Can Python Scripts Be Run Using Only Absolute File Paths?
### Introduction
In the world of programming, file paths are the unsung heroes that guide scripts to their intended destinations. When it comes to Python, a language renowned for its versatility and ease of use, understanding how file paths work can significantly enhance your coding experience. One question that often arises among developers is whether Python scripts can run using only absolute file paths. This inquiry opens the door to a deeper exploration of file handling, path management, and the nuances of executing scripts in various environments. Join us as we unravel the complexities of file paths in Python and discover how they influence your coding practices.
### Overview
File paths serve as the roadmap for Python scripts, directing them to the necessary resources and modules required for execution. While absolute file paths provide a definitive location in the file system, the question of their exclusivity in running Python scripts invites a broader discussion about relative paths and their practicality. Understanding the difference between these two types of paths is crucial for developers who want to write flexible and portable code.
Moreover, the choice between absolute and relative paths can significantly impact the ease of script deployment and maintenance. As we delve deeper into this topic, we will examine the circumstances under which Python scripts can operate with absolute paths, the advantages and disadvantages of each approach, and best practices for managing
Understanding File Paths in Python
In Python, scripts can run using both absolute and relative file paths. The distinction between these two types of paths is crucial for understanding how file handling works in Python.
Absolute file paths specify the complete path to a file from the root directory. For example, in a Unix-based system, an absolute path might look like `/home/user/documents/file.txt`. In contrast, a relative file path provides a way to access the file based on the current working directory of the script, such as `documents/file.txt`.
Using Absolute Paths
While Python scripts can utilize absolute paths, there are several considerations:
- Portability: Absolute paths can limit the portability of scripts. If a script is shared between different environments, the absolute path may not exist on another system, leading to errors.
- Dependency on Directory Structure: Scripts relying on absolute paths require the exact directory structure to remain unchanged across environments.
Using Relative Paths
Relative paths are often more flexible and are preferred in many scenarios:
- Environment Agnostic: They allow scripts to run in different environments without modification.
- Ease of Use: Developers can easily move scripts and their associated files without worrying about adjusting file paths.
Here’s a quick comparison of absolute and relative paths:
Aspect | Absolute Path | Relative Path |
---|---|---|
Definition | Complete path from the root directory | Path relative to the current directory |
Portability | Low | High |
Usage Example | /home/user/documents/file.txt | documents/file.txt |
Best Practices for Path Management
To manage file paths effectively in Python, consider the following best practices:
- Use `os.path` or `pathlib`: These libraries provide tools to manipulate paths in a platform-independent manner.
- Use configuration files: Store paths in a configuration file, which can be modified without altering the script.
- Leverage environment variables: Use environment variables to define base paths, making it easier to adapt scripts to different environments.
By following these practices, developers can write scripts that are robust and maintainable, minimizing the risk associated with hard-coded file paths.
Understanding File Paths in Python
In Python, scripts can operate using both absolute and relative file paths. Understanding the distinctions between these two types of paths is crucial for effective file management and script execution.
Absolute File Paths
An absolute file path provides the complete directory location of a file from the root of the file system. It specifies the exact location of a file or directory, making it unambiguous.
- Syntax Example:
- On Windows: `C:\Users\Username\Documents\file.txt`
- On macOS/Linux: `/home/username/documents/file.txt`
- Advantages:
- Unambiguous references to files, regardless of the working directory of the script.
- Useful in scripts that need to access files in predefined locations.
Relative File Paths
Relative file paths define the location of a file concerning the current working directory of the script. They are more flexible but can lead to confusion if the working directory changes.
- Syntax Example:
- `documents/file.txt` (if the current working directory is the parent directory of `documents`)
- `../file.txt` (navigates to the parent directory)
- Advantages:
- Easier to use and maintain, particularly when sharing scripts across different environments.
- Reduces the need for hardcoding paths that may vary between users and systems.
Running Python Scripts with Different Path Types
Python scripts can indeed run using both absolute and relative paths. The choice between them depends on the specific requirements of the project and the environment in which it is executed.
Aspect | Absolute Path | Relative Path |
---|---|---|
Clarity | Clear and precise location | Dependent on the current working directory |
Portability | Less portable across different systems | More portable, easier to share |
Risk of Errors | Minimal risk of errors related to paths | Higher risk if working directory changes |
Use Case | Best for scripts needing fixed file locations | Best for scripts that need flexibility |
Best Practices for File Path Usage in Python
To effectively manage file paths in Python, consider the following best practices:
- Use `os.path` or `pathlib`:
Utilize the built-in `os.path` module or `pathlib` for path manipulations. They provide functions to handle paths in a cross-platform manner.
- Verify Current Working Directory:
Always verify the current working directory using `os.getcwd()` when using relative paths to avoid unexpected behavior.
- Exception Handling:
Implement exception handling when working with file paths to gracefully manage errors related to file access.
- Configuration Files:
Store file paths in configuration files or environment variables, allowing for easier updates and better organization.
By understanding and applying these concepts, Python scripts can efficiently utilize both absolute and relative file paths, ensuring robust file management.
Understanding File Path Requirements in Python Scripting
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). Python scripts can run using both absolute and relative file paths. While absolute paths provide a complete route to a file, relative paths allow for more flexibility, especially in collaborative projects where directory structures may vary.
Michael Chen (Lead Python Developer, CodeCraft Solutions). It is not necessary for Python scripts to run exclusively using absolute file paths. Utilizing relative paths can simplify the code and enhance portability, making it easier to share scripts across different environments without hardcoding specific directory locations.
Lisa Tran (Data Scientist, Analytics Hub). While absolute file paths can eliminate ambiguity in locating files, they are not the only option for executing Python scripts. Developers should consider the context in which their scripts will run, as relative paths can often lead to more maintainable and adaptable code.
Frequently Asked Questions (FAQs)
Can Python scripts run only using absolute file paths?
No, Python scripts can run using both absolute and relative file paths. The choice depends on the context in which the script is executed and the current working directory.
What is an absolute file path in Python?
An absolute file path is a complete path from the root of the file system to the desired file or directory. It specifies the exact location without any ambiguity.
What is a relative file path in Python?
A relative file path refers to a location in relation to the current working directory. It does not include the root directory and can vary depending on where the script is executed.
How can I determine the current working directory in Python?
You can determine the current working directory by using the `os.getcwd()` function from the `os` module. This function returns the path of the current working directory.
Are there any advantages to using relative paths in Python scripts?
Yes, using relative paths can make scripts more portable, as they do not depend on the absolute structure of the file system. This allows the scripts to be run from different locations without modification.
Can I mix absolute and relative paths in a single Python script?
Yes, you can mix absolute and relative paths in a Python script. However, it is essential to manage the paths carefully to avoid confusion and ensure the script functions correctly regardless of the working directory.
In summary, Python scripts can run using both absolute and relative file paths. Absolute file paths specify the complete directory location of a file, starting from the root of the filesystem, while relative file paths are based on the current working directory of the script. This flexibility allows developers to choose the most appropriate method for their specific use case, depending on factors such as portability, readability, and the structure of the project.
Utilizing absolute paths can be beneficial in scenarios where the exact location of a file is critical, such as when accessing configuration files or resources that are not located within the project directory. However, this approach may reduce the portability of the script, as the absolute path may not exist on other systems. Conversely, relative paths enhance portability, making it easier to share scripts across different environments without requiring modifications to the file paths.
Ultimately, the choice between absolute and relative paths in Python scripts should be guided by the context in which the script will be executed. Developers should consider the environment, the need for portability, and the ease of maintenance when deciding on the file path method to use. Understanding the implications of each approach will lead to more robust and adaptable Python applications.
Author Profile
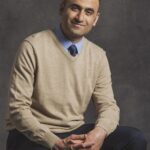
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?