How Can You Convert a String to Bytes in Python?
In the world of programming, data manipulation is a fundamental skill that every developer must master. One common task that often arises is the conversion of strings to bytes, a process that is crucial for various applications, from network communication to file handling. Python, with its rich set of built-in functions and libraries, makes this task both straightforward and efficient. Whether you’re working on a simple script or a complex application, understanding how to convert a string to bytes can enhance your coding toolkit and improve your ability to handle data effectively.
At its core, converting a string to bytes in Python involves encoding the string into a specific byte representation. This process is essential because computers operate using binary data, and strings—while human-readable—must be transformed into a format that machines can understand. Python provides several encoding options, allowing developers to choose the most appropriate method based on the requirements of their project. From UTF-8 to ASCII, the choice of encoding can impact how data is processed and transmitted.
Moreover, the conversion process is not just about changing formats; it also opens the door to a host of functionalities, such as data compression, encryption, and interoperability between different systems. By mastering this conversion, developers can ensure their applications communicate seamlessly across various platforms and handle data with precision. In the following sections
Methods for Converting Strings to Bytes
To convert a string to bytes in Python, you can use several methods that leverage built-in functionality. The most common way to achieve this is through the `encode()` method of string objects. This method allows you to specify the encoding format, with UTF-8 being the default and most widely used.
Using the encode() Method
The `encode()` method transforms a string into a bytes object. Here’s how you can use it:
“`python
string_data = “Hello, World!”
byte_data = string_data.encode() Default is ‘utf-8’
“`
You can also specify a different encoding, like `ascii`, `utf-16`, or `latin1`:
“`python
byte_data_ascii = string_data.encode(‘ascii’)
byte_data_utf16 = string_data.encode(‘utf-16’)
“`
Encoding Formats
When converting strings to bytes, it is essential to choose an appropriate encoding format based on the characters in your string. Common encoding formats include:
- UTF-8: Supports all Unicode characters and is the most common encoding on the web.
- ASCII: Limited to 128 characters; only supports basic English letters and digits.
- UTF-16: Uses two bytes per character, suitable for languages with larger character sets.
- latin1: Supports the first 256 Unicode characters, often used for Western European languages.
Encoding | Description | Byte Size |
---|---|---|
UTF-8 | Variable length, supports all Unicode characters. | 1 to 4 bytes per character |
ASCII | Fixed length, supports only basic English characters. | 1 byte per character |
UTF-16 | Fixed length, supports a large set of characters. | 2 bytes per character |
latin1 | Fixed length, supports Western European languages. | 1 byte per character |
Handling Errors during Encoding
When converting strings to bytes, you might encounter characters that cannot be encoded in the specified format. To manage such scenarios, the `encode()` method provides an optional `errors` parameter. You can choose from various error handling strategies:
- ‘strict’: Raises a `UnicodeEncodeError` (default).
- ‘ignore’: Ignores characters that cannot be encoded.
- ‘replace’: Replaces unencodable characters with a replacement character, usually `?`.
Example using the `errors` parameter:
“`python
string_with_special_characters = “Café”
byte_data_ignore = string_with_special_characters.encode(‘ascii’, errors=’ignore’)
byte_data_replace = string_with_special_characters.encode(‘ascii’, errors=’replace’)
“`
This approach ensures that your application can handle encoding errors gracefully, depending on your requirements.
Converting strings to bytes in Python is a straightforward process that can be tailored to your needs through the choice of encoding and error handling strategies. Understanding these methods and their implications allows for effective data manipulation and storage in Python applications.
Methods to Convert a String to Bytes in Python
In Python, converting a string to bytes can be accomplished using various methods. The most common way is by utilizing the built-in `encode()` method of string objects. Below are the primary methods for performing this conversion.
Using the `encode()` Method
The `encode()` method encodes a string into bytes using a specified encoding scheme. The most frequently used encodings are ‘utf-8’, ‘ascii’, and ‘latin-1’.
“`python
Example of encoding a string to bytes
string_value = “Hello, World!”
bytes_value = string_value.encode(‘utf-8′)
print(bytes_value) Output: b’Hello, World!’
“`
Supported Encodings
Here are some common encoding formats:
- UTF-8: A variable-length encoding that can represent every character in the Unicode character set.
- ASCII: A 7-bit encoding that represents English characters and control characters.
- Latin-1: A single-byte encoding that can represent the first 256 Unicode characters.
Using the `bytes()` Constructor
The `bytes()` constructor can also be employed to convert a string into bytes. This method accepts the string and an encoding type as arguments.
“`python
Example using the bytes() constructor
string_value = “Hello, World!”
bytes_value = bytes(string_value, ‘utf-8′)
print(bytes_value) Output: b’Hello, World!’
“`
Encoding Options
When converting strings to bytes, it is crucial to select the appropriate encoding based on the content of the string. Below is a comparison of different encoding types:
Encoding | Description | Use Case |
---|---|---|
UTF-8 | Supports all Unicode characters | General-purpose text encoding |
ASCII | Limited to 128 characters (0-127) | Basic English text without special characters |
Latin-1 | Supports the first 256 Unicode characters | Western European languages |
Handling Errors During Encoding
When encoding strings, it is possible to encounter characters that cannot be encoded in the specified format. Python’s `encode()` method allows you to handle these errors by using the `errors` parameter.
“`python
Example of error handling during encoding
string_value = “Hello, World! 😊”
bytes_value = string_value.encode(‘ascii’, errors=’ignore’) Ignores unencodable characters
print(bytes_value) Output: b’Hello, World! ‘
“`
Error Handling Options
- ‘ignore’: Skip the characters that cannot be encoded.
- ‘replace’: Replace unencodable characters with a placeholder (usually ‘?’).
- ‘strict’: Raise a `UnicodeEncodeError` (default behavior).
These methods provide a versatile approach to converting strings to bytes in Python. Each method has its specific use case, and understanding the implications of different encodings and error handling will aid in effective string manipulation.
Expert Insights on Converting Strings to Bytes in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Institute). “Converting a string to bytes in Python is essential for data handling, especially when dealing with network communications. The built-in `encode()` method allows developers to specify the encoding format, such as UTF-8, ensuring compatibility across different systems.”
Michael Chen (Lead Data Scientist, Tech Innovations Corp). “In Python, the conversion from string to bytes is straightforward and can be accomplished using the `bytes()` function or the `encode()` method. It’s crucial to choose the correct encoding to prevent data loss or corruption, particularly when processing international text.”
Sarah Thompson (Python Instructor, Code Academy). “Understanding how to convert strings to bytes is fundamental for any Python programmer. The `str.encode()` method is commonly used, and it’s important to be aware of the encoding standards to ensure that the byte representation accurately reflects the original string.”
Frequently Asked Questions (FAQs)
How can I convert a string to bytes in Python?
You can convert a string to bytes in Python using the `encode()` method. For example, `my_bytes = my_string.encode(‘utf-8’)` converts `my_string` to bytes using UTF-8 encoding.
What encoding should I use when converting a string to bytes?
UTF-8 is the most commonly used encoding for converting strings to bytes, as it supports all Unicode characters. However, you can also use other encodings like ‘ascii’, ‘latin-1’, or ‘utf-16’ based on your requirements.
What happens if I try to encode a string with unsupported characters?
If you attempt to encode a string with unsupported characters using a specific encoding, Python will raise a `UnicodeEncodeError`. To handle such cases, you can specify an error handling scheme, such as `my_string.encode(‘ascii’, ‘ignore’)`, which ignores unsupported characters.
Can I convert bytes back to a string in Python?
Yes, you can convert bytes back to a string using the `decode()` method. For instance, `my_string = my_bytes.decode(‘utf-8’)` converts `my_bytes` back to a string using UTF-8 decoding.
Is it possible to convert a string to bytes without specifying an encoding?
No, it is necessary to specify an encoding when converting a string to bytes in Python. The default encoding is UTF-8 if you do not specify one, but it is always good practice to define the encoding explicitly.
What is the difference between bytes and bytearray in Python?
`bytes` is an immutable sequence of bytes, while `bytearray` is a mutable sequence. You can modify a `bytearray` after its creation, but once a `bytes` object is created, it cannot be changed.
Converting a string to bytes in Python is a fundamental operation that is essential for various applications, particularly in data processing and network communication. Python provides a straightforward method for this conversion through the built-in `encode()` method. By specifying the encoding format, such as UTF-8, developers can ensure that the string is accurately represented in byte form. This functionality is crucial when dealing with text data that needs to be transmitted or stored in a binary format.
It is important to understand the significance of character encoding when converting strings to bytes. Different encodings can represent characters in different ways, which may lead to data corruption or loss if not handled correctly. UTF-8 is widely recommended due to its compatibility with a vast range of characters and its efficiency in representing ASCII characters. Additionally, Python 3’s handling of strings as Unicode by default further emphasizes the need for proper encoding practices when working with byte data.
In summary, converting strings to bytes in Python is a simple yet critical task that can be accomplished using the `encode()` method. By being mindful of the encoding used, developers can avoid common pitfalls associated with character representation. This knowledge is vital for anyone working with text data in Python, ensuring that data integrity is maintained throughout various operations
Author Profile
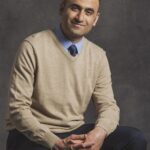
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?