How Can You Easily Add Integers in Python?
Python, renowned for its simplicity and versatility, has become a go-to programming language for beginners and experienced developers alike. One of the fundamental operations that every programmer encounters is the manipulation of numbers, particularly integers. Whether you’re building a complex application or just dabbling in coding, understanding how to add integers in Python is a crucial skill that lays the groundwork for more advanced mathematical operations. In this article, we will explore the various ways to perform integer addition in Python, ensuring you have the tools you need to tackle numerical tasks with confidence.
Adding integers in Python is not just about the mechanics of the operation; it also involves understanding the underlying principles of data types and the syntax that makes Python so user-friendly. From basic arithmetic to more complex scenarios involving user input and functions, the process is both straightforward and intuitive. We’ll delve into how Python handles integer addition, including the use of built-in operators and functions that simplify these tasks.
As we navigate through the world of integer addition, you’ll discover how Python’s dynamic typing and clear syntax make it easy to perform calculations without the steep learning curve associated with many other programming languages. Whether you’re looking to sum two numbers or perform more intricate calculations, this guide will provide you with the foundational knowledge you need to succeed in your
Basic Addition of Integers
In Python, adding integers is straightforward and can be accomplished using the `+` operator. This operator is used to perform arithmetic addition between two integer values. Here is a simple example:
“`python
a = 5
b = 10
result = a + b
print(result) Output: 15
“`
This code snippet demonstrates how to declare two integer variables, `a` and `b`, and add them together, storing the result in the variable `result`. The `print` function outputs the result to the console.
Adding Multiple Integers
When you need to add multiple integers, you can chain the `+` operator or use the built-in `sum()` function, which can be more efficient and cleaner in terms of readability. Here are both methods:
Using the `+` operator:
“`python
a = 5
b = 10
c = 15
result = a + b + c
print(result) Output: 30
“`
Using the `sum()` function:
“`python
numbers = [5, 10, 15]
result = sum(numbers)
print(result) Output: 30
“`
The `sum()` function takes an iterable as an argument and returns the total sum of its elements.
Adding Integers from User Input
To add integers based on user input, the `input()` function can be utilized. Since `input()` returns a string, you need to convert it to an integer using `int()`. Here is an example:
“`python
a = int(input(“Enter first integer: “))
b = int(input(“Enter second integer: “))
result = a + b
print(“The sum is:”, result)
“`
This code prompts the user to enter two integers and then calculates their sum.
Handling Potential Errors
When working with user input, it’s important to handle potential errors that may arise from invalid input. You can utilize a `try-except` block to catch exceptions:
“`python
try:
a = int(input(“Enter first integer: “))
b = int(input(“Enter second integer: “))
result = a + b
print(“The sum is:”, result)
except ValueError:
print(“Please enter valid integers.”)
“`
This implementation ensures that if the user inputs non-integer values, an error message is displayed instead of crashing the program.
Table of Addition Scenarios
Here is a table summarizing different scenarios for adding integers in Python:
Scenario | Code Example | Output |
---|---|---|
Basic Addition | a + b |
15 |
Multiple Addition | sum([a, b, c]) |
30 |
User Input Addition | int(input()) + int(input()) |
Dependent on user input |
Error Handling | try-except |
Error message on invalid input |
These examples and methods provide a comprehensive overview of how to add integers in Python, catering to various needs and scenarios.
Adding Integers in Python
In Python, adding integers is a straightforward process that utilizes the `+` operator. This operator can be applied directly to integer variables or literals, allowing for simple arithmetic operations.
Basic Addition
To add two integers, you simply write the integers separated by the `+` operator. Here’s a basic example:
“`python
a = 5
b = 10
result = a + b
print(result) Output: 15
“`
In this example, the integers `5` and `10` are added together, resulting in `15`.
Adding Multiple Integers
You can also add more than two integers at once. This can be done in several ways:
- Using multiple `+` operators:
“`python
a = 5
b = 10
c = 15
result = a + b + c
print(result) Output: 30
“`
- Using the `sum()` function, which is particularly useful for adding a list of integers:
“`python
numbers = [5, 10, 15]
result = sum(numbers)
print(result) Output: 30
“`
Adding Integers from User Input
To add integers based on user input, you can use the `input()` function combined with type conversion. Since `input()` returns a string, you need to convert it to an integer using `int()`. Here’s how:
“`python
a = int(input(“Enter first integer: “))
b = int(input(“Enter second integer: “))
result = a + b
print(f”The sum is: {result}”)
“`
This code snippet prompts the user to enter two integers and prints their sum.
Handling Invalid Input
When accepting user input, it’s essential to handle possible errors, such as entering non-integer values. You can use a `try-except` block to manage these situations gracefully:
“`python
try:
a = int(input(“Enter first integer: “))
b = int(input(“Enter second integer: “))
result = a + b
print(f”The sum is: {result}”)
except ValueError:
print(“Please enter valid integers.”)
“`
This ensures that the program does not crash if the user inputs invalid data.
Performance Considerations
Adding integers in Python is efficient, as it operates in constant time O(1). However, when dealing with large datasets, consider using built-in functions like `sum()` for better performance and readability.
Operation Type | Time Complexity |
---|---|
Adding two integers | O(1) |
Summing a list of n integers | O(n) |
Utilizing these techniques ensures you can handle integer addition effectively in various scenarios within Python programming.
Expert Insights on Adding Integers in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Adding integers in Python is straightforward due to its dynamic typing system. You simply use the ‘+’ operator, which allows for clean and readable code. This simplicity is one of Python’s strengths, making it accessible for beginners and efficient for experienced developers.”
Michael Chen (Lead Python Developer, CodeMasters). “When adding integers in Python, it is essential to be aware of the data types involved. Python can handle large integers seamlessly, but performance considerations may arise when dealing with extensive calculations. Utilizing built-in functions can optimize the process.”
Sarah Thompson (Computer Science Educator, Future Coders Academy). “Teaching how to add integers in Python provides a foundational understanding of programming logic. The ‘+’ operator not only performs addition but also introduces students to the concept of operators and expressions, which are crucial in coding.”
Frequently Asked Questions (FAQs)
How do I add two integers in Python?
You can add two integers in Python using the `+` operator. For example, `result = a + b`, where `a` and `b` are the integers you want to add.
Can I add integers and floats together in Python?
Yes, Python allows you to add integers and floats together. The result will be a float. For instance, `result = 5 + 3.2` will yield `8.2`.
What happens if I try to add a string to an integer in Python?
Attempting to add a string to an integer will raise a `TypeError` in Python. You must convert the string to an integer using `int()` before performing the addition.
Is there a built-in function to add integers in Python?
While there is no specific built-in function solely for addition, you can use the `sum()` function to add elements of an iterable, such as a list of integers. For example, `sum([1, 2, 3])` returns `6`.
Can I use the `+=` operator for adding integers in Python?
Yes, the `+=` operator is a shorthand for addition and assignment. For example, `a += b` is equivalent to `a = a + b`, effectively adding `b` to `a`.
How do I add multiple integers at once in Python?
You can add multiple integers at once by using the `+` operator or the `sum()` function. For instance, `result = a + b + c` or `result = sum([a, b, c])` both yield the sum of the integers.
In Python, adding integers is a straightforward process that can be accomplished using the addition operator `+`. This operator can be used with two or more integer values, and the result will be the sum of those values. Python’s dynamic typing allows for easy manipulation of integers without the need for explicit type declarations, making it user-friendly for both beginners and experienced programmers.
There are various ways to add integers in Python, including using variables, direct literals, and even within data structures like lists or tuples. For example, one can define integers as variables and then add them together, or directly sum them in a single expression. Additionally, Python supports the use of built-in functions such as `sum()` for adding elements in an iterable, which can simplify the process when dealing with multiple integers.
Key takeaways include the simplicity and flexibility of integer addition in Python. The language’s design allows for intuitive arithmetic operations, which can be performed in various contexts. Understanding how to effectively use the addition operator and related functions will enhance programming efficiency and enable more complex calculations as needed in various applications.
Author Profile
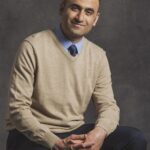
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?