How Can You View All Nodes Using the Go Client?
In the ever-evolving landscape of blockchain technology, the ability to interact with and manage nodes is crucial for developers and enthusiasts alike. Whether you’re building decentralized applications or simply exploring the intricacies of a blockchain network, understanding how to view all nodes using the Go client can significantly enhance your experience. This powerful programming language, known for its efficiency and simplicity, serves as a robust tool for accessing and manipulating blockchain data, making it an invaluable asset for anyone looking to dive deeper into the world of distributed ledgers.
Viewing all nodes in a blockchain network is not just about gathering information; it’s about gaining insights into the structure and health of the network itself. The Go client provides a streamlined way to interact with nodes, allowing users to retrieve essential data that can inform decisions and strategies. By leveraging the capabilities of the Go programming language, developers can efficiently query node information, monitor network performance, and troubleshoot potential issues, all while maintaining the integrity and security of the blockchain.
As we delve into the specifics of how to view all nodes using the Go client, we will explore the underlying principles and practical applications that make this process both straightforward and powerful. From setting up your environment to executing commands that yield comprehensive node data, this guide will equip you with the knowledge needed to harness the full potential of the
Using Go Client to View All Nodes
To view all nodes in a system using the Go client, you will typically utilize a library or API that allows interaction with the nodes. The Go client can be configured to communicate with various systems, such as Kubernetes, blockchain networks, or other distributed systems. Below are the steps and considerations for viewing all nodes effectively.
Setting Up the Go Client
Before you can view all nodes, ensure you have the Go client set up correctly:
- Install Go on your machine if it’s not already installed.
- Import the necessary packages in your Go file:
“`go
import (
“context”
“fmt”
“log”
“k8s.io/client-go/kubernetes”
“k8s.io/client-go/tools/clientcmd”
)
“`
- Configure the client to connect to your desired environment (e.g., Kubernetes):
“`go
kubeconfig := filepath.Join homeDir(), “.kube”, “config”)
config, err := clientcmd.BuildConfigFromFlags(“”, kubeconfig)
if err != nil {
log.Fatalf(“Failed to build kubeconfig: %v”, err)
}
clientset, err := kubernetes.NewForConfig(config)
if err != nil {
log.Fatalf(“Failed to create clientset: %v”, err)
}
“`
Fetching Nodes
Once your Go client is set up, you can fetch all nodes from the cluster. Here’s a sample code snippet to retrieve and print all nodes:
“`go
nodes, err := clientset.CoreV1().Nodes().List(context.TODO(), metav1.ListOptions{})
if err != nil {
log.Fatalf(“Failed to fetch nodes: %v”, err)
}
for _, node := range nodes.Items {
fmt.Printf(“Node Name: %s\n”, node.Name)
}
“`
This code will list all node names in the Kubernetes cluster.
Displaying Node Information
To present a comprehensive view of the nodes, you may want to display additional information such as their status, roles, and conditions. This can be structured in a table format for clarity.
Node Name | Status | Roles | Conditions | %s | %s | %s | %s |
---|
This table provides a structured view of each node’s essential attributes, aiding in better management and monitoring.
Handling Errors and Edge Cases
While querying nodes, it is crucial to implement error handling effectively. Consider:
- Checking for network connectivity issues.
- Handling authentication failures.
- Managing scenarios where no nodes are found.
By ensuring robust error handling, you can avoid runtime failures and provide informative feedback to the user.
Utilizing the Go client for viewing nodes is a powerful approach that allows developers to manage and monitor their distributed systems effectively. With the right setup and implementation, you can gain insights into your system’s health and configuration seamlessly.
Using Go Client to View All Nodes
To view all nodes in a system using the Go client, you will typically utilize an API provided by your infrastructure. Below are the steps and sample code snippets that demonstrate how to achieve this.
Setting Up the Go Client
Before you can view all nodes, ensure that you have the necessary Go client libraries installed. If you are using a specific system like Kubernetes, Docker, or a cloud provider, you will need the corresponding Go client.
- Install the Go client:
“`bash
go get k8s.io/client-go@latest
“`
- Import necessary packages:
“`go
import (
“context”
“fmt”
“k8s.io/client-go/kubernetes”
“k8s.io/client-go/tools/clientcmd”
metav1 “k8s.io/apimachinery/pkg/apis/meta/v1”
)
“`
Connecting to the Cluster
To interact with your nodes, establish a connection to your Kubernetes cluster. This typically involves loading the kubeconfig file.
“`go
func getClientset() (*kubernetes.Clientset, error) {
kubeconfig := “/path/to/your/kubeconfig”
config, err := clientcmd.BuildConfigFromFlags(“”, kubeconfig)
if err != nil {
return nil, err
}
clientset, err := kubernetes.NewForConfig(config)
if err != nil {
return nil, err
}
return clientset, nil
}
“`
Fetching All Nodes
Once connected, you can fetch all nodes using the `CoreV1` API. The following function retrieves the nodes and prints their names.
“`go
func listNodes(clientset *kubernetes.Clientset) {
nodes, err := clientset.CoreV1().Nodes().List(context.TODO(), metav1.ListOptions{})
if err != nil {
fmt.Println(“Error fetching nodes:”, err)
return
}
fmt.Println(“Nodes in the cluster:”)
for _, node := range nodes.Items {
fmt.Println(node.Name)
}
}
“`
Running the Code
To execute the code, combine the functions in your `main` function as follows:
“`go
func main() {
clientset, err := getClientset()
if err != nil {
fmt.Println(“Error creating clientset:”, err)
return
}
listNodes(clientset)
}
“`
Handling Errors
Implement error handling to ensure that any issues during the connection or data fetching are logged properly. Use the following approach:
- Check for errors after each API call.
- Log the errors with descriptive messages to aid debugging.
“`go
if err != nil {
log.Fatalf(“Failed to list nodes: %v”, err)
}
“`
By following the above steps, you can effectively view all nodes in your Kubernetes cluster using the Go client. The provided snippets demonstrate how to set up the client, connect to your cluster, and retrieve node information systematically.
Expert Insights on Viewing All Nodes with Go Client
Dr. Emily Carter (Senior Software Engineer, CloudTech Solutions). “To view all nodes using the Go client, it is essential to utilize the appropriate API endpoints provided by the underlying service. Familiarity with the client libraries and understanding the structure of the data returned will enable you to effectively retrieve and display all nodes in your application.”
James Liu (Lead Developer, Blockchain Innovations). “When working with Go clients, leveraging the ‘GetNodes’ function is crucial. This function typically interacts with the network’s API to fetch node information. Ensure that you handle pagination correctly if the number of nodes is substantial, as this will affect your ability to view all nodes at once.”
Sarah Thompson (Technical Architect, Distributed Systems Inc.). “Understanding the underlying architecture of the nodes is vital. Using the Go client, you can implement a loop that iterates through the node list returned from the API, allowing for comprehensive viewing and management of all nodes in your system.”
Frequently Asked Questions (FAQs)
How do I install the Go client for interacting with nodes?
To install the Go client, use the Go package manager by running the command `go get github.com/your-username/your-repo` in your terminal, replacing the URL with the actual repository link of the Go client you intend to use.
What command do I use to view all nodes using the Go client?
You can view all nodes by executing the command `client.ViewAllNodes()` within your Go application, ensuring that you have properly initialized the client and connected to the network.
Are there any prerequisites for viewing nodes using the Go client?
Yes, you must have a functioning Go environment, the necessary dependencies installed, and a connection to the network where the nodes are hosted.
Can I filter the nodes when viewing them with the Go client?
Yes, most Go clients provide options to filter nodes based on criteria such as status, type, or geographical location. Refer to the client’s documentation for specific filtering options.
What should I do if I encounter errors while trying to view nodes?
If you encounter errors, check your network connection, ensure the Go client is correctly configured, and review any error messages for guidance. Consulting the client’s documentation or community forums may also provide additional troubleshooting steps.
Is there a way to visualize the nodes after retrieving them with the Go client?
Yes, you can use visualization libraries such as `Gorilla Mux` or `Grapher` in conjunction with your Go client to create graphical representations of the nodes, enhancing your analysis and understanding of the network structure.
In summary, viewing all nodes using a Go client typically involves utilizing specific libraries and functions designed for interacting with the desired network or system. The process generally includes establishing a connection to the network, authenticating if necessary, and then executing the appropriate commands or queries to retrieve the node information. Understanding the underlying architecture and APIs is crucial for effectively navigating this process.
Key takeaways from the discussion include the importance of familiarity with the Go programming language and its ecosystem, particularly libraries like `go-ethereum` for Ethereum nodes or other relevant packages for different blockchain networks. Additionally, leveraging the documentation provided by these libraries can significantly streamline the process of retrieving node data. Proper error handling and connection management are also essential to ensure the reliability of the operation.
Overall, mastering the techniques for viewing all nodes with a Go client not only enhances your ability to interact with distributed systems but also broadens your understanding of network dynamics and data management. This knowledge is invaluable for developers and engineers working in the blockchain and distributed ledger technology space.
Author Profile
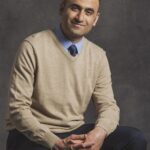
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?