How Can You Effectively Test Your JavaScript Code?
### Introduction
In the fast-paced world of web development, ensuring that your JavaScript code runs smoothly is paramount. With its ability to create dynamic and interactive user experiences, JavaScript has become a cornerstone of modern web applications. However, as applications grow in complexity, so does the need for rigorous testing. Whether you’re a seasoned developer or just starting out, understanding how to test JavaScript code is essential for delivering robust and reliable software. In this article, we will explore the various methodologies and tools available to help you effectively test your JavaScript code, ensuring that your applications not only function as intended but also provide a seamless experience for users.
Testing JavaScript code involves a combination of strategies that aim to identify bugs, ensure functionality, and validate performance. From unit tests that assess individual components to integration tests that evaluate how different parts of your application work together, the landscape of JavaScript testing is rich and varied. Additionally, the rise of testing frameworks and libraries has made it easier than ever to implement these strategies, allowing developers to write tests that are both efficient and easy to maintain.
As we delve deeper into the world of JavaScript testing, we will cover the essential concepts, tools, and best practices that can help you elevate your coding standards. Whether you’re looking to improve code quality
Unit Testing
Unit testing involves testing individual components or functions of your JavaScript code in isolation to ensure they perform as expected. This is typically done using a testing framework that provides a structure for organizing and executing tests. Popular JavaScript testing frameworks include:
- Jest
- Mocha
- Jasmine
Using these frameworks allows you to write test cases that can be executed automatically. A simple unit test might look like this in Jest:
javascript
function add(a, b) {
return a + b;
}
test(‘adds 1 + 2 to equal 3’, () => {
expect(add(1, 2)).toBe(3);
});
Integration Testing
Integration testing focuses on the interactions between different modules or services in your application. The goal is to identify issues that may arise when integrating various components. This type of testing is crucial for ensuring that your application behaves as expected when different parts work together.
Consider using tools such as:
- Cypress
- TestCafe
- Puppeteer
These tools can simulate user interactions and test how components function together. An example of an integration test using Cypress might look like this:
javascript
describe(‘User Login’, () => {
it(‘should log in successfully’, () => {
cy.visit(‘/login’);
cy.get(‘input[name=username]’).type(‘user’);
cy.get(‘input[name=password]’).type(‘password’);
cy.get(‘button[type=submit]’).click();
cy.url().should(‘include’, ‘/dashboard’);
});
});
End-to-End Testing
End-to-end (E2E) testing simulates real user scenarios and tests the entire application flow from start to finish. This ensures that all components work together seamlessly and that the application meets business requirements.
For E2E testing, tools such as Selenium, Cypress, and Playwright are commonly used. These tools provide a way to automate browser interactions and validate the user experience.
Tool | Language Support | Key Features |
---|---|---|
Selenium | Multiple languages (Java, Python, C#) | Cross-browser testing, large community support |
Cypress | JavaScript | Real-time reloads, easy debugging |
Playwright | JavaScript, Python, C# | Cross-browser support, multiple contexts |
Code Quality Tools
To ensure the quality of your JavaScript code, it’s essential to use static analysis tools that can identify potential issues before they become problems. Tools like ESLint and Prettier can help maintain code quality by enforcing coding standards and formatting.
- ESLint: A static code analysis tool that helps you identify and fix problems in your JavaScript code.
- Prettier: An opinionated code formatter that ensures a consistent style across your codebase.
Integrating these tools into your development workflow can lead to cleaner, more maintainable code and reduce the likelihood of bugs.
Continuous Integration
Incorporating testing into a continuous integration (CI) pipeline is a best practice for modern software development. CI tools such as Jenkins, Travis CI, and GitHub Actions can automate the testing process every time code is committed. This ensures that tests are run consistently and that any issues are caught early in the development cycle.
Key practices for effective CI include:
- Running all tests on each commit or pull request
- Reporting results clearly to the development team
- Ensuring that code quality tools are part of the CI process
By implementing these strategies, developers can maintain high-quality standards and deliver reliable JavaScript applications.
Unit Testing in JavaScript
Unit testing is a crucial practice in software development that involves testing individual components of the code to ensure they function as intended. In JavaScript, several frameworks facilitate unit testing.
– **Popular Testing Frameworks:**
– **Jest**: Developed by Facebook, it’s widely used for testing React applications but can be used for any JavaScript code.
– **Mocha**: A flexible test framework that can be paired with assertion libraries like Chai or Sinon.
– **Jasmine**: An all-in-one framework that offers a behavior-driven development approach.
– **Example Setup with Jest:**
- Install Jest via npm:
bash
npm install –save-dev jest
- Write a simple function to test:
javascript
function add(a, b) {
return a + b;
}
module.exports = add;
- Create a test file (`add.test.js`):
javascript
const add = require(‘./add’);
test(‘adds 1 + 2 to equal 3’, () => {
expect(add(1, 2)).toBe(3);
});
- Run tests:
bash
npx jest
Integration Testing
Integration testing focuses on verifying the interaction between multiple components or systems. This type of testing ensures that integrated parts of the application work together.
– **Tools for Integration Testing:**
– **Cypress**: A powerful testing framework for end-to-end testing and integration testing.
– **Supertest**: A popular library for testing HTTP servers.
– **Integration Testing Example with Cypress:**
- Install Cypress:
bash
npm install –save-dev cypress
- Create a basic test in `cypress/integration/sample_spec.js`:
javascript
describe(‘My First Test’, () => {
it(‘Visits the Kitchen Sink’, () => {
cy.visit(‘https://example.cypress.io’);
cy.contains(‘type’).click();
cy.url().should(‘include’, ‘/commands/actions’);
});
});
- Open Cypress:
bash
npx cypress open
End-to-End Testing
End-to-end (E2E) testing simulates user interactions with the application to ensure that it behaves as expected from start to finish.
– **E2E Testing Tools:**
– **Selenium**: An established framework for automating web applications.
– **Puppeteer**: A Node library that provides a high-level API over the Chrome DevTools Protocol.
– **Example of E2E Testing with Puppeteer:**
- Install Puppeteer:
bash
npm install puppeteer
- Write an E2E test:
javascript
const puppeteer = require(‘puppeteer’);
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto(‘https://example.com’);
await page.click(‘selector-for-button’);
await page.waitForSelector(‘selector-for-result’);
await browser.close();
})();
Static Code Analysis
Static code analysis tools help identify potential errors and enforce coding standards without executing the code.
- Popular Static Analysis Tools:
- ESLint: A widely used tool for identifying and fixing problems in JavaScript code.
- Prettier: An opinionated code formatter that ensures consistent styling.
- Setting Up ESLint:
- Install ESLint:
bash
npm install –save-dev eslint
- Initialize ESLint:
bash
npx eslint –init
- Run ESLint:
bash
npx eslint yourfile.js
Code Coverage
Code coverage measures how much of your code is tested by automated tests. It helps identify untested parts of your application.
- Tools for Measuring Code Coverage:
- Istanbul: A code coverage tool that integrates well with testing frameworks like Mocha and Jest.
- Integrating Code Coverage with Jest:
- Run Jest with coverage:
bash
npx jest –coverage
- Review the coverage report generated in the `coverage` folder.
By adopting these testing methodologies and tools, developers can ensure their JavaScript code is robust, reliable, and maintainable.
Expert Insights on Effective JavaScript Code Testing
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Testing JavaScript code effectively requires a combination of unit tests, integration tests, and end-to-end tests. Utilizing frameworks like Jest for unit testing and Cypress for end-to-end testing can significantly enhance the reliability of your codebase.”
Michael Chen (Lead Developer, Code Quality Solutions). “Incorporating test-driven development (TDD) into your workflow is crucial. By writing tests before the actual code, you ensure that your JavaScript functions perform as expected and remain maintainable over time.”
Sarah Lopez (JavaScript Consultant, Web Development Experts). “Automated testing is essential for JavaScript applications, especially when dealing with asynchronous code. Tools like Mocha and Chai can help streamline the testing process and ensure that your applications are robust against changes.”
Frequently Asked Questions (FAQs)
How can I test JavaScript code in a web browser?
You can test JavaScript code directly in a web browser by using the Developer Tools. Open the tools (usually F12 or right-click and select “Inspect”), navigate to the “Console” tab, and enter your JavaScript code there for immediate execution.
What are some popular JavaScript testing frameworks?
Popular JavaScript testing frameworks include Jest, Mocha, and Jasmine. These frameworks provide tools for writing and running tests, along with features for assertions and mocking.
How do I write a simple test using Jest?
To write a simple test using Jest, first install Jest in your project. Then, create a test file (e.g., `example.test.js`) and use the `test()` function to define a test case, followed by assertions using `expect()`. Finally, run the tests using the command `jest`.
What is the difference between unit testing and integration testing in JavaScript?
Unit testing focuses on testing individual components or functions in isolation to ensure they work as expected. Integration testing, on the other hand, tests how multiple components work together, ensuring that they interact correctly and produce the desired outcomes.
Can I test JavaScript code without a testing framework?
Yes, you can test JavaScript code without a framework by writing simple scripts that log output to the console or using assertions manually. However, using a testing framework simplifies the process and provides better organization and reporting of tests.
What tools can I use for end-to-end testing of JavaScript applications?
For end-to-end testing, popular tools include Selenium, Cypress, and Puppeteer. These tools automate browser interactions to simulate user behavior and validate that the application functions as intended across different scenarios.
Testing JavaScript code is a crucial aspect of software development that ensures the reliability and functionality of applications. Various methods and tools are available for testing, including unit testing, integration testing, and end-to-end testing. Each approach serves a specific purpose, allowing developers to identify bugs, verify functionality, and ensure that different parts of the application work together seamlessly. Popular testing frameworks such as Jest, Mocha, and Jasmine provide robust environments for writing and executing tests, making the process more efficient and manageable.
In addition to the choice of testing frameworks, understanding the importance of test coverage is vital. Test coverage measures the percentage of code that is tested by automated tests, helping developers identify untested areas that may harbor bugs. Striving for high test coverage can lead to more maintainable code and a more stable application. Furthermore, incorporating testing into the development workflow through practices like Test-Driven Development (TDD) can enhance code quality and reduce the likelihood of defects in production.
Moreover, leveraging tools such as Continuous Integration (CI) systems can automate the testing process, ensuring that tests are run consistently with every code change. This practice not only saves time but also fosters a culture of quality within development teams. By adopting a comprehensive testing strategy and
Author Profile
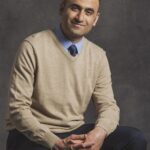
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?