How Can You Save a File in Python? A Step-by-Step Guide
In the realm of programming, the ability to effectively manage data is paramount, and saving files is one of the fundamental skills every Python developer should master. Whether you’re working on a simple script or a complex application, knowing how to save your work is essential for preserving your results and ensuring that your programs can interact with data seamlessly. In this article, we’ll delve into the various methods and best practices for saving files in Python, empowering you to handle data with confidence and efficiency.
Saving files in Python is a straightforward process, yet it offers a wealth of functionality that can cater to diverse needs. From text files to more structured formats like JSON and CSV, Python provides built-in functions and libraries that simplify file handling. Understanding these methods not only enhances your coding skills but also opens the door to more advanced data manipulation techniques.
As we explore the different approaches to file saving, you’ll discover how to create, read, and write files with ease. We will also touch on important considerations such as file paths, modes of operation, and error handling, ensuring you have a well-rounded understanding of the topic. By the end of this article, you will be equipped with the knowledge to save files effectively in Python, laying the groundwork for more complex data-driven projects.
Saving Text Files
To save text files in Python, you typically use the built-in `open()` function along with the `write()` method. Here’s a straightforward way to accomplish this:
“`python
with open(‘filename.txt’, ‘w’) as file:
file.write(“Hello, World!”)
“`
In this example, the `open()` function is used to create a new file named `filename.txt` in write mode (`’w’`). The `with` statement ensures that the file is properly closed after its suite finishes, even if an exception is raised.
Key points to remember:
- Use `’w’` mode to create a new file or overwrite an existing file.
- Use `’a’` mode to append to an existing file without erasing its content.
Saving Binary Files
For saving binary files, such as images or executable files, the process is similar but requires the use of binary mode. Here’s an example of saving a binary file:
“`python
with open(‘image.png’, ‘wb’) as binary_file:
binary_file.write(binary_data)
“`
In this case, the file is opened in binary write mode (`’wb’`), allowing you to write binary data directly to the file.
Saving JSON Files
JSON files are commonly used for data interchange. You can easily save data in JSON format using the `json` module:
“`python
import json
data = {‘name’: ‘Alice’, ‘age’: 30}
with open(‘data.json’, ‘w’) as json_file:
json.dump(data, json_file)
“`
The `json.dump()` method serializes the Python dictionary `data` and writes it to `data.json`.
Saving CSV Files
To save data in CSV format, the `csv` module is utilized. Here’s an example:
“`python
import csv
data = [[‘Name’, ‘Age’], [‘Alice’, 30], [‘Bob’, 25]]
with open(‘data.csv’, ‘w’, newline=”) as csv_file:
writer = csv.writer(csv_file)
writer.writerows(data)
“`
In this code snippet, `csv.writer()` creates a writer object, and `writer.writerows()` writes multiple rows to the CSV file.
Table of File Modes
The following table summarizes the different file modes available in Python when opening files:
Mode | Description |
---|---|
‘r’ | Read (default mode). Opens a file for reading. |
‘w’ | Write. Opens a file for writing, truncating the file first. |
‘a’ | Append. Opens a file for writing, appending to the end of the file. |
‘b’ | Binary mode. Used with other modes to handle binary files. |
‘x’ | Exclusive creation. Fails if the file already exists. |
Utilizing the correct file mode is crucial for ensuring that your data is saved appropriately and without unintended loss.
Saving Text Files in Python
To save a text file in Python, you can utilize the built-in `open()` function along with the `write()` method. This approach allows you to create a new file or overwrite an existing one.
“`python
with open(‘example.txt’, ‘w’) as file:
file.write(“Hello, World!”)
“`
- ‘w’ mode: Opens the file for writing. If the file exists, it will be overwritten.
- ‘a’ mode: Opens the file for appending, allowing you to add content without deleting existing data.
Saving Binary Files
When dealing with binary files, such as images or audio files, the process is similar but requires opening the file in binary mode.
“`python
with open(‘example.png’, ‘wb’) as file:
file.write(binary_data)
“`
- ‘wb’ mode: Opens the file for writing in binary format.
- ‘ab’ mode: Opens the file for appending in binary format.
Using JSON for Data Storage
Python provides a convenient way to save data structures as JSON files using the `json` module.
“`python
import json
data = {‘name’: ‘Alice’, ‘age’: 30}
with open(‘data.json’, ‘w’) as file:
json.dump(data, file)
“`
- `json.dump()`: Serializes a Python object and writes it to a file.
- `json.dumps()`: Serializes a Python object and returns it as a string.
Saving CSV Files
For storing tabular data, the `csv` module is ideal. You can save data in a comma-separated format easily.
“`python
import csv
data = [[‘Name’, ‘Age’], [‘Alice’, 30], [‘Bob’, 25]]
with open(‘data.csv’, ‘w’, newline=”) as file:
writer = csv.writer(file)
writer.writerows(data)
“`
- `csv.writer()`: Creates a writer object that converts data into a delimited string.
- `writerows()`: Writes multiple rows at once.
Saving Pickle Files
For serializing and saving complex Python objects, you can use the `pickle` module.
“`python
import pickle
data = {‘name’: ‘Alice’, ‘age’: 30}
with open(‘data.pkl’, ‘wb’) as file:
pickle.dump(data, file)
“`
- `pickle.dump()`: Serializes a Python object and writes it to a binary file.
- `pickle.load()`: Loads the object back from the file.
Handling File Paths
When saving files, consider using the `os` module to manage file paths effectively.
“`python
import os
file_path = os.path.join(‘directory’, ‘example.txt’)
with open(file_path, ‘w’) as file:
file.write(“Hello, World!”)
“`
- `os.path.join()`: Ensures correct path separators are used across different operating systems.
Error Handling
To ensure robust file operations, implement error handling using try-except blocks.
“`python
try:
with open(‘example.txt’, ‘w’) as file:
file.write(“Hello, World!”)
except IOError as e:
print(f”An error occurred: {e}”)
“`
- `IOError`: Catches errors related to file operations, helping to identify issues like permission errors or file not found situations.
Expert Insights on Saving Files in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When saving a file in Python, it is essential to utilize the built-in `open()` function, specifying the mode as ‘w’ for writing. This ensures that the file is created if it does not exist or overwritten if it does. Proper exception handling should also be implemented to manage any potential errors during the file operation.”
James Thompson (Lead Python Developer, CodeCraft Solutions). “For efficient file management in Python, I recommend using the `with` statement when opening files. This approach automatically handles file closing, which is crucial for preventing resource leaks and ensuring data integrity. Additionally, using the `json` module for saving structured data can greatly simplify the process.”
Linda Garcia (Data Scientist, Analytics Hub). “In data science applications, saving files in formats like CSV or JSON is common. Utilizing libraries such as `pandas` can significantly streamline this process. For example, the `to_csv()` method allows for easy export of DataFrames to CSV files, which is invaluable for data sharing and analysis.”
Frequently Asked Questions (FAQs)
How do I save a text file in Python?
To save a text file in Python, use the built-in `open()` function with the ‘w’ mode to create a new file or overwrite an existing one. Write content using the `write()` method, and close the file with `close()`. For example:
“`python
with open(‘filename.txt’, ‘w’) as file:
file.write(‘Hello, World!’)
“`
What is the difference between ‘w’ and ‘a’ modes in file handling?
The ‘w’ mode opens a file for writing and truncates the file to zero length if it exists, effectively overwriting it. The ‘a’ mode opens a file for appending, allowing you to add content to the end of the file without deleting existing data.
How can I save a file in a specific directory using Python?
To save a file in a specific directory, provide the full path in the `open()` function. For example:
“`python
with open(‘/path/to/directory/filename.txt’, ‘w’) as file:
file.write(‘Hello, World!’)
“`
Can I save binary files in Python?
Yes, you can save binary files in Python by using the ‘wb’ mode in the `open()` function. This mode allows you to write binary data. For example:
“`python
with open(‘image.png’, ‘wb’) as file:
file.write(binary_data)
“`
What should I do if I encounter a permission error while saving a file?
If you encounter a permission error, ensure that you have the necessary write permissions for the directory where you are trying to save the file. You may also consider running your script with elevated permissions or choosing a different directory.
How can I save data from a list into a file in Python?
To save data from a list into a file, iterate through the list and write each item to the file. You can join the list items into a string if needed. For example:
“`python
data = [‘item1’, ‘item2’, ‘item3’]
with open(‘output.txt’, ‘w’) as file:
file.write(‘\n’.join(data))
“`
In summary, saving a file in Python is a straightforward process that can be accomplished using built-in functions and libraries. The most common method involves using the `open()` function to create or open a file, followed by the `write()` method to write data to it. This process is essential for data persistence, allowing users to store information generated by their programs for future access.
Additionally, Python provides various modes for file handling, such as ‘w’ for writing, ‘a’ for appending, and ‘r’ for reading. Understanding these modes is crucial for effective file management. Moreover, utilizing the `with` statement is recommended for file operations, as it ensures that files are properly closed after their suite finishes, thus preventing potential data loss or corruption.
Key takeaways from this discussion include the importance of choosing the correct file mode based on the intended operation and the benefits of using context managers to handle files efficiently. By adhering to these practices, users can enhance the reliability and performance of their file-saving operations in Python.
Author Profile
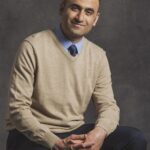
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?