What Are Floats in Python? Understanding Their Role and Usage
In the world of programming, precision and accuracy are paramount, especially when dealing with numerical data. Among the various data types that Python offers, floats stand out as a crucial component for handling real numbers. Whether you’re calculating financial figures, processing scientific data, or developing algorithms that require decimal precision, understanding floats in Python is essential for any aspiring programmer or data scientist. This article will delve into the intricacies of floats, exploring their significance, functionality, and the nuances that come with using them in your Python projects.
Floats, short for floating-point numbers, are a data type in Python that allows for the representation of real numbers, including those that contain a fractional component. Unlike integers, which are whole numbers, floats can express a wide range of values, from very small to very large, making them incredibly versatile. This flexibility is particularly beneficial in fields such as finance and engineering, where calculations often require a high degree of precision and the ability to handle decimal points.
In Python, floats are not just simple representations of numbers; they come with their own set of rules and behaviors that can affect how calculations are performed. Understanding how floats work, including their limitations and potential pitfalls, is vital for writing robust and error-free code. As we explore the topic further,
Understanding Floats in Python
Floats, or floating-point numbers, are a data type in Python that represents real numbers with decimal points. They are utilized to express fractional values, enabling precise calculations involving non-integer numbers. In Python, floats are indicated by the presence of a decimal point or by using scientific notation.
When working with floats, it is important to note their characteristics:
- Precision: Floats can represent numbers with varying degrees of precision, but they are not always exact due to the limitations of binary representation in computers.
- Range: The range of floats is extensive, allowing for representation of very small and very large values. However, the precision may decrease as the magnitude of the number increases.
- Immutable: Like other basic data types in Python, floats are immutable, meaning their values cannot be changed after creation.
Creating Floats
In Python, creating a float is straightforward. You can simply assign a decimal number to a variable, or use scientific notation to represent large numbers. Here are some examples:
“`python
Creating floats
a = 3.14 A simple float
b = 2.0 Another float
c = 1.5e2 Scientific notation (150.0)
d = -0.001 A negative float
“`
You can also convert integers or strings to floats using the built-in `float()` function:
“`python
Converting integers and strings to floats
num1 = float(5) Converts integer to float (5.0)
num2 = float(“3.142”) Converts string to float (3.142)
“`
Operations with Floats
Python supports a variety of arithmetic operations with floats, including addition, subtraction, multiplication, and division. It’s essential to be aware of the potential for precision issues when performing calculations, especially with very large or very small numbers.
Here is a summary of basic arithmetic operations with floats:
Operation | Description | Example | Result |
---|---|---|---|
Addition | Adds two floats | 1.5 + 2.3 | 3.8 |
Subtraction | Subtracts one float from another | 5.0 – 3.2 | 1.8 |
Multiplication | Multiplies two floats | 2.0 * 3.5 | 7.0 |
Division | Divides one float by another | 7.5 / 2.5 | 3.0 |
Precision and Limitations
Despite their versatility, floats have limitations due to their representation in binary. This can lead to unexpected behavior in mathematical operations. For example:
“`python
Example of precision issues
result = 0.1 + 0.2
print(result) Output may not be exactly 0.3
“`
To mitigate precision errors, the `Decimal` module from the `decimal` library can be employed, which provides a way to perform decimal arithmetic with greater precision:
“`python
from decimal import Decimal
Using Decimal for precision
d1 = Decimal(‘0.1’)
d2 = Decimal(‘0.2’)
result = d1 + d2
print(result) Will output 0.3 exactly
“`
This approach is particularly useful in financial applications where precision is paramount.
Floats in Python are a powerful tool for working with real numbers. Understanding their characteristics, how to create them, perform operations, and address precision issues is essential for effective programming and data manipulation.
Understanding Floats in Python
In Python, a float is a data type that represents a number that has a decimal point. Floats can be used to handle real numbers, allowing for a wide range of numerical calculations.
Characteristics of Floats
- Precision: Floats are represented using double precision (64 bits), which allows for a high degree of accuracy but may introduce small rounding errors in calculations.
- Range: The range of float values in Python is approximately 1.7 x 10^(-308) to 1.7 x 10^(308).
- Syntax: Floats can be defined in several ways:
- By including a decimal point (e.g., `3.14`)
- Using exponential notation (e.g., `2.5e3` which equals `2500.0`)
Creating Floats
Floats can be created in Python in various ways:
“`python
Direct assignment
a = 10.5
b = 3.0
From integer division
c = 7 / 2 Result is 3.5
Using float() function
d = float(5) Converts integer 5 to float 5.0
“`
Float Operations
Floats support a variety of arithmetic operations. The following table summarizes these operations:
Operation | Example | Result |
---|---|---|
Addition | `a + b` | `13.5` |
Subtraction | `a – b` | `7.5` |
Multiplication | `a * b` | `31.5` |
Division | `a / b` | `3.5` |
Exponentiation | `a ** 2` | `110.25` |
Common Issues with Floats
When working with floats, several issues may arise:
- Rounding Errors: Due to the binary representation of floats, certain decimal numbers cannot be represented precisely. For example, `0.1 + 0.2` may not equal `0.3`.
- Comparison: Direct comparisons between floats can be unreliable. It is advisable to use a tolerance value for checking equality.
“`python
Example of comparing floats
epsilon = 0.0001
is_equal = abs((0.1 + 0.2) – 0.3) < epsilon Returns True
```
Formatting Floats
Python offers several ways to format float values for output:
- Using f-strings (Python 3.6 and later):
“`python
pi = 3.14159
print(f”Value of pi: {pi:.2f}”) Outputs: Value of pi: 3.14
“`
- Using format() method:
“`python
print(“Value of pi: {:.2f}”.format(pi)) Outputs: Value of pi: 3.14
“`
Floats are a crucial part of numerical computations in Python, and understanding their characteristics and behavior is essential for effective programming.
Understanding Floats in Python: Perspectives from Programming Experts
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “Floats in Python are a crucial data type used to represent real numbers with decimal points. They are particularly useful in scientific computations and financial applications where precision is essential.”
Michael Thompson (Lead Data Scientist, Data Insights LLC). “In Python, floats are implemented using double-precision floating-point format, allowing for a wide range of values. However, developers must be aware of precision limitations that can arise during arithmetic operations.”
Sarah Patel (Python Instructor, Code Academy). “Understanding how to work with floats is fundamental for any Python programmer. Mastery of floats enables effective handling of calculations, especially in fields like data analysis and machine learning.”
Frequently Asked Questions (FAQs)
What are floats in Python?
Floats in Python are a data type used to represent real numbers that contain decimal points. They can represent both positive and negative values and are often used for precise calculations.
How do you create a float in Python?
A float can be created by including a decimal point in a number, such as `3.14` or `-0.001`. You can also use the `float()` function to convert an integer or a string to a float.
What is the difference between floats and integers in Python?
Floats represent real numbers with decimal points, while integers are whole numbers without any decimal component. This distinction affects how calculations are performed and the precision of the results.
Can floats be used in mathematical operations in Python?
Yes, floats can be used in all standard mathematical operations, including addition, subtraction, multiplication, and division. Python automatically handles the conversion between floats and integers as needed.
What is the precision of floats in Python?
Floats in Python are typically implemented using double-precision (64-bit) format, which provides a precision of about 15 to 17 decimal digits. However, this can lead to rounding errors in certain calculations.
How can you format floats for display in Python?
Floats can be formatted for display using f-strings, the `format()` method, or the `round()` function. For example, `f”{value:.2f}”` formats a float to two decimal places.
In Python, floats are a fundamental data type used to represent real numbers that contain decimal points. They are essential for performing arithmetic operations that require precision, such as calculations in scientific computing, financial analysis, and data manipulation. Floats in Python follow the IEEE 754 standard for floating-point arithmetic, which allows for a wide range of values, including very small and very large numbers, as well as special values like infinity and NaN (Not a Number).
One of the key characteristics of floats is their ability to represent numbers that cannot be expressed as integers. This flexibility makes them particularly useful in scenarios where exact values are not necessary, but an approximation is sufficient. However, it is important to note that floats can introduce rounding errors due to their binary representation, which can affect calculations that require high precision. Developers should be aware of these limitations and consider using the `decimal` module for situations that demand exact decimal representation.
In summary, understanding floats in Python is crucial for any programmer working with numerical data. They provide a powerful means of handling real numbers, but users must also be mindful of their limitations and potential pitfalls. By leveraging the capabilities of floats effectively, developers can enhance their applications and ensure accurate computations in various domains.
Author Profile
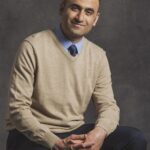
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?