What Does .format Do in Python? Understanding Its Purpose and Usage
In the world of programming, clarity and precision are paramount, especially when it comes to presenting data. Python, a language celebrated for its readability and simplicity, offers a powerful tool for formatting strings: the `.format()` method. Whether you’re crafting user-friendly output or building complex reports, understanding how to leverage this feature can elevate your coding skills and enhance the way your programs communicate information. Join us as we delve into the intricacies of `.format()`, unraveling its capabilities and exploring how it can streamline your coding experience.
The `.format()` method in Python is a versatile way to create formatted strings. It allows developers to insert variables into strings seamlessly, making it easier to construct messages that are both dynamic and informative. This method supports a variety of formatting options, enabling you to control everything from decimal precision to alignment. As you become familiar with its syntax and functionality, you’ll discover how `.format()` can be a game-changer in your coding toolkit.
Beyond basic variable substitution, `.format()` opens the door to more advanced formatting techniques. It can handle multiple placeholders within a single string, allowing for more complex outputs without sacrificing readability. This flexibility makes it an essential feature for anyone looking to produce polished and professional results in their Python projects. As we explore this topic further
Understanding the .format Method
The `.format()` method in Python is a powerful and flexible way to format strings. It allows you to interpolate variables and control the presentation of the data within a string. This method enhances the readability and maintainability of your code by separating the string structure from the data that populates it.
The syntax for using the `.format()` method is straightforward:
“`python
“string {}”.format(value)
“`
You can include placeholders within the string, indicated by curly braces `{}`, where the corresponding values will be inserted. This method supports various formatting options, allowing for different types of data representations.
Basic Usage
Here are some basic examples demonstrating the use of the `.format()` method:
“`python
name = “Alice”
age = 30
formatted_string = “My name is {} and I am {} years old.”.format(name, age)
print(formatted_string)
“`
Output:
“`
My name is Alice and I am 30 years old.
“`
In this example, the values of `name` and `age` are inserted into the string at the respective placeholders.
Positional and Keyword Arguments
The `.format()` method supports both positional and keyword arguments, providing flexibility in how you format strings.
- Positional Arguments: You can specify the order in which the variables are substituted.
“`python
formatted_string = “The {0} is {1} years old.”.format(name, age)
“`
- Keyword Arguments: You can also pass named arguments for clarity.
“`python
formatted_string = “My name is {name} and I am {age} years old.”.format(name=name, age=age)
“`
Formatting Types
The `.format()` method also allows for various formatting options, such as controlling decimal places for floats, padding numbers, and aligning text. Here’s a brief overview of some common formatting types:
- Floating Point Formatting: Control the number of decimal places.
- Padding and Alignment: Adjust the width and alignment of strings.
- Number Formatting: Format numbers with commas or currency symbols.
Here is a table summarizing some of the formatting options:
Format Specifier | Description | Example |
---|---|---|
:.2f | Float with 2 decimal places | “Value: {:.2f}”.format(3.14159) -> Value: 3.14 |
:>10 | Right-align within 10 spaces | “{:>10}”.format(“text”) -> ” text” |
:,.0f | Integer with comma as thousands separator | “Total: {:,.0f}”.format(1234567) -> Total: 1,234,567 |
Advanced Formatting
For more complex scenarios, the `.format()` method can handle nested fields and format specifications:
“`python
data = {“name”: “Bob”, “age”: 25}
formatted_string = “Name: {0[name]}, Age: {0[age]}”.format(data)
“`
In this example, the dictionary `data` is accessed directly within the format string. This capability allows for more dynamic and structured formatting scenarios.
Overall, the `.format()` method is an essential tool in Python for creating well-structured and easily understandable output. Its versatility in formatting options makes it suitable for various applications, from simple string manipulations to complex data presentations.
Understanding the .format Method in Python
The `.format()` method in Python is a powerful tool for string formatting, enabling developers to construct strings dynamically and incorporate variable data seamlessly. This method is an enhancement over the older `%` formatting and provides more flexibility and clarity.
Basic Usage of .format()
To use the `.format()` method, you include placeholders in a string, denoted by curly braces `{}`, which will be replaced by the values passed to the method.
“`python
name = “Alice”
age = 30
greeting = “Hello, my name is {} and I am {} years old.”.format(name, age)
print(greeting)
“`
Output:
“`
Hello, my name is Alice and I am 30 years old.
“`
Positional and Keyword Arguments
The `.format()` method can handle both positional and keyword arguments, offering flexibility in how values are inserted into strings.
Positional Arguments Example:
“`python
template = “The {} is {}.”
formatted = template.format(“sky”, “blue”)
print(formatted)
“`
Keyword Arguments Example:
“`python
template = “{name} has {count} apples.”
formatted = template.format(name=”John”, count=5)
print(formatted)
“`
Output:
“`
John has 5 apples.
“`
Advanced Formatting Options
The `.format()` method supports advanced formatting options, including number formatting, alignment, and padding.
Examples:
- Number Formatting:
“`python
number = 123.456789
formatted_number = “{:.2f}”.format(number) Two decimal places
print(formatted_number)
“`
- Alignment and Padding:
“`python
aligned = “|{:<10}|{:^10}|{:>10}|”.format(“left”, “center”, “right”)
print(aligned)
“`
Output:
“`
left | center | right |
---|
“`
Using Format Specifiers
Format specifiers can be used within the placeholders for more control over the output. The general syntax is `{index:format_spec}`, where `index` is the positional argument index and `format_spec` defines how to format the value.
Format Specifier | Description | Example |
---|---|---|
`:d` | Integer format | `{0:d}` |
`:.2f` | Floating-point with 2 decimal places | `{0:.2f}` |
`:>10` | Right-align in a width of 10 | `{:>10}` |
Combining Text and Variables
You can easily combine static text with variable data using the `.format()` method, enhancing code readability.
“`python
item = “book”
price = 12.99
message = “The price of the {} is ${:.2f}.”.format(item, price)
print(message)
“`
Output:
“`
The price of the book is $12.99.
“`
The `.format()` method in Python provides a rich and flexible way to format strings, accommodating various use cases from simple variable insertion to complex formatting needs. With its ability to handle both positional and keyword arguments, as well as support for format specifiers, it is an indispensable tool in a Python developer’s toolkit.
Understanding the Role of .format in Python Programming
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “The .format method in Python is a powerful tool that allows developers to create formatted strings. It enhances readability and maintainability of code by enabling the insertion of variables into strings in a clear and concise manner.”
James Liu (Lead Software Engineer, CodeCraft Solutions). “Using .format provides flexibility in string manipulation. It supports various formatting options, such as specifying number precision and alignment, which are essential for presenting data in a user-friendly way.”
Maria Gonzalez (Python Educator, LearnPython.org). “The .format method is not just about aesthetics; it plays a crucial role in internationalization and localization of applications. By using .format, developers can easily adapt their strings to different languages and formats without altering the core logic of the code.”
Frequently Asked Questions (FAQs)
What does .format do in Python?
The `.format()` method in Python is used for string formatting. It allows you to embed variables and expressions within strings, making it easier to create dynamic output.
How do you use the .format method?
To use the `.format()` method, you call it on a string containing placeholders defined by curly braces `{}`. You can pass values to be inserted into these placeholders as arguments to the method.
Can you provide an example of .format in Python?
Certainly. For example:
“`python
name = “Alice”
age = 30
formatted_string = “My name is {} and I am {} years old.”.format(name, age)
“`
This will output: “My name is Alice and I am 30 years old.”
What are the advantages of using .format over the % operator?
The `.format()` method provides more flexibility and readability compared to the `%` operator. It supports advanced formatting options, such as specifying the order of arguments and formatting types directly within the placeholders.
Is .format the only way to format strings in Python?
No, there are other methods for string formatting in Python, including f-strings (introduced in Python 3.6) and the older `%` operator. F-strings are often preferred for their simplicity and performance.
Can .format handle multiple data types?
Yes, the `.format()` method can handle various data types, including strings, integers, floats, and more. You can format and display these types together in a single string.
The `.format()` method in Python is a powerful and versatile tool for string formatting. It allows developers to create formatted strings by embedding values within placeholders. These placeholders are defined using curly braces `{}` within the string, which can be replaced with the specified values when the `.format()` method is called. This functionality enhances the readability and maintainability of code, particularly when constructing strings that require dynamic content.
One of the key advantages of using `.format()` is its ability to handle various data types seamlessly. It supports positional and keyword arguments, enabling developers to format strings in a flexible manner. Additionally, the method provides options for formatting numbers, dates, and other data types, making it suitable for a wide range of applications. This capability is particularly useful in scenarios where precise formatting is required, such as in financial applications or when generating reports.
Another significant takeaway is the `.format()` method’s compatibility with f-strings, introduced in Python 3.6. While f-strings offer a more concise syntax for string interpolation, `.format()` remains relevant due to its extensive features and backward compatibility with earlier versions of Python. Understanding both methods allows developers to choose the most appropriate string formatting technique for their specific use cases.
Author Profile
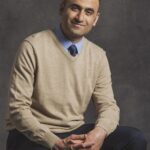
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?