Why Am I Seeing ‘String Was Not Recognized as a Valid DateTime’? Understanding and Fixing This Common Error
In the realm of programming and data management, working with dates and times is a fundamental yet often frustrating task. One common error that developers encounter is the dreaded message: “string was not recognized as a valid datetime.” This seemingly innocuous phrase can halt progress and lead to hours of debugging as developers sift through their code, trying to pinpoint the source of the problem. Understanding the intricacies of date and time formats is essential for anyone looking to build robust applications, and this article aims to demystify the issue, providing insights and solutions to navigate these challenges effectively.
When dealing with date and time data, the format in which strings are presented can vary significantly across different cultures, programming languages, and systems. This variability can lead to confusion and errors, particularly when a string representation of a date does not align with the expected format of the programming environment. The error message serves as a reminder of the importance of adhering to specific formats and standards, which can vary from one context to another.
Moreover, the implications of this error extend beyond mere annoyance; they can impact data integrity, user experience, and application functionality. By exploring the underlying causes of this error and understanding best practices for date and time handling, developers can enhance their coding skills and create more reliable software
Common Causes of the Error
The error message “string was not recognized as a valid datetime” typically arises when attempting to convert a string representation of a date and time into a DateTime object in programming environments such as Cor .NET. The underlying issues can often be traced back to several common causes:
- Incorrect Format: The date string does not match the expected format. For example, if the expected format is `MM/dd/yyyy` but the input is `dd-MM-yyyy`, the conversion will fail.
- Culture Settings: The application may be using a specific culture that interprets date formats differently. For instance, the U.S. uses `MM/dd/yyyy`, while many European countries use `dd/MM/yyyy`.
- Invalid Characters: The presence of non-numeric characters or extraneous spaces can invalidate the string.
- Null or Empty Strings: Attempting to convert an empty string or a null value can also lead to this error.
Handling DateTime Conversion
To effectively handle DateTime conversions and mitigate errors, consider implementing the following strategies:
- Use TryParse Methods: Instead of directly parsing, utilize methods such as `DateTime.TryParse()` or `DateTime.TryParseExact()` which do not throw exceptions but return a boolean indicating success or failure.
- Specify Format and Culture: When using `DateTime.ParseExact()`, explicitly define the expected format and culture settings to ensure proper interpretation of the string.
- Validate Input: Always validate the input string before attempting to parse. This includes checking for nulls, length, and unwanted characters.
- Use Exception Handling: Implement try-catch blocks to gracefully handle potential exceptions during parsing.
Here’s an example of how to implement these strategies in C:
“`csharp
string dateString = “12/31/2023”;
DateTime parsedDate;
if (DateTime.TryParse(dateString, out parsedDate))
{
Console.WriteLine(“Parsed date: ” + parsedDate);
}
else
{
Console.WriteLine(“Unable to parse the date.”);
}
“`
Best Practices for DateTime Management
To avoid common pitfalls associated with DateTime handling, adhere to these best practices:
- Consistent Formatting: Stick to a consistent date format throughout your application to minimize confusion.
- Use UTC: Store dates in Coordinated Universal Time (UTC) to avoid timezone-related issues.
- Leverage Date Libraries: Utilize robust date libraries that handle various formats and locales effectively.
Error Cause | Solution |
---|---|
Incorrect Format | Use DateTime.ParseExact with the correct format string. |
Culture Settings | Specify culture in parsing methods. |
Invalid Characters | Sanitize input strings before parsing. |
Null or Empty Strings | Check for null or empty before parsing. |
Understanding the Error
The error message “string was not recognized as a valid DateTime” typically occurs in programming environments when an attempt is made to convert a string representation of a date and time into a DateTime object, but the format of the string does not match the expected format. This can happen in various programming languages, including C, Python, and Java.
Common Causes
Several factors can lead to this error:
- Incorrect Format: The string does not follow the expected date format. For example, “2023-31-12” is not valid if the expected format is “MM/dd/yyyy”.
- Culture Inconsistencies: Different cultures have different date formats. For instance, “dd/MM/yyyy” is common in many regions, while “MM/dd/yyyy” is standard in the United States.
- Invalid Date Values: The string contains impossible dates, such as “February 30”.
- Leading/Trailing Whitespaces: Extra spaces in the string can cause parsing issues.
- Null or Empty Strings: Attempting to parse a null or empty string will also trigger this error.
Best Practices for Handling DateTime Conversion
To avoid encountering this error, consider the following best practices:
- Use TryParse Methods: Many programming languages offer methods like `DateTime.TryParse` in Cthat return a boolean indicating success or failure, preventing exceptions.
- Specify Format: When parsing, explicitly define the expected format to ensure the string is correctly interpreted.
“`csharp
string dateString = “2023-12-31”;
DateTime parsedDate;
if (DateTime.TryParseExact(dateString, “yyyy-MM-dd”, CultureInfo.InvariantCulture, DateTimeStyles.None, out parsedDate))
{
// Successfully parsed
}
else
{
// Handle parsing error
}
“`
- Culture Awareness: Be aware of the cultural context when dealing with dates. Utilize culture-specific parsing methods when necessary.
Debugging Steps
When troubleshooting this error, follow these steps:
- Inspect the Input String: Check for formatting issues, invalid dates, or unexpected characters.
- Log the Input: If the error occurs at runtime, log the string being parsed to identify the exact cause.
- Validate Before Parsing: Implement validation logic to check if the string is in the correct format before attempting to parse it.
- Use Exception Handling: Wrap parsing logic in try-catch blocks to gracefully handle errors and provide user feedback.
Example Scenarios
Here are a few scenarios that illustrate the error and solutions:
Scenario | Input String | Expected Output | Error Type |
---|---|---|---|
Correct date format | “2023-12-31” | Valid DateTime | None |
Incorrect date format | “31/12/2023” | Exception | Format mismatch |
Invalid date | “February 30, 2023” | Exception | Invalid date |
Leading/trailing whitespace | ” 2023-12-31 “ | Exception | Whitespace issue |
Null or empty string | “” | Exception | Null or empty string |
By following these guidelines and understanding the common pitfalls associated with DateTime parsing, developers can effectively mitigate the risk of encountering the “string was not recognized as a valid DateTime” error in their applications.
Understanding the “String Was Not Recognized as a Valid DateTime” Error
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘string was not recognized as a valid datetime’ typically arises when the input string does not conform to the expected date format. It is crucial for developers to ensure that the date strings being parsed match the format specified in the application settings or the system’s culture settings.”
Michael Thompson (Data Scientist, Analytics Solutions Group). “In data processing, this error can lead to significant disruptions. It is essential to implement robust validation checks to ensure that all date inputs are sanitized and formatted correctly before attempting to parse them into datetime objects.”
Linda Patel (Lead Developer, Software Quality Assurance Experts). “To resolve the ‘string was not recognized as a valid datetime’ error, developers should utilize try-catch blocks to gracefully handle exceptions. Additionally, employing libraries that provide flexible date parsing options can help mitigate this issue in diverse applications.”
Frequently Asked Questions (FAQs)
What does the error “string was not recognized as a valid datetime” mean?
This error indicates that a string being parsed into a DateTime object does not conform to the expected date and time format, making it impossible for the system to interpret it as a valid date.
What are common causes of this error?
Common causes include incorrect date formats, invalid date values (such as February 30), and cultural differences in date representation (e.g., MM/DD/YYYY vs. DD/MM/YYYY).
How can I resolve this error in my code?
To resolve the error, ensure that the string being parsed matches the expected format. Use the appropriate DateTime parsing methods and specify the format explicitly if necessary.
Are there specific formats I should use to avoid this error?
Yes, using standardized formats such as ISO 8601 (YYYY-MM-DDTHH:MM:SS) is recommended. Additionally, always verify the format against the culture settings of the application.
Can this error occur due to localization settings?
Yes, localization settings can affect date parsing. If the application’s culture settings differ from the date format provided, it may lead to this error.
What debugging steps can I take if I encounter this error?
Debugging steps include logging the string being parsed, checking the expected format, and using try-catch blocks to handle exceptions gracefully. Additionally, consider testing with known valid date strings.
The error message “string was not recognized as a valid datetime” typically arises in programming environments when a string intended to represent a date or time fails to conform to the expected format. This issue is prevalent in languages such as C, Python, and Java, where date parsing functions are sensitive to the format of the input string. The underlying cause often relates to discrepancies between the string format and the expected date format, which can vary based on culture, locale settings, or specific application requirements.
To address this error, it is essential to ensure that the string being parsed adheres to the correct date format. Developers should utilize appropriate parsing methods that specify the expected format explicitly, such as using format specifiers in Cor date format strings in Python. Additionally, implementing error handling mechanisms can help gracefully manage instances where the input string does not match the expected format, providing users with informative feedback and preventing application crashes.
Moreover, understanding the context in which the date string is generated can aid in mitigating this issue. For instance, if the date string originates from user input, it may be beneficial to validate the input format before attempting to parse it. Furthermore, leveraging libraries or frameworks that handle date and time operations can simplify the process and reduce the likelihood
Author Profile
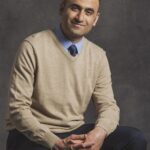
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?