How to Resolve the ‘No Main Manifest Attribute’ Error in Your Java Application?
Have you ever tried to run a Java application, only to be met with the frustrating message: “no main manifest attribute in”? This cryptic error can leave both novice and experienced developers scratching their heads, wondering what went wrong. Understanding this issue is crucial for anyone working with Java, as it can hinder the execution of your applications and stall your development process. In this article, we will unravel the mystery behind this error, exploring its causes, implications, and how to effectively resolve it.
Overview
The “no main manifest attribute” error typically arises when a Java Archive (JAR) file is executed without the necessary entry point specified in its manifest file. The manifest file is a crucial component of JAR files, containing metadata about the archive, including the main class that should be executed. Without this information, the Java Runtime Environment (JRE) cannot determine which class to run, resulting in the error message that can be a source of confusion.
In the world of Java development, encountering this error is not uncommon, especially for those new to packaging and distributing applications. Understanding the structure of JAR files and the role of the manifest is essential for troubleshooting this issue. By delving into the specifics of how to properly configure your JAR files, you
Understanding the Error Message
When you encounter the error message “no main manifest attribute in,” it typically arises when trying to execute a JAR (Java Archive) file that lacks the necessary metadata in its manifest file. This manifest file is crucial as it defines the main class of the application, which contains the `main` method that serves as the entry point for execution.
The manifest file is located in the `META-INF` directory of the JAR and is named `MANIFEST.MF`. For a JAR file to be executable, the manifest must include the following attribute:
“`
Main-Class: your.package.MainClass
“`
If this attribute is missing, the Java Runtime Environment (JRE) does not know which class to execute, resulting in the aforementioned error.
Common Causes of the Error
The error can occur due to several reasons:
- Missing Manifest File: The JAR was created without a manifest file.
- Incorrect Manifest File: The manifest file exists but does not specify a `Main-Class`.
- Invalid JAR Structure: The JAR file is improperly structured, preventing the JRE from locating the manifest.
How to Fix the Error
To resolve the “no main manifest attribute in” error, consider the following steps:
- Check the JAR Structure: Use the command `jar tf yourfile.jar` to list the contents. Ensure that `META-INF/MANIFEST.MF` is present.
- Inspect the Manifest File: If the manifest file is present, check its contents for the `Main-Class` attribute.
- Repackage the JAR: If the manifest is missing or incorrect, you may need to create a new JAR with the correct manifest.
Creating a Manifest File
When creating a JAR file, you can specify a manifest file manually. Here’s how to create a simple manifest file:
- Create a text file named `manifest.txt` with the following content:
“`
Manifest-Version: 1.0
Main-Class: your.package.MainClass
“`
- Use the following command to create the JAR with the specified manifest:
“`
jar cfm yourfile.jar manifest.txt -C path/to/classes .
“`
This command combines the manifest file with the compiled classes in the specified directory.
Example of a Correctly Structured JAR
A well-structured JAR file should include:
- The compiled Java classes
- The `META-INF` directory
- The `MANIFEST.MF` file within `META-INF`
Here’s a table summarizing the structure:
File/Directory | Description |
---|---|
yourfile.jar | The main JAR file |
META-INF/ | Contains the manifest file |
META-INF/MANIFEST.MF | Defines the main class and other metadata |
your/package/MainClass.class | The compiled Java class with the main method |
By ensuring that the manifest file is correctly specified and included in the JAR, the “no main manifest attribute in” error can be effectively resolved, allowing for successful execution of the Java application.
Understanding the Error
The error message “no main manifest attribute in” typically occurs when attempting to run a JAR (Java Archive) file that lacks a designated entry point. This situation arises when the JAR file does not contain a `MANIFEST.MF` file, or the `MANIFEST.MF` file does not specify the `Main-Class` attribute.
Causes of the Error
- Missing `MANIFEST.MF` file: The JAR file may not have been created correctly, resulting in the absence of this essential file.
- Incorrect `Main-Class` specification: The `MANIFEST.MF` file may exist, but the `Main-Class` entry is either missing or incorrectly defined.
- JAR file corruption: The JAR file might be corrupted, causing it to malfunction when executed.
Resolving the Issue
To resolve the “no main manifest attribute in” error, follow these steps:
- Check the JAR file: Ensure that the JAR file is indeed valid and not corrupted. You can do this by:
- Opening the JAR file with a compression tool (e.g., WinRAR, 7-Zip) to verify its contents.
- Checking for the presence of the `META-INF` directory and the `MANIFEST.MF` file within it.
- Examine the `MANIFEST.MF` file: If the file exists, verify its contents. It should include a line specifying the `Main-Class`, such as:
“`
Main-Class: com.example.MainClass
“`
- Recreate the JAR file: If the `MANIFEST.MF` is missing or incorrect, recreate the JAR file using the correct commands. Use the following syntax:
“`bash
jar cfm YourApp.jar MANIFEST.MF -C path/to/classes .
“`
Ensure that `MANIFEST.MF` contains the correct `Main-Class` entry.
Creating a Proper Manifest File
When creating your `MANIFEST.MF` file, make sure it is formatted correctly. Below is a sample template for a proper manifest file:
Attribute | Value |
---|---|
Main-Class | com.example.MainClass |
Class-Path | libs/some-library.jar |
Steps to Create a Manifest File
- Create a new text file named `MANIFEST.MF`.
- Add the `Main-Class` and any other necessary attributes.
- Save the file in plain text format, ensuring there are no extra spaces or lines.
Compiling and Packaging Java Applications
When compiling and packaging Java applications, follow these guidelines to avoid manifest-related errors:
- Compile your Java files: Use the Java compiler to compile your source files:
“`bash
javac -d path/to/classes src/com/example/*.java
“`
- Package into a JAR: Use the `jar` command to package your compiled classes along with the `MANIFEST.MF`:
“`bash
jar cfm YourApp.jar MANIFEST.MF -C path/to/classes .
“`
- Run the JAR file: Finally, execute your JAR file using the `java` command:
“`bash
java -jar YourApp.jar
“`
By following these steps and ensuring that the manifest file is correctly configured, you can eliminate the “no main manifest attribute in” error and successfully run your Java applications.
Understanding the “No Main Manifest Attribute” Error in Java Applications
Dr. Alice Thompson (Senior Software Engineer, Tech Innovations Inc.). “The ‘no main manifest attribute’ error typically arises when the JAR file does not specify the entry point for the application. To resolve this, ensure that your JAR file’s manifest includes the ‘Main-Class’ attribute, pointing to the class containing the main method.”
Mark Chen (Java Development Specialist, CodeCraft Academy). “This error is a common pitfall for Java developers. It serves as a reminder to double-check the build process and the manifest file. A well-defined manifest is crucial for the JAR file to execute correctly.”
Linda Patel (Lead Java Consultant, Enterprise Solutions Group). “When encountering the ‘no main manifest attribute’ error, I advise developers to revisit their project structure. Ensuring that the manifest file is correctly generated during the build process can save significant debugging time.”
Frequently Asked Questions (FAQs)
What does “no main manifest attribute” mean?
The error message “no main manifest attribute” indicates that the Java Runtime Environment (JRE) cannot find the main class to execute within a JAR file. This typically occurs when the JAR file’s manifest does not specify the entry point for the application.
How can I fix the “no main manifest attribute” error?
To resolve this error, ensure that the JAR file contains a manifest file with the `Main-Class` attribute defined. You can create or edit the manifest file and include the line `Main-Class: your.package.MainClass`, where `your.package.MainClass` is the fully qualified name of your main class.
What is a manifest file in a JAR?
A manifest file in a JAR is a special file that contains metadata about the JAR, including information about the version, the main class to execute, and any other attributes. It is located in the `META-INF` directory of the JAR.
How do I create a manifest file?
To create a manifest file, you can use a text editor to create a file named `MANIFEST.MF`. Include the necessary attributes, such as `Main-Class`, and then package it with your JAR using the `jar` command: `jar cfm yourfile.jar MANIFEST.MF -C your/classes/directory .`.
Can I run a JAR file without a main manifest attribute?
No, you cannot directly run a JAR file without a main manifest attribute specifying the main class. You can, however, extract the JAR and run the main class directly using the `java` command, specifying the classpath.
What tools can help with JAR file creation and management?
Several tools can assist with JAR file creation and management, including the Java Development Kit (JDK) command-line tools like `jar`, integrated development environments (IDEs) like Eclipse or IntelliJ IDEA, and build automation tools like Maven or Gradle.
The phrase “no main manifest attribute in” typically arises in the context of Java applications packaged as JAR files. This error indicates that the Java Runtime Environment (JRE) cannot locate the entry point for the application, which is usually specified in the manifest file of the JAR. The absence of a main class declaration in the manifest file leads to the inability of the JRE to execute the program, resulting in the error message. Understanding this issue is crucial for developers to ensure that their applications run smoothly when distributed as JAR files.
To resolve the “no main manifest attribute in” error, developers must ensure that their JAR files include a properly configured manifest file. The manifest file should specify the main class using the “Main-Class” attribute. This can be achieved by either manually editing the manifest file or using build tools such as Maven or Gradle, which can automate the process. Proper configuration not only facilitates the execution of the application but also enhances the overall user experience.
In summary, the “no main manifest attribute in” error serves as a reminder of the importance of correct JAR file configuration in Java applications. By ensuring that the manifest file is properly set up with the necessary attributes, developers can avoid this common pit
Author Profile
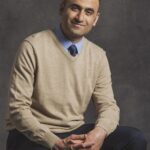
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?