How Can You Comment Out Multiple Lines in JavaScript?
### Introduction
In the dynamic world of JavaScript programming, clarity and organization are paramount. As developers, we often find ourselves needing to temporarily disable sections of code for testing or debugging purposes. This is where the art of commenting comes into play. While single-line comments are straightforward, the process of commenting out multiple lines can be slightly more nuanced. Mastering this technique not only enhances your code’s readability but also streamlines your workflow, allowing you to focus on what truly matters: writing effective and efficient code.
When working on complex projects, it’s common to encounter scenarios where you want to annotate or disable blocks of code without deleting them. This is particularly useful during collaborative efforts or when experimenting with different functionalities. Understanding how to comment out multiple lines in JavaScript can save you time and prevent potential errors, making it an essential skill for both novice and experienced developers alike.
In this article, we will explore the various methods available for commenting out multiple lines in JavaScript. From simple syntax to best practices, we’ll guide you through the nuances of this fundamental technique, ensuring you can keep your code neat and manageable. Whether you’re debugging an existing script or trying out new ideas, learning how to effectively comment out multiple lines will empower you to enhance your coding experience.
Commenting Out Multiple Lines in JavaScript
In JavaScript, there are a couple of effective methods to comment out multiple lines of code. Commenting is crucial for documentation and debugging, as it allows developers to explain their code or temporarily disable sections without deleting them.
The most common approach to comment out multiple lines is to use the block comment syntax. This is achieved by enclosing the desired code with `/*` at the beginning and `*/` at the end. Here’s an example:
javascript
/*
console.log(“This line will not execute.”);
console.log(“Neither will this line.”);
*/
Using block comments is beneficial for large sections of code, as it provides a clear and visually distinct way to indicate that the enclosed lines are not to be executed.
Another approach is to use single-line comments (`//`) for each line you wish to comment out. While this method can be more tedious, it can be useful in scenarios where you only want to disable specific lines within a larger block of code. Here’s how that looks:
javascript
// console.log(“This line will not execute.”);
// console.log(“This line will also be ignored.”);
### Comparison of Commenting Methods
Method | Syntax | Use Case |
---|---|---|
Block Comments | `/* … */` | Best for commenting out large blocks of code |
Single-line Comments | `//` | Useful for commenting out individual lines |
### Best Practices for Commenting
- Clarity: Use comments to clarify complex code. Avoid commenting on obvious lines.
- Consistency: Stick to one method of commenting in a given file to maintain readability.
- Documentation: Regularly update comments to reflect changes in the code, ensuring they remain relevant.
By employing these commenting techniques judiciously, developers can enhance the maintainability and readability of their codebases.
Commenting Out Multiple Lines in JavaScript
In JavaScript, there are primarily two ways to comment out lines of code: single-line comments and multi-line comments. Understanding how to effectively use these commenting methods can aid in debugging and improving code readability.
Using Multi-Line Comments
JavaScript supports multi-line comments, which allow developers to comment out blocks of code efficiently. This is done using the `/*` and `*/` syntax. Everything between these delimiters will be ignored by the JavaScript engine.
Example:
javascript
/*
console.log(“This line will not execute.”);
console.log(“Neither will this line.”);
*/
This method is particularly useful when you want to disable a block of code temporarily or add descriptive comments that span several lines.
Using Single-Line Comments
While single-line comments are primarily used for commenting out individual lines, they can also be used in conjunction to comment out multiple lines by placing `//` at the start of each line. However, this approach can be more cumbersome for larger blocks of code.
Example:
javascript
// console.log(“This line will not execute.”);
// console.log(“This line will also be ignored.”);
Best Practices for Commenting
When commenting out code, it’s essential to follow best practices to maintain code clarity and organization:
- Use Multi-Line Comments for Large Blocks: When dealing with extensive code sections, prefer multi-line comments to enhance readability.
- Single-Line Comments for Quick Annotations: Utilize single-line comments for short explanations or to disable a few lines.
- Avoid Commenting Out Production Code: Regularly commenting out code in production environments can lead to confusion. Instead, use version control systems to manage changes.
- Keep Comments Relevant: Ensure comments are up-to-date and relevant to the code they accompany to avoid misinformation.
Comment Type | Usage | Example |
---|---|---|
Multi-Line | Commenting out large blocks of code | /* Commented code here */ |
Single-Line | Commenting out individual lines or brief notes | // Commented line |
By adhering to these commenting techniques and practices, developers can maintain clean, understandable, and manageable codebases that facilitate easier debugging and collaboration.
Expert Insights on Commenting Out Multiple Lines in JavaScript
Jessica Tran (Senior JavaScript Developer, CodeCraft Inc.). “To effectively comment out multiple lines in JavaScript, developers can utilize the multi-line comment syntax, which involves wrapping the code within /* and */. This method enhances readability and keeps the code organized, especially during debugging.”
Michael Chen (Software Engineering Instructor, Tech University). “Understanding how to comment out multiple lines is crucial for maintaining code clarity. The /* comment */ syntax is not only efficient but also prevents potential errors during development by allowing developers to temporarily disable sections of code.”
Linda Patel (Lead Frontend Engineer, InnovateTech). “When working on larger projects, using multi-line comments can significantly streamline collaboration among team members. It is essential to ensure that comments are clear and concise, as they serve as documentation for others who may work with the code.”
Frequently Asked Questions (FAQs)
How do I comment out multiple lines in JavaScript?
You can comment out multiple lines in JavaScript by using the `/*` to start the comment and `*/` to end it. For example:
javascript
/*
This is a comment
spanning multiple lines
*/
Can I use single-line comments within a multi-line comment?
Yes, you can include single-line comments (`//`) within a multi-line comment. However, the single-line comments will not terminate the multi-line comment. They will be treated as part of the comment.
What happens if I forget to close a multi-line comment?
If you forget to close a multi-line comment, the JavaScript interpreter will treat all subsequent code as part of the comment, leading to syntax errors and preventing the script from executing properly.
Is there a shortcut in code editors for commenting multiple lines?
Most code editors and IDEs provide shortcuts for commenting multiple lines. For example, in Visual Studio Code, you can select the lines and use `Ctrl + /` (Windows) or `Cmd + /` (Mac) to toggle comments.
Are there performance implications of using comments in JavaScript?
Comments do not affect the performance of JavaScript execution as they are ignored by the interpreter. However, excessive commenting can make code harder to read and maintain.
Can I nest multi-line comments in JavaScript?
No, JavaScript does not support nested multi-line comments. If you attempt to nest them, it will lead to unexpected behavior and syntax errors. Use single-line comments for nested scenarios instead.
In JavaScript, commenting out multiple lines of code can be achieved using block comments. Block comments begin with `/*` and end with `*/`, allowing developers to encapsulate a section of code that they wish to disable or annotate. This method is particularly useful for temporarily removing large blocks of code during debugging or for adding detailed explanations without affecting the execution of the script.
Another approach to comment out multiple lines is to use single-line comments (`//`) for each line. However, this can be tedious and less efficient, especially when dealing with extensive code. Therefore, the block comment method is generally preferred for its simplicity and effectiveness in managing larger sections of code.
It is essential to remember that while comments are valuable for documentation and understanding code, excessive commenting can lead to clutter. Developers should strive for a balance, ensuring that comments enhance clarity without overwhelming the codebase. Proper commenting practices contribute to better maintainability and collaboration among team members.
Author Profile
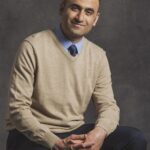
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?