How Can I Efficiently Install Multiple Chrome Extension Install Scripts?
In the ever-evolving landscape of web browsing, Chrome extensions have emerged as indispensable tools that enhance productivity, streamline workflows, and enrich the overall online experience. With thousands of extensions available, users often find themselves overwhelmed by the sheer volume of options. Fortunately, there’s a way to simplify the installation process: utilizing install scripts. These scripts allow users to install multiple Chrome extensions simultaneously, saving time and effort while customizing their browser to fit their unique needs. In this article, we will explore the world of Chrome extension install scripts, unveiling their benefits, functionalities, and how they can transform your browsing experience.
As the demand for efficiency and convenience grows, so does the need for effective methods to manage Chrome extensions. Install scripts serve as a powerful solution, enabling users to automate the installation of multiple extensions with just a few clicks. This not only minimizes the hassle of searching for each extension individually but also ensures that users can quickly set up a new browser environment or restore their preferred extensions after a reset.
In addition to streamlining the installation process, these scripts can also help users maintain consistency across devices. By creating a standardized set of extensions, users can ensure that their browsing experience remains uniform, whether they’re on a personal laptop or a work computer. As we delve deeper
Understanding Chrome Extension Installation Scripts
To streamline the process of installing multiple Chrome extensions, developers and users can leverage installation scripts. These scripts automate the installation of extensions, saving time and effort, especially when dealing with numerous extensions.
Creating an Installation Script
An installation script for Chrome extensions can be created using a simple JavaScript file that utilizes the Chrome Web Store’s API. The script will typically include a list of extension IDs or URLs and automate the installation process. Below are the steps to create a basic installation script:
- **Gather Extension IDs**: Obtain the unique IDs of the Chrome extensions you wish to install. This can be found in the extension’s URL on the Chrome Web Store.
- **Write the Script**: Create a JavaScript file that includes a function to install each extension.
Here is a sample script structure:
“`javascript
const extensions = [
“extension_id_1”,
“extension_id_2”,
“extension_id_3”
];
extensions.forEach(id => {
chrome.management.install(id, function() {
console.log(`Installed extension: ${id}`);
});
});
“`
Using a Batch Installation Method
For users who prefer a more user-friendly approach, batch installation methods can be employed. These methods can be achieved through the use of third-party tools or by utilizing Chrome’s built-in features. Below are common methods for batch installing extensions:
- Chrome Enterprise Policy: Administrators can push extensions to managed devices using policies.
- Bookmarklet: Create a bookmarklet that triggers the installation of multiple extensions when executed.
- Chrome Extension Installer: Use a third-party extension installer that allows users to select and install multiple extensions at once.
Example of an Installation Table
To assist in managing multiple extensions, a table can help organize the information regarding each extension, making it easier to track and install them.
Extension Name | Extension ID | Chrome Web Store URL |
---|---|---|
AdBlock | gighmmpiobklfepjocnamgkkbiglidom | AdBlock |
Grammarly | kbfnbcaeplbcioakkpcpgfkobkghlhen | Grammarly |
LastPass | hdcfcfkjcceoocjgfegkhkacbndfcbmj | LastPass |
By utilizing these strategies, users can effectively manage and install multiple Chrome extensions with ease. This enhances productivity and ensures that all necessary tools are readily available.
Understanding Chrome Extension Installation Scripts
Chrome extensions enhance browser functionality, but managing multiple installations can be tedious. Automation through installation scripts allows users to streamline the process. These scripts can be written in various programming languages, with JavaScript being the most common due to its native compatibility with Chrome.
Creating Installation Scripts
Installation scripts can be developed in JavaScript using the Chrome Extension APIs. The following steps outline the process:
- **Set Up Your Environment**:
- Ensure you have Node.js and npm installed for package management.
- Create a new directory for your project.
- **Initialize Your Project**:
- Run `npm init` to create a `package.json` file.
- **Install Required Packages**:
- Use `npm install` to install libraries that can facilitate automation, such as `puppeteer` or `selenium-webdriver`.
- **Develop the Script**:
- Write a script to automate the installation of extensions. Below is a sample script structure:
“`javascript
const puppeteer = require(‘puppeteer’);
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto(‘chrome://extensions’);
// Further code to manipulate the page and install extensions
await browser.close();
})();
“`
Common Installation Script Features
When creating installation scripts, consider incorporating the following features:
- Batch Installation: Ability to install multiple extensions in a single run.
- Error Handling: Catch errors during installation to ensure robustness.
- User Feedback: Provide console messages to inform users of installation progress.
Sample Installation Script for Multiple Extensions
Here is a more detailed example of a script for installing multiple Chrome extensions:
“`javascript
const puppeteer = require(‘puppeteer’);
const extensions = [
‘https://chrome.google.com/webstore/detail/extension-id1’,
‘https://chrome.google.com/webstore/detail/extension-id2’,
// Add more extensions as needed
];
(async () => {
const browser = await puppeteer.launch({ headless: });
const page = await browser.newPage();
await page.goto(‘chrome://extensions’);
await page.click(‘load-unpacked’); // Click the ‘Load unpacked’ button
for (const extension of extensions) {
await page.goto(extension);
await page.click(‘button’); // Click the install button
}
await browser.close();
})();
“`
Best Practices for Managing Scripts
To ensure your installation scripts are effective and maintainable, follow these best practices:
- Keep Scripts Modular: Organize code into functions for clarity and reusability.
- Document Code: Provide comments explaining the purpose of key sections.
- Version Control: Use Git for version control to track changes and collaborate effectively.
Security Considerations
While automating installations, be cautious of potential security risks:
- Verify Source: Ensure extensions are from reputable sources to avoid malicious software.
- Permissions Management: Regularly review and manage permissions granted to installed extensions.
Testing Your Scripts
Testing is crucial to ensure your scripts function as expected. Consider the following approaches:
- Unit Testing: Write tests for individual components of your script using frameworks like Mocha or Jest.
- Integration Testing: Test the full installation process in a controlled environment.
By following these guidelines, you can efficiently install multiple Chrome extensions through scripts, enhancing your productivity while maintaining security and reliability.
Expert Insights on Installing Multiple Chrome Extension Scripts
Dr. Emily Carter (Cybersecurity Analyst, TechSecure Labs). “When installing multiple Chrome extension scripts, it is crucial to evaluate the security risks associated with each extension. Many extensions can access sensitive data, and installing too many can increase the attack surface, making users more vulnerable to phishing and malware.”
Michael Tran (Web Development Specialist, CodeCraft). “For developers looking to install many Chrome extension scripts efficiently, leveraging automation tools like Puppeteer can streamline the process. However, it is essential to ensure that the scripts are well-optimized to prevent performance issues within the browser.”
Lisa Chen (Product Manager, Browser Innovations). “Users should be mindful of the cumulative effect of installing numerous Chrome extensions. Each extension can slow down browser performance and lead to conflicts. It is advisable to regularly review and uninstall extensions that are no longer necessary.”
Frequently Asked Questions (FAQs)
What are Chrome extension install scripts?
Chrome extension install scripts are automated scripts that facilitate the installation of multiple Chrome extensions at once. They streamline the process, saving time and effort for users who need to set up several extensions simultaneously.
How can I create a script to install multiple Chrome extensions?
To create a script for installing multiple Chrome extensions, you can use a combination of JavaScript and Chrome’s API. The script should include the extension IDs and utilize the `chrome.management` API to install them programmatically.
Are there any risks associated with using install scripts for Chrome extensions?
Yes, there are risks. Using install scripts can expose users to malicious extensions if the source is untrusted. It is essential to verify the integrity and security of the extensions before installation.
Can I install Chrome extensions in bulk without a script?
Yes, you can install Chrome extensions in bulk by visiting the Chrome Web Store and adding them one by one. However, this method is time-consuming compared to using an install script.
Is there a limit to the number of extensions I can install using a script?
While technically there is no strict limit imposed by Chrome on the number of extensions, users may experience performance issues or browser instability if too many extensions are installed simultaneously.
Where can I find reliable install scripts for Chrome extensions?
Reliable install scripts can often be found on reputable coding forums, GitHub repositories, or developer documentation. Always ensure to review the code and check user feedback to confirm its safety and effectiveness.
the process of installing multiple Chrome extensions can be streamlined through the use of install scripts. These scripts allow users to automate the installation process, saving time and effort, particularly for those who manage multiple devices or require a consistent setup across various environments. By utilizing such scripts, users can ensure that all necessary extensions are installed efficiently, reducing the risk of human error and enhancing productivity.
Moreover, it is essential to consider the security implications of using install scripts. Users should ensure that the sources of the scripts are reputable and that the extensions being installed do not compromise their privacy or security. It is advisable to review the permissions required by each extension and to keep the installed extensions updated to mitigate potential vulnerabilities.
Finally, leveraging install scripts for Chrome extensions not only simplifies the installation process but also facilitates easier management of these tools. Users can create customized scripts tailored to their specific needs, enabling them to maintain an organized and efficient browsing experience. Overall, the strategic use of install scripts can significantly enhance the usability and functionality of Chrome, making it a powerful tool for both personal and professional use.
Author Profile
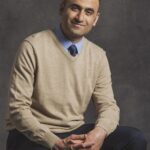
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?