Why Am I Getting an ‘xlrd.biffh.XLRDError: Excel XLSX File Not Supported’ Error?
In the world of data analysis and manipulation, Excel files have become a cornerstone for professionals across various industries. However, as technology evolves, so do the tools we use to interact with these files. One common hurdle many encounter is the `xlrd.biffh.XLRDError`, particularly when attempting to open `.xlsx` files. This error can be frustrating, especially for those who rely on Python libraries for data processing. Understanding the root cause of this issue is essential for any data enthusiast looking to streamline their workflow and avoid unnecessary roadblocks.
The `XLRDError` typically arises from compatibility issues with the `xlrd` library, which was originally designed to read older Excel file formats. As the `.xlsx` format gained popularity, users began to notice that attempts to open these newer files often resulted in this perplexing error message. While the transition to newer libraries seems like a straightforward solution, it can lead to confusion about which tools to use and how to implement them effectively.
In this article, we will delve into the intricacies of the `xlrd.biffh.XLRDError` and explore the reasons behind its occurrence with `.xlsx` files. We will also highlight alternative libraries and best practices that can help you navigate these challenges,
Understanding xlrd.biffh.XLRDError
When working with Excel files in Python, the `xlrd` library is often used for reading data from `.xls` (Excel 97-2003) files. However, users may encounter the `XLRDError` when attempting to open `.xlsx` files (Excel 2007 and later). This error indicates that the `xlrd` library does not support the `.xlsx` file format.
The reason for this limitation is that as of version 2.0.0, `xlrd` has removed support for `.xlsx` files entirely. Therefore, trying to use `xlrd` to read these newer Excel formats will result in the following error:
“`
XLRDError: Excel xlsx file not supported
“`
Alternatives for Reading .xlsx Files
To work with `.xlsx` files, users should consider alternative libraries that are designed to handle this file format. Here are some recommended options:
- openpyxl: A popular library for reading and writing `.xlsx` files. It allows for manipulation of Excel files and supports features like formulas, charts, and images.
- pandas: This powerful data manipulation library can read `.xlsx` files using `openpyxl` or `xlsxwriter` as its engine. It is particularly useful for data analysis.
- xlsxwriter: Primarily used for writing `.xlsx` files, it is also capable of reading files in conjunction with other libraries.
Sample Code for Reading .xlsx Files
Here’s how to read an `.xlsx` file using both `openpyxl` and `pandas`:
“`python
Using openpyxl
import openpyxl
workbook = openpyxl.load_workbook(‘example.xlsx’)
sheet = workbook.active
for row in sheet.iter_rows(values_only=True):
print(row)
Using pandas
import pandas as pd
df = pd.read_excel(‘example.xlsx’)
print(df)
“`
Comparison of Libraries for Excel File Handling
The table below outlines the differences between the libraries mentioned:
Library | File Types Supported | Reading Capability | Writing Capability | Additional Features |
---|---|---|---|---|
xlrd | .xls | Yes | No | Basic data reading |
openpyxl | .xlsx | Yes | Yes | Formulas, Charts, Images |
pandas | .xls, .xlsx | Yes (with engines) | Yes (with engines) | Data manipulation, Analysis |
xlsxwriter | .xlsx | No | Yes | Charting, Formatting, Conditional formatting |
By selecting the appropriate library based on your specific needs and the file format you are working with, you can effectively manage and manipulate Excel data within your Python applications.
Understanding the xlrd Library and Its Limitations
The `xlrd` library is a popular Python package primarily used for reading data from Excel files. However, recent updates have introduced limitations regarding file compatibility, particularly with the `.xlsx` format.
- Supported Formats:
- `.xls` files (Excel 97-2003)
- `.xlsb` files (Excel Binary)
- Unsupported Formats:
- `.xlsx` files (Excel 2007 and later)
The library raises an `XLRDError` if an attempt is made to open an `.xlsx` file, indicating that this format is not supported.
Causes of XLRDError
The `XLRDError` is typically encountered under the following circumstances:
- Attempting to read an `.xlsx` file using the `xlrd` library.
- Using an outdated version of `xlrd` that does not support newer Excel formats.
The error message usually appears as follows:
`XLRDError: Excel xlsx file not supported.`
Alternatives to xlrd for Reading .xlsx Files
Given the limitations of `xlrd`, users need to consider alternative libraries that can handle `.xlsx` files effectively. The following options are recommended:
- openpyxl:
- Designed for reading and writing `.xlsx` files.
- Supports advanced features like charts and images.
- pandas:
- Utilizes `openpyxl` as a backend for reading `.xlsx` files.
- Provides a versatile DataFrame structure for data manipulation and analysis.
- xlsxwriter:
- Focuses on writing `.xlsx` files.
- Ideal for creating new Excel files with formatting options.
Using openpyxl to Read .xlsx Files
To read `.xlsx` files using the `openpyxl` library, follow these steps:
- Install openpyxl:
Use pip to install the library:
“`bash
pip install openpyxl
“`
- Reading an .xlsx file:
Here is a simple example to read data from an `.xlsx` file:
“`python
import openpyxl
Load the workbook
workbook = openpyxl.load_workbook(‘example.xlsx’)
Select a sheet
sheet = workbook.active
Read a cell value
value = sheet[‘A1’].value
print(value)
“`
Comparison of Libraries for Excel File Handling
The following table summarizes the capabilities of the discussed libraries for handling Excel files:
Library | .xls Support | .xlsx Support | Writing Capability | Additional Features |
---|---|---|---|---|
xlrd | Yes | No | No | Basic file reading |
openpyxl | No | Yes | Yes | Charts, images, advanced formatting |
pandas | Yes (via xlrd) | Yes (via openpyxl) | Yes | Data manipulation, analysis |
xlsxwriter | No | Yes | Yes | Extensive formatting options |
This table allows users to quickly assess which library meets their specific needs based on the file types they work with and the functionalities required.
Understanding the xlrd.biffh.xlrderror with Excel Files
Dr. Emily Carter (Data Science Consultant, Tech Innovations Inc.). “The xlrd.biffh.xlrderror is a common issue encountered when attempting to read .xlsx files using the xlrd library, which has dropped support for this format. Users should consider switching to libraries like openpyxl or pandas for seamless handling of .xlsx files.”
Michael Thompson (Software Engineer, Data Solutions Corp.). “This error arises primarily due to the limitations of the xlrd library, which now only supports .xls files. Developers should be aware of this change in functionality and adapt their code accordingly to avoid runtime exceptions.”
Lisa Nguyen (Technical Writer, Python Programming Monthly). “The transition away from xlrd for .xlsx files highlights the importance of staying updated with library documentation. Users encountering the xlrd.biffh.xlrderror should explore alternative libraries that are actively maintained and offer broader file format support.”
Frequently Asked Questions (FAQs)
What does the error ‘xlrd.biffh.XLRDError: Excel xlsx file not supported’ mean?
This error indicates that the xlrd library, which is used for reading Excel files, does not support the newer .xlsx file format. The library was originally designed to handle .xls files.
How can I resolve the ‘xlrd.biffh.XLRDError’ when trying to open an xlsx file?
To resolve this error, you can switch to using the openpyxl library, which supports .xlsx files. Alternatively, you can save the Excel file in the older .xls format if you wish to continue using xlrd.
Is there a way to read .xlsx files using xlrd?
No, xlrd does not support .xlsx files as of version 2.0.0. Users are encouraged to use libraries like openpyxl or pandas, which can handle .xlsx files effectively.
What are some alternative libraries to read .xlsx files in Python?
Some popular alternatives include openpyxl, pandas, and xlsxwriter. These libraries provide robust functionality for reading, writing, and manipulating .xlsx files.
What version of xlrd should I use if I need to work with .xls files?
You can use xlrd version 1.2.0 or earlier to work with .xls files. Starting from version 2.0.0, xlrd has removed support for .xlsx files entirely.
Can I convert an .xlsx file to .xls format to use with xlrd?
Yes, you can convert an .xlsx file to .xls format using Excel or various online conversion tools. After conversion, you will be able to read the file using the xlrd library without encountering the error.
The error message “xlrd.biffh.XLRDError: Excel xlsx file not supported” indicates that the xlrd library, which is primarily used for reading Excel files, has limitations regarding the file formats it can handle. As of version 2.0.0, xlrd has dropped support for the .xlsx file format, focusing instead on the older .xls format. This change has significant implications for users who rely on xlrd for reading modern Excel files, as they will encounter compatibility issues when attempting to open .xlsx files.
To address this issue, users can consider alternative libraries such as openpyxl or pandas, which are capable of reading .xlsx files without encountering the limitations imposed by xlrd. Openpyxl is specifically designed for reading and writing .xlsx files, while pandas provides a more comprehensive data manipulation framework that can also handle .xlsx files effectively. Transitioning to these libraries not only resolves the compatibility problem but also enhances the functionality available for data analysis and manipulation.
In summary, the transition in xlrd’s capabilities necessitates a shift in how users handle Excel files. Understanding the limitations of xlrd and exploring alternative libraries is crucial for anyone working with Excel data in Python. By adapting to these changes
Author Profile
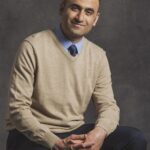
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?