What Does ‘++’ Mean in JavaScript? Unpacking the Increment Operator
In the ever-evolving world of JavaScript, understanding the nuances of its syntax is crucial for both budding developers and seasoned programmers alike. One such syntax feature that often sparks curiosity and confusion is the `++` operator. This seemingly simple symbol holds the key to powerful operations that can streamline your code and enhance its functionality. Whether you’re incrementing a variable or leveraging its unique behavior in different contexts, grasping the ins and outs of the `++` operator can significantly elevate your programming skills.
At its core, the `++` operator is a unary operator that increments a number by one. It can be used in two distinct forms: the prefix increment (`++x`) and the postfix increment (`x++`). Each form behaves slightly differently, impacting the order of operations and the value returned in expressions. This duality allows developers to choose the most appropriate form based on the specific needs of their code, making it a versatile tool in the JavaScript arsenal.
As we delve deeper into the mechanics of the `++` operator, we will explore its practical applications, potential pitfalls, and best practices for implementation. Understanding how to effectively utilize this operator can lead to cleaner, more efficient code, empowering you to tackle complex programming challenges with confidence. So, let’s unravel the mysteries of the `
Understanding the Increment Operator (++)
The `++` operator in JavaScript is known as the increment operator. It is used to increase the value of a variable by one. This operator can be utilized in two different ways: as a prefix or as a postfix.
Prefix vs. Postfix Increment
The primary distinction between the prefix and postfix forms of the increment operator lies in the timing of the increment relative to the value returned.
- Prefix Increment (`++variable`): Increments the variable’s value by one and then returns the new value.
- Postfix Increment (`variable++`): Returns the current value of the variable and then increments its value by one.
To illustrate the difference, consider the following examples:
javascript
let a = 5;
let b = ++a; // b is now 6, a is now 6
javascript
let x = 5;
let y = x++; // y is now 5, x is now 6
Behavior in Expressions
The behavior of the increment operator can lead to different results depending on its placement within expressions. Here’s a brief overview of how it behaves in various scenarios:
Code | Result | Value of Variable |
---|---|---|
let a = 3; let b = ++a; | b = 4 | a = 4 |
let x = 3; let y = x++; | y = 3 | x = 4 |
let z = 1; z += ++z; | z = 3 | z = 3 |
Use Cases
The increment operator is commonly used in various scenarios, including:
- Looping: Often seen in `for` loops to iterate through arrays or collections.
- Counting: Useful in counters where an increase by one is required.
- Conditional Statements: Can be used to adjust values conditionally within code blocks.
Potential Pitfalls
While the increment operator is straightforward, it can lead to confusion if not used carefully. Some potential issues include:
- Unintended Side Effects: The order of operations in complex expressions may yield unexpected results.
- Readability: Overuse or misuse in long expressions can reduce code clarity.
To mitigate these issues, it is recommended to use the increment operator in simple, clear contexts, ensuring that the intent of the code is easily understandable.
Understanding the Increment Operator
The `++` operator in JavaScript is known as the increment operator. It is used to increase the value of a variable by one. This operator can be utilized in two forms: prefix and postfix.
Types of Increment Operator
- Prefix Increment (`++variable`): Increments the value of the variable before it is used in an expression.
- Postfix Increment (`variable++`): Increments the value of the variable after it has been used in an expression.
Behavior of Prefix vs. Postfix
The choice between prefix and postfix can affect the behavior of your code, particularly in expressions. Below is a comparison table demonstrating the differences:
Type | Code Example | Result |
---|---|---|
Prefix |
let x = 5; let y = ++x;
|
y is 6; x is also 6.
|
Postfix |
let x = 5; let y = x++;
|
y is 5; x is 6.
|
Practical Examples
Consider the following examples to illustrate the use of the increment operator:
javascript
let count = 0;
// Using Prefix Increment
console.log(++count); // Output: 1
console.log(count); // Output: 1
// Using Postfix Increment
console.log(count++); // Output: 1
console.log(count); // Output: 2
In the above code snippet, the prefix increment increases the value of `count` before it is logged, while the postfix increment increases it after logging.
Common Use Cases
The increment operator is frequently used in various programming scenarios, including:
- Loops: Commonly used in `for` loops to iterate through arrays or collections.
- Counting: Useful for maintaining counters, such as counting the number of iterations or items processed.
- Conditional Logic: Often applied in conditions where a value needs to be incremented based on certain criteria.
Potential Pitfalls
While the increment operator is straightforward, it can lead to confusion if not used carefully:
- Side Effects: Using the increment operator in complex expressions can lead to unexpected results.
- Readability: Overusing increment operations in a single line can decrease code readability.
By understanding the increment operator’s functionality and effects, developers can utilize it effectively in their JavaScript code.
Understanding the Increment Operator in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). The ‘++’ operator in JavaScript is a crucial feature that allows developers to increment a variable’s value by one. This operator can be utilized in two forms: prefix and postfix. Understanding the distinction between these two forms is essential for effective coding, as it can impact the order of operations within expressions.
Michael Chen (JavaScript Educator, Code Academy). The ‘++’ operator is often underestimated by beginners. It not only simplifies code but also enhances readability. When used correctly, it can lead to cleaner loops and conditionals, making the codebase more maintainable. However, one must be cautious about its side effects, especially in complex expressions.
Sarah Patel (JavaScript Developer Advocate, Open Source Community). In JavaScript, the ‘++’ operator serves as a shorthand for incrementing numeric values, which is particularly useful in iterative processes. However, developers should be mindful of its behavior in different contexts, such as within function calls or when combined with other operators, to avoid unexpected results.
Frequently Asked Questions (FAQs)
What is the purpose of the ++ operator in JavaScript?
The ++ operator is used to increment a variable’s value by one. It can be utilized in two forms: prefix (++variable) and postfix (variable++), affecting the timing of the increment in expressions.
How does the prefix ++ operator differ from the postfix ++ operator?
The prefix ++ operator increments the variable’s value before the expression is evaluated, while the postfix ++ operator increments the variable’s value after the expression has been evaluated. This can lead to different results in certain contexts.
Can the ++ operator be used with non-integer values?
Yes, the ++ operator can be applied to non-integer values, such as floating-point numbers. However, it will still only increment the value by one, which may not always yield an expected result in terms of precision.
What happens if you use the ++ operator on a constant?
Using the ++ operator on a constant will result in a TypeError, as constants cannot be modified. The operator is intended for variables that can hold changing values.
Is it possible to use the ++ operator in a loop?
Yes, the ++ operator is commonly used in loops to increment the loop counter. It simplifies the syntax for increasing the counter variable with each iteration.
What are some common mistakes to avoid when using the ++ operator?
Common mistakes include confusing the prefix and postfix forms, leading to unexpected results, and using the operator on non-numeric types, which can result in NaN (Not a Number) if the value cannot be incremented.
The ‘++’ operator in JavaScript is known as the increment operator. It is used to increase the value of a variable by one. This operator can be applied in two forms: prefix and postfix. When used as a prefix (e.g., ++variable), it increments the variable’s value before it is used in an expression. Conversely, when used as a postfix (e.g., variable++), it increments the value after the current expression has been evaluated. Understanding the distinction between these two forms is crucial for predicting the behavior of code that relies on the increment operator.
One significant aspect of the increment operator is its impact on performance and readability. The ‘++’ operator is often preferred in loops and iterative processes due to its concise syntax, which can enhance code clarity and reduce the likelihood of errors. Additionally, the increment operator can be used in various contexts, including within function calls and as part of more complex expressions, demonstrating its versatility in JavaScript programming.
It is essential to note that the increment operator modifies the original variable rather than returning a new value. This characteristic can lead to unintended side effects if not carefully managed, especially in larger codebases. Developers should remain vigilant about the scope and state of variables when using the increment
Author Profile
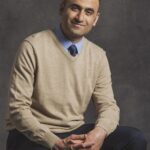
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?