How Can You Call a JavaScript Function in HTML?
### Introduction
In the dynamic world of web development, JavaScript stands out as a powerful tool that brings interactivity and functionality to websites. Whether you’re looking to create a responsive user interface, validate forms, or enhance user experience, understanding how to effectively call JavaScript functions within HTML is crucial. This process not only allows developers to manipulate the Document Object Model (DOM) but also enables them to respond to user actions, making web pages more engaging and intuitive.
As you dive into the intricacies of integrating JavaScript with HTML, you’ll discover that the methods for invoking functions can vary based on the context and requirements of your project. From inline event handlers to external script files, each approach has its own advantages and best practices. By mastering these techniques, you’ll be equipped to create seamless interactions that elevate your web applications and captivate your audience.
In this article, we will explore the various ways to call JavaScript functions in HTML, shedding light on the fundamental concepts and practical applications. Whether you’re a seasoned developer or just starting your coding journey, understanding these principles will empower you to harness the full potential of JavaScript and enhance your web development skills. Get ready to unlock the secrets of function invocation and take your web projects to the next level!
Invoking JavaScript Functions in HTML
To call a JavaScript function from HTML, you can use several methods depending on the context in which you want to execute the function. This can include responding to user events such as clicks or loading a page. Below are some common approaches to invoke JavaScript functions directly from your HTML.
Using Inline Event Handlers
One of the simplest ways to call a JavaScript function is by using inline event handlers. You can add an event attribute directly to an HTML element, which will execute the specified JavaScript function when the event occurs.
In this example, clicking the button will call the `myFunction()` JavaScript function. Inline event handlers can be used with various events such as `onclick`, `onmouseover`, and `onload`.
Using the DOM to Add Event Listeners
Alternatively, you can use JavaScript to add event listeners, which is a more modern approach. This method separates your JavaScript from your HTML and can improve code maintainability.
In this example, the `addEventListener` method is used to call `myFunction` when the button is clicked.
Calling Functions on Page Load
If you want to call a function as soon as the page loads, you can use the `onload` event of the `
` tag or the `DOMContentLoaded` event in JavaScript.…
Or using JavaScript:
Both methods ensure that `myFunction()` is executed once the page’s content is fully loaded.
Passing Arguments to Functions
You can also pass arguments to JavaScript functions when calling them from HTML. This can be done through inline event handlers or when using `addEventListener`.
Or with `addEventListener`:
document.getElementById(“myButton”).addEventListener(“click”, function() {
myFunction(‘Hello’);
});
Table of Event Attributes
Here is a table that summarizes common HTML event attributes that can be used to call JavaScript functions:
Event | Description | Example |
---|---|---|
onclick | Triggers when the element is clicked. | onclick="myFunction()" |
onmouseover | Triggers when the mouse pointer hovers over the element. | onmouseover="myFunction()" |
onload | Triggers when the page has finished loading. | onload="myFunction()" |
onchange | Triggers when the value of an element changes. | onchange="myFunction()" |
By utilizing these methods, you can effectively integrate JavaScript functions into your HTML, enhancing interactivity and functionality within your web applications.
Methods to Call a JavaScript Function in HTML
Calling a JavaScript function from HTML can be accomplished through various methods, including inline event handlers, the `addEventListener` method, and using the `onload` event for the body tag. Below are detailed descriptions of each method.
Inline Event Handlers
Inline event handlers allow you to call JavaScript functions directly within HTML tags. This method is straightforward but can lead to less maintainable code if overused.
- Advantages:
- Simple and quick to implement.
- Immediate visibility of the function call in HTML.
- Disadvantages:
- Can lead to cluttered HTML.
- Harder to maintain and debug.
Using `addEventListener` Method
The `addEventListener` method is a more modern approach that allows for better separation of JavaScript and HTML, enhancing readability and maintainability.
- Advantages:
- Cleaner separation of HTML and JavaScript.
- Multiple event listeners can be added to the same element.
- Disadvantages:
- Requires understanding of JavaScript to implement.
- Slightly more complex than inline handlers.
Using the `onload` Event
The `onload` event can be used to call a function when the webpage loads. This is useful for initializing scripts or setting up the environment.
Welcome to the Page
- Advantages:
- Automatically calls the function upon loading.
- Useful for setup tasks without user interaction.
- Disadvantages:
- The function will run automatically, which may not be desirable in all cases.
- Less control over when the function is executed.
Table of Comparison
Method | Advantages | Disadvantages |
---|---|---|
Inline Event Handlers | Simple to implement | Can clutter HTML, hard to maintain |
`addEventListener` | Clean separation, multiple listeners | Requires JavaScript knowledge |
`onload` Event | Automatically calls on page load | Runs automatically, less control |
Expert Insights on Calling JavaScript Functions in HTML
Dr. Emily Carter (Senior Web Developer, Tech Innovations Inc.). “To effectively call JavaScript functions in HTML, one can utilize the ‘onclick’ attribute in HTML elements. This method allows for a seamless integration of JavaScript with user interactions, enhancing the overall user experience.”
Michael Chen (JavaScript Educator, Code Academy). “Using the ‘script’ tag within the HTML file is crucial for defining JavaScript functions. Once declared, these functions can be invoked directly through event handlers or by calling them in the console, providing flexibility in web development.”
Sarah Patel (Front-End Engineer, Digital Solutions Group). “It is essential to ensure that the JavaScript functions are loaded before they are called. Placing the script tag at the end of the body or using the ‘defer’ attribute can prevent errors and ensure that the DOM is fully loaded before executing any JavaScript.”
Frequently Asked Questions (FAQs)
How do I call a JavaScript function from an HTML button?
You can call a JavaScript function from an HTML button by using the `onclick` attribute. For example: ``, where `myFunction` is the name of your JavaScript function.
Can I call a JavaScript function when the page loads?
Yes, you can call a JavaScript function when the page loads by using the `onload` event in the `
What is the correct way to define a JavaScript function?
A JavaScript function can be defined using the `function` keyword followed by the function name and parentheses. For example: `function myFunction() { /* code here */ }`.
How can I pass parameters to a JavaScript function from HTML?
You can pass parameters to a JavaScript function by including them in the `onclick` attribute. For example: ``.
Is it possible to call a JavaScript function from an HTML link?
Yes, you can call a JavaScript function from an HTML link by using the `href` attribute with `javascript:` syntax or by using the `onclick` event. For example: `Link Text`.
What is the difference between inline and external JavaScript function calls?
Inline JavaScript function calls are embedded directly within HTML elements using attributes like `onclick`, while external JavaScript calls are made by linking to a separate `.js` file using the `` tag, allowing for cleaner code and separation of concerns.
In summary, calling a JavaScript function in HTML is a fundamental aspect of web development that allows for dynamic interaction on web pages. This can be accomplished in several ways, including using event attributes directly in HTML elements, employing the `addEventListener` method in JavaScript, or integrating JavaScript with frameworks and libraries that enhance functionality. Each method has its own use cases and advantages, depending on the complexity of the application and the desired user experience.
One key takeaway is the importance of understanding the context in which JavaScript functions are called. Inline event handlers can be straightforward for simple tasks, but they may lead to less maintainable code as applications grow. Conversely, using JavaScript to attach event listeners promotes better separation of concerns, making it easier to manage and update code in larger projects.
Additionally, leveraging modern JavaScript features and frameworks can significantly streamline the process of calling functions and handling events. Tools like React, Angular, or Vue.js provide structured ways to manage interactions, which can enhance both performance and user experience. As web technologies continue to evolve, staying informed about best practices in function calling will be essential for effective web development.
Author Profile
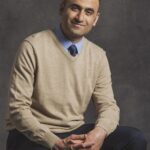
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?