Understanding the ‘next’ Function in Python: What Does It Do?
In the world of Python programming, efficiency and simplicity are paramount. As developers strive to write cleaner and more readable code, they often seek tools and functions that can streamline their workflows. One such function that stands out is `next()`. This seemingly simple built-in function holds the key to navigating through iterables with ease, enhancing both the functionality and performance of your code. Whether you’re a seasoned developer or a curious beginner, understanding how `next()` operates can significantly elevate your programming prowess.
At its core, the `next()` function is designed to retrieve the next item from an iterator. This capability is particularly valuable in scenarios where you need to process elements one at a time, rather than loading an entire collection into memory. By leveraging `next()`, you can efficiently traverse large datasets, making your applications more responsive and resource-friendly. Furthermore, `next()` allows for customization through its optional parameters, enabling you to define default values when an iterator is exhausted, thereby preventing errors and enhancing your code’s robustness.
As we delve deeper into the intricacies of the `next()` function, we’ll explore its syntax, practical applications, and some common use cases that illustrate its versatility. From simplifying loops to managing complex data flows, understanding `next()` will empower you to write more elegant and efficient
Understanding the `next()` Function
The `next()` function in Python is a built-in utility used to retrieve the next item from an iterator. It is particularly useful when working with iterators, which are objects that implement the iterator protocol, consisting of the `__iter__()` and `__next__()` methods.
When you call `next()`, you can specify the iterator from which you want to get the next item. If there are no more items left in the iterator, it raises a `StopIteration` exception. However, you can also provide a default value to return in case the iterator is exhausted, preventing the exception from being thrown.
The basic syntax of the `next()` function is as follows:
“`python
next(iterator[, default])
“`
Where:
- `iterator` is the iterator object you are working with.
- `default` is an optional value returned if the iterator is exhausted.
Using `next()` with Iterators
To better understand how `next()` operates, consider the following example:
“`python
my_list = [1, 2, 3]
my_iterator = iter(my_list)
print(next(my_iterator)) Output: 1
print(next(my_iterator)) Output: 2
print(next(my_iterator)) Output: 3
print(next(my_iterator, ‘No more items’)) Output: No more items
“`
In this example:
- We create a list and then generate an iterator from it using `iter()`.
- We call `next()` multiple times to retrieve each item.
- When the iterator is exhausted, we provide a default string, which is returned instead of raising an exception.
Practical Applications
The `next()` function is especially valuable in various scenarios, such as:
- Reading files: Iterating over lines in a file.
- Data processing: Handling streams of data where you want to process each item one at a time.
- Custom iterators: Implementing custom classes that follow the iterator protocol.
Comparison with Other Iteration Techniques
While `next()` is a straightforward method for getting the next item from an iterator, Python provides several other ways to iterate, such as using `for` loops and comprehensions. The choice of method can impact performance and readability.
Method | Description | Use Case |
---|---|---|
`next()` | Retrieves the next item from an iterator. | When you need precise control over iteration. |
`for` loop | Automatically iterates over each item in an iterable. | General iteration over lists or collections. |
List comprehensions | Creates a new list from an iterable. | When you want to transform data efficiently. |
Conclusion on Usage Considerations
When employing the `next()` function, keep the following points in mind:
- It is efficient for sequential access to items.
- Always consider using the default parameter to handle the end of the iterator gracefully.
- Use it within contexts where you need to manually control the flow of iteration.
By understanding the functionality and applications of the `next()` function, you can effectively manage iterations in your Python programs, enhancing both performance and clarity.
Functionality of `next()` in Python
The `next()` function in Python is a built-in function that is primarily used to retrieve the next item from an iterator. If a second argument is provided, it returns that value when the iterator is exhausted. This function is crucial when working with iterators and generators, which are common in Python for managing sequences of data.
Basic Syntax
The syntax of the `next()` function is as follows:
“`python
next(iterator[, default])
“`
- iterator: An iterator object from which the next item is to be retrieved.
- default (optional): A value to return if the iterator is exhausted. If not specified and the iterator is exhausted, a `StopIteration` exception is raised.
Examples of Usage
To illustrate the functionality of `next()`, consider the following examples:
Example 1: Basic Iterator Usage
“`python
my_list = [1, 2, 3]
my_iterator = iter(my_list)
print(next(my_iterator)) Output: 1
print(next(my_iterator)) Output: 2
print(next(my_iterator)) Output: 3
print(next(my_iterator)) Raises StopIteration
“`
Example 2: Using Default Value
“`python
my_list = [1, 2, 3]
my_iterator = iter(my_list)
print(next(my_iterator, ‘No more items’)) Output: 1
print(next(my_iterator, ‘No more items’)) Output: 2
print(next(my_iterator, ‘No more items’)) Output: 3
print(next(my_iterator, ‘No more items’)) Output: No more items
“`
Common Use Cases
The `next()` function is commonly utilized in several scenarios:
- Iteration Control: When manually iterating through items where the control over the iteration process is necessary.
- Generator Functions: When working with generator functions that yield values one at a time.
- Data Processing: In data pipelines where the sequence of processing elements is controlled explicitly.
Performance Considerations
Using `next()` can be more efficient than using a for-loop in scenarios where only a few items are needed from a potentially large iterable. Here are some factors to consider:
Factor | Description |
---|---|
Memory Efficiency | `next()` retrieves one item at a time, thus conserving memory when dealing with large datasets. |
Speed | It can be faster than iterating through all items when only a few are needed. |
Limitations
While `next()` is a powerful tool, it has some limitations:
- Iterator Exhaustion: Once an iterator is exhausted, it cannot be reset or reused without creating a new iterator.
- Error Handling: If the default value is not provided, attempting to access the next item from an exhausted iterator raises a `StopIteration` exception, which must be handled appropriately.
The `next()` function is an essential part of Python’s iterator protocol, providing a straightforward way to access elements in a controlled manner. Understanding its usage and implications is crucial for efficient Python programming, particularly in data manipulation and iteration control.
Understanding the Role of `next()` in Python Programming
Dr. Emily Carter (Senior Software Engineer, Python Innovations Inc.). The `next()` function in Python is essential for retrieving the next item from an iterator. It allows developers to efficiently traverse through iterable objects without needing to manually manage the index, making it a powerful tool for handling data streams and large datasets.
Michael Thompson (Lead Data Scientist, Tech Data Solutions). Utilizing `next()` is crucial when working with generators in Python. It provides a way to fetch the next value produced by the generator, enabling seamless iteration over potentially infinite sequences while maintaining memory efficiency.
Linda Zhang (Python Educator and Author, Code Academy). The `next()` function also accepts a second argument, which serves as a default value if the iterator is exhausted. This feature allows developers to avoid exceptions and handle cases where no further items are available, enhancing the robustness of the code.
Frequently Asked Questions (FAQs)
What does the `next()` function do in Python?
The `next()` function retrieves the next item from an iterator. If the iterator is exhausted, it raises a `StopIteration` exception unless a default value is provided.
How do you use the `next()` function with a default value?
You can provide a default value as the second argument in the `next()` function. For example, `next(iterator, default_value)` will return `default_value` if the iterator is exhausted.
Can `next()` be used with generators?
Yes, `next()` works seamlessly with generators, allowing you to retrieve the next value produced by the generator function.
What happens if you call `next()` on an empty iterator?
Calling `next()` on an empty iterator without a default value will raise a `StopIteration` exception, indicating that there are no more items to retrieve.
Is `next()` a built-in function in Python?
Yes, `next()` is a built-in function in Python, available in all versions, and is commonly used for iterating through items in various iterable objects.
Can you use `next()` with lists or tuples?
While `next()` is primarily used with iterators, you can convert lists or tuples into iterators using the `iter()` function and then use `next()` to access their elements.
The `next()` function in Python is a built-in function that retrieves the next item from an iterator. It is commonly used in conjunction with iterators and generators, which are essential for efficient looping and handling of data streams. The function can take two arguments: the iterator from which to retrieve the next item and an optional default value that is returned if the iterator is exhausted. This feature prevents the `StopIteration` exception from being raised when the iterator has no more items to yield.
One of the key insights regarding the `next()` function is its ability to enhance code readability and efficiency. By using `next()`, developers can write cleaner code that avoids the need for explicit loop constructs when only a single item is needed from an iterator. This is particularly useful in scenarios where data is processed in a streaming fashion or when working with large datasets where memory efficiency is a concern.
Additionally, the versatility of `next()` allows for greater control over iteration processes. The optional default value provides a safety net, enabling developers to handle cases where an iterator may not yield further items without disrupting the flow of the program. Understanding and utilizing the `next()` function can significantly improve the handling of iterators in Python, making it a valuable tool for any
Author Profile
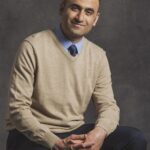
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?