How Can You Effectively Save Code in Python?
In the world of programming, the ability to save your work efficiently is as crucial as writing clean, functional code. For Python developers, understanding the various methods of saving code can streamline your workflow, enhance collaboration, and safeguard your projects against data loss. Whether you’re a novice just starting your coding journey or a seasoned developer looking to refine your skills, mastering the art of saving code in Python is essential for effective software development.
Saving code in Python involves more than just hitting the “save” button in your text editor. It encompasses a range of practices, from choosing the right file format to utilizing version control systems that track changes over time. As you delve deeper into the topic, you’ll discover how different environments—such as local machines, cloud services, and integrated development environments (IDEs)—offer unique features that can optimize your coding experience.
Moreover, understanding the importance of organizing your code files and maintaining a clear directory structure can significantly impact your productivity. By following best practices for code saving, you can ensure that your projects remain accessible, manageable, and ready for future enhancements. Join us as we explore the essential techniques and tools that will elevate your coding journey and help you save your Python code effectively.
Saving Code in Python Files
To save your Python code, you need to write it in a text editor or an Integrated Development Environment (IDE) and then save it with a `.py` file extension. This allows the Python interpreter to recognize the file as containing Python code. Here are steps to save your code:
- Choose an Editor or IDE: You can use simple text editors like Notepad (Windows) or TextEdit (Mac) for basic tasks, or opt for IDEs like PyCharm, VSCode, or Jupyter Notebook for more advanced features.
- Write Your Code: Enter your Python code in the chosen editor. Ensure that it adheres to Python’s syntax rules.
- Save the File:
- In most editors, you can use the shortcut `Ctrl + S` (or `Cmd + S` on Mac).
- Alternatively, navigate to the file menu and select “Save” or “Save As”.
- When prompted, specify the file name with the `.py` extension (e.g., `my_script.py`).
Saving Code in Jupyter Notebooks
For those using Jupyter Notebooks, saving code is slightly different due to the notebook format. Jupyter allows you to save your work in a notebook file with the `.ipynb` extension. Follow these steps:
- Create a Notebook: Launch Jupyter and create a new notebook.
- Enter Code in Cells: Write your Python code in the code cells.
- Saving the Notebook:
- Click on the “Save” icon in the toolbar or use the shortcut `Ctrl + S` (or `Cmd + S` on Mac).
- The notebook will automatically save in the current directory with a `.ipynb` extension.
Version Control for Python Code
Using version control systems like Git is highly recommended for managing changes to your Python code over time. Here are some key benefits:
- Track Changes: Monitor modifications and revert to previous versions as needed.
- Collaboration: Work seamlessly with other developers.
- Branching: Experiment with new features without affecting the main codebase.
To implement version control, follow these steps:
- Initialize a Git Repository: Open your terminal or command prompt in the directory of your Python project and run:
“`bash
git init
“`
- Stage Your Code: Add files to the staging area:
“`bash
git add my_script.py
“`
- Commit Changes: Save the staged changes:
“`bash
git commit -m “Initial commit”
“`
File Management and Organization
Organizing your Python files can greatly improve your workflow. Use the following practices:
- Directory Structure: Arrange files in directories based on functionality or modules.
- Naming Conventions: Use descriptive names for files and directories to clarify their purpose.
Here is a simple example of a directory structure:
Directory | Description |
---|---|
my_project/ | Main project directory |
src/ | Source code files |
tests/ | Test files for unit testing |
docs/ | Documentation files |
By following these practices, you can efficiently save and manage your Python code, making it easier to maintain and collaborate on projects.
Saving Code in Python
To save code in Python, you typically write your code in a text editor or integrated development environment (IDE) and then save it as a file with a `.py` extension. This allows Python to recognize the file as a script that can be executed. Below are various methods and best practices for saving your Python code effectively.
Using a Text Editor
- Select a Text Editor: Choose a text editor like Notepad, Sublime Text, or VSCode.
- Write Your Code: Enter your Python code into the editor.
- Save the File:
- Use the `File` menu and select `Save As`.
- Name your file with a `.py` extension (e.g., `my_script.py`).
- Choose the appropriate location on your computer.
- Click `Save`.
Using an Integrated Development Environment (IDE)
An IDE provides a more comprehensive environment for coding, debugging, and saving files. Examples include PyCharm, Jupyter Notebook, and Spyder.
- Open the IDE: Launch your preferred IDE.
- Create a New File:
- For most IDEs, you can find an option in the `File` menu to create a new file.
- Write Your Code: Input your code in the new file window.
- Save the File:
- Use the `File` menu and select `Save As`.
- Enter a name with the `.py` extension.
- Specify the directory where you want to save it.
- Click `Save`.
Saving in Jupyter Notebook
In Jupyter Notebook, code is saved within cells. The process differs slightly:
- Create a New Notebook: Launch Jupyter and create a new notebook.
- Write Your Code: Enter your code into the cells.
- Save Your Work:
- Click on the disk icon or use the shortcut `Ctrl + S` (or `Cmd + S` on Mac).
- The notebook is saved with a `.ipynb` extension, preserving both code and outputs.
Best Practices for Saving Code
- Version Control: Utilize tools like Git to manage changes and maintain versions of your code.
- Organized Directory Structure: Create a well-structured folder system for your projects to avoid confusion.
- Descriptive Filenames: Use meaningful filenames that describe the code’s purpose (e.g., `data_analysis.py`).
- Documentation: Include comments and documentation within your code files to make them easier to understand for future reference.
Saving Code with Command Line
You can also create and save Python files using the command line:
- Open Command Line Interface: Access your terminal (Linux/Mac) or Command Prompt (Windows).
- Navigate to Desired Directory: Use the `cd` command to change directories.
- Create a New Python File: Use a text editor command like `nano` or `vim`.
- For example, `nano my_script.py`.
- Write Your Code: Enter your Python code in the editor.
- Save and Exit:
- In `nano`, press `Ctrl + X`, then `Y` to confirm saving, and `Enter` to exit.
By following these methods and practices, you can efficiently save your Python code while ensuring it’s organized and easy to access.
Expert Insights on Saving Code in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively save code in Python, it is crucial to utilize version control systems such as Git. This not only allows for easy tracking of changes but also facilitates collaboration among team members.”
James Liu (Python Developer Advocate, CodeCraft Academy). “Using Integrated Development Environments (IDEs) like PyCharm or Visual Studio Code can significantly enhance your coding experience. These tools provide built-in features for saving and managing your code efficiently.”
Sarah Thompson (Data Scientist, Analytics Solutions Group). “It’s essential to regularly save your work, especially when dealing with large datasets or complex algorithms. Implementing auto-save features in your coding environment can help prevent data loss.”
Frequently Asked Questions (FAQs)
How do I save a Python script in a text file?
You can save a Python script by opening a text editor, writing your code, and then saving the file with a `.py` extension. For example, use “my_script.py” as the filename.
What is the recommended way to save code in an IDE?
Most Integrated Development Environments (IDEs) allow you to save your code using the “File” menu. You can typically use the shortcut `Ctrl + S` (Windows) or `Cmd + S` (Mac) to save your work quickly.
Can I save Python code directly from the command line?
Yes, you can save Python code directly from the command line using a text editor like `nano` or `vim`. For example, type `nano my_script.py`, write your code, and then save and exit the editor.
How do I save code changes in Jupyter Notebook?
In Jupyter Notebook, you can save your changes by clicking the “Save” icon (a floppy disk) or by using the keyboard shortcut `Ctrl + S`. This will save the entire notebook.
Is it possible to save Python code to a specific directory?
Yes, you can specify the directory when saving your Python file. For example, use `open(‘/path/to/directory/my_script.py’, ‘w’)` in your code to save it to a specific location.
What should I do if I forget to save my Python code?
If you forget to save your code, check if your editor or IDE has an autosave feature. If not, you may need to rewrite the code unless you have a backup or version control system in place.
In Python, saving code is a fundamental aspect of programming that allows developers to preserve their work and share it with others. The most common method of saving code is through the use of text editors or integrated development environments (IDEs) where the code can be written and saved as a `.py` file. This file format is recognized by Python interpreters, enabling the execution of the code on any compatible system. Additionally, version control systems like Git can be employed to manage changes and collaborate on code more effectively.
Another important consideration when saving code is the organization of files and directories. Structuring projects in a logical manner enhances maintainability and readability. Using descriptive names for files and folders, along with clear documentation within the code itself, contributes to better understanding and usability, especially in collaborative environments. Furthermore, utilizing virtual environments can help manage dependencies and avoid conflicts between different projects.
Lastly, backing up code is crucial to prevent data loss. Developers should consider using cloud storage solutions or external drives to ensure their work is secure. Regularly committing code to a version control system not only serves as a backup but also allows for tracking changes over time. By following these practices, programmers can effectively save their code in Python, ensuring it is organized,
Author Profile
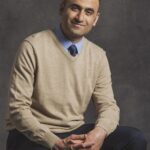
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?