How Can You Use SQL WHERE to Handle Multiple Conditions Effectively?
When working with databases, the ability to filter data effectively is crucial for extracting meaningful insights. One of the most powerful tools at your disposal in SQL is the `WHERE` clause, which allows you to specify conditions that must be met for records to be included in your results. But what happens when you need to apply multiple conditions? This is where the real magic of SQL shines, enabling you to perform complex queries that can sift through vast amounts of data with precision. Whether you’re a seasoned database administrator or a budding data analyst, mastering the art of crafting SQL queries with multiple conditions will elevate your data manipulation skills to new heights.
In SQL, the `WHERE` clause can be combined with logical operators such as `AND`, `OR`, and `NOT` to create intricate filtering criteria. These operators allow you to refine your queries by specifying exactly which records you want to retrieve based on multiple attributes. For instance, you might want to find all customers who live in a specific city and have made a purchase above a certain amount. By understanding how to structure these conditions effectively, you can ensure that your queries return only the most relevant results.
Moreover, the use of parentheses in complex conditions can greatly enhance the clarity and functionality of your SQL statements. By grouping conditions, you can dictate the
Combining Conditions with AND and OR
In SQL, multiple conditions can be combined in the `WHERE` clause using the logical operators `AND` and `OR`. Each operator plays a crucial role in filtering results based on specified criteria.
– **AND**: This operator is used when all conditions must be true for a record to be included in the result set.
– **OR**: This operator is used when at least one of the conditions must be true.
For instance, consider a table named `Employees` with the following columns: `EmployeeID`, `FirstName`, `LastName`, `Department`, and `Salary`.
To retrieve employees who work in the “Sales” department and earn more than $50,000, the query would look like this:
“`sql
SELECT *
FROM Employees
WHERE Department = ‘Sales’ AND Salary > 50000;
“`
Conversely, if you want to find employees who either work in the “Sales” department or earn more than $50,000, the query would be:
“`sql
SELECT *
FROM Employees
WHERE Department = ‘Sales’ OR Salary > 50000;
“`
Using Parentheses for Complex Conditions
When combining multiple `AND` and `OR` conditions, parentheses are essential to ensure that the logical operations are evaluated in the intended order. Without parentheses, SQL may interpret the conditions differently than expected.
For example, if you want to find employees who are either in the “Sales” department earning over $50,000 or in the “HR” department regardless of salary, the correct query would be:
“`sql
SELECT *
FROM Employees
WHERE (Department = ‘Sales’ AND Salary > 50000) OR Department = ‘HR’;
“`
This ensures that the condition for the “Sales” department is evaluated first before checking for the “HR” department.
Example with Multiple Conditions
Here’s a practical example illustrating the use of multiple conditions in a SQL query:
“`sql
SELECT *
FROM Employees
WHERE (Department = ‘Sales’ AND Salary > 50000)
OR (Department = ‘IT’ AND Salary < 70000);
```
In this example, the query retrieves employees who are either in the "Sales" department with salaries exceeding $50,000 or in the "IT" department with salaries below $70,000.
Table of SQL Logical Operators
Below is a summary table of the logical operators used in SQL:
Operator | Description | Example |
---|---|---|
AND | Returns true if both conditions are true. | WHERE condition1 AND condition2 |
OR | Returns true if at least one condition is true. | WHERE condition1 OR condition2 |
NOT | Reverses the result of a condition. | WHERE NOT condition |
Understanding how to combine multiple conditions effectively can significantly enhance your ability to query databases and retrieve precise information tailored to specific requirements.
Using WHERE Clause with Multiple Conditions
The `WHERE` clause in SQL is essential for filtering records based on specific conditions. When you need to apply multiple conditions, you can use logical operators such as `AND`, `OR`, and `NOT` to create complex queries.
Logical Operators
- AND: Ensures that all specified conditions must be true for a record to be included in the result set.
- OR: At least one of the specified conditions must be true for a record to be included.
- NOT: Excludes records that meet the specified condition.
Combining Conditions
You can combine conditions using the following structure:
“`sql
SELECT column1, column2
FROM table_name
WHERE condition1
AND condition2
OR condition3;
“`
To illustrate, consider a table named `Employees` with columns `Name`, `Age`, and `Department`. Here are examples of queries using multiple conditions:
Examples
- **Using AND:**
“`sql
SELECT Name
FROM Employees
WHERE Age > 30
AND Department = ‘Sales’;
“`
This query retrieves names of employees who are older than 30 and work in the Sales department.
- Using OR:
“`sql
SELECT Name
FROM Employees
WHERE Department = ‘HR’
OR Department = ‘IT’;
“`
This query fetches names of employees who work either in the HR or IT departments.
- Using NOT:
“`sql
SELECT Name
FROM Employees
WHERE NOT Department = ‘Marketing’;
“`
This retrieves names of all employees who do not work in the Marketing department.
Grouping Conditions
When combining `AND` and `OR`, it is crucial to use parentheses to control the order of evaluation:
“`sql
SELECT Name
FROM Employees
WHERE (Age > 30 AND Department = ‘Sales’)
OR (Age < 25 AND Department = 'IT');
```
In this example, the query retrieves employees who are either older than 30 in Sales or younger than 25 in IT.
Example with Multiple Conditions
For a more complex scenario, consider a query that filters employees based on multiple attributes:
“`sql
SELECT Name, Age, Department
FROM Employees
WHERE (Age > 30 AND Department = ‘Sales’)
OR (Age < 25 AND Department = 'IT')
AND NOT Name LIKE 'A%';
```
This query fetches employees who are either older than 30 in Sales or younger than 25 in IT, excluding those whose names start with 'A'.
Best Practices
- Use parentheses to clarify the logic of your conditions.
- Avoid excessive complexity in conditions to improve readability.
- Test queries incrementally to ensure each condition behaves as expected.
Utilizing multiple conditions in the `WHERE` clause allows for detailed data retrieval, enhancing the power and flexibility of SQL queries.
Expert Insights on Using SQL WHERE with Multiple Conditions
Maria Chen (Data Analyst, Tech Innovations Inc.). “When constructing SQL queries with multiple conditions in the WHERE clause, it is crucial to understand the logical operators involved. Using AND and OR correctly can significantly affect the outcome of your query, ensuring that you retrieve precisely the data you need.”
James Patel (Database Administrator, Cloud Solutions Group). “To optimize performance when using multiple conditions in SQL WHERE clauses, consider indexing the columns involved. This practice can reduce query execution time and improve overall database efficiency, especially with large datasets.”
Linda Gomez (SQL Developer, Data Insights LLC). “Always prioritize clarity when writing SQL statements with multiple conditions. Utilizing parentheses to group conditions can help avoid ambiguity and ensure that the query logic aligns with your intended data retrieval strategy.”
Frequently Asked Questions (FAQs)
What is the purpose of using multiple conditions in a SQL WHERE clause?
Using multiple conditions in a SQL WHERE clause allows for more precise filtering of records based on multiple criteria. This enables users to retrieve data that meets specific requirements, enhancing data analysis and reporting.
How do I combine multiple conditions in a SQL WHERE clause?
Multiple conditions can be combined using logical operators such as AND and OR. The AND operator requires all conditions to be true for a record to be included, while the OR operator includes records that meet at least one of the conditions.
Can I use parentheses in a SQL WHERE clause with multiple conditions?
Yes, parentheses can be used to group conditions and control the order of evaluation. This is particularly useful when combining AND and OR operators, ensuring that the intended logic is applied correctly.
What is the difference between using AND and OR in a SQL WHERE clause?
The AND operator returns records only when all specified conditions are true, while the OR operator returns records if any of the conditions are true. This fundamental difference affects the result set significantly.
Are there any limitations to the number of conditions I can use in a SQL WHERE clause?
Most SQL databases do not impose a strict limit on the number of conditions in a WHERE clause. However, excessive complexity can impact performance and readability, so it’s advisable to keep conditions manageable.
How can I optimize queries with multiple conditions in a SQL WHERE clause?
To optimize queries, ensure that indexed columns are used in conditions, avoid unnecessary complexity, and consider using subqueries or temporary tables for large datasets. Additionally, analyze execution plans to identify potential bottlenecks.
In SQL, the WHERE clause is a fundamental component used to filter records based on specific conditions. When dealing with multiple conditions, it is essential to understand how to combine these conditions effectively to retrieve the desired results. The most common logical operators used for this purpose are AND, OR, and NOT. Each operator serves a distinct function in refining the query results, allowing for precise data manipulation and retrieval.
Using the AND operator, all specified conditions must be true for a record to be included in the results. Conversely, the OR operator allows for flexibility, where if any one of the conditions is true, the record will be returned. The NOT operator can be utilized to exclude records that meet certain criteria. It is also important to use parentheses to group conditions appropriately, ensuring that the SQL engine evaluates them in the intended order, especially when mixing AND and OR operators.
When constructing SQL queries with multiple conditions, performance considerations should also be taken into account. Complex queries can lead to longer execution times, particularly with large datasets. Therefore, optimizing queries by indexing relevant columns and minimizing the use of wildcard characters can enhance performance. Additionally, understanding the data structure and relationships within the database can lead to more efficient query design.
In summary
Author Profile
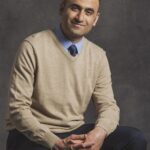
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?