What Is the Boolean Data Type in MySQL and How Do You Use It?
In the world of databases, the way we handle data types can significantly impact the efficiency and functionality of our applications. Among these data types, the `bool` or boolean data type stands out as a fundamental building block for logical operations and decision-making processes. While many developers may be familiar with boolean logic from programming languages, its implementation in MySQL offers unique characteristics and considerations that are essential for effective database design. In this article, we will delve into the boolean data type in MySQL, exploring its definition, usage, and the nuances that set it apart from other data types.
Overview of the Boolean Data Type in MySQL
MySQL does not have a dedicated boolean data type, but it provides a way to represent boolean values using the `TINYINT` data type. In this context, boolean values are typically represented as `0` for and `1` for true. This representation allows developers to seamlessly integrate logical conditions into their queries and manage state information within their databases. Understanding how MySQL interprets and handles these values is crucial for ensuring data integrity and optimizing query performance.
As we explore the boolean data type further, we will also discuss best practices for using boolean values in MySQL, including how to effectively query and manipulate data based on
Understanding the Boolean Data Type in MySQL
In MySQL, the Boolean data type is not explicitly defined as a separate type; instead, it is represented as a TINYINT. The Boolean type can take on values that represent true or , which are typically denoted as 1 and 0, respectively. This approach allows for flexibility in how logical values are stored and manipulated within the database.
Boolean Representation
Although MySQL does not have a native Boolean data type, it can effectively handle boolean logic through the following conventions:
- True is represented as `1`
- is represented as `0`
This implementation allows developers to use standard numeric operations on boolean values while maintaining clarity in their logical expressions.
Creating a Boolean-Like Column
To create a column that functions similarly to a Boolean data type, you can define a column with the TINYINT type. Here is an example SQL statement:
“`sql
CREATE TABLE example_table (
id INT AUTO_INCREMENT PRIMARY KEY,
is_active TINYINT(1) NOT NULL
);
“`
In this example, the `is_active` column is intended to store boolean-like values, where `1` indicates that the record is active, and `0` indicates that it is not.
Usage in Queries
Using boolean-like values in queries is straightforward. You can easily filter records based on these values. For instance, to select all active records from the `example_table`, you can execute the following SQL statement:
“`sql
SELECT * FROM example_table WHERE is_active = 1;
“`
Boolean Operations
MySQL supports various logical operations that can be performed on boolean values. The common logical operators include:
– **AND**: Returns true if both operands are true.
– **OR**: Returns true if at least one operand is true.
– **NOT**: Returns true if the operand is .
You can use these operators in combination with boolean-like columns in your queries. For example:
“`sql
SELECT * FROM example_table WHERE is_active = 1 AND id > 5;
“`
Table of Boolean Representations
The following table summarizes the representation of boolean values within MySQL:
Boolean Value | MySQL Representation |
---|---|
True | 1 |
0 |
Best Practices
When using boolean-like data in MySQL, consider the following best practices:
- Use TINYINT(1): This is the conventional way to represent boolean values.
- Be Consistent: Ensure that your application logic consistently uses `1` and `0` for true and .
- Indexing: If you frequently query boolean columns, consider indexing them to improve performance.
By adhering to these guidelines, you can effectively utilize boolean-like functionality in MySQL to manage logical data efficiently.
Understanding the Boolean Data Type in MySQL
MySQL does not have a dedicated Boolean data type. Instead, it utilizes the `TINYINT` data type to represent Boolean values. In this context, a value of `0` typically denotes “, while any non-zero value, most commonly `1`, represents `TRUE`.
Storage and Representation
The `TINYINT` data type is a single-byte integer that can store values from `-128` to `127` when signed, or from `0` to `255` when unsigned. However, for Boolean purposes, the following conventions are usually adopted:
- 0: “
- 1: `TRUE`
This allows for efficient storage while providing a clear representation of Boolean logic.
Creating Boolean Columns
When defining a column meant to store Boolean values, you can use the `TINYINT` data type in your table schema. Here is an example of how to create a table with a Boolean column:
“`sql
CREATE TABLE example_table (
id INT AUTO_INCREMENT PRIMARY KEY,
is_active TINYINT(1) NOT NULL
);
“`
In this example, the `is_active` column is intended to represent a Boolean value indicating whether an entry is active or not.
Inserting Boolean Values
When inserting data into a table with a Boolean column, you can use either `0` or `1` directly, or you may use the keywords `TRUE` and “, which MySQL automatically converts to the corresponding numeric values. Here’s how you can insert values:
“`sql
INSERT INTO example_table (is_active) VALUES (1); — TRUE
INSERT INTO example_table (is_active) VALUES (0); —
INSERT INTO example_table (is_active) VALUES (TRUE); — TRUE
INSERT INTO example_table (is_active) VALUES (); —
“`
Querying Boolean Values
Querying the Boolean values stored as `TINYINT` is straightforward. You can use standard SQL syntax to filter results based on the Boolean values:
“`sql
SELECT * FROM example_table WHERE is_active = 1; — Fetches all active entries
SELECT * FROM example_table WHERE is_active = 0; — Fetches all inactive entries
“`
Best Practices
When working with Boolean values in MySQL, consider the following best practices:
- Use TINYINT(1): This is the standard practice for representing Boolean values.
- Index Boolean Columns: If a Boolean column is frequently queried, consider indexing it to improve performance.
- Avoid Using NULL: For Boolean columns, it is often better to avoid NULL values to maintain clear logic in your application.
Conclusion on Boolean Handling
In summary, while MySQL does not provide a specific Boolean data type, it effectively handles Boolean logic using the `TINYINT` data type. This approach allows for flexible and efficient storage of Boolean values within databases.
Understanding the Boolean Data Type in MySQL
Dr. Emily Carter (Database Architect, Tech Innovations Inc.). “The boolean data type in MySQL is represented using TINYINT(1), where a value of 0 signifies and 1 signifies true. This approach allows for efficient storage and easy manipulation of binary data.”
Mark Thompson (Senior Software Engineer, Data Solutions Group). “While MySQL does not have a dedicated BOOLEAN type, using TINYINT(1) effectively serves the purpose. It is crucial for developers to understand this to avoid confusion and ensure proper data handling.”
Linda Zhang (MySQL Database Consultant, Bright Data Services). “When designing databases, it is essential to utilize the boolean representation wisely. Using TINYINT(1) can enhance query performance and reduce storage requirements, making it a practical choice for applications requiring binary states.”
Frequently Asked Questions (FAQs)
What is the boolean data type in MySQL?
The boolean data type in MySQL is a synonym for the TINYINT data type, where 0 represents and 1 represents true.
How do you define a boolean column in a MySQL table?
You can define a boolean column in a MySQL table using the syntax: `column_name TINYINT(1)`. This allows for the storage of boolean values.
Can MySQL store values other than 0 and 1 in a boolean column?
Yes, while boolean columns are intended for 0 and 1, MySQL allows any integer value. However, only 0 and 1 will be interpreted as and true, respectively.
How can you retrieve boolean values from a MySQL database?
You can retrieve boolean values using standard SQL queries. For instance, `SELECT column_name FROM table_name WHERE column_name = 1;` retrieves all rows where the boolean value is true.
Is there a difference between BOOLEAN and BOOL in MySQL?
No, BOOLEAN and BOOL are synonyms in MySQL. Both are treated as TINYINT(1) and function identically in terms of storage and behavior.
Can you use boolean expressions in MySQL queries?
Yes, MySQL supports boolean expressions in queries. You can use logical operators such as AND, OR, and NOT to evaluate conditions involving boolean columns.
The Boolean data type in MySQL is primarily represented using the TINYINT data type, where values of 0 and 1 are used to denote and true, respectively. Although MySQL does not have a dedicated Boolean type, the use of TINYINT allows for efficient storage and manipulation of binary states. This representation aligns with the common programming conventions where Boolean values are typically expressed as binary integers. Consequently, developers can utilize this approach to implement logical conditions and control flow within their database operations.
In MySQL, the BOOLEAN and BOOL keywords are synonyms for TINYINT(1), which means they are interchangeable in SQL statements. This can lead to some confusion for those expecting a distinct Boolean type. However, the flexibility of using TINYINT allows for broader applications, such as storing additional integer values if necessary. It is essential for developers to be aware of this implementation detail to avoid misunderstandings in data handling and to ensure accurate query results.
When designing database schemas, incorporating Boolean values can significantly enhance the clarity and efficiency of data representation. It is advisable to maintain consistent practices when naming columns intended to store Boolean values, such as using prefixes like ‘is_’, ‘has_’, or ‘can_’. This not only improves code readability
Author Profile
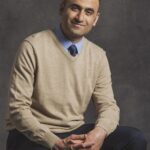
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?