How Can You Use SELECT INTO from a Stored Procedure in SQL Server?
In the world of database management, SQL Server stands out as a powerful tool that enables developers and data analysts to manipulate and retrieve data efficiently. One of the most compelling features of SQL Server is its ability to streamline data operations through stored procedures, which encapsulate complex queries and logic into reusable components. Among the many functionalities that stored procedures offer, the `SELECT INTO` statement is particularly noteworthy. This command not only simplifies the process of creating new tables from existing data but also enhances performance by reducing the need for multiple queries. In this article, we will delve into the intricacies of using `SELECT INTO` within stored procedures, exploring its syntax, best practices, and potential pitfalls.
When working with SQL Server, the `SELECT INTO` statement serves as a versatile tool for data manipulation. It allows users to select data from one or more tables and insert it directly into a new table, all in a single operation. This capability is especially useful for tasks such as data archiving, reporting, and creating temporary tables for complex data transformations. By leveraging stored procedures, developers can encapsulate this functionality, making it easier to maintain and execute these operations consistently.
However, while the `SELECT INTO` statement offers significant advantages, it also comes with its own set of considerations
Understanding SELECT INTO in Stored Procedures
The `SELECT INTO` statement in SQL Server is a powerful tool that allows users to create a new table based on the results of a query. When used within a stored procedure, it can facilitate complex data manipulations and temporary data storage without the need for prior table creation. This is particularly useful for scenarios where data transformation or aggregation is necessary before final output.
To utilize `SELECT INTO` within a stored procedure, the syntax generally follows this format:
“`sql
CREATE PROCEDURE ProcedureName
AS
BEGIN
SELECT Column1, Column2, …
INTO NewTable
FROM ExistingTable
WHERE Conditions;
END;
“`
By executing this stored procedure, SQL Server will create `NewTable` with the specified columns extracted from `ExistingTable`, based on any conditions provided.
Key Benefits of Using SELECT INTO
Utilizing `SELECT INTO` in stored procedures offers several advantages:
- Simplicity: Automatically creates a new table with the desired structure.
- Efficiency: Reduces the number of steps required to create and populate a new table.
- Flexibility: Supports a wide range of SQL queries, including joins and aggregations.
- Isolation: The new table is separate, preventing impacts on existing data structures.
Example of SELECT INTO in a Stored Procedure
Consider an example where you want to create a summary table of sales data from an existing sales records table. The procedure would look like this:
“`sql
CREATE PROCEDURE CreateSalesSummary
AS
BEGIN
SELECT ProductID, SUM(Quantity) AS TotalQuantity, SUM(Amount) AS TotalSales
INTO SalesSummary
FROM SalesRecords
GROUP BY ProductID;
END;
“`
When executed, this procedure will create a new table called `SalesSummary` that aggregates sales data by `ProductID`.
Common Use Cases for SELECT INTO
The following are common scenarios where `SELECT INTO` is particularly useful:
- Data Archiving: Moving historical data into a new table for archiving while keeping the original table intact.
- Data Transformation: Creating a new table that contains transformed data for reporting or further analysis.
- Temporary Tables: Generating tables that will only be used for the duration of a session or specific procedure execution.
Considerations When Using SELECT INTO
While `SELECT INTO` is beneficial, there are important considerations to keep in mind:
- Table Creation: The new table will be created with the same data types as the selected columns, but it will not inherit indexes, constraints, or triggers from the original table.
- Performance: Creating large tables can have performance implications; consider using temporary tables for large data sets.
- Permissions: Ensure that the executing user has the necessary permissions to create tables in the target schema.
Aspect | Details |
---|---|
Creation of Table | Creates new table with selected columns |
Data Types | Follows the data types of the source columns |
Indexes & Constraints | Not copied to the new table |
Performance | Considered for large datasets |
Using SELECT INTO in a Stored Procedure
The `SELECT INTO` statement in SQL Server allows you to create a new table and populate it with data from an existing table. This can be effectively utilized within stored procedures to facilitate data manipulation and reporting.
Syntax of SELECT INTO
The basic syntax for using `SELECT INTO` is as follows:
“`sql
SELECT column1, column2, …
INTO new_table
FROM existing_table
WHERE condition;
“`
This command selects specified columns from the `existing_table` and creates a new table named `new_table` populated with the results.
Implementing SELECT INTO in a Stored Procedure
To create a stored procedure that utilizes `SELECT INTO`, follow these steps:
- Define the stored procedure.
- Use the `SELECT INTO` statement to create the new table.
Example:
“`sql
CREATE PROCEDURE CreateNewTable
AS
BEGIN
SELECT EmployeeID, FirstName, LastName
INTO NewEmployees
FROM Employees
WHERE HireDate > ‘2022-01-01’;
END;
“`
In this example, the stored procedure `CreateNewTable` creates a new table named `NewEmployees` containing records of employees hired after January 1, 2022.
Considerations and Best Practices
When using `SELECT INTO` within stored procedures, consider the following:
- Table Existence: If `NewEmployees` already exists, the procedure will fail. Ensure the table does not exist before running the procedure, or use `DROP TABLE` to remove it.
- Data Types: The new table inherits the data types of the selected columns. Ensure compatibility when working with different data sources.
- Indexes and Constraints: The new table will not have any indexes or constraints from the source table. You may need to add these manually after table creation.
Example with Error Handling
To enhance robustness, you can include error handling in your stored procedure:
“`sql
CREATE PROCEDURE CreateNewTableWithErrorHandling
AS
BEGIN
BEGIN TRY
IF OBJECT_ID(‘NewEmployees’, ‘U’) IS NOT NULL
DROP TABLE NewEmployees;
SELECT EmployeeID, FirstName, LastName
INTO NewEmployees
FROM Employees
WHERE HireDate > ‘2022-01-01’;
END TRY
BEGIN CATCH
SELECT ERROR_NUMBER() AS ErrorNumber,
ERROR_MESSAGE() AS ErrorMessage;
END CATCH;
END;
“`
This version checks for the existence of `NewEmployees` and drops it if it exists, handling any potential errors during execution.
Performance Considerations
- Batch Size: When dealing with large datasets, consider processing in batches to avoid locking and performance degradation.
- Transaction Management: For critical operations, encapsulate `SELECT INTO` within a transaction to maintain data integrity.
Aspect | Recommendation |
---|---|
Existence Check | Use `OBJECT_ID` to check before drop. |
Error Handling | Implement `TRY…CATCH` blocks. |
Data Volume | Process in smaller batches if needed. |
Transaction Scope | Use transactions for data integrity. |
Utilizing `SELECT INTO` within stored procedures is a powerful method for creating and populating tables dynamically. By following best practices and considering performance implications, you can effectively manage data operations in SQL Server.
Expert Insights on SQL Server’s SELECT INTO from Stored Procedures
Maria Chen (Senior Database Administrator, Tech Solutions Inc.). “Using the SELECT INTO statement within a stored procedure in SQL Server is a powerful way to create new tables based on existing data. However, it is crucial to ensure that the target table does not already exist, as this will result in an error. Proper error handling and checks should be implemented to enhance the robustness of the procedure.”
James Patel (SQL Server Developer, Data Insights Group). “When utilizing SELECT INTO in a stored procedure, performance can be significantly impacted, especially with large datasets. It is advisable to consider indexing strategies and the overall database design to optimize the execution time. Additionally, testing with various data volumes can provide insights into potential bottlenecks.”
Linda Gomez (Data Architect, Innovative Tech Solutions). “Incorporating SELECT INTO within stored procedures can streamline data transformation processes. However, one must be cautious about data types and constraints, as the new table will inherit the structure of the selected columns. It is essential to review the implications of this inheritance to avoid data integrity issues.”
Frequently Asked Questions (FAQs)
What is the purpose of using SELECT INTO in SQL Server?
SELECT INTO is used to create a new table and populate it with the result set of a query. It allows for efficient data transfer from one table to another or the creation of a temporary table for further processing.
Can I use SELECT INTO within a stored procedure in SQL Server?
Yes, you can use SELECT INTO within a stored procedure. This allows you to dynamically create tables based on the results of queries executed within the procedure.
What are the limitations of using SELECT INTO?
SELECT INTO cannot be used to insert data into an existing table. Additionally, the new table inherits the data types of the selected columns but does not inherit indexes, constraints, or triggers from the source table.
How does SELECT INTO handle data types and NULL values?
When using SELECT INTO, SQL Server automatically determines the data types of the new table’s columns based on the source columns. NULL values from the source are preserved in the new table unless specified otherwise.
Is it possible to use SELECT INTO with joins in a stored procedure?
Yes, SELECT INTO can be used with joins in a stored procedure. This allows you to create a new table that contains the result of complex queries involving multiple tables.
What happens if the target table already exists when using SELECT INTO?
If the target table already exists, SQL Server will return an error when attempting to use SELECT INTO. To avoid this, ensure that the table does not exist before executing the SELECT INTO command or consider using INSERT INTO instead.
In SQL Server, the `SELECT INTO` statement is a powerful feature that allows users to create a new table based on the results of a query. This functionality can be particularly useful when working with stored procedures, as it enables developers to encapsulate complex data manipulation logic while simultaneously creating and populating tables. By utilizing `SELECT INTO` within a stored procedure, users can streamline data processing tasks and enhance the overall efficiency of their database operations.
When implementing `SELECT INTO` in a stored procedure, it is crucial to ensure that the target table does not already exist, as this will result in an error. Additionally, the structure of the new table is determined by the columns and data types of the selected data. This means that developers must carefully plan the query to ensure that the resulting table meets the intended design specifications. Proper error handling and validation mechanisms should also be incorporated to manage potential issues during execution.
One key takeaway is that using `SELECT INTO` within stored procedures can significantly reduce the amount of code required for data manipulation tasks. This approach not only simplifies the process but also enhances maintainability and readability. Furthermore, it is advisable to consider performance implications, as creating large tables from extensive datasets can impact system resources. Therefore, developers
Author Profile
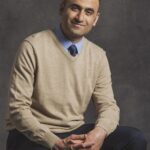
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?