How Can You Easily Copy a File in Python?
In the world of programming, the ability to manipulate files is a fundamental skill that every developer should master. Whether you’re working on a personal project, automating tasks, or developing a complex application, knowing how to copy a file in Python can save you time and streamline your workflow. Python, with its simple syntax and powerful libraries, makes file handling a breeze, allowing you to focus on what truly matters: your code and its functionality.
Copying files in Python is not just about duplicating content; it’s about understanding the underlying mechanisms that make file operations efficient and reliable. With built-in libraries like `shutil`, Python provides a straightforward approach to file manipulation, enabling you to replicate files with just a few lines of code. This capability is particularly useful in scenarios such as backing up important data, organizing project files, or even during data processing tasks where temporary copies are needed.
As we delve deeper into this topic, we’ll explore various methods for copying files, discuss best practices, and highlight common pitfalls to avoid. Whether you’re a beginner looking to grasp the basics or an experienced programmer seeking to refine your skills, this guide will equip you with the knowledge you need to handle file copying in Python effectively. Get ready to unlock the potential of Python’s file handling capabilities and enhance your programming toolkit
Using the shutil Module
The `shutil` module in Python provides a high-level interface for file operations, including copying files. It is a versatile library that simplifies various file operations. To copy a file, you can use the `shutil.copy()` or `shutil.copy2()` functions.
- `shutil.copy(src, dst)`: Copies the source file to the destination. The destination can be either a directory or a file path.
- `shutil.copy2(src, dst)`: Similar to `shutil.copy()`, but also attempts to preserve the file’s metadata.
Example usage of `shutil`:
“`python
import shutil
Copy a file
shutil.copy(‘source_file.txt’, ‘destination_file.txt’)
Copy a file and preserve metadata
shutil.copy2(‘source_file.txt’, ‘destination_directory/’)
“`
Using the os Module
The `os` module also allows file copying, but it requires more manual handling. You will need to read the contents of the source file and write them to the destination file. This method provides more control over the copying process.
Example:
“`python
import os
Function to copy a file
def copy_file(src, dst):
with open(src, ‘rb’) as fsrc:
with open(dst, ‘wb’) as fdst:
fdst.write(fsrc.read())
Usage
copy_file(‘source_file.txt’, ‘destination_file.txt’)
“`
Copying Files in a Directory
When working with multiple files, you may want to copy all files from one directory to another. The `shutil` module provides `shutil.copytree()` for this purpose.
Example:
“`python
shutil.copytree(‘source_directory/’, ‘destination_directory/’)
“`
This function will recursively copy an entire directory tree rooted at the source directory to the destination directory.
Performance Considerations
When copying files, especially large ones, performance can be a concern. Below is a comparison of the methods discussed:
Method | Metadata Preservation | Speed |
---|---|---|
shutil.copy() | No | Fast |
shutil.copy2() | Yes | Moderate |
os module (read/write) | No | Slow |
Choosing the appropriate method depends on your specific needs. For simple use cases, `shutil.copy()` is typically sufficient, while `shutil.copy2()` is ideal when metadata preservation is crucial. In contrast, using the `os` module offers greater flexibility at the cost of speed.
Copying Files Using the `shutil` Module
The `shutil` module in Python provides a simple way to perform high-level file operations, including copying files. It offers a straightforward function to copy files while maintaining their metadata.
- Basic Syntax:
“`python
import shutil
shutil.copy(src, dst)
“`
- Parameters:
- `src`: The path to the source file you wish to copy.
- `dst`: The destination path where the file will be copied.
- Example:
“`python
import shutil
src_file = ‘path/to/source/file.txt’
dst_file = ‘path/to/destination/file.txt’
shutil.copy(src_file, dst_file)
“`
This code snippet copies `file.txt` from the source directory to the destination directory.
Copying Files with Metadata
If you need to copy a file along with its metadata (such as permissions, timestamps), you can use the `shutil.copy2()` function.
- Basic Syntax:
“`python
shutil.copy2(src, dst)
“`
- Example:
“`python
import shutil
src_file = ‘path/to/source/file.txt’
dst_file = ‘path/to/destination/file.txt’
shutil.copy2(src_file, dst_file)
“`
This method ensures that the original file’s metadata is preserved in the copied file.
Using `os` Module for File Copying
Another method to copy files is by using the `os` module in combination with `shutil`. While `os` alone does not provide a direct copy function, it can be used for file operations.
- Using `os` for File Operations:
“`python
import os
import shutil
src_file = ‘path/to/source/file.txt’
dst_file = ‘path/to/destination/file.txt’
Ensure the destination directory exists
os.makedirs(os.path.dirname(dst_file), exist_ok=True)
shutil.copy(src_file, dst_file)
“`
In this example, `os.makedirs` ensures that the destination directory exists before copying the file.
Handling Errors During File Copying
When copying files, it is important to handle potential errors, such as file not found or permission issues. You can manage these exceptions using a try-except block.
- Example:
“`python
import shutil
src_file = ‘path/to/source/file.txt’
dst_file = ‘path/to/destination/file.txt’
try:
shutil.copy(src_file, dst_file)
print(“File copied successfully.”)
except FileNotFoundError:
print(“Source file does not exist.”)
except PermissionError:
print(“Permission denied.”)
except Exception as e:
print(f”An error occurred: {e}”)
“`
This structure allows your program to respond gracefully to errors that may arise during the copying process.
Copying Directories
The `shutil` module also enables the copying of entire directories using the `shutil.copytree()` function.
- Basic Syntax:
“`python
shutil.copytree(src, dst)
“`
- Example:
“`python
import shutil
src_dir = ‘path/to/source/directory’
dst_dir = ‘path/to/destination/directory’
shutil.copytree(src_dir, dst_dir)
“`
This command copies all contents from the source directory to the destination directory, including subdirectories and files.
- Options:
The `copytree` function includes parameters to control the copy process:
- `dirs_exist_ok`: If set to `True`, it allows the destination directory to exist and merges the contents.
- Example with Option:
“`python
shutil.copytree(src_dir, dst_dir, dirs_exist_ok=True)
“`
This allows for more flexible directory copying.
Expert Insights on Copying Files in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When copying files in Python, utilizing the `shutil` module is highly recommended due to its simplicity and efficiency. This module provides a straightforward method to copy files and directories, making it an essential tool for developers.”
Mark Thompson (Python Developer Advocate, CodeCraft). “For anyone looking to copy files in Python, it’s crucial to handle exceptions properly. Implementing error handling ensures that your program can manage issues like file not found or permission errors gracefully, which is vital for robust applications.”
Linda Zhao (Data Scientist, Analytics Solutions). “In data processing tasks, copying files efficiently can save significant time. Using `shutil.copy2()` not only copies the file but also preserves its metadata, which is often important when dealing with datasets.”
Frequently Asked Questions (FAQs)
How can I copy a file in Python using the shutil module?
You can copy a file in Python using the `shutil` module by importing it and using the `shutil.copy()` function. For example:
“`python
import shutil
shutil.copy(‘source_file.txt’, ‘destination_file.txt’)
“`
Is there a way to copy a file without overwriting the existing file?
Yes, you can check if the destination file exists before copying. Use `os.path.exists()` to verify its presence and handle the situation accordingly.
Can I copy a file and preserve its metadata in Python?
Yes, to copy a file while preserving its metadata, use `shutil.copy2()` instead of `shutil.copy()`. This function maintains the original file’s metadata, including timestamps.
What is the difference between shutil.copy() and shutil.copyfile()?
`shutil.copy()` copies the file and preserves its metadata, while `shutil.copyfile()` only copies the file’s contents without preserving any metadata.
How do I copy a directory and its contents in Python?
To copy a directory and its contents, use `shutil.copytree()`. For example:
“`python
shutil.copytree(‘source_directory’, ‘destination_directory’)
“`
This function recursively copies all files and subdirectories.
Can I copy files across different file systems in Python?
Yes, Python’s `shutil` module allows you to copy files across different file systems without any issues, as long as the destination is accessible.
Copying a file in Python can be accomplished using several methods, with the most common approach being the use of the built-in `shutil` module. This module provides a straightforward function, `shutil.copy()`, which allows users to duplicate files with ease. The function not only copies the file’s contents but also preserves the file’s metadata, such as permissions and timestamps, if necessary. Understanding how to utilize this module effectively is essential for file management tasks in Python programming.
Another method for copying files involves using the `os` module, which provides lower-level file operations. While `os` can be used to read and write files, it requires more lines of code and does not inherently manage file metadata as efficiently as `shutil`. Therefore, for most use cases, especially when simplicity and efficiency are priorities, `shutil` is the recommended choice for copying files in Python.
In summary, the `shutil` module stands out as the most effective tool for copying files in Python due to its ease of use and ability to maintain file properties. Developers should familiarize themselves with this module to streamline their file handling processes. Additionally, understanding the differences between `shutil` and `os` can help programmers make informed decisions
Author Profile
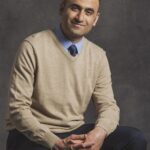
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?