World\n”
cleaned_text = text.strip()
print(cleaned_text) Output: Hello World
“`
Using Regular Expressions
For more complex scenarios, the `re` module can be used. This is particularly useful if you want to remove new lines while considering other whitespace characters.
“`python
import re
text = “Hello\nWorld\nThis is a test.”
cleaned_text = re.sub(r’\n+’, ‘ ‘, text)
print(cleaned_text) Output: Hello World This is a test.
“`
Comparison of Methods
Method | Description | Use Case |
---|---|---|
`str.replace()` | Replaces all instances of `\n` with specified string. | When you want to remove all new lines. |
`str.split()` + `str.join()` | Splits string and joins without new lines. | When you need more control over formatting. |
`str.strip()` | Removes leading and trailing new lines. | When you only want to clean edges. |
Regular Expressions (`re`) | Flexible pattern matching for complex replacements. | When dealing with various whitespace. |
These methods provide flexibility in handling new lines in Python strings, catering to different formatting needs and preferences.
Expert Insights on Removing New Lines from Strings in Python
Dr. Emily Carter (Senior Software Engineer, CodeSolutions Inc.). “To effectively remove new lines from a string in Python, the most straightforward method is to use the `replace()` function. This allows developers to specify the newline character and replace it with an empty string, thereby cleaning up the text efficiently.”
Michael Thompson (Python Developer and Author, Pythonic Programming). “Utilizing the `re` module for regular expressions can offer a powerful alternative for removing new lines. By employing `re.sub()`, developers can target not only newline characters but also other whitespace characters, providing a more comprehensive solution.”
Sarah Johnson (Data Scientist, Analytics Hub). “In data preprocessing, it is crucial to handle new lines properly. Using the `strip()` method can be beneficial when you want to remove new lines from the beginning and end of a string, while `splitlines()` followed by `join()` can be used to eliminate new lines throughout the string.”
Frequently Asked Questions (FAQs)
How can I remove new lines from a string in Python?
You can remove new lines from a string using the `replace()` method. For example, `string.replace(‘\n’, ”)` will replace all newline characters with an empty string.
What is the difference between `strip()`, `rstrip()`, and `lstrip()` methods in Python?
The `strip()` method removes new lines and whitespace from both ends of a string, while `rstrip()` removes them from the right end and `lstrip()` from the left end only.
Can I use regular expressions to remove new lines from a string?
Yes, you can use the `re` module. The expression `re.sub(r’\n+’, ”, string)` will replace all occurrences of new lines with an empty string.
Is there a way to remove new lines while preserving spaces in a string?
Yes, you can use `string.replace(‘\n’, ‘ ‘)` to replace new lines with a space, thus preserving the spacing in the string.
What happens if I have multiple consecutive new lines in a string?
Using `replace()` or `re.sub()` will remove all consecutive new lines, but if you want to replace them with a single space, you can use `re.sub(r’\n+’, ‘ ‘, string)`.
Are there any performance considerations when removing new lines from large strings?
For large strings, using `re.sub()` may be more efficient for multiple replacements, as it processes the string in a single pass compared to multiple calls to `replace()`.
In Python, removing new lines from a string can be accomplished through various methods, each suited to different scenarios. The most common approaches include using the `str.replace()` method, the `str.join()` method combined with `str.split()`, and regular expressions through the `re` module. Each of these methods effectively eliminates newline characters, allowing for cleaner string manipulation and formatting.
The `str.replace()` method is straightforward and ideal for simple cases where you want to replace newline characters (`\n`) with an empty string. This method is easy to implement and understand, making it a popular choice for many developers. On the other hand, using `str.join()` with `str.split()` is particularly useful when you want to remove all types of whitespace, not just new lines. This method splits the string into a list of words and then joins them back together without any whitespace.
For more complex scenarios, especially when dealing with different types of line breaks (like `\r\n` or `\r`), regular expressions provide a powerful and flexible solution. The `re.sub()` function allows for pattern matching and can remove all forms of newline characters in one go. This method is beneficial when processing strings from various sources where newline formats may
Author Profile
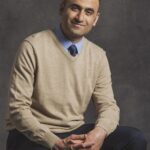
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?