How Can You Resolve an Arithmetic Overflow Error When Converting Numeric Data Types?
In the world of programming and database management, precision is paramount. However, even the most meticulous developers can encounter unexpected challenges, one of which is the notorious “arithmetic overflow error converting numeric to data type numeric.” This seemingly cryptic error message can halt operations and lead to significant frustration, particularly when dealing with large datasets or complex calculations. Understanding the nuances of this error not only empowers developers to troubleshoot effectively but also enhances their ability to design robust applications that handle numerical data with finesse.
When a system attempts to perform a calculation that exceeds the limits of the designated numeric data type, an arithmetic overflow error occurs. This situation often arises when dealing with large integers or high-precision decimal values, where the result surpasses the maximum allowable size for the data type in use. Such errors can manifest in various programming environments, particularly in SQL databases, where numeric precision is strictly enforced. As a result, developers must be vigilant about the types of data they are working with and the potential for overflow during arithmetic operations.
Moreover, the implications of an arithmetic overflow error extend beyond mere inconvenience. They can lead to data corruption, inaccurate results, and even system crashes if not addressed promptly. By exploring the causes and prevention strategies for this error, developers can safeguard their applications against unexpected failures
Understanding Arithmetic Overflow Errors
Arithmetic overflow errors occur when a calculation exceeds the maximum limit that can be stored in a numeric data type. This issue is common in various programming environments and database systems, particularly when dealing with large numbers or performing operations that yield results beyond the defined range of the data type.
When converting numeric values to a different type, such as numeric to decimal, the system checks whether the value can be accurately represented within the target type’s constraints. If it cannot, an overflow error is triggered.
Common Causes of Overflow Errors
Several factors can lead to arithmetic overflow errors during numeric conversions, including:
- Inadequate Data Types: Using data types with insufficient precision or scale for the values being processed.
- Mathematical Operations: Performing operations such as addition or multiplication that yield results larger than the maximum value of the chosen data type.
- Data Input Errors: Inputs that unexpectedly exceed anticipated limits can also cause overflow when processed.
Examples of Overflow Scenarios
To illustrate, consider the following scenarios:
- Integer Overflow: Trying to store the result of a calculation that exceeds the maximum value of a 32-bit integer (2,147,483,647) can result in an overflow error.
- Decimal Conversion: Attempting to convert a value like 12345678901234567890 to a decimal type that cannot accommodate its precision results in an error.
Data Type | Maximum Value | Example of Overflow |
---|---|---|
Integer | 2,147,483,647 | 2,147,483,648 + 1 |
Decimal(10,2) | 99,999,999.99 | 100,000,000.00 |
Float | 3.40282347E+38 | 3.40282348E+38 |
Handling Overflow Errors
To mitigate the risk of arithmetic overflow errors, consider the following strategies:
- Use Appropriate Data Types: Select data types that match the expected range and precision of your calculations.
- Implement Error Handling: Use try-catch blocks or equivalent error handling mechanisms to gracefully manage overflow errors when they occur.
- Validate Input Data: Ensure that input values are validated before performing operations to avoid unexpected overflows.
By understanding the nature of arithmetic overflow errors and implementing preventive measures, developers can effectively manage numeric conversions and maintain data integrity.
Understanding Arithmetic Overflow Errors
Arithmetic overflow errors occur when a calculation produces a result that exceeds the maximum limit of a data type. In databases, particularly when converting numeric values, this error often arises due to incompatible data type definitions or unexpected large values.
Common Causes of Arithmetic Overflow Errors
Several factors can lead to an arithmetic overflow error when converting numeric types:
- Data Type Limits: Each numeric data type has defined limits. For example:
- `TINYINT`: 0 to 255
- `SMALLINT`: -32,768 to 32,767
- `INT`: -2,147,483,648 to 2,147,483,647
- `BIGINT`: -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807
- Precision and Scale: When converting between numeric types, the precision (total number of digits) and scale (number of digits to the right of the decimal) must be compatible. For instance, trying to store a value with more decimal places than allowed by the target data type will cause an overflow.
- Implicit Conversions: Automatic type conversions might lead to an overflow if the source numeric value exceeds the range of the target type.
Examples of Arithmetic Overflow Errors
Consider the following scenarios that can trigger an overflow error:
- Example 1: Converting a large integer to `SMALLINT`:
“`sql
SELECT CAST(40000 AS SMALLINT); — Results in overflow error
“`
- Example 2: Inserting a value with excessive precision:
“`sql
CREATE TABLE Sales (Amount NUMERIC(5, 2));
INSERT INTO Sales VALUES (123.456); — Results in overflow error
“`
Best Practices to Avoid Overflow Errors
To minimize the risk of encountering arithmetic overflow errors, consider the following best practices:
- Select Appropriate Data Types: Always choose data types that can accommodate the expected range of values. Use `BIGINT` for large integers and `DECIMAL` for precise decimal values.
- Perform Validations: Before inserting or updating data, validate values to ensure they fall within acceptable ranges.
- Explicitly Define Precision and Scale: When using `NUMERIC` or `DECIMAL`, explicitly define precision and scale, and ensure that these match the expected data.
- Use TRY_CAST or TRY_CONVERT: In SQL Server, utilize `TRY_CAST` or `TRY_CONVERT` to safely attempt conversions, returning `NULL` instead of an error when an overflow occurs.
Handling Arithmetic Overflow Errors
When an arithmetic overflow error occurs, you can handle it in the following ways:
- Error Handling in SQL:
- Utilize `BEGIN TRY…END TRY` and `BEGIN CATCH…END CATCH` blocks to manage exceptions.
- Log Errors: Implement logging mechanisms to capture details of overflow incidents for debugging and analysis.
- Fallback Strategies: Design fallback strategies to manage operations that may encounter overflow, such as using alternative calculations or notifying users of input errors.
Understanding arithmetic overflow errors and their causes is crucial for database management. By following best practices and implementing proper error handling techniques, you can effectively mitigate and manage these errors in your applications.
Understanding Arithmetic Overflow Errors in Numeric Data Types
Dr. Emily Carter (Data Integrity Specialist, Tech Solutions Inc.). “Arithmetic overflow errors typically occur when a calculation exceeds the maximum limit of the numeric data type being used. It is crucial for developers to implement proper data validation and type checking to prevent such issues, especially in financial applications where precision is paramount.”
Michael Chen (Senior Database Administrator, CloudData Corp.). “To mitigate arithmetic overflow errors, one should consider using larger numeric types or implementing error handling mechanisms that can gracefully manage unexpected values. Regular audits of data types in database schemas can also help identify potential overflow risks before they become problematic.”
Sarah Patel (Software Engineer, FinTech Innovations). “When dealing with numeric conversions, it is essential to anticipate the possibility of overflow. Utilizing libraries that support arbitrary precision arithmetic can be a game changer, especially in applications that handle large datasets or complex calculations, ensuring that overflow errors are minimized.”
Frequently Asked Questions (FAQs)
What is an arithmetic overflow error?
An arithmetic overflow error occurs when a calculation produces a result that exceeds the maximum limit of the data type used to store it. This typically happens during operations involving numeric data types.
What causes an arithmetic overflow error when converting numeric to data type numeric?
This error is caused when the value being converted exceeds the precision or scale defined for the target numeric data type. For example, trying to store a number with too many digits or decimal places can trigger this error.
How can I identify if an arithmetic overflow error has occurred?
You can identify this error through error messages generated by your database or application. Additionally, reviewing logs or debugging output can help pinpoint the operation that caused the overflow.
What are some common scenarios that lead to this error?
Common scenarios include summing large datasets, multiplying large numbers, or attempting to convert a string representation of a number that exceeds the numeric limits of the target data type.
How can I prevent arithmetic overflow errors in my applications?
To prevent these errors, ensure that your numeric data types have sufficient precision and scale. Implement input validation to check for potential overflows before performing calculations, and consider using larger data types if necessary.
What steps can I take to resolve an arithmetic overflow error?
To resolve this error, review the data types used in your calculations and conversions. Adjust the precision or scale of the target data type, or modify the input values to ensure they are within acceptable limits.
Arithmetic overflow errors occur when a calculation produces a result that exceeds the maximum limit of the data type being used. In the context of converting numeric values to the data type numeric, this error typically arises when the precision and scale defined for the numeric type cannot accommodate the value being processed. For example, if a numeric type is defined with a precision of 5 and a scale of 2, it can only represent values up to 999.99. Any attempt to store a larger value will result in an overflow error.
Understanding the root causes of arithmetic overflow errors is crucial for database management and programming. It is essential to ensure that the data types used in calculations are appropriately defined to handle the expected range of values. This may involve increasing the precision and scale of numeric types or implementing error handling mechanisms to catch potential overflow situations before they occur. By doing so, developers can enhance the robustness of their applications and ensure data integrity.
In summary, arithmetic overflow errors when converting numeric types highlight the importance of careful data type selection and validation in programming. Developers should be proactive in assessing the limits of numeric data types and adjusting them as necessary to prevent such errors. This approach not only improves application performance but also enhances user experience by minimizing unexpected failures
Author Profile
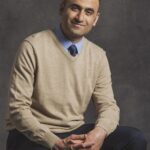
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?