How Do You Create an Empty Dictionary in Python?
In the world of Python programming, dictionaries stand out as one of the most versatile and powerful data structures. They allow you to store data in key-value pairs, making it easy to retrieve, manipulate, and organize information efficiently. Whether you’re building a complex application or simply managing data for a small project, knowing how to create and utilize dictionaries is essential. In this article, we will explore the fundamental concept of creating an empty dictionary in Python, a crucial first step that opens the door to countless possibilities in your coding journey.
Creating an empty dictionary is a straightforward yet vital skill for any Python developer. This foundational concept serves as the building block for more complex data structures and algorithms. With an empty dictionary, you can dynamically add, modify, and remove entries as your program evolves, allowing for greater flexibility and control over your data. Understanding the syntax and methods associated with empty dictionaries will not only enhance your coding efficiency but also pave the way for more advanced programming techniques.
As we delve into the specifics of creating an empty dictionary, we will cover various approaches, including the use of literal syntax and the built-in `dict()` function. Additionally, we will touch upon practical scenarios where initializing an empty dictionary can streamline your code and improve its readability. By the end of this article, you
Creating an Empty Dictionary
In Python, creating an empty dictionary can be accomplished using one of two primary methods. Each method is straightforward and serves as a foundation for initializing dictionaries for various applications.
The first method employs curly braces, which is the most common approach. By using an empty pair of braces, you can quickly establish a new dictionary.
“`python
empty_dict = {}
“`
The second method utilizes the `dict()` constructor. This method is particularly useful if you prefer a more explicit approach or if you need to create a dictionary in a context where you might also want to pass initial values.
“`python
empty_dict = dict()
“`
Both methods achieve the same result, and the choice between them often comes down to personal preference or specific use cases in your code.
Using the Empty Dictionary
An empty dictionary can be populated dynamically, which makes it an essential tool in many programming scenarios. Here are some common operations you can perform on an empty dictionary:
- Adding Key-Value Pairs: You can easily add new entries to the dictionary using square brackets.
“`python
empty_dict[‘key1’] = ‘value1’
empty_dict[‘key2’] = ‘value2’
“`
- Updating Values: If a key already exists, assigning a new value to that key updates the existing entry.
“`python
empty_dict[‘key1’] = ‘new_value1’
“`
- Accessing Values: You can retrieve values by referencing their keys.
“`python
value = empty_dict[‘key1’]
“`
- Deleting Entries: You can remove entries using the `del` statement.
“`python
del empty_dict[‘key2’]
“`
Example of Empty Dictionary in Use
Here is a practical example demonstrating the use of an empty dictionary, showing how it can be populated and manipulated.
“`python
Create an empty dictionary
my_dict = {}
Add key-value pairs
my_dict[‘name’] = ‘Alice’
my_dict[‘age’] = 30
my_dict[‘city’] = ‘New York’
Update a value
my_dict[‘age’] = 31
Access a value
print(my_dict[‘name’]) Output: Alice
Delete a key-value pair
del my_dict[‘city’]
“`
Table of Dictionary Operations
The following table summarizes common operations that can be performed on dictionaries in Python:
Operation | Syntax | Description |
---|---|---|
Add Key-Value Pair | dict[key] = value | Adds a new entry or updates an existing key. |
Access Value | dict[key] | Retrieves the value associated with the specified key. |
Delete Entry | del dict[key] | Removes the key-value pair from the dictionary. |
Check Key Existence | key in dict | Returns True if the key is in the dictionary, otherwise. |
Understanding how to create and manipulate an empty dictionary is fundamental for effective programming in Python, providing a flexible structure for managing key-value pairs in your applications.
Creating an Empty Dictionary in Python
In Python, creating an empty dictionary can be accomplished in two primary ways. Both methods are widely accepted and commonly used in programming practices.
Method 1: Using Curly Braces
The most straightforward way to create an empty dictionary is by using curly braces `{}`. This method is concise and is often preferred for its simplicity.
“`python
empty_dict = {}
“`
Method 2: Using the `dict()` Constructor
Another approach to create an empty dictionary is by using the built-in `dict()` function. This method is equally effective and can be beneficial in scenarios where you might want to dynamically create dictionaries.
“`python
empty_dict = dict()
“`
Comparison of Methods
Method | Syntax | Advantages | Disadvantages |
---|---|---|---|
Curly Braces | `{}` | Simple and concise | None |
`dict()` Constructor | `dict()` | More explicit, can enhance readability | Slightly less concise |
Both methods yield the same result, and the choice between them often comes down to personal preference or specific coding standards in a project.
Adding Elements to the Dictionary
Once you have created an empty dictionary, you can add elements to it. This can be done using key-value pairs. Here’s how you can do that:
“`python
empty_dict[‘key1’] = ‘value1’
empty_dict[‘key2’] = ‘value2’
“`
After adding these elements, the dictionary will look like this:
“`python
{‘key1’: ‘value1’, ‘key2’: ‘value2’}
“`
Checking if a Dictionary is Empty
You can easily check if a dictionary is empty by using a simple condition:
“`python
if not empty_dict:
print(“The dictionary is empty.”)
“`
This condition evaluates to `True` if the dictionary has no items.
Creating an empty dictionary in Python is a fundamental skill that can enhance your coding efficiency. Whether you choose to use curly braces or the `dict()` constructor, both methods provide you with a clean slate to start populating your dictionary with data.
Expert Insights on Creating an Empty Dictionary in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Group). “Creating an empty dictionary in Python is straightforward and can be accomplished using either the curly braces method or the built-in dict() function. This simplicity is one of the reasons Python is favored for rapid development.”
Michael Chen (Lead Data Scientist, Tech Innovations Inc.). “In data science applications, initializing an empty dictionary is often the first step in data aggregation processes. It allows for dynamic key-value pair assignments as data is processed, making it a crucial tool in Python programming.”
Sarah Patel (Python Instructor, Code Academy). “When teaching beginners how to create an empty dictionary, I emphasize the importance of understanding its mutable nature. This allows learners to appreciate how dictionaries can be modified, which is essential for effective coding practices.”
Frequently Asked Questions (FAQs)
How do I create an empty dictionary in Python?
You can create an empty dictionary in Python using either the curly braces `{}` or the `dict()` constructor. For example, `my_dict = {}` or `my_dict = dict()`.
What is the difference between using `{}` and `dict()` to create a dictionary?
Both methods create an empty dictionary, but using `{}` is generally preferred for its simplicity and readability. The `dict()` constructor can be more useful when initializing a dictionary with specific key-value pairs.
Can I add items to an empty dictionary after creating it?
Yes, you can add items to an empty dictionary at any time by assigning values to keys. For example, `my_dict[‘key’] = ‘value’` adds a new key-value pair.
Is it possible to create a dictionary with default values?
Yes, you can create a dictionary with default values using the `fromkeys()` method. For example, `my_dict = dict.fromkeys([‘key1’, ‘key2’], ‘default_value’)` initializes keys with the same default value.
What are common use cases for empty dictionaries in Python?
Empty dictionaries are commonly used for collecting data, storing results during iterations, or initializing configurations that will be populated later in the program.
Are there any performance considerations when using dictionaries in Python?
Dictionaries in Python are implemented as hash tables, providing average-case O(1) time complexity for lookups, insertions, and deletions. However, excessive resizing during growth can impact performance, so it’s advisable to preallocate size if the number of entries is known in advance.
Creating an empty dictionary in Python is a straightforward process that can be accomplished using two primary methods. The first method involves using curly braces, which is the most common approach. By simply declaring a variable followed by an equals sign and two curly braces (e.g., `my_dict = {}`), you can initialize an empty dictionary. The second method utilizes the built-in `dict()` function, which also results in an empty dictionary when called without any arguments (e.g., `my_dict = dict()`). Both methods are equally effective and can be used based on personal or stylistic preference.
Understanding how to create an empty dictionary is fundamental for Python programming, as dictionaries are versatile data structures that allow for the storage of key-value pairs. They are particularly useful for situations where you need to associate unique keys with specific values, enabling efficient data retrieval and manipulation. By starting with an empty dictionary, programmers can dynamically add entries as needed during the execution of their code.
In summary, the creation of an empty dictionary in Python can be achieved through either curly braces or the `dict()` function. Both approaches are valid and serve the same purpose, allowing developers to build dictionaries that can be populated with data later. Mastering this concept is essential
Author Profile
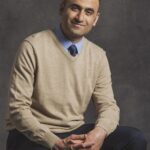
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?