How Can I Effectively Clear Event Listeners in JavaScript?
### Introduction
In the dynamic world of web development, event listeners play a crucial role in creating interactive and responsive user experiences. They allow developers to respond to user actions, such as clicks, key presses, and mouse movements, making websites feel alive and engaging. However, as applications grow in complexity, managing these event listeners becomes increasingly important to ensure optimal performance and prevent memory leaks. If you’ve ever found yourself grappling with unresponsive elements or sluggish interfaces, understanding how to clear event listeners in JavaScript is essential. This article will guide you through the nuances of effectively managing event listeners, empowering you to build cleaner, more efficient code.
When working with event listeners, it’s easy to overlook the importance of removing them when they are no longer needed. Failing to do so can lead to unnecessary memory consumption and can cause unexpected behavior in your applications. Whether you’re dealing with temporary listeners that should only respond to a single interaction or managing a complex state where listeners need to be dynamically added and removed, knowing the right techniques can save you time and frustration.
In this article, we will explore various methods for clearing event listeners in JavaScript, highlighting best practices and common pitfalls to avoid. From understanding the difference between anonymous and named functions to leveraging modern JavaScript features, you’ll gain
Removing Event Listeners
To effectively manage event listeners in JavaScript, it is crucial to know how to remove them when they are no longer needed. This is especially important for optimizing performance and preventing memory leaks in applications.
Event listeners can be removed using the `removeEventListener` method. However, this method requires that the exact same function reference be used when removing the listener. This means that if you defined an anonymous function, you will not be able to remove it later, since there is no reference to that function.
Here is the general syntax for removing an event listener:
javascript
element.removeEventListener(event, function, useCapture);
- event: The name of the event (e.g., ‘click’, ‘mouseover’).
- function: The function that was originally registered as the event listener.
- useCapture: A boolean that indicates whether the event should be captured or not.
Example of Adding and Removing an Event Listener
Consider the following example, which demonstrates how to properly add and remove an event listener:
javascript
function handleClick() {
console.log(‘Element clicked!’);
}
const button = document.getElementById(‘myButton’);
// Adding the event listener
button.addEventListener(‘click’, handleClick);
// Removing the event listener
button.removeEventListener(‘click’, handleClick);
In this example, the `handleClick` function is defined separately, allowing it to be referenced when removing the event listener. If an anonymous function was used, it would not be possible to remove it.
Common Scenarios for Clearing Event Listeners
There are several scenarios where clearing event listeners is necessary:
- Dynamic Element Creation: When elements are created and destroyed dynamically in the DOM, ensuring that event listeners are removed from elements that no longer exist helps avoid memory leaks.
- Single-Use Listeners: If an event listener should only respond to an event once, it is good practice to remove it after the first invocation.
- State Changes: In applications where the state changes frequently, you may need to update or remove event listeners based on the current state.
Best Practices for Event Listener Management
To manage event listeners effectively, consider the following best practices:
- Always use named functions instead of anonymous functions for event listeners if you plan to remove them later.
- Use `once` option in `addEventListener` to automatically remove the listener after its first invocation:
javascript
element.addEventListener(‘click’, handleClick, { once: true });
- Monitor the performance of your application to identify and eliminate any unnecessary event listeners.
Scenario | Action |
---|---|
Dynamic Elements | Remove listeners on element destruction |
Single Use | Use `once` option or remove after execution |
State Change | Update or remove listeners based on state |
By adhering to these practices, you can ensure that your application remains efficient and free of memory-related issues associated with event listeners.
Understanding Event Listeners in JavaScript
Event listeners are functions that are executed in response to specific events occurring within the DOM, such as clicks, key presses, or mouse movements. When you attach an event listener, it’s essential to manage it properly to prevent memory leaks or unintended behavior, especially when elements are removed or replaced in the DOM.
Removing Event Listeners
To effectively clear event listeners, you must ensure that you remove them accurately. The method for removing an event listener is `removeEventListener`, which requires that you specify the same parameters used when attaching the listener.
Syntax for Adding and Removing Event Listeners
Here is the syntax for adding and removing event listeners:
javascript
element.addEventListener(‘event’, handlerFunction, options);
element.removeEventListener(‘event’, handlerFunction, options);
- event: A string representing the event type, such as ‘click’ or ‘mouseover’.
- handlerFunction: The function that will be executed when the event occurs. It must be the same function reference used in `addEventListener`.
- options: An optional object that can include properties like `capture`, `once`, and `passive`.
Example of Clearing an Event Listener
javascript
function handleClick() {
console.log(“Element clicked!”);
}
// Adding the event listener
const button = document.getElementById(“myButton”);
button.addEventListener(“click”, handleClick);
// Removing the event listener
button.removeEventListener(“click”, handleClick);
In this example, the `handleClick` function is attached to the button element when it is clicked. To clear the listener, you call `removeEventListener` with the exact same parameters.
Challenges with Anonymous Functions
If you use an anonymous function as an event listener, you cannot remove it later, since there is no reference to the original function. For example:
javascript
button.addEventListener(“click”, function() {
console.log(“Element clicked!”);
});
// This will NOT remove the listener
button.removeEventListener(“click”, function() {
console.log(“Element clicked!”);
});
To avoid this issue, always use named functions when attaching event listeners if you plan to remove them later.
Removing All Event Listeners from an Element
JavaScript does not provide a built-in method to remove all event listeners from an element directly. However, you can achieve this using a workaround by replacing the element with a clone. This effectively resets the element without listeners:
javascript
const oldElement = document.getElementById(“myElement”);
const newElement = oldElement.cloneNode(true);
oldElement.parentNode.replaceChild(newElement, oldElement);
This method creates a deep clone of the element, including its attributes and child elements, but without any attached event listeners.
Considerations for Performance
When managing event listeners, consider the following:
- Memory Management: Regularly remove event listeners from elements that are no longer in use to avoid memory leaks.
- Performance: Excessive event listeners can lead to performance issues, especially on frequently triggered events like scroll or resize.
- Debouncing: For events that fire rapidly, consider debouncing techniques to limit the number of times an event handler runs.
By implementing these practices, you can maintain a cleaner and more efficient codebase while effectively managing event listeners in JavaScript.
Expert Insights on Clearing Event Listeners in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively clear event listeners in JavaScript, it is crucial to maintain a reference to the original function used when adding the listener. This allows developers to use the `removeEventListener` method accurately, preventing memory leaks and ensuring optimal performance of applications.”
James Liu (JavaScript Framework Specialist, CodeCraft Academy). “One common mistake developers make is trying to remove event listeners without preserving the function reference. Always ensure that the function you pass to `addEventListener` is the same one you use with `removeEventListener`. This practice is essential for maintaining clean and efficient code.”
Sarah Thompson (Front-End Developer, Web Solutions Group). “In scenarios where anonymous functions are used as event listeners, it becomes impossible to remove them later. To avoid this pitfall, always define your event handler functions separately. This approach not only simplifies the removal process but also enhances code readability.”
Frequently Asked Questions (FAQs)
How do I remove an event listener in JavaScript?
To remove an event listener in JavaScript, use the `removeEventListener` method. Ensure you pass the same event type and handler function that was used with `addEventListener`.
What is the difference between `removeEventListener` and `stopPropagation`?
`removeEventListener` completely removes an event listener from an element, while `stopPropagation` prevents the event from bubbling up to parent elements but does not remove the listener.
Can I clear all event listeners from an element in JavaScript?
JavaScript does not provide a built-in method to clear all event listeners from an element. You must manually remove each listener using `removeEventListener`.
What parameters are required for `removeEventListener`?
`removeEventListener` requires three parameters: the event type (e.g., ‘click’), the specific event handler function, and an optional options object that matches the one used in `addEventListener`.
How can I keep track of event listeners for removal?
To track event listeners, store references to the functions used as handlers in an array or object. This allows you to easily reference and remove them later.
Is it possible to clear event listeners for multiple elements at once?
You can iterate over a collection of elements and call `removeEventListener` on each one. However, you will need to ensure you have the correct function references for each element.
In JavaScript, managing event listeners is crucial for maintaining optimal performance and preventing memory leaks. To clear event listeners, developers can utilize the `removeEventListener` method, which requires a reference to the original function used when the listener was added. This method effectively detaches the specified event handler from the target element, ensuring that it no longer responds to the event.
It is important to note that when adding event listeners, especially in dynamic applications, storing a reference to the function is essential. Anonymous functions cannot be removed using `removeEventListener` since they do not have a reference. Therefore, defining named functions or using arrow functions assigned to variables is a best practice for managing event listeners efficiently.
Additionally, understanding the context in which event listeners are added and removed can help prevent unintended behavior. For instance, if an event listener is attached within a loop or a conditional statement, it may lead to multiple instances of the same listener being added, which can complicate removal. Regularly reviewing and clearing event listeners when they are no longer needed will contribute to better resource management and application performance.
Author Profile
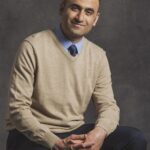
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?