Why Am I Getting a ModuleNotFoundError: No Module Named ‘configparser’?
Are you a Python enthusiast who has recently encountered the frustrating `ModuleNotFoundError: No module named ‘configparser’`? If so, you’re not alone. This error message can be a roadblock for both beginners and seasoned developers alike, often surfacing unexpectedly during the execution of scripts or applications. Understanding the root causes of this error and how to resolve it is crucial for maintaining a smooth coding experience. In this article, we will delve into the intricacies of the `configparser` module, its significance in Python programming, and the common pitfalls that lead to this error.
The `configparser` module is an essential part of Python’s standard library, designed to handle configuration files in a straightforward and user-friendly manner. It allows developers to read and write configuration data in a structured format, making it easier to manage application settings. However, the `ModuleNotFoundError` can arise when the interpreter cannot locate the module, often due to issues related to the Python environment or version mismatches.
In the following sections, we will explore the typical scenarios that trigger this error, including installation problems and environment discrepancies. By equipping yourself with the knowledge to troubleshoot and resolve these issues, you’ll be able to enhance your coding skills and ensure that your projects run seamlessly. Whether
Understanding the ConfigParser Module
The `configparser` module in Python is a powerful tool used for handling configuration files. It allows developers to read, write, and manage configuration settings in a structured way. This module is particularly useful for applications that require customizable settings, enabling users to easily change parameters without modifying the source code.
Key features of the `configparser` module include:
- Sections: Configuration files can be organized into sections, making it easier to group related settings.
- Options: Each section can have multiple options, which can hold different values.
- Data Types: The module supports various data types including strings, integers, and booleans, allowing for flexible configuration.
A typical configuration file might look like this:
“`ini
[general]
app_name = MyApp
version = 1.0
[database]
host = localhost
port = 5432
user = admin
password = secret
“`
Common Causes of ModuleNotFoundError
When encountering the error `ModuleNotFoundError: No module named ‘configparser’`, it is important to investigate the underlying reasons. Here are some common causes:
- Python Version: The `configparser` module is included in Python 3.x but is named `ConfigParser` in Python 2.x. If you are using Python 2.x, you should replace `import configparser` with `import ConfigParser`.
- Environment Issues: The module may not be installed in the current environment. This is particularly relevant if you’re using virtual environments or different Python installations.
- File Name Conflicts: If your script is named `configparser.py`, Python may attempt to import your script instead of the standard library module, leading to confusion and errors.
To check your Python version, you can run:
“`bash
python –version
“`
Resolving the ModuleNotFoundError
To resolve the `ModuleNotFoundError`, follow these steps:
- Check Python Version: Ensure you are using Python 3.x where `configparser` is available.
- Install Missing Module: If you’re in a virtual environment, ensure it’s activated and install the module if it’s missing. Use the following command:
“`bash
pip install configparser
“`
- Rename Conflicting Scripts: If your script name conflicts with the module name, rename your script to avoid the conflict.
Installation and Verification
To verify that the `configparser` module is correctly installed and operational, you can run a simple test script:
“`python
import configparser
config = configparser.ConfigParser()
config.read(‘example.ini’)
print(config[‘general’][‘app_name’])
“`
If this script runs without errors and prints the application name, the module is functioning correctly.
Issue | Resolution |
---|---|
Incorrect Python version | Use Python 3.x and import configparser |
Module not installed | Run pip install configparser |
File name conflict | Rename your script |
By following these steps and understanding the module’s structure, you can effectively troubleshoot and utilize the `configparser` module in your Python applications.
Understanding the Error
The error message `ModuleNotFoundError: No module named ‘configparser’` indicates that the Python interpreter cannot locate the `configparser` module. This typically occurs when the module is either not installed or not accessible in the current Python environment.
- Python 2 vs Python 3:
- In Python 2, `configparser` is known as `ConfigParser` (with an uppercase ‘C’ and ‘P’).
- In Python 3, it is correctly referred to as `configparser`.
Common Causes
Several factors can lead to this error:
- Incorrect Python Version: If your code is written for Python 3 but you are using Python 2, it will not recognize `configparser`.
- Virtual Environment Issues: If you are working within a virtual environment, the module may not be installed in that specific environment.
- Missing Installation: The `configparser` module might not be installed at all, particularly in environments that do not include standard libraries.
Resolving the Error
To fix the `ModuleNotFoundError`, follow these steps:
- Check Python Version:
- Run `python –version` or `python3 –version` in your terminal to ensure you’re using the correct version.
- Use the appropriate command for your codebase.
- Install the Module:
- For Python 3, ensure you use `pip3`:
“`bash
pip3 install configparser
“`
- For Python 2, use:
“`bash
pip install ConfigParser
“`
- Check Your Virtual Environment:
- If using a virtual environment, activate it:
“`bash
source /path/to/venv/bin/activate
“`
- Then install `configparser` if it’s not already present.
- Verify Installation:
- After installation, check if the module is available:
“`python
import configparser
“`
Best Practices
To avoid encountering this error in the future, consider the following practices:
- Use Virtual Environments: Always work in a virtual environment to manage dependencies effectively.
- Consistent Python Version: Ensure that your scripts are executed with the same Python version for which they were developed.
- Documentation and Comments: Comment your code to indicate dependencies, especially if you are sharing it with others.
Table of Common Modules by Python Version
Module Name | Python 2 Name | Python 3 Name | Installation Command |
---|---|---|---|
ConfigParser | ConfigParser | configparser | `pip install ConfigParser` |
requests | requests | requests | `pip install requests` |
numpy | numpy | numpy | `pip install numpy` |
By adhering to these guidelines, you can effectively manage module dependencies and reduce the likelihood of encountering similar errors in your Python projects.
Understanding the `ModuleNotFoundError` for ConfigParser in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “The error ‘ModuleNotFoundError: No module named configparser’ typically arises when a developer attempts to run a Python 2 script in a Python 3 environment. ConfigParser was renamed to configparser in Python 3, so ensuring the correct version of Python is being used is crucial for resolving this issue.”
James Liu (Software Engineer, Open Source Contributor). “When encountering this error, it is essential to verify that the script is not only compatible with Python 3 but also that the environment is set up correctly. Sometimes, virtual environments may not have the required packages installed, leading to such import errors. A simple ‘pip install configparser’ can often resolve the issue.”
Linda Gomez (Technical Educator, Python Programming Institute). “Understanding the differences between Python 2 and Python 3 is fundamental for any developer. The ‘ModuleNotFoundError’ for configparser serves as a reminder to check for compatibility issues and to update legacy code. Developers should also consider using tools like ‘2to3’ to assist in migrating their codebase.”
Frequently Asked Questions (FAQs)
What causes the “ModuleNotFoundError: No module named ‘configparser'” error?
The error occurs when Python cannot locate the `configparser` module. This typically happens if the module is not installed or if the Python environment is misconfigured.
How can I resolve the “ModuleNotFoundError: No module named ‘configparser'” error?
To resolve this error, ensure that you are using the correct Python version. For Python 3, `configparser` is included by default. If you are using Python 2, you may need to install it using `pip install configparser`.
Is `configparser` available in both Python 2 and Python 3?
`configparser` is a built-in module in Python 3. In Python 2, it is named `ConfigParser` (with a capital ‘C’). Ensure you use the correct naming convention based on your Python version.
What should I do if I have multiple Python versions installed?
If multiple Python versions are installed, specify the version when installing packages. Use `pip3 install configparser` for Python 3 or `pip install ConfigParser` for Python 2 to avoid confusion.
Can I use an alternative to `configparser`?
Yes, alternatives such as `json` or `yaml` can be used for configuration management, depending on your project’s requirements. These formats may offer more flexibility or readability.
How can I check if `configparser` is installed in my environment?
You can check if `configparser` is installed by running `pip show configparser` in your terminal. If it is not listed, you will need to install it according to your Python version.
The error message “ModuleNotFoundError: No module named ‘configparser'” typically indicates that the Python interpreter is unable to locate the ‘configparser’ module. This situation often arises when users are working with Python 2.x, where ‘ConfigParser’ (note the capitalization) is the correct module name. In contrast, Python 3.x uses ‘configparser’ as the module name, reflecting the changes made in the transition between the two major versions of Python.
To resolve this issue, users should first verify the version of Python they are using. If they are running Python 2.x, they should switch to Python 3.x to utilize the updated module name. Additionally, ensuring that the correct environment is activated, especially when using virtual environments, is crucial to avoid such errors. Users can check their current environment and installed packages to confirm that ‘configparser’ is available in their Python 3.x setup.
Furthermore, this error serves as a reminder of the importance of understanding the differences between Python versions, particularly when dealing with libraries and modules. Developers should be cautious about version compatibility when writing or maintaining code. Utilizing tools like virtual environments can help manage dependencies and avoid conflicts, thereby enhancing the overall development experience.
Author Profile
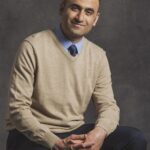
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?