How Can You Effectively Use the Merge Raster Function in Python?
### Introduction
In the realm of geospatial analysis, the ability to manipulate and combine raster datasets is a vital skill for researchers, environmental scientists, and data analysts alike. The `merge raster` function in Python offers a powerful tool for those looking to integrate multiple raster files into a single cohesive dataset. Whether you’re working with satellite imagery, elevation data, or land cover maps, mastering this function can significantly enhance your data processing capabilities. In this article, we will explore the intricacies of using the `merge raster` function, unlocking its potential to streamline your geospatial projects and improve the accuracy of your analyses.
Merging raster data is not just a technical task; it’s a crucial step in creating comprehensive datasets that can reveal insights otherwise hidden in isolated files. By combining multiple rasters, you can create a seamless representation of your area of interest, facilitating better visualization and analysis. Python, with its rich ecosystem of libraries such as Rasterio and GDAL, provides an accessible and efficient way to perform this operation, allowing users to handle large datasets with ease.
As we delve deeper into the topic, we will cover the fundamental concepts behind raster merging, the necessary libraries, and practical examples that illustrate the process. Whether you’re a seasoned programmer or just starting your journey in geospatial analysis,
Understanding the Merge Raster Function
The merge raster function is essential for combining multiple raster datasets into a single raster file. This capability is particularly useful in geographic information systems (GIS) and remote sensing applications, where data from different sources or areas need to be integrated for analysis.
Using the Merge Function with Rasterio
Rasterio is a powerful library in Python for reading and writing raster data. To use the merge function in Rasterio, follow these steps:
- Install Rasterio: Ensure you have Rasterio installed in your Python environment. You can install it using pip:
pip install rasterio
- Import the Required Libraries: You will need to import Rasterio and the merge function from the Rasterio library.
python
import rasterio
from rasterio.merge import merge
- Read the Raster Files: Open the raster files you want to merge. You can use `rasterio.open()` to read them into a list.
python
src_files_to_mosaic = [rasterio.open(file) for file in [‘raster1.tif’, ‘raster2.tif’]]
- Merge the Rasters: Call the `merge` function, which takes the list of opened raster files and returns a single merged raster dataset.
python
mosaic, out_trans = merge(src_files_to_mosaic)
- Save the Merged Raster: Finally, write the merged raster to a new file using `rasterio.open()` in write mode.
python
with rasterio.open(‘merged_raster.tif’, ‘w’, driver=’GTiff’,
height=mosaic.shape[1], width=mosaic.shape[2],
count=1, dtype=mosaic.dtype,
crs=src_files_to_mosaic[0].crs,
transform=out_trans) as dst:
dst.write(mosaic, 1)
Parameters and Options
When using the merge function, there are several parameters to consider:
- src_files: A list of opened raster files to merge.
- method: The resampling method to use. Options include `’nearest’`, `’bilinear’`, and `’cubic’`.
- nodata: A value that indicates no data in the raster.
The following table summarizes these parameters:
Parameter | Description |
---|---|
src_files | List of raster datasets to merge |
method | Resampling method for merging |
nodata | Value to represent no data |
Example: Merging Raster Files
Below is a complete example demonstrating the merging of two raster files:
python
import rasterio
from rasterio.merge import merge
# Open raster files
src_files_to_mosaic = [rasterio.open(file) for file in [‘raster1.tif’, ‘raster2.tif’]]
# Merge the rasters
mosaic, out_trans = merge(src_files_to_mosaic)
# Save the merged raster
with rasterio.open(‘merged_raster.tif’, ‘w’, driver=’GTiff’,
height=mosaic.shape[1], width=mosaic.shape[2],
count=1, dtype=mosaic.dtype,
crs=src_files_to_mosaic[0].crs,
transform=out_trans) as dst:
dst.write(mosaic, 1)
This code will successfully merge the specified raster files into a new file named `merged_raster.tif`.
Understanding the Merge Raster Function
The merge raster function is essential for combining multiple raster datasets into a single raster file. This function can be particularly useful in geographic information systems (GIS) and remote sensing applications where data from different sources need to be integrated.
Using Rasterio for Merging Rasters
One of the most popular libraries for handling raster data in Python is Rasterio. The `merge` function provided by Rasterio allows for efficient merging of raster files. Below are the steps to utilize this functionality:
- Install Rasterio: Ensure that you have Rasterio installed in your Python environment. You can install it using pip:
bash
pip install rasterio
- Import Required Libraries: Begin your Python script by importing the necessary libraries.
python
import rasterio
from rasterio.merge import merge
- Read Raster Files: Load the raster files you intend to merge. This can be done using a context manager to ensure proper handling.
python
files_to_merge = [‘file1.tif’, ‘file2.tif’, ‘file3.tif’]
src_files_to_mosaic = [rasterio.open(fp) for fp in files_to_merge]
- Merge the Rasters: Use the `merge` function to combine the raster datasets.
python
mosaic, out_trans = merge(src_files_to_mosaic)
- Save the Merged Raster: Finally, write the merged raster to a new file.
python
with rasterio.open(‘merged_output.tif’, ‘w’, driver=’GTiff’,
height=mosaic.shape[1],
width=mosaic.shape[2],
count=1,
dtype=mosaic.dtype,
crs=src_files_to_mosaic[0].crs,
transform=out_trans) as dst:
dst.write(mosaic, 1)
Handling Different Raster Properties
When merging rasters, discrepancies in spatial resolution, coordinate reference systems (CRS), and data types may occur. The following considerations should be taken into account:
- Spatial Resolution: Ensure that all rasters have the same resolution. If they differ, consider resampling the rasters before merging.
- Coordinate Reference Systems: All input rasters should be in the same CRS. If they are not, reproject the rasters using `rasterio.warp`.
- Data Types: Check that the data types are compatible. If they are not, convert them appropriately before merging.
Example of Resampling and Reprojecting
In scenarios where rasters need to be resampled or reprojected, the following code snippets can be employed:
- Resampling:
python
from rasterio.enums import Resampling
with rasterio.open(‘input_file.tif’) as src:
data = src.read(
out_shape=(
src.count,
int(src.height * 0.5),
int(src.width * 0.5),
),
resampling=Resampling.bilinear,
)
- Reprojecting:
python
from rasterio.warp import calculate_default_transform, reproject, Resampling
with rasterio.open(‘input_file.tif’) as src:
transform, width, height = calculate_default_transform(
src.crs, target_crs, src.width, src.height, *src.bounds)
kwargs = src.meta.copy()
kwargs.update({
‘crs’: target_crs,
‘transform’: transform,
‘width’: width,
‘height’: height,
})
with rasterio.open(‘reprojected_file.tif’, ‘w’, **kwargs) as dst:
for i in range(1, src.count + 1):
reproject(
source=rasterio.band(src, i),
destination=rasterio.band(dst, i),
src_transform=src.transform,
src_crs=src.crs,
dst_transform=transform,
dst_crs=target_crs,
resampling=Resampling.nearest)
This structured approach allows for effective utilization of the merge raster function in Python, particularly using the Rasterio library, while addressing common issues associated with raster data integration.
Expert Insights on Using the Merge Raster Function in Python
Dr. Emily Chen (Geospatial Data Scientist, GeoAnalytics Corp). The merge raster function in Python is a powerful tool for combining multiple raster datasets into a single coherent dataset. It is essential to ensure that the rasters are aligned properly in terms of their coordinate systems and resolutions. Utilizing libraries like Rasterio or GDAL can streamline this process significantly, allowing for efficient handling of large datasets.
Michael Thompson (Remote Sensing Specialist, Earth Observation Institute). When using the merge raster function, one must pay attention to the data types of the rasters being merged. Inconsistencies can lead to errors or unexpected results. I recommend preprocessing the rasters to ensure they share the same data type and extent, which will facilitate a smoother merging process.
Sarah Patel (Environmental Analyst, GreenTech Solutions). The merge raster function is not only useful for combining datasets but also for enhancing data analysis capabilities. By merging rasters, analysts can create comprehensive environmental models. I suggest leveraging Python’s NumPy alongside raster libraries to perform additional calculations post-merge, which can yield deeper insights into the data.
Frequently Asked Questions (FAQs)
What is the purpose of the merge raster function in Python?
The merge raster function in Python is used to combine multiple raster datasets into a single raster file. This is particularly useful for creating a seamless representation of spatial data from various sources.
Which libraries in Python can be used to merge raster files?
Popular libraries for merging raster files in Python include Rasterio, GDAL, and Geopandas. Each library offers functions that facilitate the merging process effectively.
How do I install the necessary libraries for merging rasters?
You can install the required libraries using pip. For example, use the command `pip install rasterio` or `pip install gdal` to install Rasterio and GDAL, respectively.
Can you provide a basic example of how to use the merge raster function?
Certainly. Using Rasterio, you can merge rasters with the following code snippet:
python
import rasterio
from rasterio.merge import merge
import glob
# List of raster files to merge
raster_files = glob.glob(‘path/to/rasters/*.tif’)
# Open the raster files
src_files_to_mosaic = [rasterio.open(fp) for fp in raster_files]
# Merge the rasters
mosaic, out_trans = merge(src_files_to_mosaic)
# Save the merged raster
with rasterio.open(‘merged_output.tif’, ‘w’, **src_files_to_mosaic[0].meta) as dest:
dest.write(mosaic)
What are the common issues encountered when merging rasters?
Common issues include differing coordinate reference systems (CRS), varying pixel sizes, and data type mismatches. It is essential to ensure that all rasters share the same CRS and resolution before merging.
Is it possible to merge rasters with different resolutions?
Yes, it is possible to merge rasters with different resolutions, but it requires resampling the rasters to a common resolution before merging. This can be done using the `rasterio.warp` module or similar functionality in other libraries.
The merge raster function in Python is a powerful tool for combining multiple raster datasets into a single cohesive dataset. This function is particularly useful in geographic information systems (GIS) and remote sensing applications where users often need to analyze and visualize data from various sources. The primary libraries used for this purpose include Rasterio, GDAL, and Geopandas, each offering unique functionalities and capabilities for raster data manipulation.
To effectively use the merge raster function, users typically begin by importing the necessary libraries and loading the raster files they wish to merge. The process involves reading the individual raster datasets, aligning them based on their spatial reference systems, and then combining them into a single output raster. It is crucial to ensure that the rasters share the same coordinate reference system to avoid discrepancies in the merged output.
Key takeaways from the discussion include the importance of preprocessing raster data, such as clipping or reprojecting, to ensure compatibility before merging. Additionally, understanding the parameters and options available in the merge functions can enhance the final output quality and performance. Lastly, users should be aware of the potential challenges, such as handling large datasets and managing memory usage during the merging process.
Author Profile
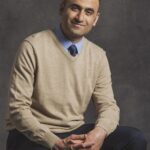
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?