How Can You Effectively Merge Two Objects in JavaScript?
In the ever-evolving landscape of JavaScript programming, the ability to manipulate and combine data structures is paramount. Among these data structures, objects stand out as powerful tools for organizing and managing complex information. Whether you’re building a dynamic web application or simply looking to streamline your code, knowing how to merge two objects can significantly enhance your efficiency and effectiveness as a developer. This skill not only simplifies data handling but also opens up a world of possibilities for creating more sophisticated and responsive applications.
Merging objects in JavaScript involves combining properties from two or more objects into a single cohesive entity. This process can be particularly useful when dealing with configurations, settings, or any scenario where you need to consolidate information without losing any critical data. JavaScript offers several methods to achieve this, each with its own nuances and use cases. From the traditional approach using loops to modern techniques leveraging built-in methods, understanding these options will empower you to choose the best solution for your specific needs.
As you delve deeper into the topic, you’ll discover the intricacies of object merging, including how to handle property conflicts and the implications of shallow versus deep merging. With practical examples and clear explanations, you’ll be well-equipped to implement these techniques in your own projects, enhancing both your coding skills and the functionality of your applications.
Using Object.assign()
Object.assign() is a built-in JavaScript method that allows you to copy the values of all enumerable properties from one or more source objects to a target object. This method is particularly useful for merging objects, as it combines the properties of multiple objects into a single object.
Here is the syntax for Object.assign():
javascript
Object.assign(target, …sources);
- target: The object to which properties will be copied.
- sources: One or more objects from which properties will be copied.
Example of merging two objects using Object.assign():
javascript
const obj1 = { a: 1, b: 2 };
const obj2 = { b: 3, c: 4 };
const merged = Object.assign({}, obj1, obj2);
console.log(merged); // { a: 1, b: 3, c: 4 }
In this example, the property `b` is overwritten by the value from `obj2`, demonstrating that later sources take precedence over earlier ones.
Using the Spread Operator
The spread operator (`…`) provides a concise syntax for merging objects. It allows for more readable and simpler code when combining multiple object properties.
Here is how you can use the spread operator to merge two objects:
javascript
const obj1 = { a: 1, b: 2 };
const obj2 = { b: 3, c: 4 };
const merged = { …obj1, …obj2 };
console.log(merged); // { a: 1, b: 3, c: 4 }
Similar to Object.assign(), the spread operator will overwrite properties from earlier objects with those from later objects.
Handling Nested Objects
When merging objects that contain nested properties, both Object.assign() and the spread operator perform a shallow merge. This means that if you have nested objects, only the first level of properties will be merged. To achieve a deep merge, you will need to implement a custom function or use a library like Lodash.
Example of shallow merge:
javascript
const obj1 = { a: 1, nested: { b: 2 } };
const obj2 = { nested: { c: 3 } };
const merged = { …obj1, …obj2 };
console.log(merged); // { a: 1, nested: { c: 3 } }
In this case, the `nested` property from `obj2` has completely replaced the `nested` property from `obj1`.
Using Lodash for Deep Merging
Lodash is a popular utility library that provides a wide range of functions, including a deep merge function. You can utilize the `_.merge()` method for merging objects deeply.
Example of deep merging with Lodash:
javascript
const _ = require(‘lodash’);
const obj1 = { a: 1, nested: { b: 2 } };
const obj2 = { nested: { c: 3 } };
const merged = _.merge({}, obj1, obj2);
console.log(merged); // { a: 1, nested: { b: 2, c: 3 } }
This effectively combines the properties of both objects without losing any data from the nested structure.
Comparison Table of Merging Methods
Method | Shallow Merge | Deep Merge | Overwrite Behavior |
---|---|---|---|
Object.assign() | Yes | No | Later sources overwrite earlier ones |
Spread Operator | Yes | No | Later sources overwrite earlier ones |
Lodash _.merge() | No | Yes | Combines properties |
This table summarizes the key differences between the various methods for merging objects in JavaScript, helping developers choose the most appropriate technique based on their specific needs.
Merging Two Objects in JavaScript
Merging two objects in JavaScript can be achieved using several methods. The most commonly used techniques include the spread operator, `Object.assign()`, and libraries like Lodash. Each method has its own advantages and can be chosen based on the specific requirements of your project.
Using the Spread Operator
The spread operator (`…`) is a modern and concise way to merge objects. It allows for a clean syntax and is widely supported in ES6 and later versions.
javascript
const obj1 = { a: 1, b: 2 };
const obj2 = { b: 3, c: 4 };
const merged = { …obj1, …obj2 };
console.log(merged); // Output: { a: 1, b: 3, c: 4 }
- The properties of `obj2` will overwrite those of `obj1` in case of a conflict.
- This method creates a shallow copy of the objects, meaning nested objects are not cloned but referenced.
Using Object.assign()
`Object.assign()` is another method that merges objects by copying the values of all enumerable own properties from source objects to a target object.
javascript
const obj1 = { a: 1, b: 2 };
const obj2 = { b: 3, c: 4 };
const merged = Object.assign({}, obj1, obj2);
console.log(merged); // Output: { a: 1, b: 3, c: 4 }
- The first argument is the target object (which is empty in this case).
- Similar to the spread operator, properties from later objects overwrite those from earlier ones.
- This method also performs a shallow merge.
Using Lodash for Deep Merging
For scenarios that require deep merging of objects—where nested objects should be merged rather than overwritten—Lodash provides a convenient utility function called `_.merge()`.
javascript
const _ = require(‘lodash’);
const obj1 = { a: { b: 2 }, c: 4 };
const obj2 = { a: { d: 3 }, e: 5 };
const merged = _.merge({}, obj1, obj2);
console.log(merged); // Output: { a: { b: 2, d: 3 }, c: 4, e: 5 }
- Lodash’s `merge` function recursively merges properties.
- It is essential for scenarios where nested structures need to be preserved and merged correctly.
Considerations
When merging objects, consider the following:
- Shallow vs. Deep Merging:
- Use the spread operator or `Object.assign()` for shallow merges.
- Use Lodash or a custom function for deep merges.
- Property Overwriting:
- Be aware that properties in later objects will overwrite properties in earlier ones.
- Performance:
- For large objects or in performance-critical applications, evaluate the method used as some may have overhead.
In summary, depending on the complexity of the objects being merged and your specific needs, you can choose from the spread operator, `Object.assign()`, or a library like Lodash to achieve the desired result effectively.
Expert Insights on Merging Objects in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When merging two objects in JavaScript, the spread operator is one of the most efficient methods. It allows for a clean and concise syntax, making the code more readable and maintainable. For example, using `{…obj1, …obj2}` merges the properties of both objects, with `obj2` properties overwriting those in `obj1` in case of conflicts.”
James Thompson (JavaScript Framework Specialist, CodeCraft Academy). “Using `Object.assign()` is another robust approach to merging objects. This method is particularly useful when you need to merge multiple objects into a target object. However, developers should be cautious about the fact that it performs a shallow copy, which can lead to unintended side effects if the objects contain nested structures.”
Linda Martinez (Front-End Developer, Web Solutions Group). “For those working with immutable data structures, consider libraries like Lodash or Immer. These libraries provide powerful utilities for merging objects without mutating the original data, which is essential in functional programming paradigms. Using `_.merge()` from Lodash is a great way to perform deep merges, ensuring that nested objects are combined correctly.”
Frequently Asked Questions (FAQs)
How can I merge two objects in JavaScript?
You can merge two objects in JavaScript using the `Object.assign()` method or the spread operator (`…`). For example, `Object.assign({}, obj1, obj2)` or `{…obj1, …obj2}` will create a new object that combines the properties of both `obj1` and `obj2`.
What happens if there are duplicate properties when merging objects?
When merging objects with duplicate properties, the values from the second object will overwrite the values from the first object. This behavior applies to both `Object.assign()` and the spread operator.
Can I merge more than two objects at once?
Yes, you can merge multiple objects at once using both `Object.assign()` and the spread operator. For instance, `Object.assign({}, obj1, obj2, obj3)` or `{…obj1, …obj2, …obj3}` will combine all specified objects into a new object.
Is it possible to merge nested objects in JavaScript?
The default methods (`Object.assign()` and the spread operator) perform a shallow merge, which means nested objects will not be deeply merged. For deep merging, you may need to use a library like Lodash with its `_.merge()` method or implement a custom deep merge function.
What are some performance considerations when merging large objects?
Merging large objects can impact performance, particularly with deep merges or when using libraries. It’s advisable to minimize the size of objects being merged and to use efficient algorithms for deep merging to optimize performance.
Are there any libraries that simplify object merging in JavaScript?
Yes, libraries such as Lodash and Ramda provide utility functions that simplify object merging. Lodash’s `_.merge()` and Ramda’s `R.merge()` can handle both shallow and deep merges, offering more flexibility than native methods.
Merging two objects in JavaScript is a common task that can be accomplished using various methods. The most popular approaches include using the Object.assign() method, the spread operator, and utility libraries like Lodash. Each method has its own advantages and use cases, depending on the specific requirements of the task at hand.
The Object.assign() method allows for shallow copying of properties from source objects to a target object. This method is straightforward and works well for simple object merging. However, it does not handle deep merging, which can be a limitation when dealing with nested objects.
On the other hand, the spread operator provides a more concise syntax for merging objects and is often preferred for its readability. It also performs shallow merging, similar to Object.assign(). For deep merging, developers may turn to libraries like Lodash, which offer more robust solutions that can handle nested structures effectively.
Overall, understanding the nuances of each method is essential for selecting the appropriate approach to merge objects in JavaScript. By leveraging these techniques, developers can effectively manage and manipulate object data within their applications, enhancing code efficiency and maintainability.
Author Profile
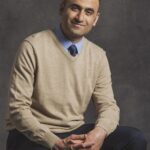
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?