How Can I Center My QR Code in HTML?
In today’s digital landscape, QR codes have become an essential tool for bridging the gap between the physical and virtual worlds. Whether you’re promoting a product, sharing a website, or facilitating easy access to contact information, a well-placed QR code can significantly enhance user engagement. However, simply generating a QR code is just the beginning; presenting it effectively on your website is crucial. One common challenge many web developers face is ensuring that their QR codes are perfectly centered within their HTML layout. If you’ve ever wondered how to achieve that sleek, professional look, you’re in the right place!
Centering a QR code in HTML may seem like a straightforward task, but it involves understanding a few key concepts of CSS and HTML structure. By mastering these techniques, you can ensure that your QR code stands out and is easily accessible to users, regardless of the device they’re using. From utilizing flexbox to employing traditional text alignment methods, there are various strategies to achieve the desired effect.
In this article, we will explore the different approaches to centering QR codes within your web pages. You’ll learn about the importance of responsive design, how to adjust your code for various screen sizes, and the best practices for creating an aesthetically pleasing layout. Whether you’re a seasoned developer or just starting out, these tips will help
Centering a QR Code in HTML
To center a QR code in HTML, you can use a combination of CSS properties that align the image horizontally and vertically within its parent container. There are several methods to achieve this, depending on the layout and structure of your HTML. Below are some common techniques.
Using Flexbox
Flexbox is a powerful layout module that makes it easy to center items in a container. Here’s how to center a QR code using Flexbox:
“`html

“`
“`css
.qr-container {
display: flex;
justify-content: center; /* Aligns horizontally */
align-items: center; /* Aligns vertically */
height: 100vh; /* Full viewport height */
}
.qr-code {
max-width: 100%; /* Ensures responsiveness */
}
“`
In this example, the `.qr-container` div becomes a flex container, centering the QR code both vertically and horizontally.
Using Grid Layout
CSS Grid Layout can also be used to center the QR code. Here’s a simple implementation:
“`html

“`
“`css
.qr-grid {
display: grid;
place-items: center; /* Centers items in both axes */
height: 100vh; /* Full viewport height */
}
.qr-code {
max-width: 100%; /* Ensures responsiveness */
}
“`
The `place-items: center;` property is a shorthand for centering items in both the horizontal and vertical directions.
Using Text Alignment
If you prefer a simpler approach and your QR code is an inline element, you can center it using text alignment. This method is effective for images wrapped in block elements.
“`html

“`
“`css
.text-center {
text-align: center; /* Centers inline elements */
height: 100vh; /* Full viewport height */
display: flex;
justify-content: center; /* Optional for horizontal centering */
align-items: center; /* Optional for vertical centering */
}
“`
While this method is straightforward, it may not perfectly center the image if the container’s height is not explicitly defined.
Comparison Table of Centering Methods
Method | Flexibility | Browser Support | Use Case |
---|---|---|---|
Flexbox | Highly flexible | Modern browsers | Responsive layouts |
Grid Layout | Very flexible | Modern browsers | Complex layouts |
Text Alignment | Limited flexibility | All browsers | Simple layouts |
Each method has its advantages and is suitable for different scenarios. Choose the one that best fits your design requirements and project specifications.
Centering a QR Code in HTML
To effectively center a QR code within your HTML layout, you can utilize various CSS techniques. Here are the most common methods:
Using Flexbox
Flexbox is a modern layout module that allows for easy centering of elements. Here’s how to apply it:
“`html

“`
“`css
.flex-container {
display: flex;
justify-content: center; /* Centers horizontally */
align-items: center; /* Centers vertically */
height: 100vh; /* Full viewport height */
}
.qr-code {
max-width: 100%; /* Responsive resizing */
height: auto; /* Maintain aspect ratio */
}
“`
Using CSS Grid
CSS Grid can also be employed for centering the QR code. The implementation is straightforward:
“`html

“`
“`css
.grid-container {
display: grid;
place-items: center; /* Centers both horizontally and vertically */
height: 100vh; /* Full viewport height */
}
.qr-code {
max-width: 100%; /* Responsive resizing */
height: auto; /* Maintain aspect ratio */
}
“`
Using Margin Auto
A traditional method involves using margins. This approach is particularly useful for block-level elements.
“`html

“`
“`css
.qr-container {
text-align: center; /* Centers inline elements */
}
.qr-code {
display: block; /* Make it a block element */
margin: 0 auto; /* Auto margins for horizontal centering */
max-width: 100%; /* Responsive resizing */
height: auto; /* Maintain aspect ratio */
}
“`
Using Text Align for Inline Images
If your QR code is an inline element, you can center it using `text-align`:
“`html

“`
“`css
.text-center {
text-align: center; /* Centers inline elements */
}
.qr-code {
max-width: 100%; /* Responsive resizing */
height: auto; /* Maintain aspect ratio */
}
“`
Responsive Design Considerations
When centering a QR code, it is crucial to ensure responsiveness. Consider the following tips:
- Use percentages for width or max-width to ensure the QR code scales with different screen sizes.
- Set height to auto to maintain the aspect ratio.
- Test on multiple devices to verify that the QR code remains centered and visually appealing.
By implementing these methods, you can ensure that your QR code is not only centered but also adaptable to various screen sizes, enhancing user experience.
Expert Strategies for Centering QR Codes in HTML
Jessica Lin (Web Development Specialist, CodeCraft Solutions). “To effectively center a QR code in HTML, utilizing CSS Flexbox is a highly efficient method. By applying ‘display: flex’ and ‘justify-content: center’ to the parent container, you ensure that the QR code is perfectly aligned in the center of the page.”
Michael Chen (Senior Front-End Developer, Tech Innovations Inc.). “Another effective approach is to use margin auto in combination with a defined width for the QR code image. Setting the image to ‘display: block’ and using ‘margin: 0 auto’ will center the QR code horizontally within its parent element.”
Linda Garcia (UI/UX Designer, Creative Web Agency). “For responsive designs, consider wrapping your QR code in a div with a text-align property set to center. This method not only centers the QR code but also ensures it remains visually appealing across different screen sizes.”
Frequently Asked Questions (FAQs)
How do I center my QR code in HTML?
To center your QR code in HTML, you can use CSS. Wrap the QR code in a `
Can I use CSS Grid to center my QR code?
Yes, you can use CSS Grid to center your QR code. Set the parent container to `display: grid;` and use `place-items: center;` to center the QR code both vertically and horizontally.
What HTML tags should I use for a QR code?
You can use the `` tag to display your QR code, ensuring to set the `src` attribute to the URL of the QR code image. It is also advisable to include an `alt` attribute for accessibility.
Is it necessary to specify a width for the QR code?
While it is not strictly necessary, specifying a width for the QR code image can help maintain its aspect ratio and ensure it displays correctly across different devices. Use CSS to define the width as needed.
How do I ensure my QR code is responsive?
To make your QR code responsive, set its width to a percentage (e.g., `width: 100%;`) and ensure the height is set to `auto`. This allows the QR code to scale appropriately with the size of its container.
Can I center a QR code using inline styles?
Yes, you can center a QR code using inline styles. Apply `style=”text-align: center;”` to the parent `
Centering a QR code in HTML is a straightforward process that can significantly enhance the visual appeal of a webpage. By utilizing CSS properties such as `display`, `margin`, and `text-align`, developers can effectively position QR codes in the center of their designated containers. This ensures that the QR code is not only visually appealing but also easily accessible for users, which is crucial for its intended functionality.
One of the most common methods to center a QR code is by applying the `text-align: center;` property to the parent container. Additionally, using `margin: auto;` on the QR code image itself can help achieve a perfect centering effect. It is essential to consider the display type of the QR code element; using `block` or `inline-block` can yield different results in centering. Properly structuring the HTML and CSS will lead to a seamless integration of the QR code into the webpage.
In summary, centering a QR code in HTML requires a combination of proper HTML structure and CSS styling techniques. By following best practices and utilizing the right properties, developers can ensure that QR codes are not only centered but also enhance the overall user experience. This attention to detail can make a significant difference in how users
Author Profile
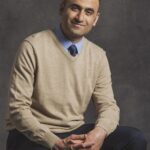
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?