How Can You Print to the Console in JavaScript?
### Introduction
In the realm of web development, JavaScript stands as a fundamental pillar, empowering developers to create dynamic and interactive web experiences. One of the most essential skills for any aspiring JavaScript programmer is mastering the art of outputting messages to the console. Whether you’re debugging a complex application or simply trying to understand how your code behaves, knowing how to print to the console is an invaluable tool in your programming arsenal. This article will guide you through the various methods of utilizing the console in JavaScript, ensuring you can effectively communicate with your code and troubleshoot with confidence.
Printing to the console in JavaScript is not just about displaying text; it’s a powerful way to gain insights into your code’s execution and state. The console serves as a real-time feedback mechanism, allowing developers to log variables, track function calls, and monitor application performance. By leveraging the console, you can dissect your code’s behavior, making it easier to identify errors and optimize your scripts.
As we delve deeper into the topic, you’ll discover the different functions available for console output, how to format your messages for clarity, and tips for using the console effectively during development. Whether you’re a novice just starting your coding journey or a seasoned developer looking to brush up on your skills, understanding how to print to the
Utilizing the Console Object
The console in JavaScript is primarily accessed via the `console` object, which provides various methods for outputting information to the web console. This functionality is crucial for debugging and inspecting the state of your application during development.
The primary methods available for printing messages to the console include:
- `console.log()`: Outputs a general message.
- `console.error()`: Outputs an error message, usually formatted in red.
- `console.warn()`: Outputs a warning message, often highlighted to draw attention.
- `console.info()`: Outputs an informational message, typically styled differently from logs.
- `console.debug()`: Outputs a debug message, useful for verbose debugging.
Basic Console Output
To print messages to the console, use the `console.log()` method. This method can accept multiple arguments, which will be concatenated into a single string. Here’s a simple example:
javascript
console.log(“Hello, World!”);
You can also log variables:
javascript
let name = “Alice”;
console.log(“Hello, ” + name);
For better readability and debugging, you can format your output using template literals:
javascript
let age = 25;
console.log(`Hello, ${name}. You are ${age} years old.`);
Advanced Console Features
The console object offers additional capabilities that enhance debugging and logging:
- Grouping logs: You can group related logs together for better organization:
javascript
console.group(“User Info”);
console.log(“Name: Alice”);
console.log(“Age: 25”);
console.groupEnd();
- Timing operations: You can measure the time taken by a block of code:
javascript
console.time(“Loop Time”);
for (let i = 0; i < 1000; i++) {}
console.timeEnd("Loop Time");
- Viewing objects: You can inspect objects using `console.table()` for a tabular representation:
javascript
let users = [
{ name: “Alice”, age: 25 },
{ name: “Bob”, age: 30 }
];
console.table(users);
Table of Console Methods
Method | Description |
---|---|
console.log() | |
console.error() | Outputs an error message to the console. |
console.warn() | Outputs a warning message to the console. |
console.info() | Outputs an informational message to the console. |
console.debug() | Outputs a debug message to the console. |
console.table() | Displays tabular data as a table. |
console.group() | Starts a new log group. |
console.groupEnd() | Ends the current log group. |
console.time() | Starts a timer with a specified label. |
console.timeEnd() | Stops the timer and outputs the elapsed time. |
Using `console.log` for Output
The primary method for printing output to the console in JavaScript is through the `console.log()` function. This function is versatile and can handle various data types, including strings, numbers, objects, and arrays.
- Basic Syntax:
javascript
console.log(value);
- Examples:
javascript
console.log(“Hello, World!”); // Output: Hello, World!
console.log(42); // Output: 42
console.log({ name: “Alice” }); // Output: { name: “Alice” }
console.log([1, 2, 3]); // Output: [1, 2, 3]
Formatting Output
To enhance readability, `console.log` supports string interpolation and formatting options. You can use template literals or concatenation for structured output.
- Template Literals:
javascript
const name = “Bob”;
console.log(`User: ${name}`); // Output: User: Bob
- Concatenation:
javascript
const age = 30;
console.log(“Age: ” + age); // Output: Age: 30
Console Methods for Different Purposes
JavaScript provides several console methods tailored for specific tasks, enhancing the debugging and logging experience.
Method | Description |
---|---|
`console.error()` | Outputs an error message, usually in red. |
`console.warn()` | Outputs a warning message, typically yellow. |
`console.info()` | Outputs informational messages, generally styled differently. |
`console.table()` | Displays tabular data in a visually appealing format. |
- Example:
javascript
console.error(“This is an error!”); // Output: This is an error!
console.warn(“This is a warning!”); // Output: This is a warning!
Logging Objects and Arrays
When printing complex objects or arrays, `console.log` can also display the structure of the data, allowing for easier inspection.
- Example:
javascript
const user = {
name: “Charlie”,
age: 28,
hobbies: [“reading”, “gaming”]
};
console.log(user); // Output: { name: “Charlie”, age: 28, hobbies: [“reading”, “gaming”] }
- Using `console.table`:
This method is particularly useful for arrays and objects, presenting them in a tabular format.
javascript
const users = [
{ name: “Alice”, age: 30 },
{ name: “Bob”, age: 25 }
];
console.table(users);
Advanced Features
JavaScript console also supports advanced features, such as grouping logs and timing operations.
- Grouping Logs:
Use `console.group()` and `console.groupEnd()` to group related log messages.
javascript
console.group(“User Information”);
console.log(“Name: Alice”);
console.log(“Age: 30”);
console.groupEnd();
- Timing Operations:
Measure how long an operation takes using `console.time()` and `console.timeEnd()`.
javascript
console.time(“Process Time”);
// Some code execution
console.timeEnd(“Process Time”); // Outputs the elapsed time
By leveraging these methods and features, developers can effectively utilize the console for debugging and monitoring JavaScript applications.
Expert Insights on Printing to Console in JavaScript
Dr. Emily Carter (Senior JavaScript Developer, Tech Innovations Inc.). “Utilizing the console in JavaScript is fundamental for debugging and monitoring application behavior. The `console.log()` method is the most commonly used function for outputting information to the console, allowing developers to track variable values and application flow effectively.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “When printing to the console, it’s essential to understand the various console methods available. Besides `console.log()`, methods like `console.error()` and `console.warn()` provide different levels of output, which can help in categorizing messages and errors during development.”
Sarah Patel (JavaScript Educator, Web Development Academy). “For beginners, mastering the console is a crucial step in learning JavaScript. I encourage my students to experiment with `console.table()` for displaying arrays and objects in a more readable format, which enhances their debugging skills and understanding of data structures.”
Frequently Asked Questions (FAQs)
How do I print a message to the console in JavaScript?
You can print a message to the console in JavaScript using the `console.log()` method. For example, `console.log(“Hello, World!”);` will output “Hello, World!” to the console.
What are the different console methods available in JavaScript?
JavaScript provides several console methods, including `console.log()`, `console.error()`, `console.warn()`, `console.info()`, and `console.table()`, each serving different purposes for logging messages, errors, warnings, and structured data.
Can I print variables to the console in JavaScript?
Yes, you can print variables to the console by passing them as arguments to `console.log()`. For example, `let x = 5; console.log(x);` will output the value of `x`, which is 5.
Is it possible to format console output in JavaScript?
Yes, you can format console output using placeholders within strings. For example, `console.log(“Value: %d”, 10);` will format the number 10 as an integer, and `console.log(“Hello, %s”, “World”);` will format “World” as a string.
How can I clear the console in JavaScript?
You can clear the console by using the `console.clear()` method. This will remove all previous messages from the console, providing a clean slate for further output.
What is the difference between console.log() and console.error()?
`console.log()` is used for general logging of information, while `console.error()` is specifically intended for logging error messages. The latter typically displays messages in red, making them stand out for debugging purposes.
In summary, printing to the console in JavaScript is primarily accomplished using the `console.log()` method. This method is part of the Console API, which provides various functions that allow developers to output messages, warnings, errors, and other information to the browser’s console. Utilizing `console.log()` is essential for debugging and monitoring the flow of a program, as it helps developers understand how their code is executing and identify potential issues.
Additionally, JavaScript offers other console methods such as `console.error()`, `console.warn()`, and `console.info()`, which serve specific purposes for categorizing messages. These methods enhance the debugging process by allowing developers to differentiate between types of messages, making it easier to spot errors or warnings in the console output. Understanding these various methods can significantly improve the efficiency of debugging and logging practices.
Moreover, using the console effectively can lead to better code quality and maintainability. By incorporating console statements thoughtfully, developers can trace the execution of their code and gain insights into variable states at different points in time. This practice not only aids in immediate debugging but also serves as documentation for future reference, making it easier for other developers to understand the code’s behavior.
Author Profile
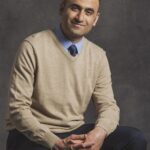
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?