How Can You Remove Leading Zeros in SQL?
In the world of databases, data integrity and formatting are paramount. One common issue that developers and database administrators encounter is the presence of leading zeros in numeric fields. While leading zeros might be necessary for certain identifiers, they can often lead to complications in data processing, sorting, and analysis. Whether you’re working with strings that represent numerical values or manipulating data for reporting purposes, knowing how to effectively remove leading zeros in SQL can streamline your operations and enhance data accuracy.
This article delves into the various methods and functions available in SQL for stripping away those pesky leading zeros. From built-in string manipulation functions to more complex queries, we will explore the tools at your disposal for achieving clean, usable data. Understanding the nuances of different SQL dialects will also be crucial, as the approach may vary depending on the database system you are using.
By the end of this guide, you will be equipped with the knowledge to tackle leading zeros confidently, ensuring your data is formatted correctly for whatever application you have in mind. Let’s dive into the specifics of removing leading zeros in SQL and discover how to optimize your database management practices.
Using CAST and CONVERT to Remove Leading Zeros
One common approach to eliminate leading zeros from a string in SQL is by utilizing the `CAST` or `CONVERT` functions. These functions can transform a string to a numeric type, effectively discarding any leading zeros.
For instance, consider the following SQL statement:
“`sql
SELECT CAST(‘000123’ AS INT) AS NumberWithoutLeadingZeros;
“`
The result will be:
NumberWithoutLeadingZeros |
---|
123 |
Similarly, using `CONVERT` achieves the same result:
“`sql
SELECT CONVERT(INT, ‘000123’) AS NumberWithoutLeadingZeros;
“`
Both methods are straightforward and effective for converting strings to integers, thereby removing any leading zeros.
Using REPLACE for Specific Patterns
In some scenarios, you may only want to remove leading zeros while retaining other zeros within the string. The `REPLACE` function can be customized to target specific patterns.
Here’s how you can accomplish this:
“`sql
SELECT REPLACE(‘000123’, ‘000’, ”) AS CleanedNumber;
“`
This will yield:
CleanedNumber |
---|
123 |
However, this method is limited to cases where you know the exact pattern of leading zeros you wish to remove.
Regular Expressions for Advanced Cases
For more complex cases, especially when dealing with variable-length strings containing leading zeros, using a regular expression can be very effective. Some SQL databases, such as PostgreSQL or MySQL, provide support for regex functions.
For example, in PostgreSQL, you can use `REGEXP_REPLACE`:
“`sql
SELECT REGEXP_REPLACE(‘000123’, ‘^0+’, ”) AS CleanedNumber;
“`
This expression will match any leading zeros and replace them with an empty string, resulting in:
CleanedNumber |
---|
123 |
Using String Manipulation Functions
Another option is to use string manipulation functions, such as `SUBSTRING` and `PATINDEX`, to identify and remove leading zeros.
Here’s an example:
“`sql
SELECT
SUBSTRING(‘000123’, PATINDEX(‘%[1-9]%’, ‘000123’), LEN(‘000123’)) AS NumberWithoutLeadingZeros;
“`
This approach identifies the position of the first non-zero character and extracts the substring from that point forward. The output will be:
NumberWithoutLeadingZeros |
---|
123 |
Summary of Methods
The following table summarizes the different methods to remove leading zeros in SQL:
Method | Function Used | Notes |
---|---|---|
CAST / CONVERT | CAST, CONVERT | Simple and effective for numeric conversions. |
REPLACE | REPLACE | Best for known patterns of leading zeros. |
Regular Expressions | REGEXP_REPLACE | Advanced pattern matching, available in some databases. |
String Manipulation | SUBSTRING, PATINDEX | Flexible for variable-length strings. |
Methods to Remove Leading Zeros in SQL
In SQL, removing leading zeros from strings or numeric values can be accomplished through various functions and techniques, depending on the specific SQL dialect in use. Below are common methods applicable to different databases.
Using CAST or CONVERT Functions
For SQL Server, MySQL, and PostgreSQL, the `CAST` or `CONVERT` functions can be utilized to transform a string into a numeric type, effectively removing leading zeros.
Example in SQL Server:
“`sql
SELECT CAST(‘000123’ AS INT) AS Result;
“`
Example in MySQL:
“`sql
SELECT CAST(‘000123’ AS UNSIGNED) AS Result;
“`
Example in PostgreSQL:
“`sql
SELECT CAST(‘000123’ AS INTEGER) AS Result;
“`
Using TRIM and LTRIM Functions
You can also use the `LTRIM` function to remove leading spaces, but for leading zeros, a combination of string manipulation functions may be necessary.
Example in SQL Server:
“`sql
SELECT LTRIM(STR(CAST(‘000123’ AS INT))) AS Result;
“`
Example in MySQL:
“`sql
SELECT LTRIM(LEADING ‘0’ FROM ‘000123’) AS Result;
“`
Example in PostgreSQL:
“`sql
SELECT LTRIM(‘000123’, ‘0’) AS Result;
“`
Regular Expressions
For databases that support regular expressions, such as PostgreSQL, you can use regex functions to remove leading zeros.
Example in PostgreSQL:
“`sql
SELECT REGEXP_REPLACE(‘000123’, ‘^0+’, ”) AS Result;
“`
For MySQL 8.0 and later, you can use:
“`sql
SELECT REGEXP_REPLACE(‘000123’, ‘^0+’, ”) AS Result;
“`
Using Mathematical Operations
In some cases, performing a mathematical operation can convert a string with leading zeros into a number, thus removing them.
Example in SQL Server:
“`sql
SELECT ‘000123’ * 1 AS Result;
“`
Example in MySQL:
“`sql
SELECT ‘000123’ + 0 AS Result;
“`
Example in PostgreSQL:
“`sql
SELECT ‘000123’::INTEGER AS Result;
“`
Performance Considerations
When selecting a method to remove leading zeros, consider the following:
- Data Type: If the column is already a numeric type, leading zeros are not stored.
- Performance: Mathematical operations may perform better than string manipulations.
- Database Version: Functions and capabilities may vary depending on the version of the database.
Example Table of SQL Queries
Database | Method | Query Example |
---|---|---|
SQL Server | CAST | `SELECT CAST(‘000123’ AS INT);` |
MySQL | LTRIM | `SELECT LTRIM(LEADING ‘0’ FROM ‘000123’);` |
PostgreSQL | REGEXP_REPLACE | `SELECT REGEXP_REPLACE(‘000123’, ‘^0+’, ”);` |
SQL Server | Mathematical Operation | `SELECT ‘000123’ * 1;` |
This table summarizes the methods for removing leading zeros across different SQL dialects, providing a quick reference for implementation.
Expert Insights on Removing Leading Zeros in SQL
Dr. Emily Carter (Database Architect, Tech Solutions Inc.). “Removing leading zeros in SQL can be achieved efficiently using the CAST or CONVERT functions. This approach not only simplifies data manipulation but also enhances performance when dealing with large datasets.”
James Liu (Senior Data Analyst, Data Insights Group). “It is crucial to consider the data type when removing leading zeros. Converting a string to an integer will inherently strip leading zeros, but one must ensure that the data integrity is maintained throughout the process.”
Linda Martinez (SQL Consultant, QueryMasters LLC). “Utilizing the TRIM function in combination with string manipulation techniques can be a robust solution for removing leading zeros. This method allows for greater flexibility, especially when dealing with mixed data formats.”
Frequently Asked Questions (FAQs)
How can I remove leading zeros from a string in SQL?
You can use the `CAST` or `CONVERT` functions to change the string to an integer, which automatically removes leading zeros. For example: `SELECT CAST(your_column AS INT) FROM your_table;`.
Is there a way to remove leading zeros without converting to an integer?
Yes, you can use the `REPLACE` function in combination with `SUBSTRING` to remove leading zeros. For instance: `SELECT REPLACE(your_column, ‘0’, ”) WHERE your_column LIKE ‘0%’;`.
What happens if I remove leading zeros from a numeric value?
Removing leading zeros from a numeric value does not affect its value, as integers do not store leading zeros. However, it may change the format of the output if the value is originally stored as a string.
Can I remove leading zeros from a column in a SQL table permanently?
Yes, you can update the column using an `UPDATE` statement along with the `CAST` function. For example: `UPDATE your_table SET your_column = CAST(your_column AS INT);`.
Are there any performance considerations when removing leading zeros in SQL?
Performance may vary based on the size of the dataset and the complexity of the query. Using `CAST` or `CONVERT` is generally efficient, but testing on large datasets is advisable to gauge performance impacts.
Is there a difference between removing leading zeros from a string and a number?
Yes, when dealing with strings, leading zeros can be removed using string manipulation functions, while for numeric types, they are inherently not stored. The approach varies based on the data type being used.
Removing leading zeros in SQL is a common requirement when dealing with numeric data that may have been stored as strings. This process is essential for ensuring accurate data representation and facilitating numerical operations. Various SQL functions, such as CAST, CONVERT, and the use of string manipulation functions like LTRIM, can effectively strip leading zeros from string values. Understanding the specific requirements of your database system is crucial, as syntax and functions may vary across different SQL implementations.
One key takeaway is the importance of data type consideration when removing leading zeros. Converting a string to an integer will automatically eliminate any leading zeros, but this approach may not be suitable if the original data needs to retain its string format for further processing. Therefore, it is essential to choose the appropriate method based on the intended use of the data after the transformation.
Additionally, it’s important to test the removal of leading zeros thoroughly, especially in scenarios where the data might include valid leading zeros that should be preserved. Implementing a robust validation process can help prevent data loss and ensure that the integrity of the dataset is maintained. Overall, removing leading zeros in SQL requires careful planning and execution to align with the specific needs of the application and the data being handled.
Author Profile
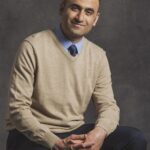
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?